Comprehensive Guide to Matplotlib.axis.Axis.format_cursor_data() Function in Python
Matplotlib.axis.Axis.format_cursor_data() function in Python is an essential tool for customizing the display of cursor data in Matplotlib plots. This function allows developers to format the data displayed when hovering over points on a plot, providing a more user-friendly and informative experience. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.format_cursor_data() function in depth, covering its usage, parameters, and various applications in data visualization.
Understanding the Matplotlib.axis.Axis.format_cursor_data() Function
The Matplotlib.axis.Axis.format_cursor_data() function is a method of the Axis class in Matplotlib. Its primary purpose is to format the data displayed when the cursor hovers over a point on the plot. By default, Matplotlib displays the raw coordinate values, but this function allows you to customize the format of these values.
Let’s start with a simple example to illustrate the basic usage of the Matplotlib.axis.Axis.format_cursor_data() function:
import matplotlib.pyplot as plt
import numpy as np
def format_coord(x, y):
return f'x={x:.2f}, y={y:.2f} (how2matplotlib.com)'
fig, ax = plt.subplots()
ax.plot(np.random.rand(10), np.random.rand(10), 'o')
ax.format_coord = format_coord
plt.title('Basic Matplotlib.axis.Axis.format_cursor_data() Example')
plt.show()
Output:
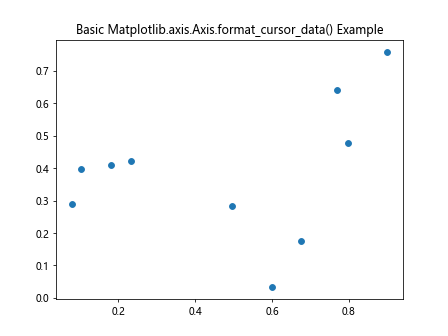
In this example, we define a custom format_coord function that formats the x and y coordinates with two decimal places. We then assign this function to the format_coord attribute of the axis object. This customizes the display of cursor data when hovering over the plot.
Customizing Cursor Data Format with Matplotlib.axis.Axis.format_cursor_data()
The Matplotlib.axis.Axis.format_cursor_data() function provides great flexibility in formatting cursor data. You can customize the display to show additional information, change the number format, or even add custom labels.
Here’s an example that demonstrates more advanced formatting:
import matplotlib.pyplot as plt
import numpy as np
def format_cursor_data(x):
return f'Value: {x:.3f} units (how2matplotlib.com)'
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y = np.sin(x)
line, = ax.plot(x, y)
ax.xaxis.format_cursor_data = format_cursor_data
ax.yaxis.format_cursor_data = format_cursor_data
plt.title('Advanced Matplotlib.axis.Axis.format_cursor_data() Example')
plt.show()
Output:
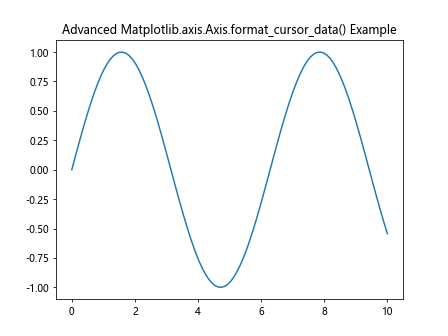
In this example, we define a custom format_cursor_data function that adds the word “units” to the formatted value. We then apply this function to both the x and y axes separately.
Applying Matplotlib.axis.Axis.format_cursor_data() to Different Plot Types
The Matplotlib.axis.Axis.format_cursor_data() function can be applied to various types of plots in Matplotlib. Let’s explore how to use it with different plot types.
Scatter Plots
Scatter plots are commonly used to display the relationship between two variables. Here’s how you can customize the cursor data format for a scatter plot:
import matplotlib.pyplot as plt
import numpy as np
def format_scatter_data(x):
return f'Point: {x:.2f} (how2matplotlib.com)'
fig, ax = plt.subplots()
x = np.random.rand(50)
y = np.random.rand(50)
scatter = ax.scatter(x, y, c=np.random.rand(50), s=500*np.random.rand(50))
ax.xaxis.format_cursor_data = format_scatter_data
ax.yaxis.format_cursor_data = format_scatter_data
plt.title('Scatter Plot with Matplotlib.axis.Axis.format_cursor_data()')
plt.colorbar(scatter)
plt.show()
Output:
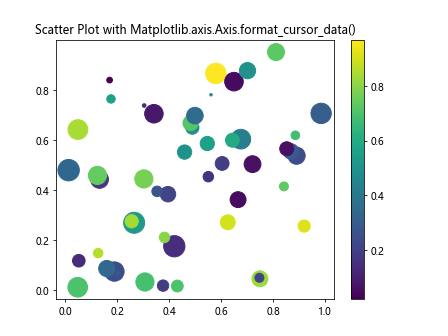
In this example, we create a scatter plot with randomly colored and sized points. The cursor data format is customized to display “Point: ” before the coordinate value.
Bar Charts
Bar charts are useful for comparing quantities across different categories. Here’s how to apply the Matplotlib.axis.Axis.format_cursor_data() function to a bar chart:
import matplotlib.pyplot as plt
import numpy as np
def format_bar_data(x):
return f'Bar height: {x:.1f} (how2matplotlib.com)'
fig, ax = plt.subplots()
categories = ['A', 'B', 'C', 'D', 'E']
values = np.random.randint(1, 100, 5)
bars = ax.bar(categories, values)
ax.yaxis.format_cursor_data = format_bar_data
plt.title('Bar Chart with Matplotlib.axis.Axis.format_cursor_data()')
plt.show()
Output:
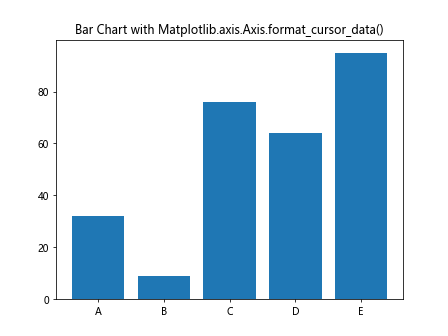
In this bar chart example, we customize the y-axis cursor data to display “Bar height: ” followed by the value with one decimal place.
Line Plots
Line plots are commonly used to show trends over time or other continuous variables. Here’s how to use the Matplotlib.axis.Axis.format_cursor_data() function with a line plot:
import matplotlib.pyplot as plt
import numpy as np
def format_line_data(x):
return f'Data point: {x:.3f} (how2matplotlib.com)'
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y = np.sin(x) * np.exp(-0.1 * x)
line, = ax.plot(x, y)
ax.xaxis.format_cursor_data = format_line_data
ax.yaxis.format_cursor_data = format_line_data
plt.title('Line Plot with Matplotlib.axis.Axis.format_cursor_data()')
plt.show()
Output:
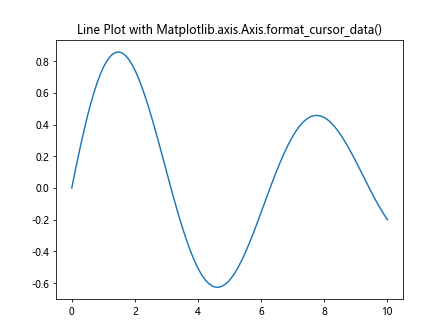
In this line plot example, we format both x and y axis cursor data to display “Data point: ” followed by the value with three decimal places.
Advanced Techniques with Matplotlib.axis.Axis.format_cursor_data()
The Matplotlib.axis.Axis.format_cursor_data() function can be used in more advanced ways to provide even more informative cursor data. Let’s explore some advanced techniques.
Displaying Multiple Data Points
In some cases, you might want to display information about multiple data points when hovering over a plot. Here’s an example that shows how to achieve this:
import matplotlib.pyplot as plt
import numpy as np
def format_multi_data(x):
index = np.argmin(np.abs(x_data - x))
return f'X: {x_data[index]:.2f}, Y1: {y1_data[index]:.2f}, Y2: {y2_data[index]:.2f} (how2matplotlib.com)'
fig, ax = plt.subplots()
x_data = np.linspace(0, 10, 100)
y1_data = np.sin(x_data)
y2_data = np.cos(x_data)
ax.plot(x_data, y1_data, label='sin')
ax.plot(x_data, y2_data, label='cos')
ax.xaxis.format_cursor_data = format_multi_data
plt.title('Multiple Data Points with Matplotlib.axis.Axis.format_cursor_data()')
plt.legend()
plt.show()
Output:
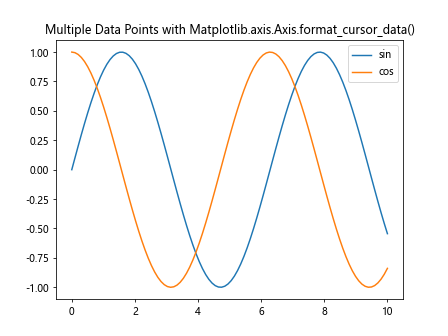
In this example, we plot two lines (sine and cosine) and display the values of both functions for the x-coordinate under the cursor.
Formatting Date Data
When working with time series data, it’s often useful to format the date information in a readable way. Here’s how you can use the Matplotlib.axis.Axis.format_cursor_data() function with date data:
import matplotlib.pyplot as plt
import numpy as np
from datetime import datetime, timedelta
def format_date_data(x):
date = start_date + timedelta(days=int(x))
return f'Date: {date.strftime("%Y-%m-%d")}, Value: {y_data[int(x)]:.2f} (how2matplotlib.com)'
fig, ax = plt.subplots()
start_date = datetime(2023, 1, 1)
dates = [start_date + timedelta(days=i) for i in range(100)]
y_data = np.cumsum(np.random.randn(100))
ax.plot(dates, y_data)
ax.xaxis.format_cursor_data = format_date_data
plt.title('Date Formatting with Matplotlib.axis.Axis.format_cursor_data()')
plt.gcf().autofmt_xdate()
plt.show()
Output:
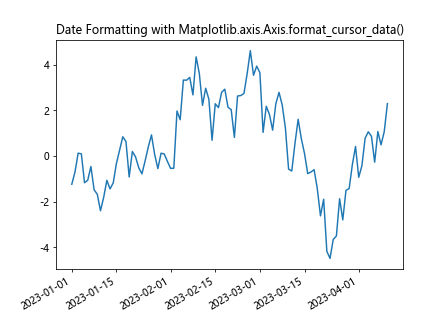
In this example, we create a time series plot and format the x-axis cursor data to display the date in a readable format along with the corresponding y-value.
Combining Matplotlib.axis.Axis.format_cursor_data() with Other Matplotlib Features
The Matplotlib.axis.Axis.format_cursor_data() function can be combined with other Matplotlib features to create more interactive and informative plots. Let’s explore some of these combinations.
Using format_cursor_data() with Subplots
When working with multiple subplots, you can apply different formatting to each subplot’s cursor data. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
def format_data_1(x):
return f'Subplot 1: {x:.2f} (how2matplotlib.com)'
def format_data_2(x):
return f'Subplot 2: {x:.3f} (how2matplotlib.com)'
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(8, 8))
x = np.linspace(0, 10, 100)
ax1.plot(x, np.sin(x))
ax2.plot(x, np.cos(x))
ax1.xaxis.format_cursor_data = format_data_1
ax2.xaxis.format_cursor_data = format_data_2
plt.suptitle('Subplots with Matplotlib.axis.Axis.format_cursor_data()')
plt.tight_layout()
plt.show()
Output:
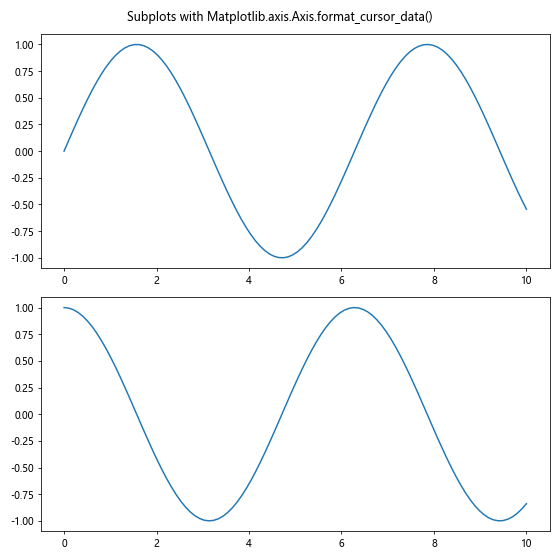
In this example, we create two subplots and apply different formatting to the cursor data of each subplot.
Combining format_cursor_data() with Annotations
You can use the Matplotlib.axis.Axis.format_cursor_data() function in combination with annotations to provide additional context to your plots. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
def format_annotated_data(x):
index = np.argmin(np.abs(x_data - x))
return f'X: {x_data[index]:.2f}, Y: {y_data[index]:.2f}, Annotation: {annotations[index]} (how2matplotlib.com)'
fig, ax = plt.subplots()
x_data = np.linspace(0, 10, 10)
y_data = np.sin(x_data)
annotations = ['Point ' + str(i) for i in range(10)]
ax.plot(x_data, y_data, 'o-')
for i, (x, y, annot) in enumerate(zip(x_data, y_data, annotations)):
ax.annotate(annot, (x, y), xytext=(5, 5), textcoords='offset points')
ax.xaxis.format_cursor_data = format_annotated_data
plt.title('Annotations with Matplotlib.axis.Axis.format_cursor_data()')
plt.show()
Output:
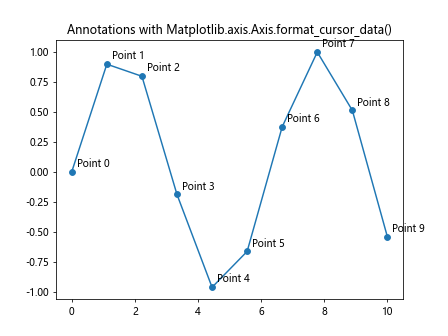
In this example, we create a plot with annotations and include the annotation text in the cursor data format.
Best Practices for Using Matplotlib.axis.Axis.format_cursor_data()
When using the Matplotlib.axis.Axis.format_cursor_data() function, it’s important to follow some best practices to ensure your plots are informative and user-friendly. Here are some tips:
- Keep it concise: While it’s tempting to include a lot of information in the cursor data, try to keep it concise and relevant. Too much information can be overwhelming for users.
Use appropriate precision: Format numerical values with an appropriate number of decimal places. Too many decimal places can be distracting, while too few might not provide enough precision.
Consider the context: Think about what information would be most useful to users in the context of your plot. For example, in a time series plot, showing the date and value might be more useful than showing raw x and y coordinates.
Be consistent: If you’re using the Matplotlib.axis.Axis.format_cursor_data() function across multiple plots in a project, try to maintain a consistent formatting style.
Handle edge cases: Make sure your formatting function can handle edge cases, such as NaN values or data points that fall outside the plot range.
Here’s an example that demonstrates these best practices: