Comprehensive Guide to Matplotlib.axis.Axis.get_tick_space() Function in Python
Matplotlib.axis.Axis.get_tick_space() function in Python is an essential tool for customizing and optimizing the tick spacing in Matplotlib plots. This function is part of the Matplotlib library, which is widely used for creating static, animated, and interactive visualizations in Python. The get_tick_space() function specifically belongs to the Axis class within the axis module of Matplotlib. It plays a crucial role in determining the appropriate spacing between ticks on an axis, which is vital for creating clear and readable plots.
Understanding the Basics of Matplotlib.axis.Axis.get_tick_space()
Matplotlib.axis.Axis.get_tick_space() function in Python is primarily used to retrieve the spacing between major ticks on an axis. This spacing is calculated based on the current axis limits and the size of the axis. Understanding how to use this function effectively can greatly enhance the readability and aesthetics of your plots.
Let’s start with a simple example to demonstrate the basic usage of get_tick_space():
import matplotlib.pyplot as plt
import numpy as np
# Create a simple plot
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y = np.sin(x)
ax.plot(x, y, label='Sine wave')
# Get the tick space for the x-axis
tick_space = ax.xaxis.get_tick_space()
print(f"Tick space on x-axis: {tick_space}")
plt.title('Sine Wave Plot - how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.show()
Output:
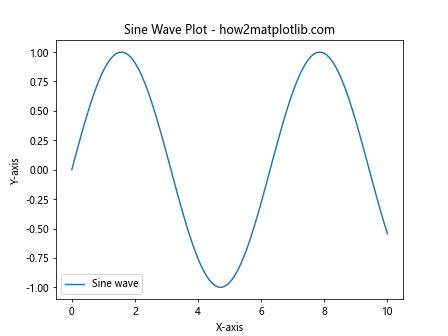
In this example, we create a simple sine wave plot and use get_tick_space() to retrieve the spacing between major ticks on the x-axis. The function returns a float value representing the spacing in data units.
Importance of Matplotlib.axis.Axis.get_tick_space() in Plot Customization
Matplotlib.axis.Axis.get_tick_space() function in Python plays a crucial role in plot customization. It allows developers to understand the current tick spacing and make informed decisions about adjusting it. This is particularly useful when dealing with datasets of varying scales or when creating plots with specific spacing requirements.
Here’s an example that demonstrates how get_tick_space() can be used in conjunction with other Matplotlib functions to customize tick spacing:
import matplotlib.pyplot as plt
import numpy as np
# Create data
x = np.linspace(0, 20, 100)
y = x**2
# Create plot
fig, ax = plt.subplots()
ax.plot(x, y, label='Quadratic function')
# Get current tick space
current_space = ax.xaxis.get_tick_space()
print(f"Current tick space: {current_space}")
# Set new tick locations
new_ticks = np.arange(0, 21, current_space * 2)
ax.set_xticks(new_ticks)
plt.title('Customized Tick Spacing - how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.show()
Output:
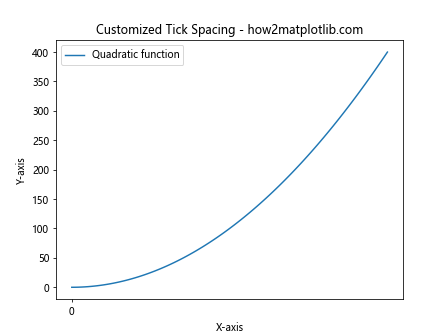
In this example, we use get_tick_space() to determine the current tick spacing, then double it to create new, more spaced-out tick locations. This demonstrates how get_tick_space() can be used as a basis for custom tick spacing.
Advanced Usage of Matplotlib.axis.Axis.get_tick_space()
Matplotlib.axis.Axis.get_tick_space() function in Python can be utilized in more advanced scenarios, such as creating adaptive tick spacing based on the data range or plot size. This can be particularly useful when dealing with dynamic data or responsive plot layouts.
Here’s an example that demonstrates a more advanced use case:
import matplotlib.pyplot as plt
import numpy as np
def adaptive_ticks(ax, axis='x', factor=1.5):
if axis == 'x':
space = ax.xaxis.get_tick_space()
vmin, vmax = ax.get_xlim()
else:
space = ax.yaxis.get_tick_space()
vmin, vmax = ax.get_ylim()
new_space = space * factor
new_ticks = np.arange(vmin, vmax, new_space)
if axis == 'x':
ax.set_xticks(new_ticks)
else:
ax.set_yticks(new_ticks)
# Create data
x = np.linspace(0, 50, 200)
y = np.sin(x) * x
# Create plot
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y, label='Sine * x')
# Apply adaptive ticks
adaptive_ticks(ax, 'x', 2)
adaptive_ticks(ax, 'y', 1.5)
plt.title('Adaptive Tick Spacing - how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
plt.show()
Output:
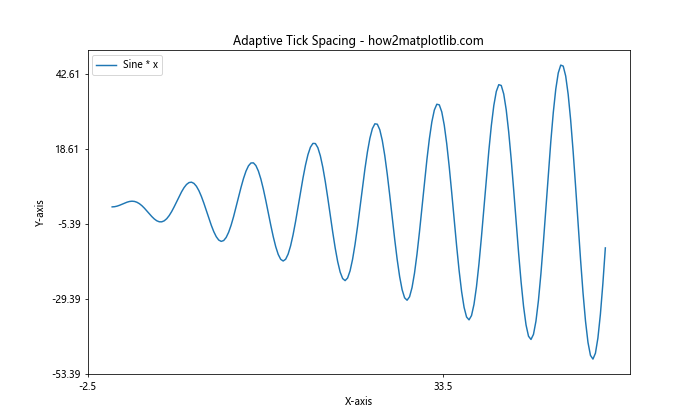
In this advanced example, we create a function called adaptive_ticks
that uses get_tick_space() to calculate new tick locations based on a given factor. This allows for dynamic adjustment of tick spacing on both x and y axes.
Combining Matplotlib.axis.Axis.get_tick_space() with Other Axis Functions
Matplotlib.axis.Axis.get_tick_space() function in Python can be effectively combined with other axis-related functions to achieve more complex customizations. This synergy allows for fine-tuned control over the appearance and behavior of plot axes.
Let’s explore an example that combines get_tick_space() with other axis functions:
import matplotlib.pyplot as plt
import numpy as np
# Create data
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
# Create plot
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(8, 10))
# Plot on first subplot
ax1.plot(x, y1, label='Sine')
ax1.set_title('Sine Wave - how2matplotlib.com')
# Plot on second subplot
ax2.plot(x, y2, label='Cosine')
ax2.set_title('Cosine Wave - how2matplotlib.com')
# Customize ticks using get_tick_space
for ax in (ax1, ax2):
space = ax.xaxis.get_tick_space()
ax.xaxis.set_major_locator(plt.MultipleLocator(space * 1.5))
ax.xaxis.set_minor_locator(plt.MultipleLocator(space / 2))
ax.grid(which='minor', alpha=0.2)
ax.grid(which='major', alpha=0.5)
plt.tight_layout()
plt.show()
Output:
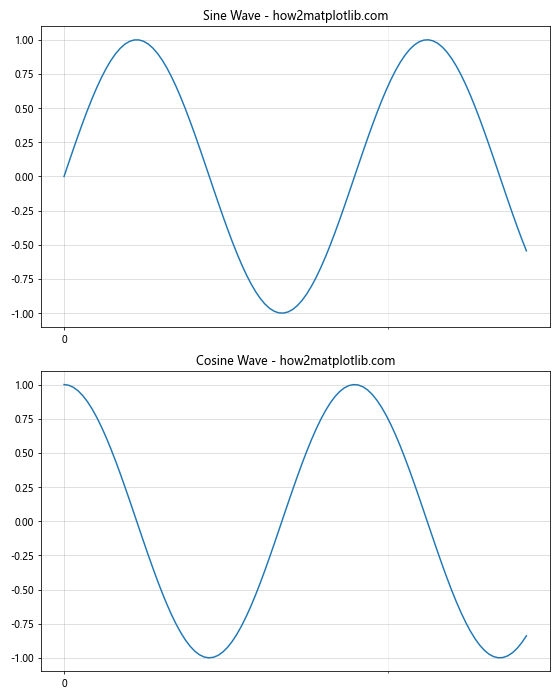
In this example, we use get_tick_space() in combination with set_major_locator() and set_minor_locator() to create a custom tick layout with both major and minor ticks. This demonstrates how get_tick_space() can be used as a reference point for more complex tick customizations.
Matplotlib.axis.Axis.get_tick_space() in Different Plot Types
Matplotlib.axis.Axis.get_tick_space() function in Python can be applied to various types of plots, each with its own unique considerations. Understanding how to use this function across different plot types can greatly enhance your data visualization capabilities.
Let’s explore how get_tick_space() can be used in different plot types:
Bar Plot Example
import matplotlib.pyplot as plt
import numpy as np
categories = ['A', 'B', 'C', 'D', 'E']
values = [23, 45, 56, 78, 32]
fig, ax = plt.subplots(figsize=(10, 6))
bars = ax.bar(categories, values)
# Customize x-axis ticks
space = ax.xaxis.get_tick_space()
ax.set_xticks(np.arange(len(categories)))
ax.set_xticklabels(categories, rotation=45)
# Add value labels on top of bars
for bar in bars:
height = bar.get_height()
ax.text(bar.get_x() + bar.get_width()/2., height,
f'{height}',
ha='center', va='bottom')
plt.title('Bar Plot with Custom Ticks - how2matplotlib.com')
plt.xlabel('Categories')
plt.ylabel('Values')
plt.tight_layout()
plt.show()
Output:
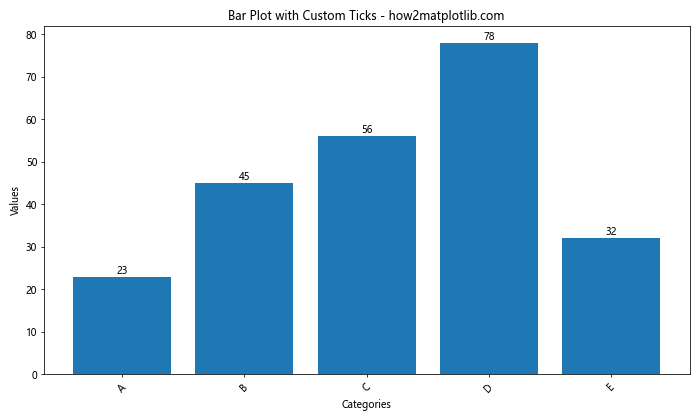
In this bar plot example, we use get_tick_space() to help position the category labels correctly on the x-axis.
Scatter Plot Example
import matplotlib.pyplot as plt
import numpy as np
# Generate random data
np.random.seed(42)
x = np.random.rand(50)
y = np.random.rand(50)
colors = np.random.rand(50)
sizes = 1000 * np.random.rand(50)
fig, ax = plt.subplots(figsize=(10, 8))
scatter = ax.scatter(x, y, c=colors, s=sizes, alpha=0.5)
# Customize ticks
x_space = ax.xaxis.get_tick_space()
y_space = ax.yaxis.get_tick_space()
ax.set_xticks(np.arange(0, 1.1, x_space))
ax.set_yticks(np.arange(0, 1.1, y_space))
plt.title('Scatter Plot with Custom Ticks - how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.colorbar(scatter, label='Color Scale')
plt.tight_layout()
plt.show()
Output:
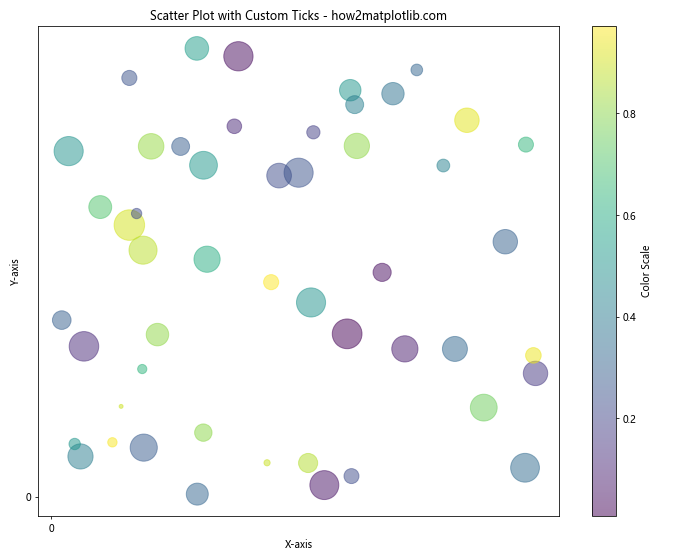
In this scatter plot example, we use get_tick_space() for both x and y axes to create evenly spaced ticks that cover the entire range of the data.
Handling Edge Cases with Matplotlib.axis.Axis.get_tick_space()
Matplotlib.axis.Axis.get_tick_space() function in Python may behave differently in certain edge cases, such as when dealing with very small or very large ranges of data. It’s important to understand these scenarios to effectively use the function in all situations.
Let’s explore some edge cases and how to handle them:
Small Data Range Example
import matplotlib.pyplot as plt
import numpy as np
# Create data with a small range
x = np.linspace(0, 0.001, 100)
y = np.sin(1000 * x)
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y)
# Get and print the tick space
space = ax.xaxis.get_tick_space()
print(f"Original tick space: {space}")
# Adjust tick spacing for small range
if space < 1e-4:
new_ticks = np.linspace(x.min(), x.max(), 5)
ax.set_xticks(new_ticks)
ax.xaxis.set_major_formatter(plt.FormatStrFormatter('%.5f'))
plt.title('Small Range Plot - how2matplotlib.com')
plt.xlabel('X-axis (small range)')
plt.ylabel('Y-axis')
plt.tight_layout()
plt.show()
Output:
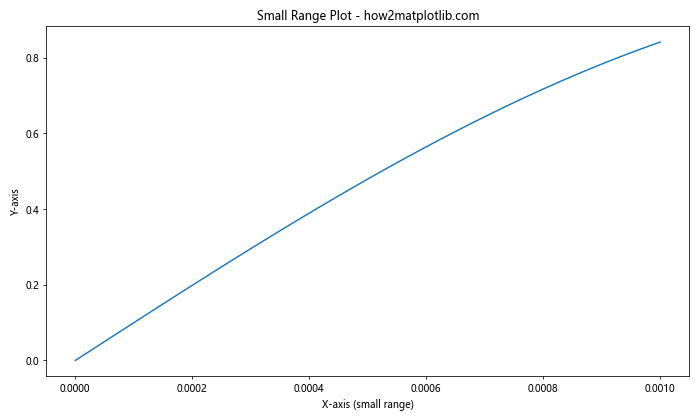
In this example, we handle a small data range by checking if the tick space is very small and adjusting it accordingly.
Large Data Range Example
import matplotlib.pyplot as plt
import numpy as np
# Create data with a large range
x = np.logspace(0, 9, 100)
y = np.log10(x)
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y)
# Get and print the tick space
space = ax.xaxis.get_tick_space()
print(f"Original tick space: {space}")
# Use log scale for x-axis
ax.set_xscale('log')
# Customize tick labels
ax.xaxis.set_major_formatter(plt.FuncFormatter(lambda x, p: f'{x:.0e}'))
plt.title('Large Range Plot - how2matplotlib.com')
plt.xlabel('X-axis (log scale)')
plt.ylabel('Y-axis')
plt.tight_layout()
plt.show()
Output:
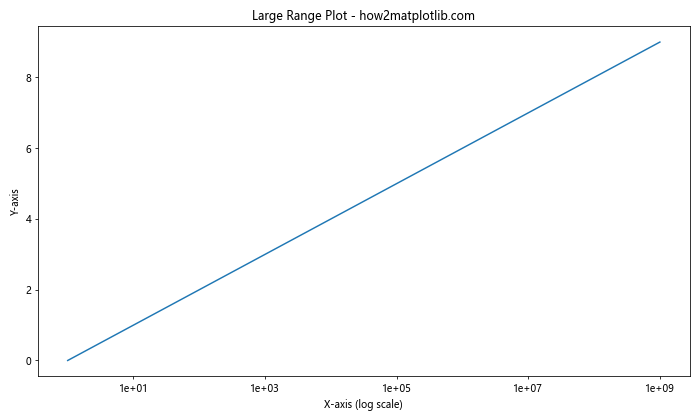
In this example, we handle a large data range by using a logarithmic scale and customizing the tick labels.
Matplotlib.axis.Axis.get_tick_space() in Interactive Plots
Matplotlib.axis.Axis.get_tick_space() function in Python can be particularly useful in interactive plots where the axis limits may change dynamically. In such cases, get_tick_space() can help maintain appropriate tick spacing as the plot is zoomed or panned.
Let's create an example of an interactive plot that uses get_tick_space():
import matplotlib.pyplot as plt
from matplotlib.widgets import RectangleSelector
import numpy as np
def onselect(eclick, erelease):
x1, y1 = eclick.xdata, eclick.ydata
x2, y2 = erelease.xdata, erelease.ydata
ax.set_xlim(min(x1, x2), max(x1, x2))
ax.set_ylim(min(y1, y2), max(y1, y2))
# Update tick spacing
x_space = ax.xaxis.get_tick_space()
y_space = ax.yaxis.get_tick_space()
ax.xaxis.set_major_locator(plt.MultipleLocator(x_space))
ax.yaxis.set_major_locator(plt.MultipleLocator(y_space))
fig.canvas.draw()
# Create data
x = np.linspace(0, 10, 1000)
y = np.sin(x) * np.exp(-x/10)
# Create plot
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y)
# Set up RectangleSelector
rs = RectangleSelector(ax, onselect, useblit=True,
button=[1], minspanx=5, minspany=5,
spancoords='pixels', interactive=True)
plt.title('Interactive Plot - how2matplotlib.com')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.show()
Output:
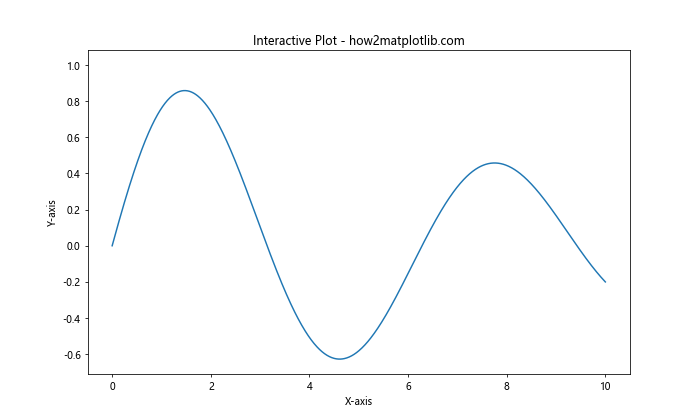
In this interactive plot example, we use get_tick_space() to dynamically adjust the tick spacing when the user zooms into a specific region of the plot using the RectangleSelector tool.
Advanced Techniques Using Matplotlib.axis.Axis.get_tick_space()
Matplotlib.axis.Axis.get_tick_space() function in Python can be used in more advanced ways to create highly customized and dynamic plots. These techniques can be particularly useful for complex data visualization tasks or when creating interactive dashboards.
Let's explore some advanced techniques:
Dynamic Tick Adjustment Based on Zoom Level
import matplotlib.pyplot as plt
from matplotlib.widgets import Slider
import numpy as np
def update(val):
zoom = zoom_slider.val
ax.set_xlim(5-zoom, 5+zoom)
ax.set_ylim(-zoom, zoom)
# Dynamically adjust tick spacing
x_space = ax.xaxis.get_tick_space()
y_space = ax.yaxis.get_tick_space()
ax.xaxis.set_major_locator(plt.MultipleLocator(x_space * 2))
ax.yaxis.set_major_locator(plt.MultipleLocator(y_space * 2))
fig.canvas.draw_idle()
# Create data
x = np.linspace(0, 10, 1000)
y = np.sin(x)
# Create plot
fig, ax = plt.subplots(figsize=(10, 8))
line, = ax.plot(x, y)
# Add zoom slider
ax_zoom = plt.axes([0.2, 0.02, 0.6, 0.03])
zoom_slider = Slider(ax_zoom, 'Zoom', 0.1, 5, valinit=5)
zoom_slider.on_changed(update)
plt.suptitle('Dynamic Tick Adjustment - how2matplotlib.com')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
plt.tight_layout()
plt.show()
Output:
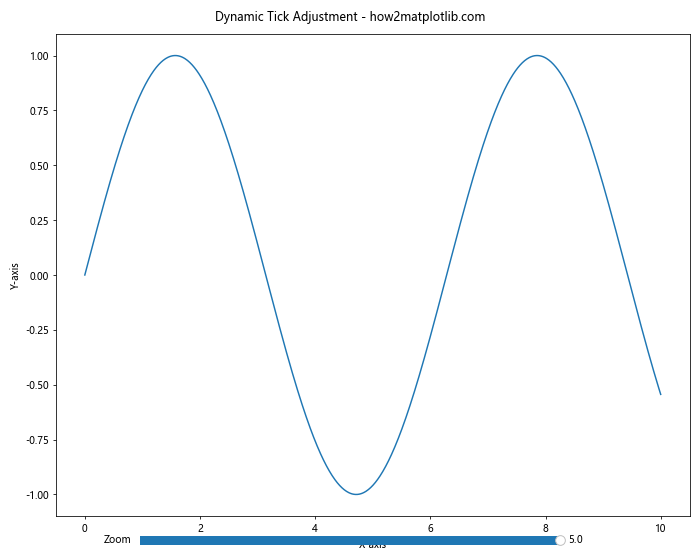
In this advanced example, we create a plot with a zoom slider. As the user adjusts the zoom level, we dynamically update the tick spacing using get_tick_space().
Custom Tick Formatter Using get_tick_space()
import matplotlib.pyplot as plt
import numpy as np
class CustomFormatter(plt.Formatter):
def __init__(self, ax):
self.ax = ax
def __call__(self, x, pos=None):
space = self.ax.xaxis.get_tick_space()
if space > 1:
return f'{x:.0f}'
elif space > 0.1:
return f'{x:.1f}'
else:
return f'{x:.2e}'
# Create data
x = np.logspace(-2, 2, 100)
y = np.sin(x)
# Create plot
fig, ax = plt.subplots(figsize=(12, 6))
ax.plot(x, y)
# Set logarithmic x-scale
ax.set_xscale('log')
# Apply custom formatter
ax.xaxis.set_major_formatter(CustomFormatter(ax))
plt.title('Custom Tick Formatter - how2matplotlib.com')
plt.xlabel('X-axis (log scale)')
plt.ylabel('Y-axis')
plt.grid(True, which="both", ls="-", alpha=0.2)
plt.tight_layout()
plt.show()
Output:
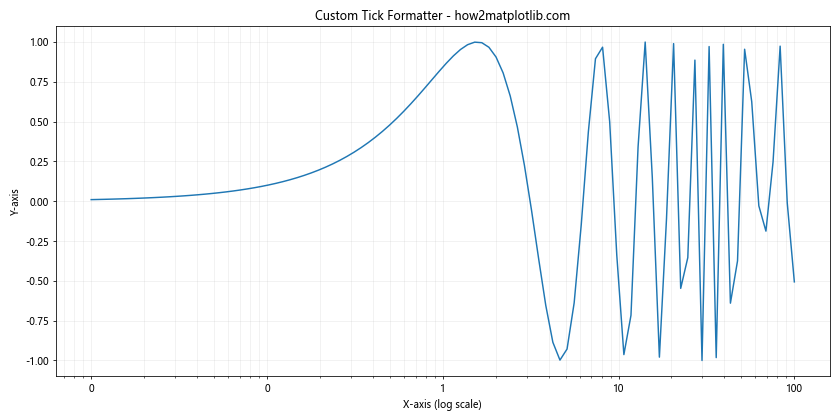
In this example, we create a custom tick formatter that adjusts the number of decimal places based on the tick space. This can be particularly useful for plots with varying scales or logarithmic axes.
Matplotlib.axis.Axis.get_tick_space() in Real-world Applications
Matplotlib.axis.Axis.get_tick_space() function in Python has numerous real-world applications in data visualization and scientific plotting. Let's explore some scenarios where this function can be particularly useful:
Scientific Data Plotting
import matplotlib.pyplot as plt
import numpy as np
def plot_wave(ax, frequency, amplitude, phase):
x = np.linspace(0, 2*np.pi, 1000)
y = amplitude * np.sin(frequency * x + phase)
ax.plot(x, y, label=f'f={frequency:.1f}, A={amplitude:.1f}, φ={phase:.1f}')
# Create plot
fig, ax = plt.subplots(figsize=(12, 6))
# Plot multiple waves
plot_wave(ax, 1, 1, 0)
plot_wave(ax, 2, 0.5, np.pi/4)
plot_wave(ax, 3, 0.3, np.pi/2)
# Customize ticks using get_tick_space
x_space = ax.xaxis.get_tick_space()
y_space = ax.yaxis.get_tick_space()
ax.xaxis.set_major_locator(plt.MultipleLocator(x_space))
ax.xaxis.set_minor_locator(plt.MultipleLocator(x_space / 4))
ax.yaxis.set_major_locator(plt.MultipleLocator(y_space))
ax.yaxis.set_minor_locator(plt.MultipleLocator(y_space / 4))
ax.grid(which='major', linestyle='-', linewidth='0.5', color='gray')
ax.grid(which='minor', linestyle=':', linewidth='0.5', color='lightgray')
plt.title('Wave Superposition - how2matplotlib.com')
plt.xlabel('Phase (radians)')
plt.ylabel('Amplitude')
plt.legend()
plt.tight_layout()
plt.show()
Output:
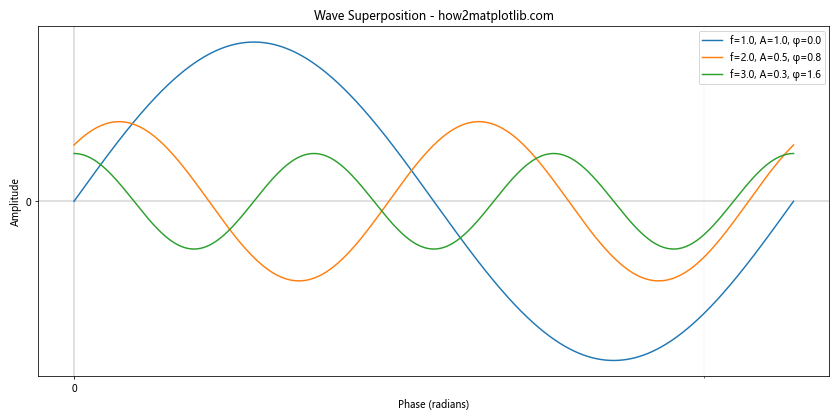
In this scientific plotting example, we use get_tick_space() to create a consistent and readable tick spacing for both the x and y axes when plotting multiple superimposed waves.
Comparing Matplotlib.axis.Axis.get_tick_space() with Other Tick Spacing Methods
While Matplotlib.axis.Axis.get_tick_space() function in Python is a powerful tool for customizing tick spacing, it's worth comparing it with other methods available in Matplotlib. Understanding the strengths and weaknesses of each approach can help you choose the best method for your specific visualization needs.
Let's compare get_tick_space() with some other common methods:
1. get_tick_space() vs. set_xticks() / set_yticks()
import matplotlib.pyplot as plt
import numpy as np
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create subplots
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(10, 10))
# Plot using get_tick_space()
ax1.plot(x, y)
space = ax1.xaxis.get_tick_space()
ax1.xaxis.set_major_locator(plt.MultipleLocator(space * 1.5))
ax1.set_title('Using get_tick_space() - how2matplotlib.com')
# Plot using set_xticks()
ax2.plot(x, y)
ax2.set_xticks(np.arange(0, 11, 2))
ax2.set_title('Using set_xticks() - how2matplotlib.com')
plt.tight_layout()
plt.show()
Output:
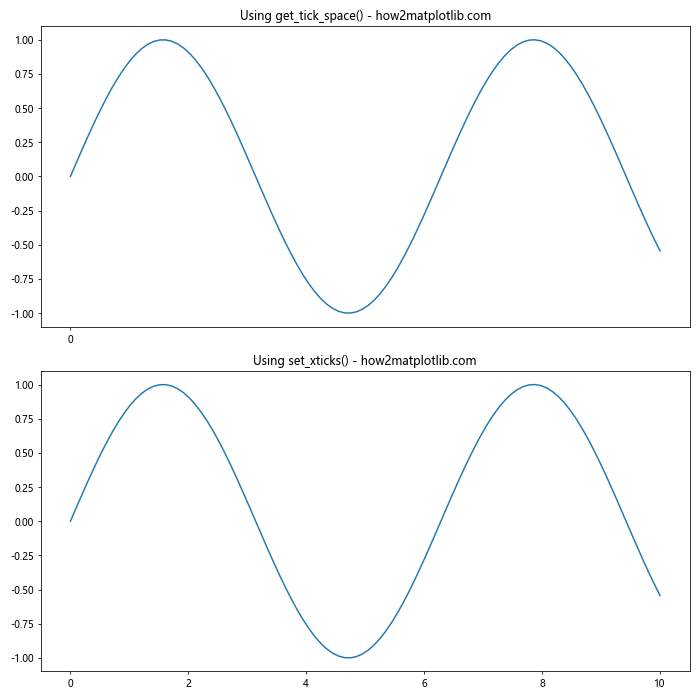
In this comparison, get_tick_space() allows for more dynamic tick spacing based on the current view, while set_xticks() provides more direct control over tick locations.
2. get_tick_space() vs. AutoLocator
import matplotlib.pyplot as plt
import numpy as np
# Create data
x = np.logspace(0, 3, 100)
y = np.log10(x)
# Create subplots
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(10, 10))
# Plot using get_tick_space()
ax1.plot(x, y)
ax1.set_xscale('log')
space = ax1.xaxis.get_tick_space()
ax1.xaxis.set_major_locator(plt.LogLocator(base=10, numticks=int(10/space)))
ax1.set_title('Using get_tick_space() - how2matplotlib.com')
# Plot using AutoLocator
ax2.plot(x, y)
ax2.set_xscale('log')
ax2.xaxis.set_major_locator(plt.AutoLocator())
ax2.set_title('Using AutoLocator - how2matplotlib.com')
plt.tight_layout()
plt.show()
In this comparison, get_tick_space() allows for more fine-tuned control over the number of ticks, while AutoLocator automatically chooses tick locations based on the data range.
Troubleshooting Common Issues with Matplotlib.axis.Axis.get_tick_space()
When working with Matplotlib.axis.Axis.get_tick_space() function inWhen working with Matplotlib.axis.Axis.get_tick_space() function in Python, you may encounter some common issues. Here are some problems you might face and how to troubleshoot them:
1. Unexpected Tick Spacing
Sometimes, get_tick_space() might return a value that leads to unexpected tick spacing. This can often be resolved by scaling the returned value or using it as a starting point for further calculations.
import matplotlib.pyplot as plt
import numpy as np
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create plot
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(10, 10))
# Plot with unexpected spacing
ax1.plot(x, y)
space = ax1.xaxis.get_tick_space()
ax1.xaxis.set_major_locator(plt.MultipleLocator(space))
ax1.set_title('Unexpected Spacing - how2matplotlib.com')
# Plot with adjusted spacing
ax2.plot(x, y)
adjusted_space = max(1, space * 1.5) # Ensure minimum spacing of 1
ax2.xaxis.set_major_locator(plt.MultipleLocator(adjusted_space))
ax2.set_title('Adjusted Spacing - how2matplotlib.com')
plt.tight_layout()
plt.show()
Output:
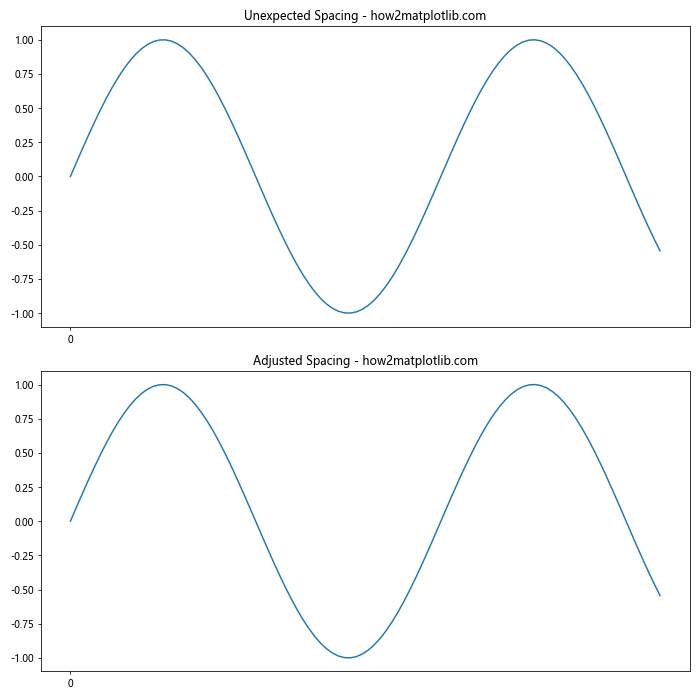
2. Issues with Logarithmic Scales
When using logarithmic scales, get_tick_space() might not provide immediately usable values. You may need to apply logarithmic transformations.
import matplotlib.pyplot as plt
import numpy as np
# Create data
x = np.logspace(0, 3, 100)
y = x**2
# Create plot
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(10, 10))
# Plot with issues on log scale
ax1.plot(x, y)
ax1.set_xscale('log')
space = ax1.xaxis.get_tick_space()
ax1.xaxis.set_major_locator(plt.MultipleLocator(space))
ax1.set_title('Issues with Log Scale - how2matplotlib.com')
# Plot with corrected log scale handling
ax2.plot(x, y)
ax2.set_xscale('log')
log_space = np.log10(space)
ax2.xaxis.set_major_locator(plt.LogLocator(base=10, numticks=int(10/log_space)))
ax2.set_title('Corrected Log Scale Handling - how2matplotlib.com')
plt.tight_layout()
plt.show()
Output:
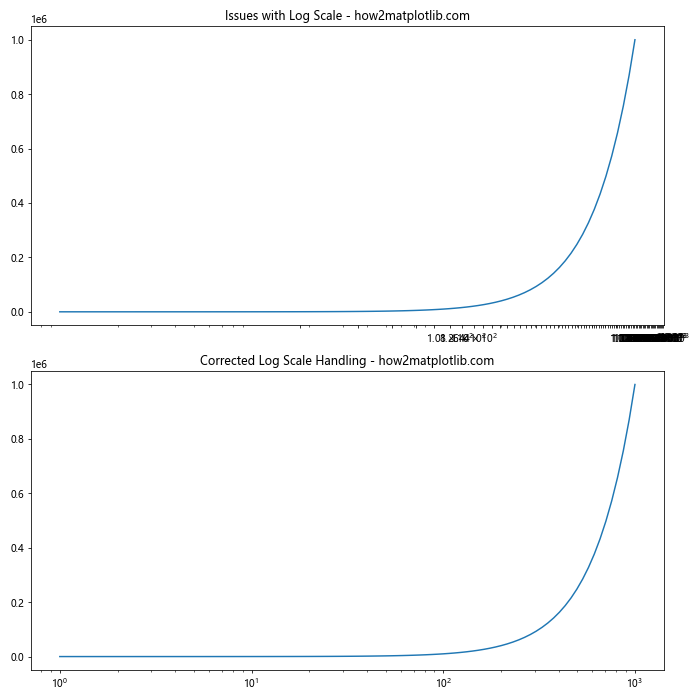
Future Developments and Alternatives to Matplotlib.axis.Axis.get_tick_space()
While Matplotlib.axis.Axis.get_tick_space() function in Python is a useful tool, it's important to stay informed about future developments in Matplotlib and consider alternative approaches for tick spacing customization.
Potential Future Developments
- Enhanced automatic tick spacing algorithms that adapt to different data types and scales.
- Integration with machine learning techniques for optimal tick placement.
- Improved handling of edge cases and unusual data distributions.
Alternative Approaches
- Using Locator classes: Matplotlib provides various Locator classes that can be used for tick spacing.
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, (ax1, ax2, ax3) = plt.subplots(3, 1, figsize=(10, 15))
# MaxNLocator
ax1.plot(x, y)
ax1.xaxis.set_major_locator(plt.MaxNLocator(5))
ax1.set_title('MaxNLocator - how2matplotlib.com')
# LinearLocator
ax2.plot(x, y)
ax2.xaxis.set_major_locator(plt.LinearLocator(numticks=6))
ax2.set_title('LinearLocator - how2matplotlib.com')
# MultipleLocator
ax3.plot(x, y)
ax3.xaxis.set_major_locator(plt.MultipleLocator(base=2))
ax3.set_title('MultipleLocator - how2matplotlib.com')
plt.tight_layout()
plt.show()
Output:
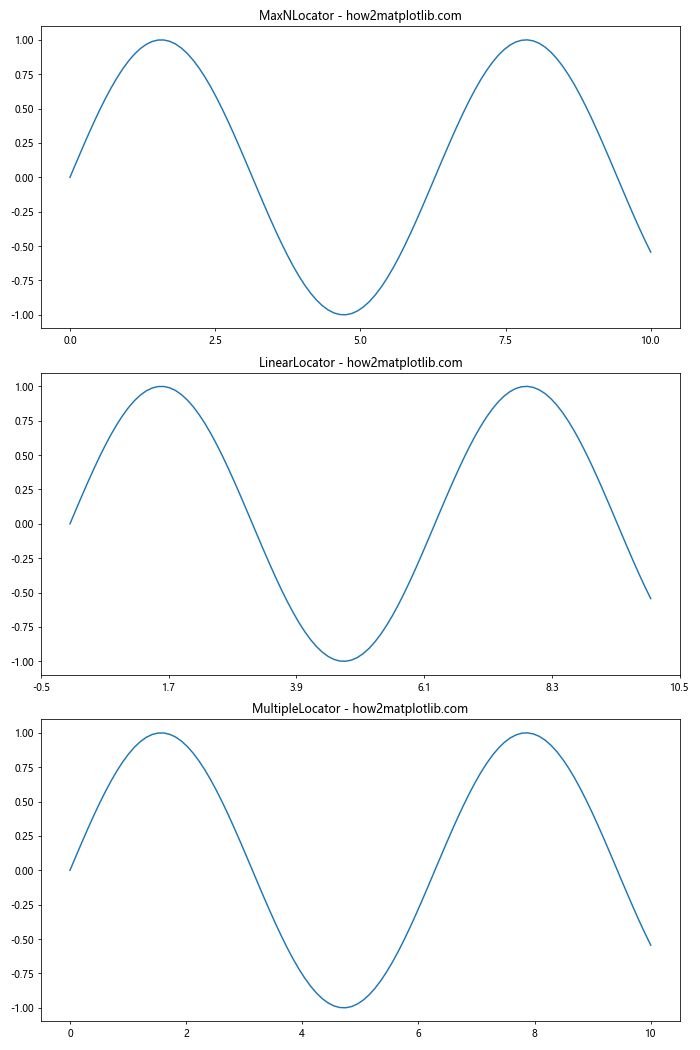
- Using tick_params: The tick_params method allows for fine-grained control over tick appearance and spacing.
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
fig, ax = plt.subplots(figsize=(10, 6))
ax.plot(x, y)
ax.tick_params(axis='x', which='major', length=10, width=2, color='red', pad=15)
ax.tick_params(axis='x', which='minor', length=5, width=1, color='blue', pad=10)
ax.xaxis.set_major_locator(plt.MultipleLocator(2))
ax.xaxis.set_minor_locator(plt.MultipleLocator(0.5))
plt.title('Custom Tick Params - how2matplotlib.com')
plt.tight_layout()
plt.show()
Output:
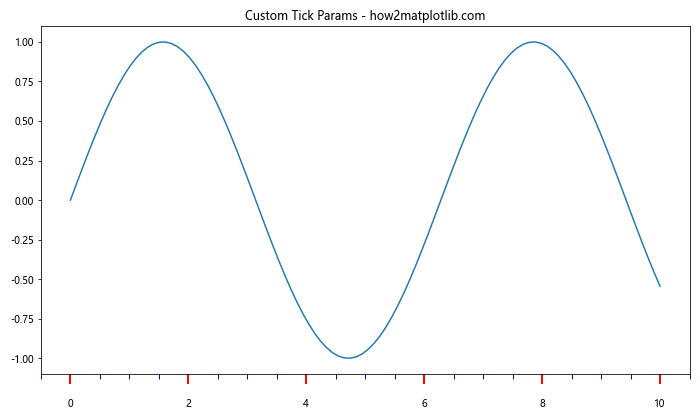
- Using FuncFormatter: For complete control over tick label formatting, you can use FuncFormatter.
import matplotlib.pyplot as plt
import numpy as np
def custom_formatter(x, pos):
if x.is_integer():
return f"{int(x)}°"
else:
return f"{x:.1f}°"
x = np.linspace(0, 360, 100)
y = np.sin(np.radians(x))
fig, ax = plt.subplots(figsize=(12, 6))
ax.plot(x, y)
ax.xaxis.set_major_locator(plt.MultipleLocator(45))
ax.xaxis.set_major_formatter(plt.FuncFormatter(custom_formatter))
plt.title('Custom Tick Formatter - how2matplotlib.com')
plt.tight_layout()
plt.show()
Output:
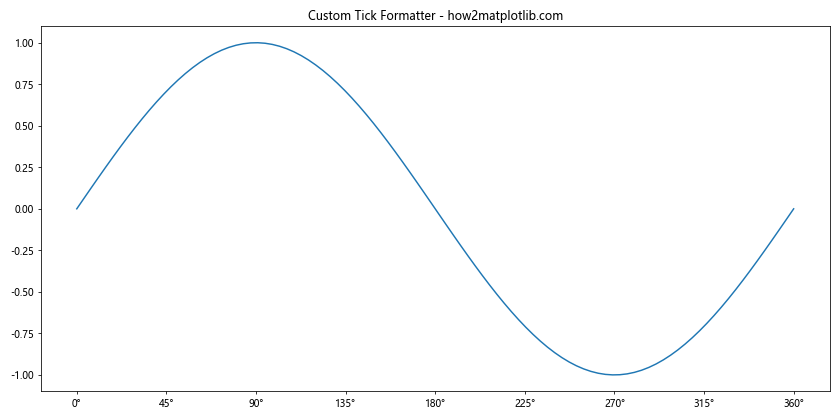
Conclusion
Matplotlib.axis.Axis.get_tick_space() function in Python is a powerful tool for customizing tick spacing in Matplotlib plots. Throughout this comprehensive guide, we've explored its basic usage, advanced techniques, real-world applications, and potential pitfalls. We've seen how get_tick_space() can be used to create more readable and aesthetically pleasing plots, especially when dealing with different scales and plot types.