Comprehensive Guide to Matplotlib.axis.Tick.set_rasterized() Function in Python
Matplotlib.axis.Tick.set_rasterized() function in Python is a powerful tool for optimizing plot rendering in Matplotlib. This function allows you to control whether individual tick marks are rasterized or not, which can significantly impact the performance and appearance of your plots. In this comprehensive guide, we’ll explore the Matplotlib.axis.Tick.set_rasterized() function in depth, covering its usage, benefits, and various applications in data visualization.
Understanding the Matplotlib.axis.Tick.set_rasterized() Function
The Matplotlib.axis.Tick.set_rasterized() function is a method of the Tick class in Matplotlib’s axis module. It is used to set the rasterization state of a tick mark. Rasterization is the process of converting vector graphics into a bitmap image. When applied to tick marks, it can help reduce file size and improve rendering performance, especially for plots with a large number of ticks.
Basic Syntax
The basic syntax of the Matplotlib.axis.Tick.set_rasterized() function is as follows:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
tick = ax.xaxis.get_major_ticks()[0]
tick.set_rasterized(True)
plt.title("How to use set_rasterized() - how2matplotlib.com")
plt.show()
Output:
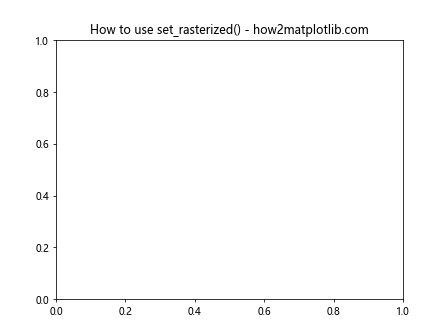
In this example, we set the rasterization state of the first major tick on the x-axis to True. This means that the tick will be rasterized when the plot is rendered.
Benefits of Using Matplotlib.axis.Tick.set_rasterized()
The Matplotlib.axis.Tick.set_rasterized() function offers several benefits:
- Improved performance: Rasterizing tick marks can significantly reduce rendering time for plots with many ticks.
- Reduced file size: Rasterized ticks take up less space in saved files, especially in vector formats like PDF or SVG.
- Better appearance: In some cases, rasterized ticks can look better when zoomed in or printed at high resolutions.
Let’s explore these benefits with some examples.
Example: Improving Performance with Rasterized Ticks
import matplotlib.pyplot as plt
import numpy as np
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 5))
# Plot with non-rasterized ticks
x = np.linspace(0, 10, 1000)
y = np.sin(x)
ax1.plot(x, y)
ax1.set_title("Non-rasterized Ticks - how2matplotlib.com")
# Plot with rasterized ticks
ax2.plot(x, y)
ax2.set_title("Rasterized Ticks - how2matplotlib.com")
for tick in ax2.xaxis.get_major_ticks() + ax2.yaxis.get_major_ticks():
tick.set_rasterized(True)
plt.tight_layout()
plt.show()
Output:
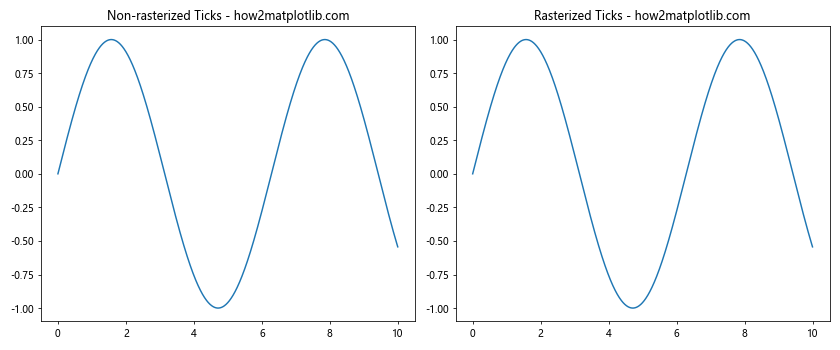
In this example, we create two plots side by side. The left plot has non-rasterized ticks, while the right plot has rasterized ticks. You may notice that the right plot renders faster, especially if you have a large number of ticks.
When to Use Matplotlib.axis.Tick.set_rasterized()
The Matplotlib.axis.Tick.set_rasterized() function is particularly useful in certain scenarios:
- Plots with Many Ticks: If your plot has a large number of ticks, rasterizing them can significantly improve rendering performance and reduce file size.
High-Resolution Outputs: When creating plots for high-resolution prints or displays, rasterized ticks can sometimes look better than vector ticks.
Reducing File Size: If you need to create many plots or embed plots in documents, using rasterized ticks can help keep file sizes manageable.
Let’s look at an example where rasterized ticks are beneficial: