How to Add Title to Subplots in Matplotlib
Matplotlib is a powerful library for creating static, interactive, and animated visualizations in Python. One common task when using Matplotlib is adding titles to subplots within a figure. Titles help to clearly indicate what each subplot represents, making the visualizations more understandable. This article will guide you through various methods to add titles to subplots in Matplotlib, providing detailed examples for each method.
1. Basic Subplot Titles
The simplest way to add a title to a subplot in Matplotlib is by using the set_title
method of the axes object. Here’s how you can create a figure with multiple subplots and add a title to each subplot.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add titles to each subplot
axs[0, 0].set_title('Top Left - how2matplotlib.com')
axs[0, 1].set_title('Top Right - how2matplotlib.com')
axs[1, 0].set_title('Bottom Left - how2matplotlib.com')
axs[1, 1].set_title('Bottom Right - how2matplotlib.com')
# Display the figure
plt.show()
Output:
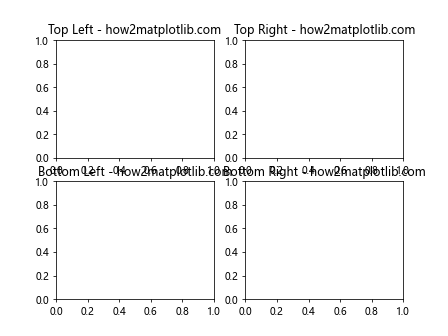
2. Adjusting Title Position
Sometimes, the default position of the title may not be ideal, especially when dealing with overlapping elements or specific layout requirements. You can adjust the position of the title using the loc
and pad
parameters.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add titles with adjusted positions
axs[0, 0].set_title('Top Left - how2matplotlib.com', loc='left', pad=20)
axs[0, 1].set_title('Top Right - how2matplotlib.com', loc='right', pad=20)
axs[1, 0].set_title('Bottom Left - how2matplotlib.com', loc='left', pad=20)
axs[1, 1].set_title('Bottom Right - how2matplotlib.com', loc='right', pad=20)
# Display the figure
plt.show()
Output:
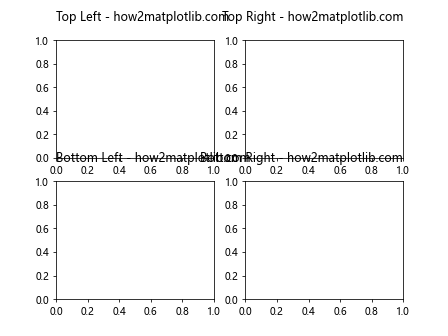
3. Styling Titles
Matplotlib allows you to style the titles by adjusting font properties, colors, and more. This can be useful for making certain titles stand out or to match specific styling guidelines.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add styled titles
axs[0, 0].set_title('Top Left - how2matplotlib.com', fontsize=14, color='red', fontweight='bold')
axs[0, 1].set_title('Top Right - how2matplotlib.com', fontsize=14, color='blue', fontweight='bold')
axs[1, 0].set_title('Bottom Left - how2matplotlib.com', fontsize=14, color='green', fontweight='bold')
axs[1, 1].set_title('Bottom Right - how2matplotlib.com', fontsize=14, color='purple', fontweight='bold')
# Display the figure
plt.show()
4. Using suptitle
for a Common Title
In addition to individual subplot titles, you might want to add a common title for the entire figure. This can be done using the suptitle
method of the figure object.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add subplot titles
axs[0, 0].set_title('Top Left - how2matplotlib.com')
axs[0, 1].set_title('Top Right - how2matplotlib.com')
axs[1, 0].set_title('Bottom Left - how2matplotlib.com')
axs[1, 1].set_title('Bottom Right - how2matplotlib.com')
# Add a common title for the entire figure
fig.suptitle('Common Title - how2matplotlib.com', fontsize=16, fontweight='bold')
# Display the figure
plt.show()
Output:
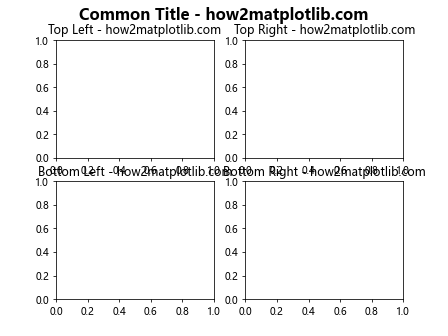
5. Titles with Special Characters
Sometimes, you might need to include special characters or mathematical expressions in your titles. Matplotlib supports LaTeX-style formatting for incorporating these elements.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add titles with special characters
axs[0, 0].set_title(r'$\alpha$ - Alpha - how2matplotlib.com')
axs[0, 1].set_title(r'$\beta$ - Beta - how2matplotlib.com')
axs[1, 0].set_title(r'$\gamma$ - Gamma - how2matplotlib.com')
axs[1, 1].set_title(r'$\delta$ - Delta - how2matplotlib.com')
# Display the figure
plt.show()
Output:
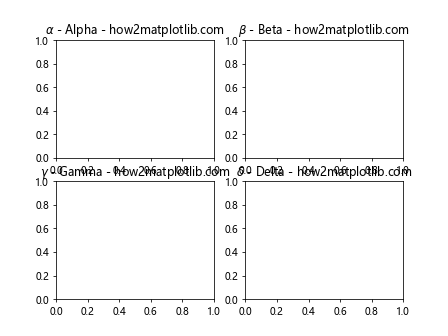
6. Rotated Titles
In certain cases, particularly when dealing with narrow subplots, you might want to rotate the titles to fit better within the available space.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add rotated titles
axs[0, 0].set_title('Top Left - how2matplotlib.com', rotation=45)
axs[0, 1].set_title('Top Right - how2matplotlib.com', rotation=45)
axs[1, 0].set_title('Bottom Left - how2matplotlib.com', rotation=45)
axs[1, 1].set_title('Bottom Right - how2matplotlib.com', rotation=45)
# Display the figure
plt.show()
Output:
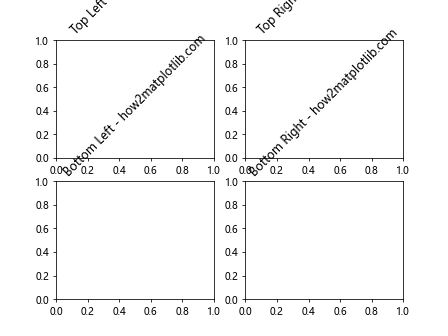
7. Titles with Background Color
Adding a background color to titles can enhance readability, especially when the plot background is cluttered or has similar colors to the text.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add titles with background color
axs[0, 0].set_title('Top Left - how2matplotlib.com', backgroundcolor='yellow')
axs[0, 1].set_title('Top Right - how2matplotlib.com', backgroundcolor='lightblue')
axs[1, 0].set_title('Bottom Left - how2matplotlib.com', backgroundcolor='lightgreen')
axs[1, 1].set_title('Bottom Right - how2matplotlib.com', backgroundcolor='lightcoral')
# Display the figure
plt.show()
Output:
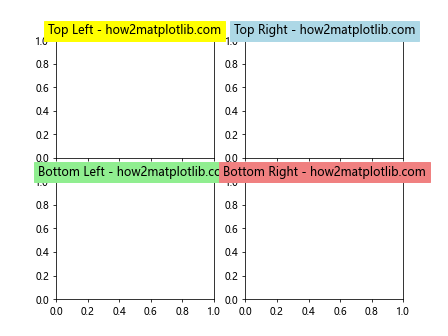
8. Transparent Titles
For a more subtle effect, you might want to make the title background slightly transparent. This can be achieved by adjusting the alpha
parameter.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add titles with transparency
axs[0, 0].set_title('Top Left - how2matplotlib.com', backgroundcolor='yellow', alpha=0.5)
axs[0, 1].set_title('Top Right - how2matplotlib.com', backgroundcolor='lightblue', alpha=0.5)
axs[1, 0].set_title('Bottom Left - how2matplotlib.com', backgroundcolor='lightgreen', alpha=0.5)
axs[1, 1].set_title('Bottom Right - how2matplotlib.com', backgroundcolor='lightcoral', alpha=0.5)
# Display the figure
plt.show()
Output:
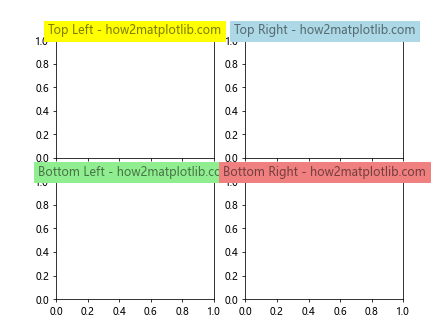
9. Titles with Multiple Lines
If you need to include more information in a title, you can use multiple lines by including newline characters (\n
) in the title string.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Add titles with multiple lines
axs[0, 0].set_title('Top Left\nhow2matplotlib.com')
axs[0, 1].set_title('Top Right\nhow2matplotlib.com')
axs[1, 0].set_title('Bottom Left\nhow2matplotlib.com')
axs[1, 1].set_title('Bottom Right\nhow2matplotlib.com')
# Display the figure
plt.show()
Output:
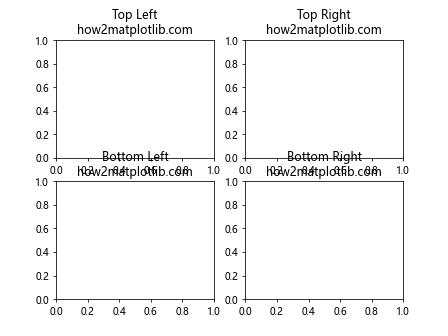
10. Titles with Font Properties
You can further customize titles by specifying font properties directly through the fontdict
parameter. This allows for detailed customization of the font type, size, weight, and more.
import matplotlib.pyplot as plt
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Define font properties
font = {'family': 'serif',
'color': 'darkred',
'weight': 'normal',
'size': 16,
}
# Add titles with font properties
axs[0, 0].set_title('Top Left - how2matplotlib.com', fontdict=font)
axs[0, 1].set_title('Top Right - how2matplotlib.com', fontdict=font)
axs[1, 0].set_title('Bottom Left - how2matplotlib.com', fontdict=font)
axs[1, 1].set_title('Bottom Right - how2matplotlib.com', fontdict=font)
# Display the figure
plt.show()
Output:
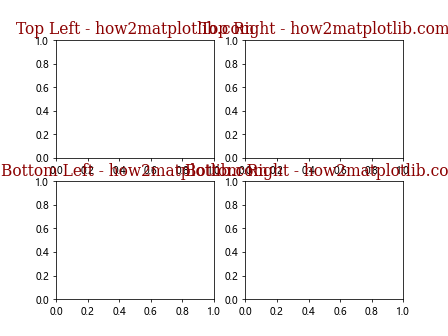
11. Titles with Outline or Shadow
Adding an outline or shadow to the text can make the titles stand out more, especially on complex backgrounds.
import matplotlib.pyplot as plt
from matplotlib.patheffects import withStroke
# Create a figure and a set of subplots
fig, axs = plt.subplots(2, 2)
# Define path effects
effects = [withStroke(linewidth=3, foreground='black')]
# Add titles with outline effect
axs[0, 0].set_title('Top Left - how2matplotlib.com', path_effects=effects)
axs[0, 1].set_title('Top Right - how2matplotlib.com', path_effects=effects)
axs[1, 0].set_title('Bottom Left - how2matplotlib.com', path_effects=effects)
axs[1, 1].set_title('Bottom Right - how2matplotlib.com', path_effects=effects)
# Display the figure
plt.show()
Output:
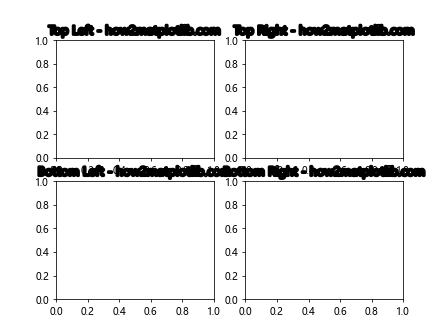
12. Dynamic Title Updates
In interactive applications, you might need to update titles dynamically based on user input or other events. Here’s how you can update a title after the plot has been displayed.
import matplotlib.pyplot as plt
# Create a figure and a subplot
fig, ax = plt.subplots()
# Initial title
ax.set_title('Initial Title - how2matplotlib.com')
# Display the plot
plt.show()
# Update the title
ax.set_title('Updated Title - how2matplotlib.com')
# Redraw the figure
fig.canvas.draw()
Output:
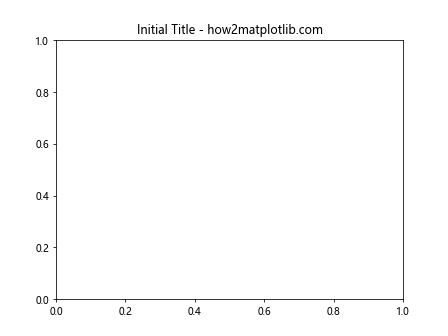
Conclusion
Adding titles to subplots in Matplotlib is a straightforward process that can significantly enhance the readability and professionalism of your charts. Whether you need simple, styled, or dynamically updated titles, Matplotlib provides a range of options to customize your subplot titles to fit your needs. By following the examples provided in this guide, you can effectively communicate the purpose and context of each subplot in your visualizations.