Comprehensive Guide to Matplotlib.axis.Axis.get_rasterized() Function in Python
Matplotlib.axis.Axis.get_rasterized() function in Python is an essential tool for managing the rasterization of axis elements in Matplotlib plots. This function allows developers to determine whether an axis is set to be rasterized or not, providing crucial information for optimizing plot rendering and file size. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.get_rasterized() function in depth, covering its usage, benefits, and practical applications in various scenarios.
Understanding Matplotlib.axis.Axis.get_rasterized() Function
The Matplotlib.axis.Axis.get_rasterized() function is a method of the Axis class in Matplotlib. It returns a boolean value indicating whether the axis is set to be rasterized. Rasterization is the process of converting vector graphics into a bitmap image, which can be beneficial for reducing file size and improving rendering performance in certain situations.
Let’s start with a simple example to demonstrate how to use the Matplotlib.axis.Axis.get_rasterized() function:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Check if the x-axis is rasterized
is_rasterized = ax.xaxis.get_rasterized()
print(f"Is x-axis rasterized? {is_rasterized}")
plt.title("Matplotlib.axis.Axis.get_rasterized() Example")
plt.legend()
plt.show()
Output:
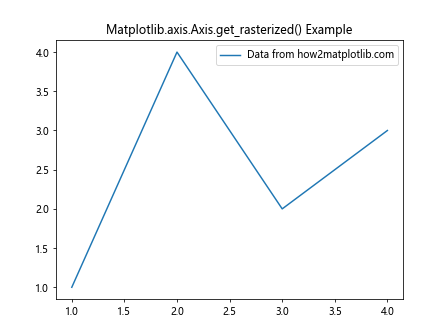
In this example, we create a simple plot and use the get_rasterized() function to check if the x-axis is rasterized. By default, axes are not rasterized, so the function will return False.
Benefits of Using Matplotlib.axis.Axis.get_rasterized() Function
The Matplotlib.axis.Axis.get_rasterized() function offers several benefits for developers working with Matplotlib:
- Performance optimization: By checking the rasterization status, you can make informed decisions about whether to rasterize certain elements to improve rendering performance.
File size management: Rasterization can help reduce file size, especially for complex plots with many vector elements.
Quality control: Knowing whether an axis is rasterized allows you to ensure the desired output quality, especially when working with high-resolution displays or print media.
Debugging: The function can be useful for debugging issues related to plot appearance or export quality.
Let’s explore an example that demonstrates how to use the get_rasterized() function in combination with setting rasterization: