How to Master Matplotlib Textbox Widgets
Matplotlib Textbox Widgets are powerful tools for creating interactive visualizations in Python. These widgets allow users to input text directly into a plot, enabling dynamic updates and real-time data manipulation. In this comprehensive guide, we’ll explore the various aspects of Matplotlib Textbox Widgets, from basic implementation to advanced techniques. By the end of this article, you’ll have a thorough understanding of how to leverage these widgets to enhance your data visualization projects.
Understanding Matplotlib Textbox Widgets
Matplotlib Textbox Widgets are interactive elements that can be added to Matplotlib plots. They provide a way for users to input text directly into the plot, which can then be used to update the visualization or perform other actions. These widgets are part of the matplotlib.widgets
module and offer a range of customization options to suit different needs.
Let’s start with a simple example to illustrate how to create a basic Matplotlib Textbox Widget:
import matplotlib.pyplot as plt
from matplotlib.widgets import TextBox
fig, ax = plt.subplots()
ax.set_title("Matplotlib Textbox Widget Example - how2matplotlib.com")
initial_text = ""
textbox = TextBox(ax.inset_axes([0.1, 0.05, 0.8, 0.075]), "Enter text:", initial=initial_text)
def submit(text):
ax.clear()
ax.set_title("Matplotlib Textbox Widget Example - how2matplotlib.com")
ax.text(0.5, 0.5, f"You entered: {text}", ha='center', va='center')
plt.draw()
textbox.on_submit(submit)
plt.show()
Output:
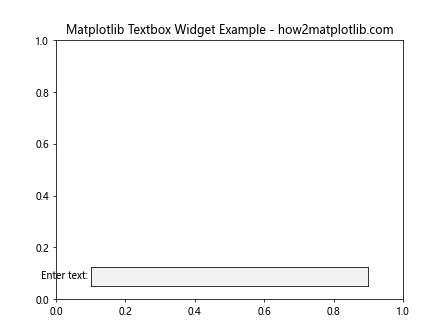
In this example, we create a simple Matplotlib plot with a Textbox Widget. The widget is positioned at the bottom of the plot using inset_axes
. When the user enters text and presses Enter, the submit
function is called, which updates the plot with the entered text.
Customizing Matplotlib Textbox Widgets
Matplotlib Textbox Widgets offer various customization options to tailor their appearance and behavior. Let’s explore some of these options:
Changing the Textbox Color
You can customize the color of the Textbox Widget to match your plot’s theme:
import matplotlib.pyplot as plt
from matplotlib.widgets import TextBox
fig, ax = plt.subplots()
ax.set_title("Customized Matplotlib Textbox Widget - how2matplotlib.com")
textbox = TextBox(ax.inset_axes([0.1, 0.05, 0.8, 0.075]), "Enter text:",
initial="how2matplotlib.com",
color='lightblue', hovercolor='lightgreen')
def submit(text):
ax.clear()
ax.set_title("Customized Matplotlib Textbox Widget - how2matplotlib.com")
ax.text(0.5, 0.5, f"You entered: {text}", ha='center', va='center')
plt.draw()
textbox.on_submit(submit)
plt.show()
Output:
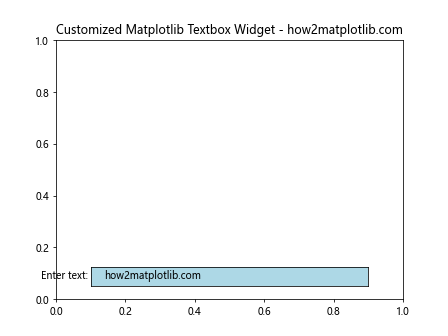
In this example, we set the color
and hovercolor
properties of the Textbox Widget to customize its appearance.
Adjusting Textbox Size and Position
You can control the size and position of the Textbox Widget within the plot:
import matplotlib.pyplot as plt
from matplotlib.widgets import TextBox
fig, ax = plt.subplots()
ax.set_title("Resized Matplotlib Textbox Widget - how2matplotlib.com")
textbox = TextBox(ax.inset_axes([0.2, 0.8, 0.6, 0.1]), "Enter text:",
initial="how2matplotlib.com")
def submit(text):
ax.clear()
ax.set_title("Resized Matplotlib Textbox Widget - how2matplotlib.com")
ax.text(0.5, 0.5, f"You entered: {text}", ha='center', va='center')
plt.draw()
textbox.on_submit(submit)
plt.show()
Output:
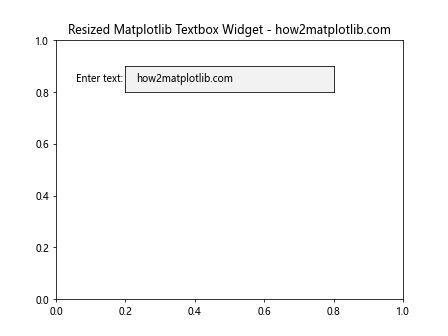
Here, we’ve adjusted the position and size of the Textbox Widget by modifying the parameters of inset_axes
.
Handling User Input with Matplotlib Textbox Widgets
One of the key features of Matplotlib Textbox Widgets is their ability to handle user input. Let’s explore different ways to process and respond to user input:
Real-time Updates
You can update the plot in real-time as the user types:
import matplotlib.pyplot as plt
from matplotlib.widgets import TextBox
fig, ax = plt.subplots()
ax.set_title("Real-time Matplotlib Textbox Widget - how2matplotlib.com")
textbox = TextBox(ax.inset_axes([0.1, 0.05, 0.8, 0.075]), "Enter text:",
initial="how2matplotlib.com")
def update(text):
ax.clear()
ax.set_title("Real-time Matplotlib Textbox Widget - how2matplotlib.com")
ax.text(0.5, 0.5, f"Current text: {text}", ha='center', va='center')
plt.draw()
textbox.on_text_change(update)
plt.show()
Output:
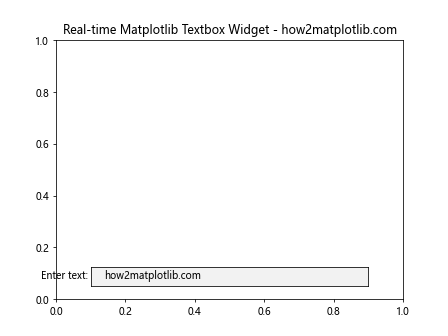
In this example, we use the on_text_change
method to update the plot every time the text in the Textbox Widget changes.
Numeric Input Validation
You can validate numeric input and use it to update the plot:
import matplotlib.pyplot as plt
from matplotlib.widgets import TextBox
import numpy as np
fig, ax = plt.subplots()
ax.set_title("Numeric Input Matplotlib Textbox Widget - how2matplotlib.com")
x = np.linspace(0, 10, 100)
line, = ax.plot(x, np.sin(x))
textbox = TextBox(ax.inset_axes([0.1, 0.05, 0.8, 0.075]), "Frequency:",
initial="1")
def update(text):
try:
freq = float(text)
line.set_ydata(np.sin(freq * x))
plt.draw()
except ValueError:
pass
textbox.on_submit(update)
plt.show()
Output:
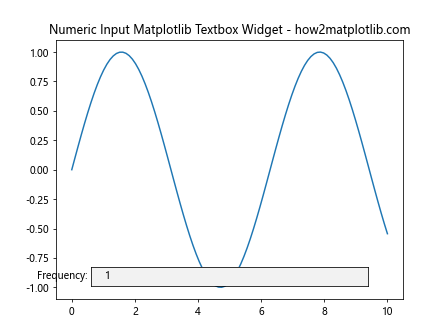
This example demonstrates how to use a Textbox Widget to adjust the frequency of a sine wave in real-time.
Advanced Techniques with Matplotlib Textbox Widgets
Now that we’ve covered the basics, let’s explore some advanced techniques for working with Matplotlib Textbox Widgets:
Multiple Textbox Widgets
You can use multiple Textbox Widgets to control different aspects of your plot:
import matplotlib.pyplot as plt
from matplotlib.widgets import TextBox
import numpy as np
fig, ax = plt.subplots()
ax.set_title("Multiple Matplotlib Textbox Widgets - how2matplotlib.com")
x = np.linspace(0, 10, 100)
line, = ax.plot(x, np.sin(x))
freq_textbox = TextBox(ax.inset_axes([0.1, 0.05, 0.3, 0.075]), "Frequency:",
initial="1")
amp_textbox = TextBox(ax.inset_axes([0.6, 0.05, 0.3, 0.075]), "Amplitude:",
initial="1")
def update(val):
try:
freq = float(freq_textbox.text)
amp = float(amp_textbox.text)
line.set_ydata(amp * np.sin(freq * x))
plt.draw()
except ValueError:
pass
freq_textbox.on_submit(update)
amp_textbox.on_submit(update)
plt.show()
Output:
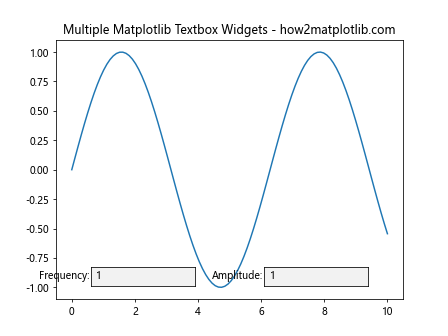
This example uses two Textbox Widgets to control both the frequency and amplitude of a sine wave.
Combining Textbox Widgets with Other Widgets
You can combine Textbox Widgets with other Matplotlib widgets for more complex interactions:
import matplotlib.pyplot as plt
from matplotlib.widgets import TextBox, Button
import numpy as np
fig, ax = plt.subplots()
ax.set_title("Combined Matplotlib Widgets - how2matplotlib.com")
x = np.linspace(0, 10, 100)
line, = ax.plot(x, np.sin(x))
textbox = TextBox(ax.inset_axes([0.1, 0.05, 0.3, 0.075]), "Frequency:",
initial="1")
button = Button(ax.inset_axes([0.6, 0.05, 0.3, 0.075]), "Reset")
def update(text):
try:
freq = float(text)
line.set_ydata(np.sin(freq * x))
plt.draw()
except ValueError:
pass
def reset(event):
textbox.set_val("1")
line.set_ydata(np.sin(x))
plt.draw()
textbox.on_submit(update)
button.on_clicked(reset)
plt.show()
Output:
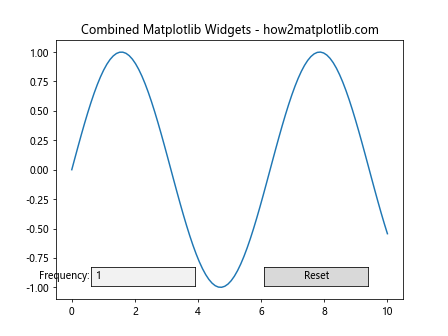
This example combines a Textbox Widget with a Button Widget to allow users to adjust the frequency of a sine wave and reset it to the default value.
Best Practices for Using Matplotlib Textbox Widgets
When working with Matplotlib Textbox Widgets, it’s important to follow some best practices to ensure your visualizations are effective and user-friendly:
- Provide clear labels: Always include a descriptive label for your Textbox Widget to indicate what type of input is expected.
Use appropriate input validation: Implement input validation to handle unexpected user input gracefully.
Position widgets thoughtfully: Place Textbox Widgets in locations that don’t obscure important parts of your plot.
Provide feedback: Use visual cues or text updates to confirm that user input has been processed.
Combine with other widgets: Consider using Textbox Widgets in conjunction with other widget types for more complex interactions.
Let’s implement these best practices in an example: