Comprehensive Guide to Matplotlib.axis.Axis.get_pickradius() Function in Python
Matplotlib.axis.Axis.get_pickradius() function in Python is an essential tool for customizing the interactive behavior of plots in Matplotlib. This function allows you to retrieve the pick radius used for mouse-click events on axis lines. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.get_pickradius() function in depth, covering its usage, parameters, return values, and practical applications. We’ll also provide numerous examples to illustrate how this function can be utilized effectively in various scenarios.
Understanding the Matplotlib.axis.Axis.get_pickradius() Function
The Matplotlib.axis.Axis.get_pickradius() function is a method of the Axis class in Matplotlib. It is used to get the pick radius for mouse-click events on axis lines. The pick radius determines the distance from the axis line within which a mouse click will be considered as a pick event. This function is particularly useful when you want to create interactive plots where users can select or interact with specific parts of the axis.
Let’s start with a simple example to demonstrate how to use the Matplotlib.axis.Axis.get_pickradius() function:
import matplotlib.pyplot as plt
# Create a simple plot
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
# Get the pick radius for the x-axis
x_pick_radius = ax.xaxis.get_pickradius()
print(f"X-axis pick radius: {x_pick_radius}")
# Get the pick radius for the y-axis
y_pick_radius = ax.yaxis.get_pickradius()
print(f"Y-axis pick radius: {y_pick_radius}")
plt.legend()
plt.show()
Output:
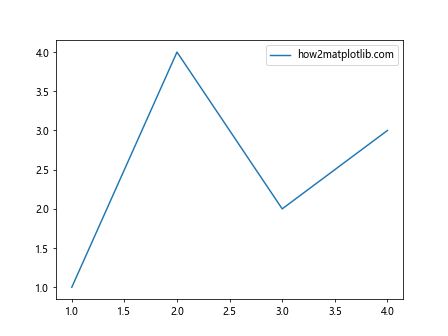
In this example, we create a simple line plot and then use the Matplotlib.axis.Axis.get_pickradius() function to retrieve the pick radius for both the x-axis and y-axis. The function is called on the xaxis and yaxis attributes of the Axes object.
Parameters of Matplotlib.axis.Axis.get_pickradius()
The Matplotlib.axis.Axis.get_pickradius() function doesn’t take any parameters. It’s a simple getter method that returns the current pick radius value for the axis.
Return Value of Matplotlib.axis.Axis.get_pickradius()
The Matplotlib.axis.Axis.get_pickradius() function returns a float value representing the pick radius in points. This value determines the distance from the axis line within which a mouse click will be considered as a pick event.
Let’s look at another example to illustrate how we can use the returned value:
import matplotlib.pyplot as plt
# Create a plot with multiple subplots
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(8, 8))
# Plot data in both subplots
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
ax2.plot([1, 2, 3, 4], [3, 1, 4, 2], label='how2matplotlib.com')
# Get and print pick radius for all axes
axes = [ax1.xaxis, ax1.yaxis, ax2.xaxis, ax2.yaxis]
for i, axis in enumerate(axes):
pick_radius = axis.get_pickradius()
print(f"Subplot {i//2 + 1}, {'X' if i%2 == 0 else 'Y'}-axis pick radius: {pick_radius}")
plt.tight_layout()
plt.show()
Output:
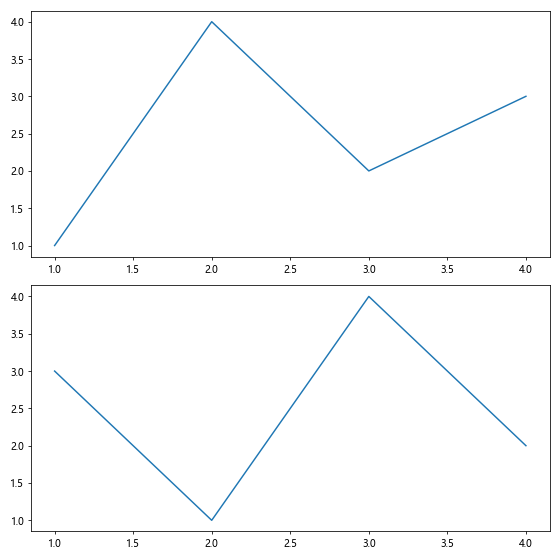
In this example, we create a figure with two subplots and use Matplotlib.axis.Axis.get_pickradius() to retrieve the pick radius for all axes. This demonstrates how the function can be used across multiple subplots and axes.
Practical Applications of Matplotlib.axis.Axis.get_pickradius()
The Matplotlib.axis.Axis.get_pickradius() function is particularly useful in scenarios where you need to create interactive plots or customize the behavior of mouse events near axis lines. Here are some practical applications:
- Interactive Data Selection: You can use the pick radius to allow users to select data points near the axis lines.
Custom Tooltips: Create custom tooltips that appear when the user hovers near axis tick marks.
Axis Line Highlighting: Implement highlighting of axis lines when the mouse is within the pick radius.
Event Handling: Use the pick radius in combination with event handlers to create responsive plots.
Let’s explore these applications with some examples: