How to Use Matplotlib.axes.Axes.remove_callback() in Python
Matplotlib.axes.Axes.remove_callback() in Python is an essential method for managing event callbacks in Matplotlib plots. This comprehensive guide will explore the intricacies of using Matplotlib.axes.Axes.remove_callback() in Python, providing detailed explanations and practical examples to help you master this powerful feature of Matplotlib.
Understanding Matplotlib.axes.Axes.remove_callback() in Python
Matplotlib.axes.Axes.remove_callback() in Python is a method that allows you to remove a previously registered callback function from a specific event on an Axes object. This method is particularly useful when you need to dynamically manage event handlers in your Matplotlib plots.
The syntax for Matplotlib.axes.Axes.remove_callback() in Python is as follows:
Axes.remove_callback(oid)
Where oid
is the callback id returned by add_callback()
when the callback was initially registered.
Let’s dive deeper into how Matplotlib.axes.Axes.remove_callback() in Python works and explore its various applications.
Registering Callbacks with Matplotlib.axes.Axes.add_callback()
Before we can use Matplotlib.axes.Axes.remove_callback() in Python, we need to understand how to register callbacks using the add_callback()
method. Here’s a simple example:
import matplotlib.pyplot as plt
def callback_function(ax):
print("Callback triggered for how2matplotlib.com")
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
callback_id = ax.add_callback(callback_function)
plt.show()
Output:
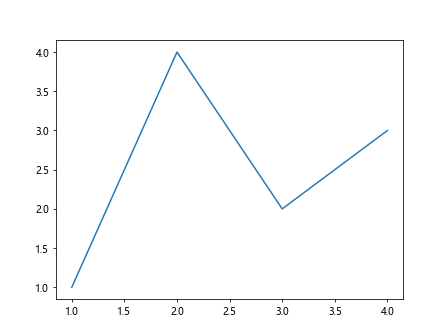
In this example, we define a simple callback function and register it using add_callback()
. The add_callback()
method returns a callback id, which we’ll use later with Matplotlib.axes.Axes.remove_callback() in Python.
Using Matplotlib.axes.Axes.remove_callback() in Python
Now that we have registered a callback, let’s see how to use Matplotlib.axes.Axes.remove_callback() in Python to remove it:
import matplotlib.pyplot as plt
def callback_function(ax):
print("Callback triggered for how2matplotlib.com")
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
callback_id = ax.add_callback(callback_function)
# Remove the callback
ax.remove_callback(callback_id)
plt.show()
Output:
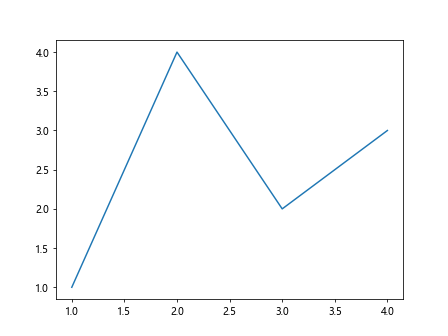
In this example, we first register the callback using add_callback()
, and then immediately remove it using Matplotlib.axes.Axes.remove_callback() in Python. This demonstrates how to dynamically manage callbacks in your Matplotlib plots.
Practical Applications of Matplotlib.axes.Axes.remove_callback() in Python
Matplotlib.axes.Axes.remove_callback() in Python has several practical applications in data visualization and interactive plotting. Let’s explore some of these use cases:
1. Temporary Event Handling
One common use case for Matplotlib.axes.Axes.remove_callback() in Python is handling temporary events. For example, you might want to add a callback that triggers only for a specific number of times:
import matplotlib.pyplot as plt
class CountdownCallback:
def __init__(self, max_count):
self.count = 0
self.max_count = max_count
def __call__(self, ax):
self.count += 1
print(f"Callback triggered {self.count} times for how2matplotlib.com")
if self.count >= self.max_count:
ax.remove_callback(self.callback_id)
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
countdown = CountdownCallback(3)
countdown.callback_id = ax.add_callback(countdown)
plt.show()
Output:
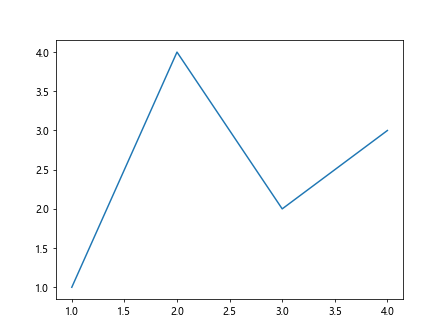
In this example, we create a CountdownCallback
class that removes itself after being triggered a specified number of times. This demonstrates how Matplotlib.axes.Axes.remove_callback() in Python can be used for self-managing callbacks.
2. Dynamic Callback Management
Matplotlib.axes.Axes.remove_callback() in Python is particularly useful when you need to dynamically add and remove callbacks based on certain conditions:
import matplotlib.pyplot as plt
import numpy as np
def on_click(event):
print(f"Clicked at x={event.xdata}, y={event.ydata} on how2matplotlib.com")
def toggle_callback(event):
global callback_id
if event.key == 't':
if callback_id is None:
callback_id = fig.canvas.mpl_connect('button_press_event', on_click)
print("Callback added for how2matplotlib.com")
else:
fig.canvas.mpl_disconnect(callback_id)
callback_id = None
print("Callback removed for how2matplotlib.com")
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
callback_id = None
fig.canvas.mpl_connect('key_press_event', toggle_callback)
plt.show()
Output:
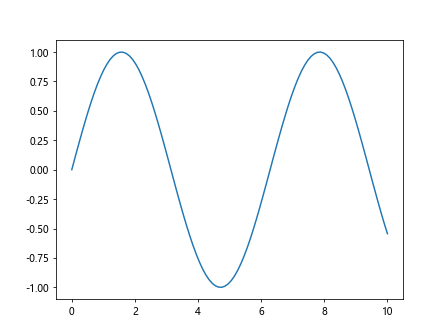
In this example, we use Matplotlib.axes.Axes.remove_callback() in Python (through mpl_disconnect()
) to toggle a click event callback on and off when the ‘t’ key is pressed. This demonstrates how to dynamically manage callbacks based on user input.
3. Conditional Callback Removal
Sometimes, you may want to remove a callback based on certain conditions within the callback itself. Here’s an example using Matplotlib.axes.Axes.remove_callback() in Python:
import matplotlib.pyplot as plt
import numpy as np
class ConditionalCallback:
def __init__(self, threshold):
self.threshold = threshold
self.count = 0
def __call__(self, event):
if event.ydata is not None and event.ydata > self.threshold:
self.count += 1
print(f"High value detected ({event.ydata}) at how2matplotlib.com")
if self.count >= 3:
event.canvas.mpl_disconnect(self.cid)
print("Callback removed after 3 high values for how2matplotlib.com")
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
callback = ConditionalCallback(0.8)
callback.cid = fig.canvas.mpl_connect('motion_notify_event', callback)
plt.show()
Output:
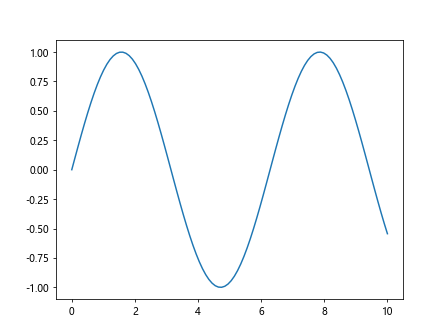
In this example, we create a ConditionalCallback
class that removes itself after detecting three high values. This demonstrates how Matplotlib.axes.Axes.remove_callback() in Python can be used for condition-based callback management.
Advanced Techniques with Matplotlib.axes.Axes.remove_callback() in Python
Now that we’ve covered the basics, let’s explore some advanced techniques using Matplotlib.axes.Axes.remove_callback() in Python.
1. Multiple Callback Management
When working with complex visualizations, you might need to manage multiple callbacks simultaneously. Here’s an example of how to use Matplotlib.axes.Axes.remove_callback() in Python with multiple callbacks:
import matplotlib.pyplot as plt
import numpy as np
def callback1(event):
print("Callback 1 triggered for how2matplotlib.com")
def callback2(event):
print("Callback 2 triggered for how2matplotlib.com")
def toggle_callbacks(event):
global callback_ids
if event.key == '1':
if 1 in callback_ids:
fig.canvas.mpl_disconnect(callback_ids[1])
del callback_ids[1]
print("Callback 1 removed for how2matplotlib.com")
else:
callback_ids[1] = fig.canvas.mpl_connect('button_press_event', callback1)
print("Callback 1 added for how2matplotlib.com")
elif event.key == '2':
if 2 in callback_ids:
fig.canvas.mpl_disconnect(callback_ids[2])
del callback_ids[2]
print("Callback 2 removed for how2matplotlib.com")
else:
callback_ids[2] = fig.canvas.mpl_connect('button_press_event', callback2)
print("Callback 2 added for how2matplotlib.com")
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
callback_ids = {}
fig.canvas.mpl_connect('key_press_event', toggle_callbacks)
plt.show()
Output:
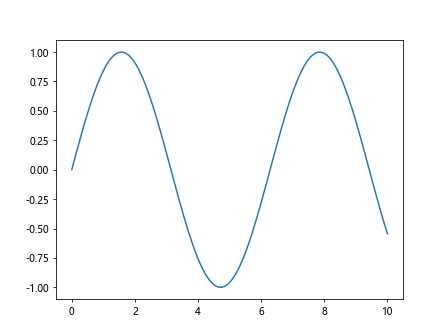
In this example, we manage two separate callbacks using a dictionary to store their ids. We can toggle each callback on and off independently using the ‘1’ and ‘2’ keys. This demonstrates how Matplotlib.axes.Axes.remove_callback() in Python can be used to manage multiple callbacks in a single application.
2. Callback Chaining
Another advanced technique is callback chaining, where one callback can trigger or remove another. Here’s an example using Matplotlib.axes.Axes.remove_callback() in Python:
import matplotlib.pyplot as plt
import numpy as np
class ChainedCallback:
def __init__(self):
self.stage = 1
def callback1(self, event):
print(f"Stage {self.stage} callback triggered for how2matplotlib.com")
if self.stage == 1:
event.canvas.mpl_disconnect(self.cid1)
self.cid2 = event.canvas.mpl_connect('button_press_event', self.callback2)
self.stage = 2
def callback2(self, event):
print(f"Stage {self.stage} callback triggered for how2matplotlib.com")
if self.stage == 2:
event.canvas.mpl_disconnect(self.cid2)
self.cid3 = event.canvas.mpl_connect('button_press_event', self.callback3)
self.stage = 3
def callback3(self, event):
print(f"Stage {self.stage} callback triggered for how2matplotlib.com")
print("All callbacks completed for how2matplotlib.com")
event.canvas.mpl_disconnect(self.cid3)
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
chained_callback = ChainedCallback()
chained_callback.cid1 = fig.canvas.mpl_connect('button_press_event', chained_callback.callback1)
plt.show()
Output:
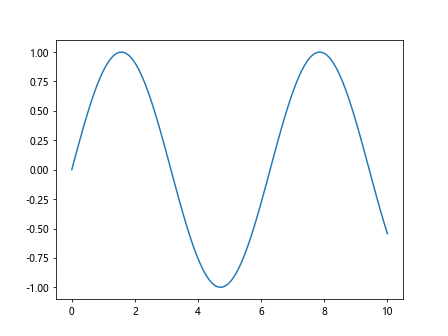
In this example, we create a ChainedCallback
class that progresses through three stages, each with its own callback. Each callback removes itself and adds the next one in the chain using Matplotlib.axes.Axes.remove_callback() in Python (through mpl_disconnect()
).
3. Timed Callback Removal
Sometimes, you might want to remove a callback after a certain amount of time has passed. Here’s an example of how to achieve this using Matplotlib.axes.Axes.remove_callback() in Python:
import matplotlib.pyplot as plt
import numpy as np
import time
class TimedCallback:
def __init__(self, duration):
self.start_time = time.time()
self.duration = duration
def __call__(self, event):
current_time = time.time()
elapsed_time = current_time - self.start_time
print(f"Callback triggered at {elapsed_time:.2f}s for how2matplotlib.com")
if elapsed_time >= self.duration:
event.canvas.mpl_disconnect(self.cid)
print(f"Callback removed after {self.duration}s for how2matplotlib.com")
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
timed_callback = TimedCallback(5) # Remove after 5 seconds
timed_callback.cid = fig.canvas.mpl_connect('button_press_event', timed_callback)
plt.show()
Output:
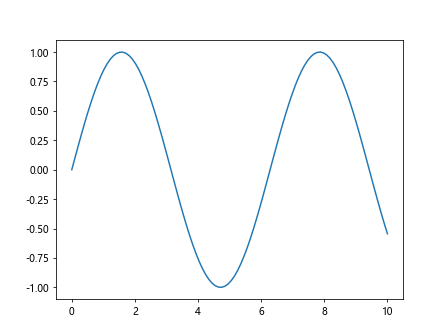
In this example, we create a TimedCallback
class that removes itself after a specified duration using Matplotlib.axes.Axes.remove_callback() in Python (through mpl_disconnect()
). This is useful for creating temporary event handlers that automatically clean up after themselves.
Troubleshooting Matplotlib.axes.Axes.remove_callback() in Python
When working with Matplotlib.axes.Axes.remove_callback() in Python, you may encounter some common issues. Here are some troubleshooting tips:
- Invalid callback id: If you try to remove a callback with an invalid id, you’ll get an error. Always ensure that you’re using the correct callback id.
Callback already removed: If you try to remove a callback that has already been removed, you’ll get an error. Keep track of which callbacks have been removed to avoid this issue.
Callback not triggering: If your callback isn’t triggering, make sure you’ve connected it to the correct event and that the event is actually occurring.
Memory leaks: If you’re not properly removing callbacks, you may experience memory leaks. Always remove callbacks when they’re no longer needed.
Here’s an example that demonstrates how to handle these issues: