Comprehensive Guide to Matplotlib.artist.Artist.update_from() in Python
Matplotlib.artist.Artist.update_from() in Python is a powerful method that allows you to update the properties of one Artist object with the properties of another. This function is an essential tool for customizing and refining your data visualizations in Matplotlib. In this comprehensive guide, we’ll explore the various aspects of Matplotlib.artist.Artist.update_from(), its usage, and how it can enhance your plotting capabilities.
Understanding Matplotlib.artist.Artist.update_from() in Python
Matplotlib.artist.Artist.update_from() is a method that belongs to the Artist class in Matplotlib. The Artist class is the base class for all visible elements in a Matplotlib figure, including lines, text, and patches. The update_from() method allows you to copy properties from one Artist object to another, which can be incredibly useful when you want to maintain consistency across multiple plot elements or when you want to create variations of existing artists.
Let’s start with a simple example to demonstrate how Matplotlib.artist.Artist.update_from() works:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Create two rectangles
rect1 = plt.Rectangle((0.1, 0.1), 0.4, 0.4, facecolor='red', edgecolor='black', linewidth=2)
rect2 = plt.Rectangle((0.6, 0.6), 0.3, 0.3)
# Update rect2 properties from rect1
rect2.update_from(rect1)
# Add rectangles to the plot
ax.add_patch(rect1)
ax.add_patch(rect2)
ax.set_title('Matplotlib.artist.Artist.update_from() Example - how2matplotlib.com')
plt.show()
Output:
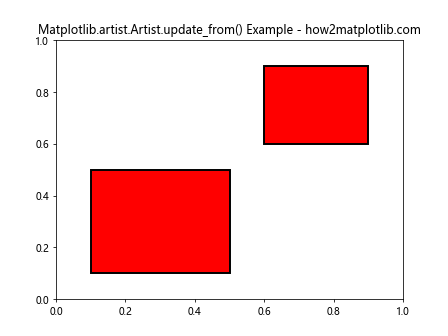
In this example, we create two Rectangle objects, rect1 and rect2. We then use the update_from() method to copy the properties of rect1 to rect2. As a result, rect2 will inherit the facecolor, edgecolor, and linewidth properties from rect1.
Key Features of Matplotlib.artist.Artist.update_from() in Python
Matplotlib.artist.Artist.update_from() offers several key features that make it a versatile tool for customizing your plots:
- Property Copying: It allows you to copy properties from one Artist object to another, maintaining consistency across plot elements.
Selective Updates: You can choose which properties to update by specifying them in the method call.
Efficiency: Instead of manually setting multiple properties, you can use update_from() to quickly apply a set of properties to multiple artists.
Flexibility: It works with various types of Artist objects, including lines, patches, and text elements.
Let’s explore these features in more detail with some examples.