Comprehensive Guide to Matplotlib.axis.Axis.is_transform_set() Function in Python
Matplotlib.axis.Axis.is_transform_set() function in Python is an essential method in the Matplotlib library for working with axis transformations. This function is used to determine whether a transform has been set for an axis object. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.is_transform_set() function in detail, covering its usage, parameters, return values, and practical applications in various scenarios.
Understanding the Matplotlib.axis.Axis.is_transform_set() Function
The Matplotlib.axis.Axis.is_transform_set() function is a method of the Axis class in Matplotlib. It is used to check if a transform has been set for the axis. This function is particularly useful when you need to determine whether any custom transformations have been applied to an axis before performing further operations or modifications.
Let’s start with a simple example to demonstrate how to use the Matplotlib.axis.Axis.is_transform_set() function:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Check if a transform is set for the x-axis
is_transform_set = ax.xaxis.is_transform_set()
print(f"Is transform set for x-axis? {is_transform_set}")
plt.title("Matplotlib.axis.Axis.is_transform_set() Example - how2matplotlib.com")
plt.show()
Output:
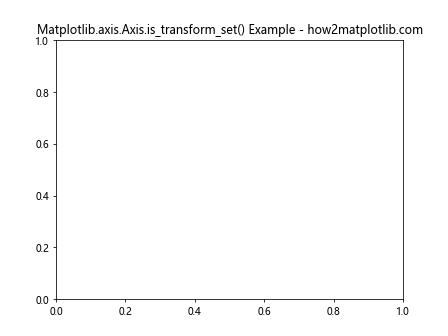
In this example, we create a figure and axis using plt.subplots(). Then, we use the is_transform_set() function to check if a transform has been set for the x-axis. The function returns a boolean value indicating whether a transform is set or not.
Exploring the Parameters of Matplotlib.axis.Axis.is_transform_set()
The Matplotlib.axis.Axis.is_transform_set() function doesn’t take any parameters. It is a simple method that returns a boolean value based on the internal state of the axis object.
Let’s look at another example to illustrate this:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Check if a transform is set for the y-axis
is_y_transform_set = ax.yaxis.is_transform_set()
print(f"Is transform set for y-axis? {is_y_transform_set}")
plt.title("Matplotlib.axis.Axis.is_transform_set() Parameter Example - how2matplotlib.com")
plt.show()
Output:
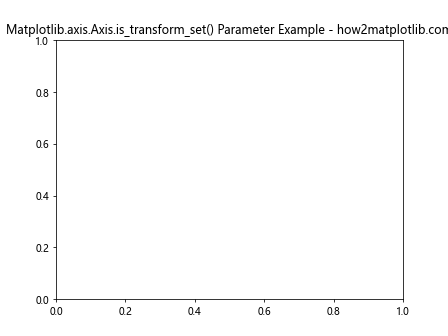
In this example, we check if a transform is set for the y-axis using the is_transform_set() function. As you can see, we don’t pass any parameters to the function.
Return Value of Matplotlib.axis.Axis.is_transform_set()
The Matplotlib.axis.Axis.is_transform_set() function returns a boolean value:
- True: If a transform has been set for the axis
- False: If no transform has been set for the axis
Let’s demonstrate this with an example:
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
# Create a figure and axis
fig, ax = plt.subplots()
# Check if a transform is set for the x-axis before setting a transform
is_transform_set_before = ax.xaxis.is_transform_set()
print(f"Is transform set for x-axis before? {is_transform_set_before}")
# Set a transform for the x-axis
ax.xaxis.set_transform(transforms.Affine2D().scale(2, 1) + ax.transData)
# Check if a transform is set for the x-axis after setting a transform
is_transform_set_after = ax.xaxis.is_transform_set()
print(f"Is transform set for x-axis after? {is_transform_set_after}")
plt.title("Matplotlib.axis.Axis.is_transform_set() Return Value Example - how2matplotlib.com")
plt.show()
Output:
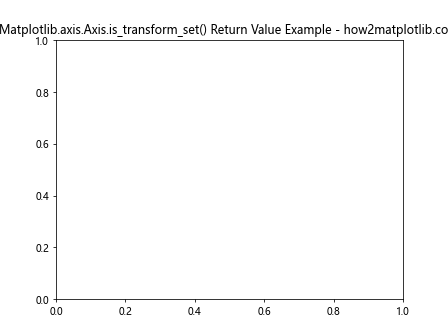
In this example, we first check if a transform is set for the x-axis before setting any transform. Then, we set a transform using the set_transform() method. After setting the transform, we check again using is_transform_set(). The return values will be different before and after setting the transform.
Practical Applications of Matplotlib.axis.Axis.is_transform_set()
The Matplotlib.axis.Axis.is_transform_set() function has several practical applications in data visualization and plot customization. Let’s explore some of these applications with examples.
1. Conditional Axis Transformation
One common use case for is_transform_set() is to apply a transformation only if one hasn’t been set already. This can be useful when you want to ensure that you don’t overwrite any existing transformations.
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
# Create a figure and axis
fig, ax = plt.subplots()
# Check if a transform is set for the x-axis
if not ax.xaxis.is_transform_set():
# Apply a transformation only if one hasn't been set
ax.xaxis.set_transform(transforms.Affine2D().scale(2, 1) + ax.transData)
plt.plot([1, 2, 3], [1, 2, 3], label="Data")
plt.title("Conditional Axis Transformation - how2matplotlib.com")
plt.legend()
plt.show()
Output:
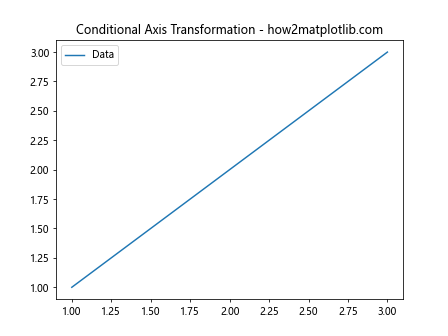
In this example, we check if a transform is set for the x-axis using is_transform_set(). If no transform is set, we apply a scaling transformation to the x-axis.
2. Debugging Axis Transformations
The is_transform_set() function can be useful for debugging purposes, especially when working with complex plots that involve multiple transformations.
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
# Create a figure and axis
fig, ax = plt.subplots()
# Apply some transformations
ax.xaxis.set_transform(transforms.Affine2D().scale(2, 1) + ax.transData)
ax.yaxis.set_transform(transforms.Affine2D().translate(0, 1) + ax.transData)
# Debug transformations
print(f"X-axis transform set: {ax.xaxis.is_transform_set()}")
print(f"Y-axis transform set: {ax.yaxis.is_transform_set()}")
plt.plot([1, 2, 3], [1, 2, 3], label="Data")
plt.title("Debugging Axis Transformations - how2matplotlib.com")
plt.legend()
plt.show()
Output:
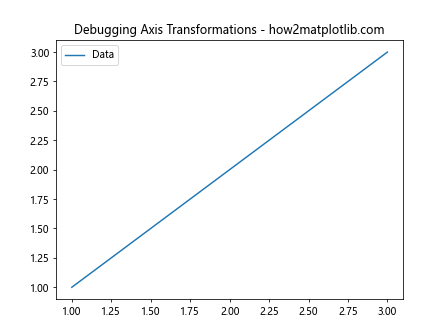
In this example, we apply different transformations to the x-axis and y-axis. We then use is_transform_set() to verify that the transformations have been applied correctly.
3. Resetting Axis Transformations
The is_transform_set() function can be used in conjunction with other methods to reset axis transformations when needed.
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
# Create a figure and axis
fig, ax = plt.subplots()
# Apply a transformation
ax.xaxis.set_transform(transforms.Affine2D().scale(2, 1) + ax.transData)
# Check if transform is set
print(f"Transform set before reset: {ax.xaxis.is_transform_set()}")
# Reset the transformation if it's set
if ax.xaxis.is_transform_set():
ax.xaxis.set_transform(None)
# Check if transform is set after reset
print(f"Transform set after reset: {ax.xaxis.is_transform_set()}")
plt.plot([1, 2, 3], [1, 2, 3], label="Data")
plt.title("Resetting Axis Transformations - how2matplotlib.com")
plt.legend()
plt.show()
Output:
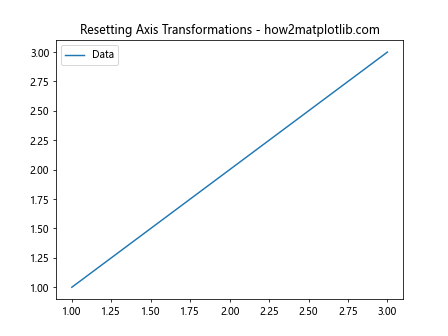
In this example, we first apply a transformation to the x-axis. We then use is_transform_set() to check if a transform is set, and if it is, we reset it by setting the transform to None.
Advanced Usage of Matplotlib.axis.Axis.is_transform_set()
While the Matplotlib.axis.Axis.is_transform_set() function is relatively simple, it can be used in more advanced scenarios when working with complex plots and custom visualizations. Let’s explore some of these advanced use cases.
Combining Multiple Axis Transformations
When working with complex plots, you might need to apply multiple transformations to an axis. The is_transform_set() function can help you manage these transformations effectively.
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
# Create a figure and axis
fig, ax = plt.subplots()
# Define multiple transformations
scale_transform = transforms.Affine2D().scale(2, 1)
rotate_transform = transforms.Affine2D().rotate_deg(30)
# Apply transformations conditionally
if not ax.xaxis.is_transform_set():
ax.xaxis.set_transform(scale_transform + rotate_transform + ax.transData)
# Check if transform is set
print(f"X-axis transform set: {ax.xaxis.is_transform_set()}")
plt.plot([1, 2, 3], [1, 2, 3], label="Data")
plt.title("Combining Multiple Axis Transformations - how2matplotlib.com")
plt.legend()
plt.show()
Output:
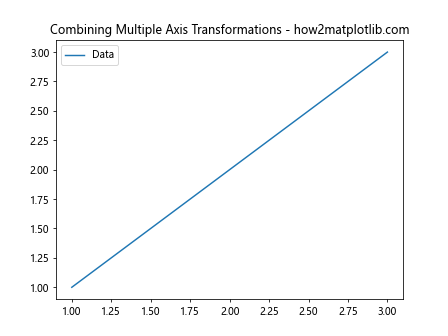
In this example, we define two separate transformations: a scaling transformation and a rotation transformation. We then combine these transformations and apply them to the x-axis only if no transform has been set previously.
Best Practices for Using Matplotlib.axis.Axis.is_transform_set()
When working with the Matplotlib.axis.Axis.is_transform_set() function, there are several best practices to keep in mind:
- Always check before applying transformations: Use is_transform_set() to check if a transform has already been applied before setting a new one. This helps prevent unintended overwriting of existing transformations.
Use in conjunction with other transform methods: Combine is_transform_set() with other transform-related methods like set_transform(), get_transform(), and set_scale() for more comprehensive axis management.
Be aware of the transform stack: Remember that Matplotlib uses a transform stack, and is_transform_set() only checks if any transform has been set, not the specific details of the transform.
Use for debugging: Utilize is_transform_set() in your debugging process when working with complex plots to ensure transformations are applied as expected.
Consider performance: While is_transform_set() is a lightweight function, avoid calling it unnecessarily in tight loops or performance-critical sections of your code.
Let’s look at an example that demonstrates some of these best practices: