Comprehensive Guide to Matplotlib.axis.Axis.axis_date() Function in Python
Matplotlib.axis.Axis.axis_date() function in Python is a powerful tool for handling date and time data on plot axes. This function is essential for creating time-based visualizations and is particularly useful when working with time series data. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.axis_date() function in depth, covering its usage, parameters, and various applications in data visualization.
Understanding the Basics of Matplotlib.axis.Axis.axis_date()
The Matplotlib.axis.Axis.axis_date() function is a method of the Axis class in Matplotlib. Its primary purpose is to set up the axis for handling date values. When you call this function on an axis, it configures the axis to properly display and format date and time information.
Let’s start with a simple example to illustrate the basic usage of Matplotlib.axis.Axis.axis_date():
import matplotlib.pyplot as plt
import datetime
# Create some sample data
dates = [datetime.datetime(2023, 1, 1), datetime.datetime(2023, 6, 1), datetime.datetime(2023, 12, 31)]
values = [10, 20, 15]
# Create the plot
fig, ax = plt.subplots()
ax.plot(dates, values)
# Apply axis_date() to the x-axis
ax.xaxis.axis_date()
plt.title("Basic usage of axis_date() - how2matplotlib.com")
plt.show()
Output:
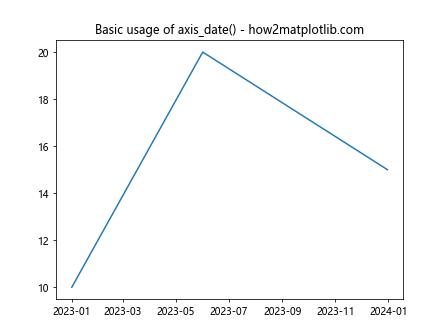
In this example, we create a simple line plot with dates on the x-axis. By calling ax.xaxis.axis_date()
, we ensure that the x-axis is properly formatted to display dates.
Customizing Date Formats with Matplotlib.axis.Axis.axis_date()
One of the key features of the Matplotlib.axis.Axis.axis_date() function is its ability to customize date formats. You can specify different date formats to suit your visualization needs.
Here’s an example demonstrating how to customize the date format:
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import datetime
# Create sample data
dates = [datetime.datetime(2023, i, 1) for i in range(1, 13)]
values = [10, 12, 15, 18, 20, 22, 25, 23, 21, 19, 16, 13]
fig, ax = plt.subplots()
ax.plot(dates, values)
# Apply axis_date() with custom format
ax.xaxis.axis_date()
ax.xaxis.set_major_formatter(mdates.DateFormatter('%b %Y'))
plt.title("Custom date format with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
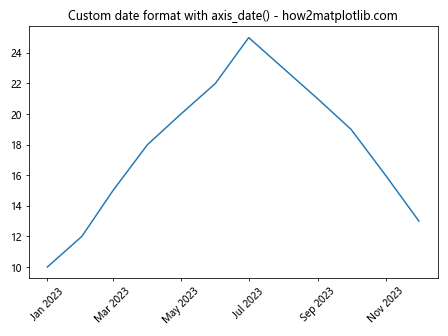
In this example, we use mdates.DateFormatter('%b %Y')
to display dates in the format “Month Year” (e.g., “Jan 2023”).
Using Matplotlib.axis.Axis.axis_date() with Different Time Scales
The Matplotlib.axis.Axis.axis_date() function can handle various time scales, from years down to milliseconds. Let’s explore how to work with different time scales:
Daily Data
import matplotlib.pyplot as plt
import datetime
# Create daily data
dates = [datetime.datetime(2023, 1, 1) + datetime.timedelta(days=i) for i in range(30)]
values = [i**2 for i in range(30)]
fig, ax = plt.subplots()
ax.plot(dates, values)
ax.xaxis.axis_date()
plt.title("Daily data with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
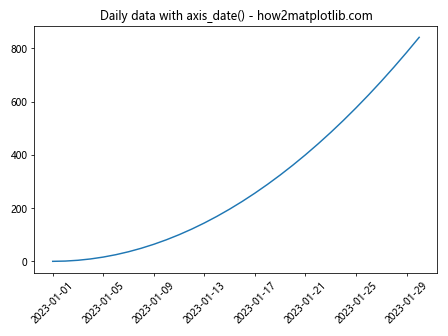
Hourly Data
import matplotlib.pyplot as plt
import datetime
# Create hourly data
dates = [datetime.datetime(2023, 1, 1) + datetime.timedelta(hours=i) for i in range(24)]
values = [i**2 for i in range(24)]
fig, ax = plt.subplots()
ax.plot(dates, values)
ax.xaxis.axis_date()
plt.title("Hourly data with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
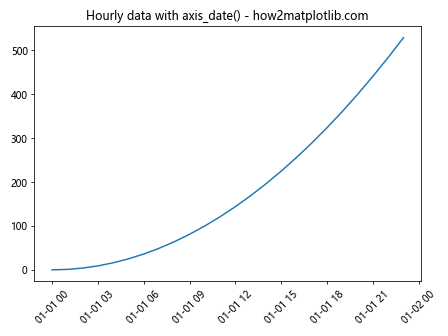
Minute Data
import matplotlib.pyplot as plt
import datetime
# Create minute data
dates = [datetime.datetime(2023, 1, 1, 0, 0) + datetime.timedelta(minutes=i) for i in range(60)]
values = [i**2 for i in range(60)]
fig, ax = plt.subplots()
ax.plot(dates, values)
ax.xaxis.axis_date()
plt.title("Minute data with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
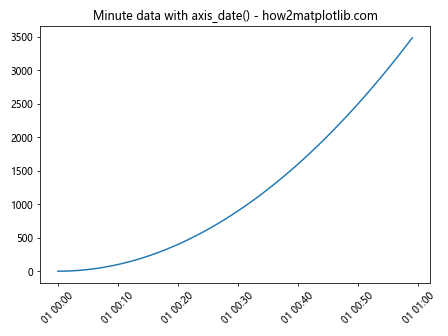
Combining Matplotlib.axis.Axis.axis_date() with Locators
Matplotlib provides various locator classes that work well with the axis_date() function. These locators help in placing tick marks at appropriate intervals on the date axis.
Using YearLocator
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import datetime
# Create sample data
dates = [datetime.datetime(year, 1, 1) for year in range(2010, 2024)]
values = [i**2 for i in range(len(dates))]
fig, ax = plt.subplots()
ax.plot(dates, values)
ax.xaxis.axis_date()
ax.xaxis.set_major_locator(mdates.YearLocator())
ax.xaxis.set_major_formatter(mdates.DateFormatter('%Y'))
plt.title("YearLocator with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
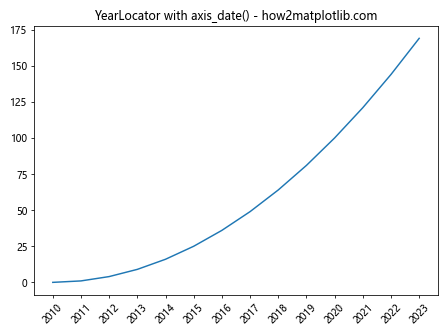
Using MonthLocator
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import datetime
# Create sample data
dates = [datetime.datetime(2023, month, 1) for month in range(1, 13)]
values = [i**2 for i in range(len(dates))]
fig, ax = plt.subplots()
ax.plot(dates, values)
ax.xaxis.axis_date()
ax.xaxis.set_major_locator(mdates.MonthLocator())
ax.xaxis.set_major_formatter(mdates.DateFormatter('%b'))
plt.title("MonthLocator with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
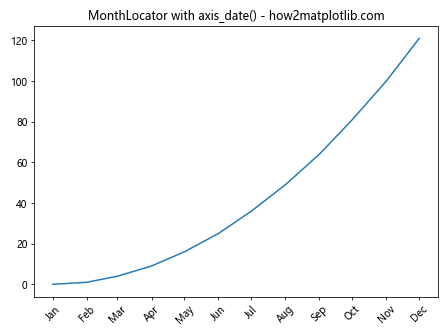
Using DayLocator
import matplotlib.pyplot as plt
import matplotlib.dates as mdates
import datetime
# Create sample data
dates = [datetime.datetime(2023, 1, day) for day in range(1, 32)]
values = [i**2 for i in range(len(dates))]
fig, ax = plt.subplots()
ax.plot(dates, values)
ax.xaxis.axis_date()
ax.xaxis.set_major_locator(mdates.DayLocator(interval=5))
ax.xaxis.set_major_formatter(mdates.DateFormatter('%d'))
plt.title("DayLocator with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
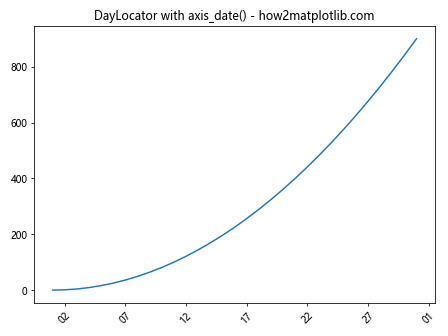
Advanced Techniques with Matplotlib.axis.Axis.axis_date()
Now that we’ve covered the basics, let’s explore some advanced techniques using the Matplotlib.axis.Axis.axis_date() function.
Handling Time Zones
When working with date and time data from different time zones, it’s important to handle them correctly. Here’s an example of how to use axis_date() with time zone aware datetime objects:
import matplotlib.pyplot as plt
import datetime
import pytz
# Create time zone aware datetime objects
tz = pytz.timezone('US/Eastern')
dates = [tz.localize(datetime.datetime(2023, 1, 1) + datetime.timedelta(hours=i)) for i in range(24)]
values = [i**2 for i in range(24)]
fig, ax = plt.subplots()
ax.plot(dates, values)
ax.xaxis.axis_date(tz=tz)
plt.title("Time zone handling with axis_date() - how2matplotlib.com")
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
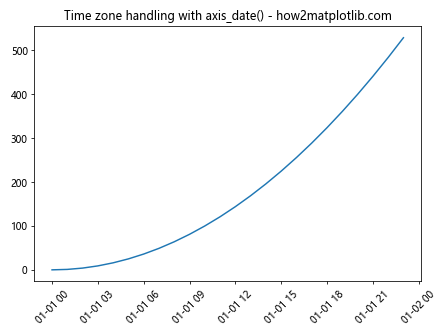
In this example, we create time zone aware datetime objects and pass the time zone to axis_date() to ensure correct handling of the dates.
Combining Multiple Time Series
The Matplotlib.axis.Axis.axis_date() function is particularly useful when plotting multiple time series on the same axis. Here’s an example:
import matplotlib.pyplot as plt
import datetime
# Create two sets of sample data
dates1 = [datetime.datetime(2023, 1, 1) + datetime.timedelta(days=i) for i in range(30)]
values1 = [i**2 for i in range(30)]
dates2 = [datetime.datetime(2023, 1, 15) + datetime.timedelta(days=i) for i in range(30)]
values2 = [i**3 for i in range(30)]
fig, ax = plt.subplots()
ax.plot(dates1, values1, label='Series 1')
ax.plot(dates2, values2, label='Series 2')
ax.xaxis.axis_date()
plt.title("Multiple time series with axis_date() - how2matplotlib.com")
plt.legend()
plt.xticks(rotation=45)
plt.tight_layout()
plt.show()
Output:
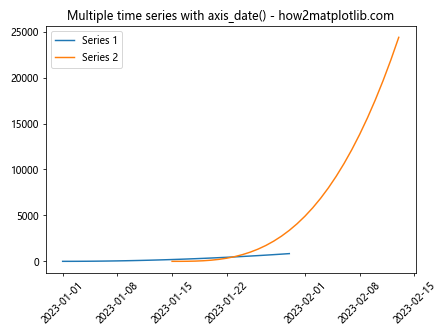
This example demonstrates how axis_date() can handle multiple time series with different date ranges on the same plot.
Creating a Date Slider
The Matplotlib.axis.Axis.axis_date() function can be combined with Matplotlib’s widgets to create interactive date-based visualizations. Here’s an example of creating a simple date slider:
import matplotlib.pyplot as plt
from matplotlib.widgets import Slider
import datetime
import numpy as np
# Create sample data
start_date = datetime.datetime(2023, 1, 1)
dates = [start_date + datetime.timedelta(days=i) for i in range(365)]
values = np.random.rand(365)
fig, ax = plt.subplots()
line, = ax.plot(dates, values)
ax.xaxis.axis_date()
plt.subplots_adjust(bottom=0.25)
# Create the slider
slider_ax = plt.axes([0.2, 0.1, 0.6, 0.03])
date_slider = Slider(slider_ax, 'Date', 0, 364, valinit=0, valstep=1)
def update(val):
index = int(date_slider.val)
ax.set_xlim(dates[index], dates[index+30])
fig.canvas.draw_idle()
date_slider.on_changed(update)
plt.title("Date slider with axis_date() - how2matplotlib.com")
plt.show()
Output:
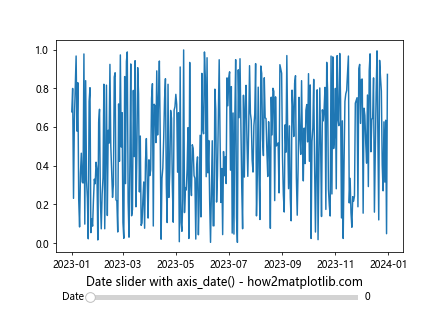
This example creates a slider that allows the user to interactively explore different date ranges in the plot.
Best Practices for Using Matplotlib.axis.Axis.axis_date()
When working with the Matplotlib.axis.Axis.axis_date() function, there are several best practices to keep in mind:
- Always use datetime objects: The axis_date() function works best with Python’s datetime objects. Avoid using string representations of dates.
Set appropriate locators and formatters: Use the right combination of locators and formatters to ensure your date axis is readable and informative.
Handle time zones correctly: When working with data from different time zones, make sure to use time zone aware datetime objects and specify the time zone in axis_date().
Rotate tick labels: For better readability, especially with longer date formats, rotate the tick labels using plt.xticks(rotation=45).
Use tight_layout(): To prevent overlapping labels, use plt.tight_layout() to automatically adjust the plot layout.
Here’s an example incorporating these best practices: