Mastering Matplotlib.artist.Artist.set_sketch_params() in Python: A Comprehensive Guide
Matplotlib.artist.Artist.set_sketch_params() in Python is a powerful method that allows you to add sketch-like effects to your plots. This function is part of the Matplotlib library, which is widely used for creating static, animated, and interactive visualizations in Python. In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.artist.Artist.set_sketch_params(), providing detailed explanations and numerous examples to help you master this versatile tool.
Understanding Matplotlib.artist.Artist.set_sketch_params()
Matplotlib.artist.Artist.set_sketch_params() is a method that belongs to the Artist class in Matplotlib. It’s used to set the sketch parameters for the artist, which can create a hand-drawn or sketch-like appearance for various plot elements. This function is particularly useful when you want to give your visualizations a more organic, less computer-generated look.
The basic syntax of Matplotlib.artist.Artist.set_sketch_params() is as follows:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
artist = ax.plot([1, 2, 3], [1, 2, 3])[0]
artist.set_sketch_params(scale=1, length=10, randomness=1)
plt.title("How to use set_sketch_params() - how2matplotlib.com")
plt.show()
Output:
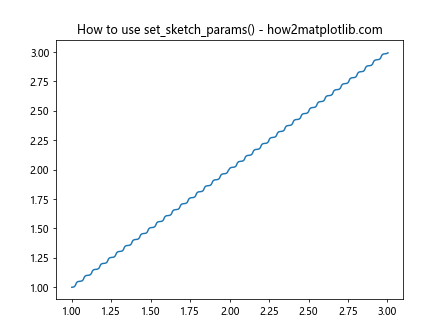
In this example, we create a simple line plot and apply sketch parameters to it. The scale
parameter controls the overall scale of the sketch effect, length
determines the length of the sketch strokes, and randomness
adds a random factor to the sketch effect.
Parameters of Matplotlib.artist.Artist.set_sketch_params()
Matplotlib.artist.Artist.set_sketch_params() accepts several parameters that allow you to fine-tune the sketch effect. Let’s explore each of these parameters in detail:
- scale: This parameter controls the overall scale of the sketch effect. A larger value will make the sketch effect more pronounced.
length: This parameter determines the length of the sketch strokes. Longer strokes can create a more exaggerated sketch effect.
randomness: This parameter adds a random factor to the sketch effect, making it look more natural and hand-drawn.
Let’s see how we can use these parameters in practice: