Comprehensive Guide to Using Matplotlib.artist.Artist.set_url() in Python for Data Visualization
Matplotlib.artist.Artist.set_url() in Python is a powerful method that allows you to add interactivity to your plots by setting a URL for an artist object. This function is part of the Matplotlib library, which is widely used for creating static, animated, and interactive visualizations in Python. In this comprehensive guide, we’ll explore the various aspects of Matplotlib.artist.Artist.set_url() and how it can enhance your data visualization projects.
Understanding Matplotlib.artist.Artist.set_url() in Python
Matplotlib.artist.Artist.set_url() is a method that belongs to the Artist class in Matplotlib. The Artist class is the base class for all drawable objects in Matplotlib, including lines, text, and patches. The set_url() method allows you to associate a URL with an artist object, which can be useful for creating interactive plots or adding metadata to your visualizations.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.set_url():
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
circle = plt.Circle((0.5, 0.5), 0.2, color='blue')
circle.set_url('https://how2matplotlib.com')
ax.add_artist(circle)
plt.show()
Output:
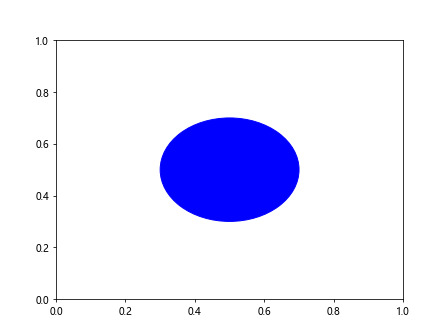
In this example, we create a circle using plt.Circle() and then use the set_url() method to associate a URL with the circle. When you hover over the circle in an interactive environment, you’ll be able to see the URL.
Advanced Techniques for Using Matplotlib.artist.Artist.set_url() in Python
Matplotlib.artist.Artist.set_url() can be used in more advanced ways to create complex, interactive visualizations. Let’s explore some advanced techniques:
Creating Clickable Legends with Matplotlib.artist.Artist.set_url()
You can use set_url() to make legend entries clickable, providing additional information about each data series:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, ax = plt.subplots()
line1, = ax.plot(x, y1, label='Sine')
line2, = ax.plot(x, y2, label='Cosine')
line1.set_url('https://how2matplotlib.com/sine')
line2.set_url('https://how2matplotlib.com/cosine')
legend = ax.legend()
for text in legend.get_texts():
text.set_url(f'https://how2matplotlib.com/{text.get_text().lower()}')
plt.title('Clickable Legend Example')
plt.show()
Output:
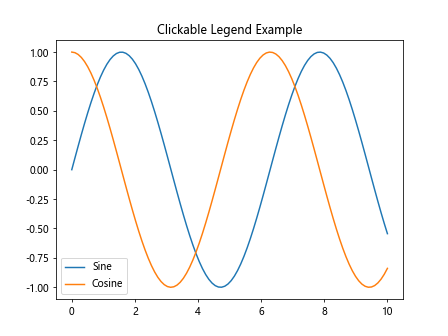
In this example, we associate URLs with both the plotted lines and the legend entries, making the entire plot more interactive.
Using Matplotlib.artist.Artist.set_url() with Annotations
You can combine set_url() with annotations to create informative tooltips:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y = np.sin(x)
line, = ax.plot(x, y)
annotation = ax.annotate('Peak', xy=(np.pi/2, 1), xytext=(3, 1.5),
arrowprops=dict(facecolor='black', shrink=0.05))
annotation.set_url('https://how2matplotlib.com/sine_peak')
plt.title('Annotation with URL')
plt.show()
Output:
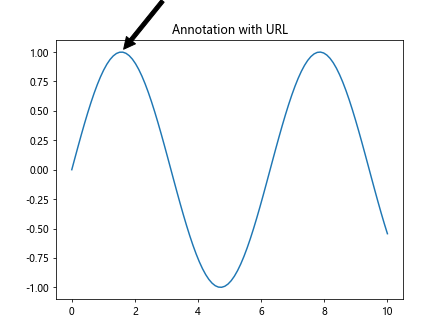
This example creates an annotation for the peak of the sine wave and associates a URL with it, providing additional context for the annotated feature.
Best Practices for Using Matplotlib.artist.Artist.set_url() in Python
When working with Matplotlib.artist.Artist.set_url(), it’s important to follow some best practices to ensure your visualizations are effective and user-friendly:
- Use meaningful URLs: Ensure that the URLs you set provide relevant and useful information related to the plot element.
Maintain consistency: If you’re using set_url() across multiple elements in your plot, maintain a consistent structure for your URLs.
Consider accessibility: Remember that not all users may be able to interact with your plot in the same way. Provide alternative means of accessing the information when possible.
Test in different environments: The behavior of set_url() may vary depending on the environment in which your plot is displayed. Test your visualizations in different contexts to ensure they work as expected.
Here’s an example that demonstrates these best practices: