Comprehensive Guide to Matplotlib.artist.Artist.update() in Python:
Matplotlib.artist.Artist.update() in Python is a powerful method that allows you to update the properties of an Artist object in Matplotlib. This function is essential for creating dynamic and interactive visualizations, as it enables you to modify various aspects of your plots on the fly. In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.artist.Artist.update(), providing you with detailed explanations and practical examples to help you master this crucial aspect of data visualization in Python.
Understanding Matplotlib.artist.Artist.update() and Its Importance
Matplotlib.artist.Artist.update() is a method that belongs to the Artist class in Matplotlib. The Artist class is the base class for all visible elements in a Matplotlib figure, including lines, text, patches, and more. The update() method allows you to modify the properties of these Artist objects after they have been created, providing a flexible way to adjust your visualizations dynamically.
The importance of Matplotlib.artist.Artist.update() lies in its ability to:
- Modify existing plot elements without recreating them
- Update multiple properties of an Artist object in a single call
- Improve performance by avoiding unnecessary redraws
- Enable interactive and animated visualizations
Let’s dive deeper into how Matplotlib.artist.Artist.update() works and explore its various applications.
Basic Syntax and Usage of Matplotlib.artist.Artist.update()
The basic syntax for using Matplotlib.artist.Artist.update() is as follows:
artist.update(props)
Where artist
is an instance of an Artist object, and props
is a dictionary containing the properties you want to update and their new values.
Here’s a simple example to illustrate the usage of Matplotlib.artist.Artist.update():
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot a line
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Original Line')
# Update the line properties
line.update({'color': 'red', 'linewidth': 2, 'linestyle': '--'})
plt.title('How to use Matplotlib.artist.Artist.update() - how2matplotlib.com')
plt.legend()
plt.show()
Output:
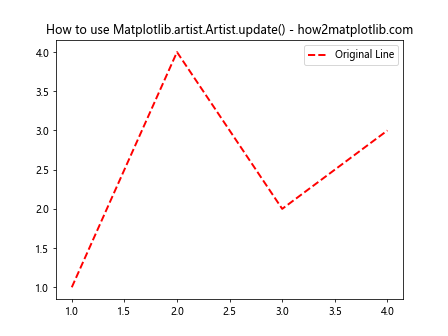
In this example, we create a simple line plot and then use Matplotlib.artist.Artist.update() to change the color, line width, and line style of the line. The update() method allows us to modify multiple properties in a single call, making it more efficient than setting each property individually.
Updating Different Types of Artists with Matplotlib.artist.Artist.update()
Matplotlib.artist.Artist.update() can be used with various types of Artist objects. Let’s explore how to update different types of artists using this method.
Updating Line2D Objects
Line2D objects represent lines in a plot. Here’s an example of updating a Line2D object:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
# Create a line
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Original Line')
# Update line properties
line.update({
'color': 'blue',
'linewidth': 3,
'linestyle': ':',
'marker': 'o',
'markersize': 8,
'markerfacecolor': 'red',
'markeredgecolor': 'black'
})
plt.title('Updating Line2D with Matplotlib.artist.Artist.update() - how2matplotlib.com')
plt.legend()
plt.show()
Output:
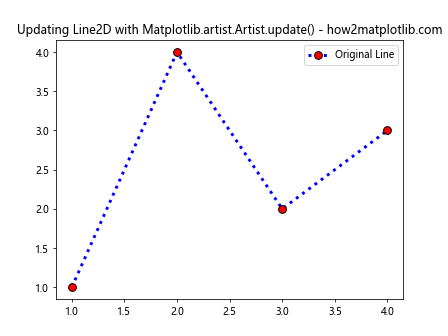
In this example, we use Matplotlib.artist.Artist.update() to modify various properties of the Line2D object, including color, line width, line style, marker type, marker size, and marker colors.
Updating Text Objects
Text objects represent text elements in a plot. Here’s how you can update a Text object:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
# Create a text object
text = ax.text(0.5, 0.5, 'Original Text', ha='center', va='center')
# Update text properties
text.update({
'text': 'Updated Text',
'fontsize': 16,
'color': 'green',
'rotation': 45,
'fontweight': 'bold',
'fontstyle': 'italic'
})
plt.title('Updating Text with Matplotlib.artist.Artist.update() - how2matplotlib.com')
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.show()
Output:
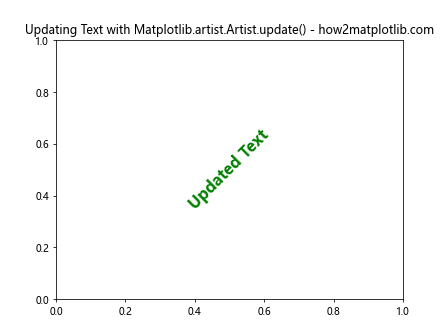
In this example, we use Matplotlib.artist.Artist.update() to change the text content, font size, color, rotation, font weight, and font style of a Text object.
Updating Patch Objects
Patch objects represent filled shapes in a plot. Here’s an example of updating a Rectangle patch:
import matplotlib.pyplot as plt
from matplotlib.patches import Rectangle
fig, ax = plt.subplots()
# Create a rectangle patch
rect = Rectangle((0.2, 0.2), 0.6, 0.6, facecolor='blue', edgecolor='black')
ax.add_patch(rect)
# Update patch properties
rect.update({
'facecolor': 'yellow',
'edgecolor': 'red',
'linewidth': 3,
'alpha': 0.7,
'linestyle': '--'
})
plt.title('Updating Patch with Matplotlib.artist.Artist.update() - how2matplotlib.com')
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.show()
Output:
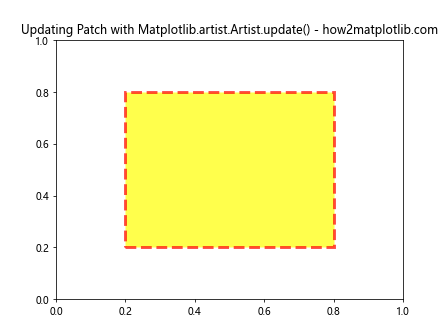
In this example, we use Matplotlib.artist.Artist.update() to modify the face color, edge color, line width, transparency, and line style of a Rectangle patch.
Advanced Techniques with Matplotlib.artist.Artist.update()
Now that we’ve covered the basics, let’s explore some advanced techniques using Matplotlib.artist.Artist.update().
Updating Multiple Artists at Once
You can update multiple artists simultaneously using a loop or list comprehension. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
# Create multiple lines
lines = [ax.plot(np.random.rand(10))[0] for _ in range(5)]
# Update all lines at once
for line in lines:
line.update({
'color': np.random.rand(3),
'linewidth': np.random.randint(1, 5),
'linestyle': np.random.choice(['-', '--', ':', '-.'])
})
plt.title('Updating Multiple Artists with Matplotlib.artist.Artist.update() - how2matplotlib.com')
plt.show()
Output:
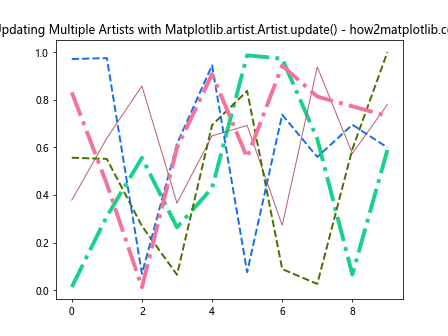
In this example, we create multiple lines and update their properties using Matplotlib.artist.Artist.update() in a loop, assigning random colors, line widths, and line styles to each line.
Updating Artists Based on Data
You can use Matplotlib.artist.Artist.update() to update artists based on changing data. Here’s an example of a simple animation:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
# Create initial line
x = np.linspace(0, 2*np.pi, 100)
line, = ax.plot(x, np.sin(x))
def update(frame):
# Update line data
y = np.sin(x + frame/10)
line.set_ydata(y)
# Update line properties based on data
line.update({
'color': plt.cm.viridis(frame/100),
'linewidth': 1 + frame/50
})
return line,
ani = FuncAnimation(fig, update, frames=100, interval=50, blit=True)
plt.title('Updating Artists Based on Data with Matplotlib.artist.Artist.update() - how2matplotlib.com')
plt.show()
Output:
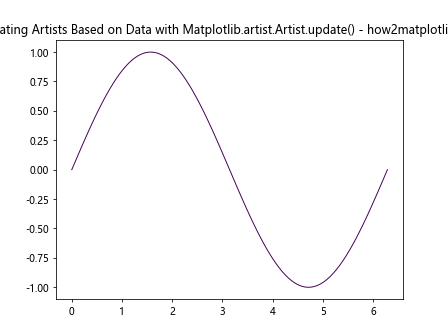
In this example, we create an animation where the line’s data and properties are updated based on the current frame. Matplotlib.artist.Artist.update() is used to change the line’s color and width dynamically.
Updating Artists in Response to User Interaction
Matplotlib.artist.Artist.update() can be used to create interactive visualizations that respond to user input. Here’s an example of a plot that updates when the user clicks on it:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
# Create initial scatter plot
x = np.random.rand(20)
y = np.random.rand(20)
scatter = ax.scatter(x, y, c='blue', s=50)
def on_click(event):
if event.inaxes == ax:
# Add new point
new_x, new_y = event.xdata, event.ydata
x.append(new_x)
y.append(new_y)
# Update scatter plot
scatter.set_offsets(np.column_stack((x, y)))
scatter.update({
'sizes': np.linspace(20, 200, len(x)),
'color': plt.cm.viridis(np.linspace(0, 1, len(x)))
})
fig.canvas.draw_idle()
fig.canvas.mpl_connect('button_press_event', on_click)
plt.title('Interactive Plot with Matplotlib.artist.Artist.update() - how2matplotlib.com')
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.show()
Output:
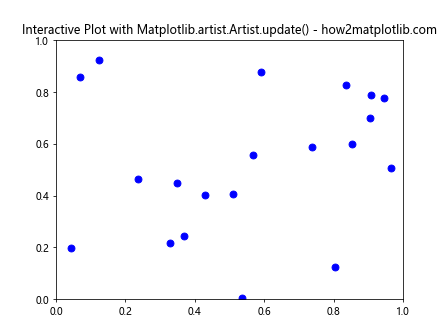
In this example, we create an interactive scatter plot that adds new points when the user clicks on the plot. Matplotlib.artist.Artist.update() is used to update the sizes and colors of the scatter points based on the number of points.
Best Practices and Performance Considerations
When using Matplotlib.artist.Artist.update(), it’s important to keep in mind some best practices and performance considerations:
- Update multiple properties at once: Instead of calling update() multiple times for different properties, combine them into a single dictionary to improve performance.
Use set
_*
methods for single property updates: For updating a single property, using the specific set_* method (e.g., set_color()) can be more efficient than update().Avoid unnecessary updates: Only update properties that have actually changed to prevent unnecessary redraws.
Use blitting for animations: When creating animations, use the blit parameter in FuncAnimation to improve performance by only redrawing the changed parts of the plot.
Consider using collections: For large numbers of similar artists (e.g., many scatter points), using collections can be more efficient than individual artists.
Here’s an example demonstrating some of these best practices: