Comprehensive Guide to Matplotlib.axis.Axis.cla() Function in Python:
Matplotlib.axis.Axis.cla() function in Python is a powerful tool for clearing the content of an axis in Matplotlib plots. This function is essential for resetting an axis to its default state, allowing you to create new plots or modify existing ones without interference from previous content. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.cla() function in depth, covering its usage, benefits, and various applications in data visualization.
Understanding the Matplotlib.axis.Axis.cla() Function
The Matplotlib.axis.Axis.cla() function is a method of the Axis class in Matplotlib. It stands for “clear axis” and is used to remove all the content from a specific axis, including lines, text, and other plot elements. This function is particularly useful when you want to reuse an existing figure or axis for a new plot without creating an entirely new figure.
Let’s start with a simple example to demonstrate the basic usage of Matplotlib.axis.Axis.cla():
import matplotlib.pyplot as plt
import numpy as np
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
x = np.linspace(0, 10, 100)
y = np.sin(x)
ax.plot(x, y, label='Sin(x)')
ax.set_title('How2matplotlib.com: Sin(x) Plot')
ax.legend()
# Clear the axis
ax.cla()
# Plot new data
y_new = np.cos(x)
ax.plot(x, y_new, label='Cos(x)')
ax.set_title('How2matplotlib.com: Cos(x) Plot')
ax.legend()
plt.show()
Output:
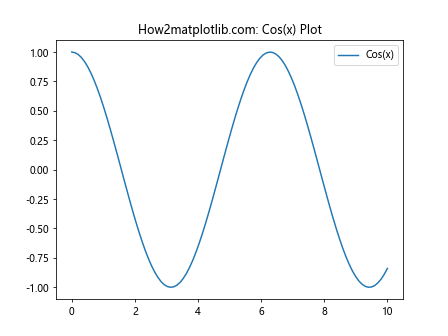
In this example, we first create a plot of sin(x), then use Matplotlib.axis.Axis.cla() to clear the axis content, and finally plot cos(x) on the same axis. The Matplotlib.axis.Axis.cla() function removes all the previous plot elements, allowing us to create a new plot without any interference from the previous one.
Benefits of Using Matplotlib.axis.Axis.cla()
The Matplotlib.axis.Axis.cla() function offers several advantages when working with Matplotlib plots:
- Memory efficiency: By clearing and reusing existing axes, you can reduce memory usage, especially when creating multiple plots in a loop.
Cleaner code: It allows you to update plots in-place, leading to more concise and readable code.
Interactive plotting: When creating interactive applications or animations, Matplotlib.axis.Axis.cla() is essential for updating plot content dynamically.
Flexibility: You can selectively clear specific axes in a multi-plot figure, giving you fine-grained control over your visualizations.
Let’s explore these benefits with more examples.