How to Use Matplotlib.artist.Artist.get_gid() in Python
Matplotlib.artist.Artist.get_gid() in Python is an essential method for working with graphics in Matplotlib. This comprehensive guide will explore the various aspects of using Matplotlib.artist.Artist.get_gid() in Python, providing detailed explanations and practical examples to help you master this powerful feature.
Understanding Matplotlib.artist.Artist.get_gid() in Python
Matplotlib.artist.Artist.get_gid() in Python is a method that belongs to the Artist class in Matplotlib. It is used to retrieve the group id (gid) of an artist object. The gid is a string that can be used to identify and group related artists in a Matplotlib figure. This method is particularly useful when you need to manipulate or reference specific elements in your plots.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.get_gid() in Python:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
line[0].set_gid('my_line')
gid = line[0].get_gid()
print(f"The gid of the line is: {gid}")
plt.show()
Output:
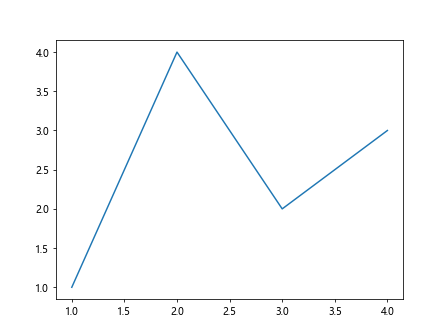
In this example, we create a simple line plot and set the gid of the line to ‘my_line’. We then use Matplotlib.artist.Artist.get_gid() in Python to retrieve the gid and print it. This demonstrates the basic usage of the method.
Setting and Getting GIDs with Matplotlib.artist.Artist.get_gid() in Python
To fully utilize Matplotlib.artist.Artist.get_gid() in Python, it’s important to understand how to set and get gids for different types of artists. Let’s explore this with various examples:
Setting and Getting GIDs for Text Objects
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
text = ax.text(0.5, 0.5, 'how2matplotlib.com', ha='center', va='center')
text.set_gid('my_text')
gid = text.get_gid()
print(f"The gid of the text is: {gid}")
plt.show()
Output:
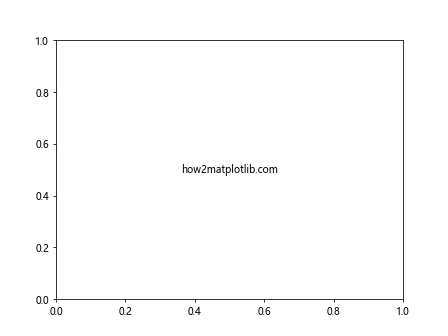
In this example, we create a text object, set its gid, and then use Matplotlib.artist.Artist.get_gid() in Python to retrieve it.
Setting and Getting GIDs for Patches
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.2, fill=False)
ax.add_patch(circle)
circle.set_gid('my_circle')
gid = circle.get_gid()
print(f"The gid of the circle is: {gid}")
plt.show()
Output:
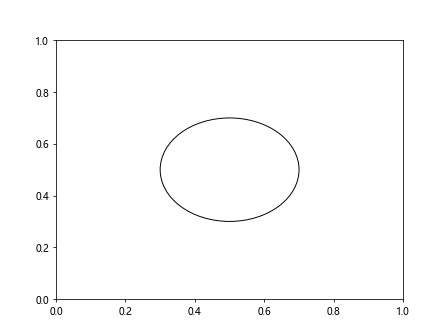
Here, we create a circle patch, add it to the axes, set its gid, and then use Matplotlib.artist.Artist.get_gid() in Python to retrieve it.
Advanced Usage of Matplotlib.artist.Artist.get_gid() in Python
Now that we’ve covered the basics, let’s explore some more advanced uses of Matplotlib.artist.Artist.get_gid() in Python.
Using GIDs for Selective Styling
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
lines = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], 'r-', [1, 2, 3, 4], [2, 3, 4, 1], 'b-')
lines[0].set_gid('line1')
lines[1].set_gid('line2')
for line in lines:
if line.get_gid() == 'line1':
line.set_linewidth(3)
elif line.get_gid() == 'line2':
line.set_linestyle('--')
plt.title('how2matplotlib.com')
plt.show()
Output:
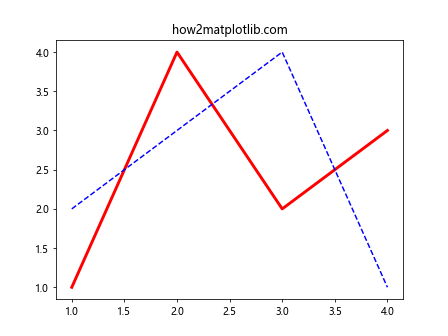
In this example, we use Matplotlib.artist.Artist.get_gid() in Python to selectively style different lines based on their gids.
Using GIDs with Event Handling
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line = ax.plot([1, 2, 3, 4], [1, 4, 2, 3])[0]
line.set_gid('interactive_line')
def on_pick(event):
if event.artist.get_gid() == 'interactive_line':
print("You clicked on the line!")
fig.canvas.mpl_connect('pick_event', on_pick)
line.set_picker(5) # Make the line pickable
plt.title('how2matplotlib.com - Click the line!')
plt.show()
Output:
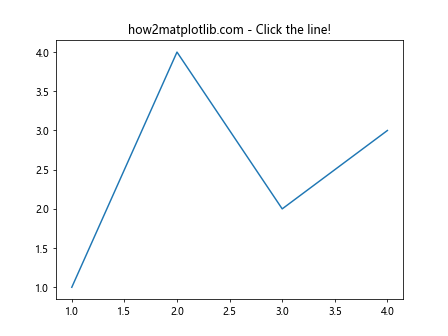
This example demonstrates how to use Matplotlib.artist.Artist.get_gid() in Python with event handling to create interactive plots.
Best Practices for Using Matplotlib.artist.Artist.get_gid() in Python
When working with Matplotlib.artist.Artist.get_gid() in Python, it’s important to follow some best practices to ensure your code is efficient and maintainable:
- Use descriptive gids: Choose gid names that clearly describe the purpose or content of the artist.
Be consistent: Use a consistent naming convention for your gids across your project.
Don’t overuse: Only set gids for artists that you actually need to reference or manipulate later.
Check for None: Remember that get_gid() may return None if no gid has been set.
Let’s see an example that incorporates these best practices: