How to Create a Contour Plot using Matplotlib in Python
Contour Plot using Matplotlib – Python is a powerful visualization technique that allows you to represent three-dimensional data on a two-dimensional plane. In this comprehensive guide, we’ll explore the ins and outs of creating contour plots using Matplotlib in Python. We’ll cover everything from basic concepts to advanced techniques, providing you with the knowledge and skills to create stunning contour plots for your data visualization needs.
Understanding Contour Plots and Matplotlib
Before we dive into the specifics of creating contour plots using Matplotlib in Python, let’s first understand what contour plots are and why Matplotlib is an excellent choice for creating them.
What is a Contour Plot?
A contour plot, also known as a level plot or isoline plot, is a graphical technique used to display three-dimensional data on a two-dimensional surface. It uses contour lines to represent points of equal value in a 3D surface. Contour plots are widely used in various fields, including meteorology, geography, and data science, to visualize complex data relationships.
Why Use Matplotlib for Contour Plots?
Matplotlib is a comprehensive plotting library for Python that provides a wide range of plotting functions, including contour plots. It offers flexibility, customization options, and integration with other Python libraries, making it an ideal choice for creating contour plots. Matplotlib’s contour plotting capabilities allow you to create both 2D and 3D contour plots with ease.
Now that we have a basic understanding of contour plots and Matplotlib, let’s explore how to create contour plots using Matplotlib in Python.
Setting Up Your Environment
Before we start creating contour plots using Matplotlib in Python, make sure you have the necessary libraries installed. You’ll need:
- Python (3.6 or later)
- Matplotlib
- NumPy
You can install Matplotlib and NumPy using pip:
pip install matplotlib numpy
Once you have these libraries installed, you’re ready to start creating contour plots using Matplotlib in Python.
Basic Contour Plot using Matplotlib – Python
Let’s start with a basic contour plot using Matplotlib in Python. We’ll create a simple function and visualize it using a contour plot.
import numpy as np
import matplotlib.pyplot as plt
# Create data for the contour plot
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
# Create the contour plot
plt.figure(figsize=(10, 8))
contour = plt.contour(X, Y, Z)
plt.colorbar(contour)
plt.title('Basic Contour Plot using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
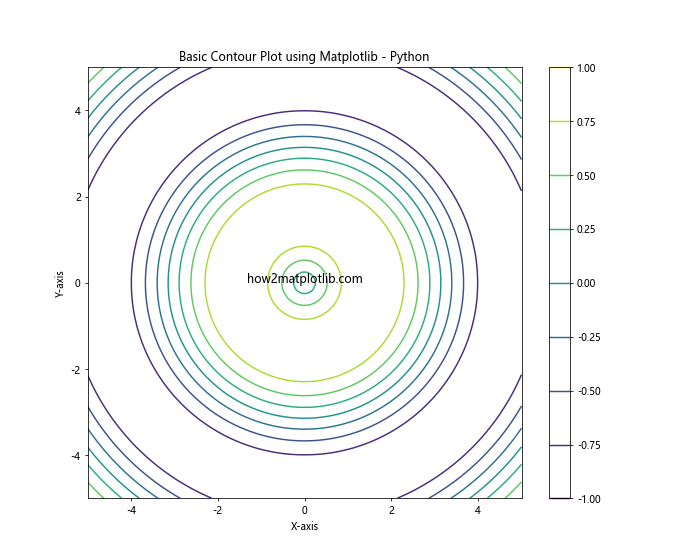
In this example, we create a basic contour plot using Matplotlib in Python. Here’s what each part of the code does:
- We import the necessary libraries: NumPy for numerical computations and Matplotlib for plotting.
- We create the data for our contour plot using NumPy’s
linspace
andmeshgrid
functions. - We define a function
Z
that we want to visualize using the contour plot. - We create a new figure with
plt.figure()
and set its size. - We use
plt.contour()
to create the contour plot. - We add a colorbar to show the range of values represented by the contours.
- We set the title, x-label, and y-label for the plot.
- Finally, we display the plot using
plt.show()
.
This basic example demonstrates how to create a simple contour plot using Matplotlib in Python. Now, let’s explore more advanced techniques and customizations.
Customizing Contour Plots with Matplotlib
Matplotlib offers various options to customize your contour plots. Let’s look at some ways to enhance your contour plots using Matplotlib in Python.
Changing Contour Colors
You can customize the colors of your contour plot using Matplotlib’s color maps.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
plt.figure(figsize=(10, 8))
contour = plt.contour(X, Y, Z, cmap='viridis')
plt.colorbar(contour)
plt.title('Customized Color Contour Plot using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
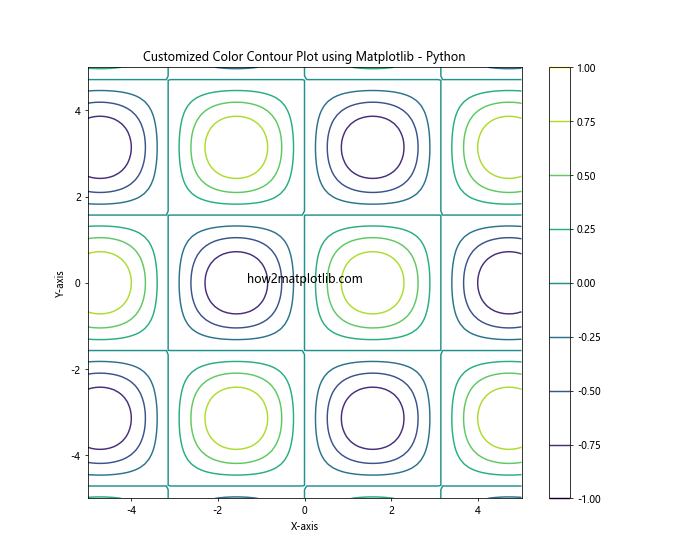
In this example, we use the ‘viridis’ colormap to change the colors of the contour plot. Matplotlib offers many other colormaps that you can experiment with to find the best visualization for your data.
Adding Contour Labels
You can add labels to your contour lines to make your plot more informative.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = X**2 + Y**2
plt.figure(figsize=(10, 8))
contour = plt.contour(X, Y, Z)
plt.clabel(contour, inline=True, fontsize=8)
plt.colorbar(contour)
plt.title('Contour Plot with Labels using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
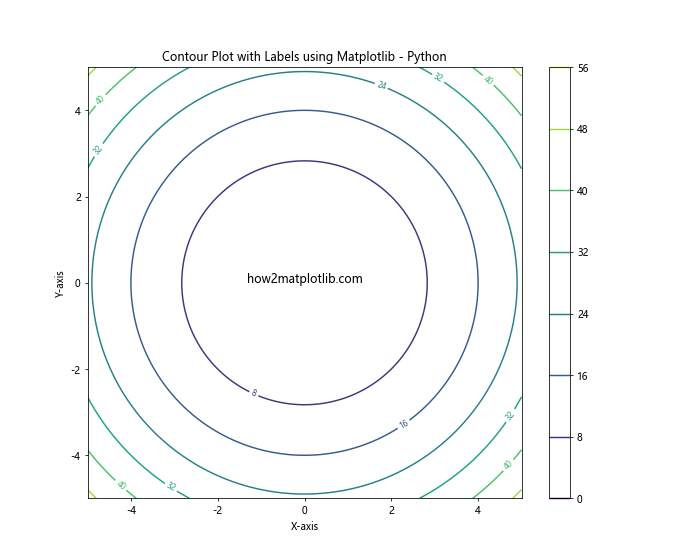
In this example, we use plt.clabel()
to add labels to the contour lines. The inline=True
parameter ensures that the labels are placed along the contour lines.
Customizing Contour Levels
You can specify custom levels for your contour plot to highlight specific ranges of values.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
plt.figure(figsize=(10, 8))
levels = [-0.75, -0.5, -0.25, 0, 0.25, 0.5, 0.75]
contour = plt.contour(X, Y, Z, levels=levels)
plt.clabel(contour, inline=True, fontsize=8)
plt.colorbar(contour)
plt.title('Contour Plot with Custom Levels using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
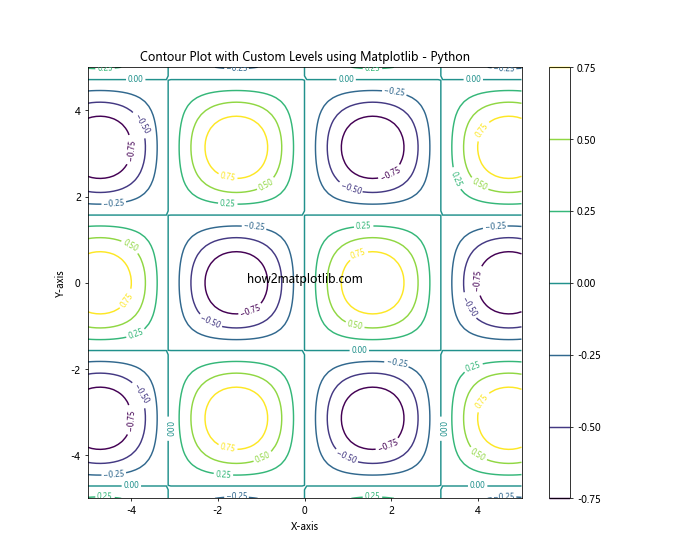
In this example, we specify custom levels for the contour plot using the levels
parameter in plt.contour()
. This allows you to focus on specific ranges of values in your data.
Creating Filled Contour Plots
Filled contour plots, also known as contourf plots, fill the areas between contour lines with colors. This can provide a more visually appealing representation of your data.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
plt.figure(figsize=(10, 8))
contourf = plt.contourf(X, Y, Z, cmap='coolwarm')
plt.colorbar(contourf)
plt.title('Filled Contour Plot using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
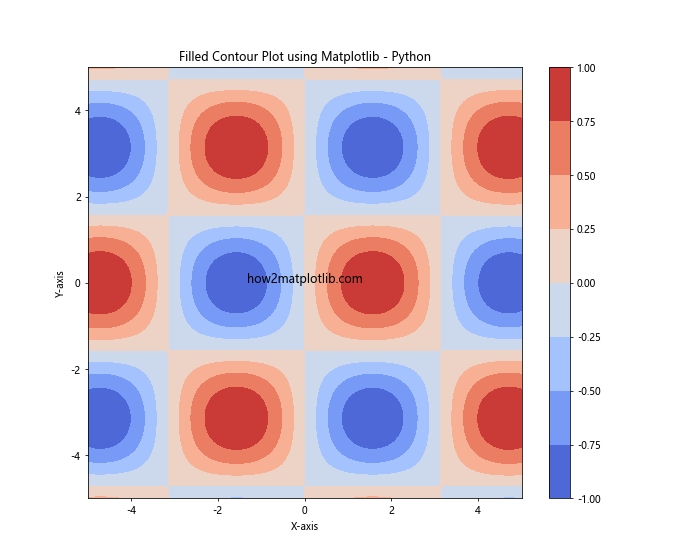
In this example, we use plt.contourf()
instead of plt.contour()
to create a filled contour plot. The ‘coolwarm’ colormap is used to provide a visually appealing color scheme.
Combining Contour and Filled Contour Plots
You can combine regular contour lines with filled contours to create a more informative visualization.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = X**2 - Y**2
plt.figure(figsize=(10, 8))
contourf = plt.contourf(X, Y, Z, cmap='RdYlBu')
contour = plt.contour(X, Y, Z, colors='k', linewidths=0.5)
plt.clabel(contour, inline=True, fontsize=8)
plt.colorbar(contourf)
plt.title('Combined Contour and Filled Contour Plot using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
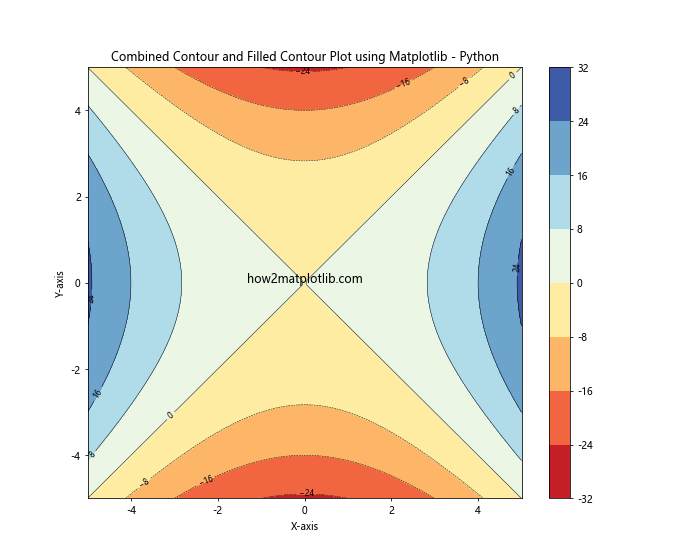
In this example, we first create a filled contour plot using plt.contourf()
, then overlay regular contour lines using plt.contour()
. The contour lines are set to black (‘k’) with a reduced line width for better visibility.
Creating 3D Contour Plots
While 2D contour plots are useful, sometimes you may want to visualize your data in 3D. Matplotlib allows you to create 3D contour plots as well.
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
fig = plt.figure(figsize=(12, 8))
ax = fig.add_subplot(111, projection='3d')
contour = ax.contour3D(X, Y, Z, 50, cmap='viridis')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_zlabel('Z-axis')
plt.colorbar(contour)
plt.title('3D Contour Plot using Matplotlib - Python')
ax.text(0, 0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
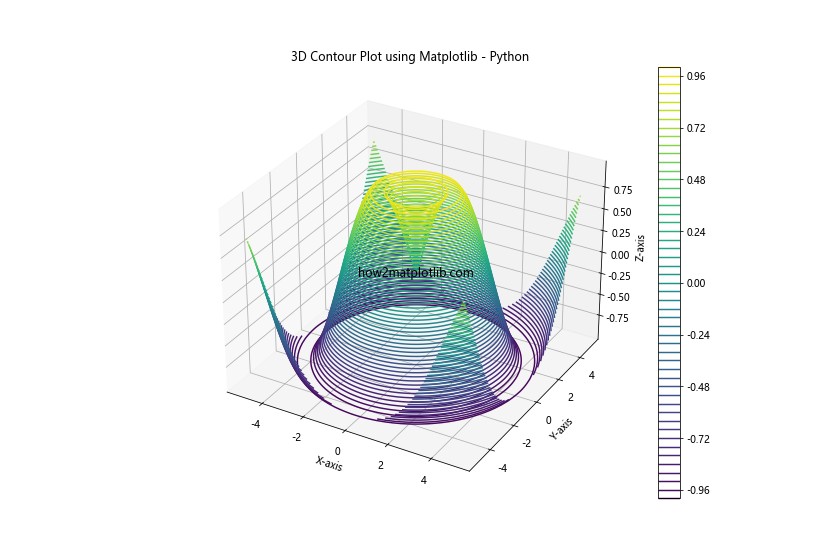
In this example, we create a 3D contour plot using ax.contour3D()
. We use the mpl_toolkits.mplot3d
module to enable 3D plotting capabilities.
Advanced Techniques for Contour Plots using Matplotlib – Python
Now that we’ve covered the basics, let’s explore some advanced techniques for creating contour plots using Matplotlib in Python.
Log-scaled Contour Plots
When dealing with data that spans multiple orders of magnitude, log-scaled contour plots can be useful.
import numpy as np
import matplotlib.pyplot as plt
x = np.logspace(0, 2, 100)
y = np.logspace(0, 2, 100)
X, Y = np.meshgrid(x, y)
Z = X * Y
plt.figure(figsize=(10, 8))
contour = plt.contour(X, Y, Z, locator=plt.LogLocator())
plt.clabel(contour, inline=True, fontsize=8)
plt.xscale('log')
plt.yscale('log')
plt.colorbar(contour)
plt.title('Log-scaled Contour Plot using Matplotlib - Python')
plt.xlabel('X-axis (log scale)')
plt.ylabel('Y-axis (log scale)')
plt.text(10, 10, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
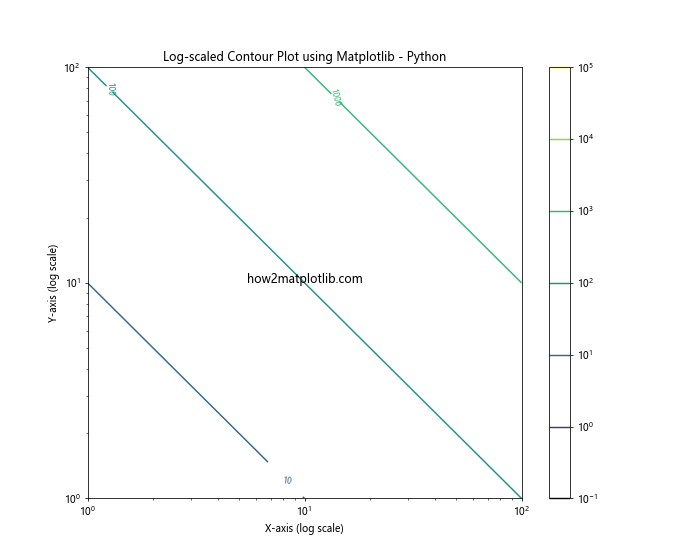
In this example, we use np.logspace()
to create logarithmically spaced data. We set the x and y scales to logarithmic using plt.xscale('log')
and plt.yscale('log')
. The locator=plt.LogLocator()
parameter in plt.contour()
ensures that the contour levels are also logarithmically spaced.
Polar Contour Plots
Matplotlib allows you to create contour plots in polar coordinates, which can be useful for certain types of data.
import numpy as np
import matplotlib.pyplot as plt
r = np.linspace(0, 2, 100)
theta = np.linspace(0, 2*np.pi, 100)
R, Theta = np.meshgrid(r, theta)
Z = R**2 * (1 - R/2) * np.cos(Theta)
plt.figure(figsize=(10, 8))
ax = plt.subplot(111, projection='polar')
contour = ax.contourf(Theta, R, Z, cmap='coolwarm')
plt.colorbar(contour)
plt.title('Polar Contour Plot using Matplotlib - Python')
ax.set_rmax(2)
ax.set_rticks([0.5, 1, 1.5, 2])
ax.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
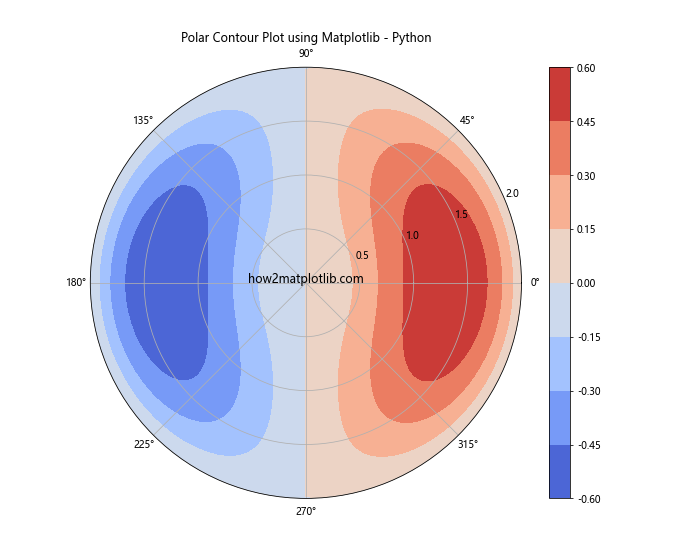
In this example, we create a polar contour plot by setting the subplot projection to ‘polar’. We use ax.contourf()
to create a filled contour plot in polar coordinates.
Contour Plots with Irregular Data
Sometimes, your data might not be on a regular grid. Matplotlib can handle irregular data using tricontour()
and tricontourf()
.
import numpy as np
import matplotlib.pyplot as plt
np.random.seed(19680801)
x = np.random.rand(1000) * 4 - 2
y = np.random.rand(1000) * 4 - 2
z = x * np.exp(-x**2 - y**2)
plt.figure(figsize=(10, 8))
contour = plt.tricontourf(x, y, z, cmap='viridis')
plt.colorbar(contour)
plt.title('Contour Plot with Irregular Data using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
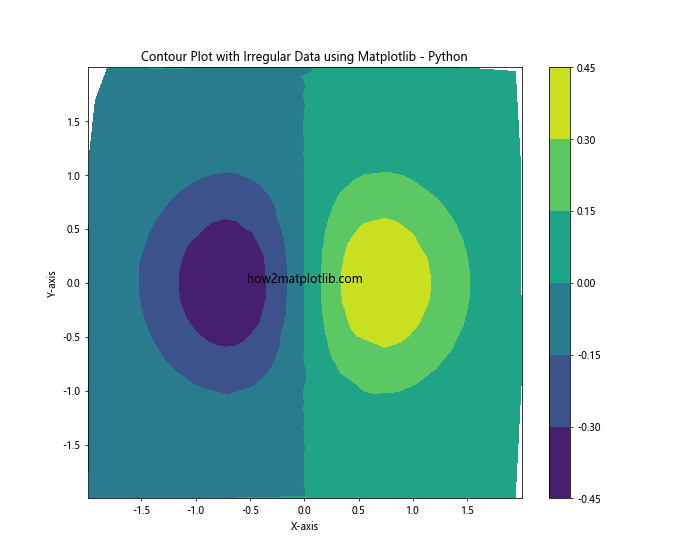
In this example, we generate random x and y coordinates and calculate z values based on these coordinates. We then use plt.tricontourf()
to create a filled contour plot from this irregular data.
Contour Plots with Masked Arrays
Matplotlib allows you to mask certain parts of your data, which can be useful when dealing with incomplete or invalid data.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
mask = np.sqrt(X**2 + Y**2) > 4
Z_masked = np.ma.array(Z, mask=mask)
plt.figure(figsize=(10, 8))
contour = plt.contourf(X, Y, Z_masked, cmap='viridis')
plt.colorbar(contour)
plt.title('Contour Plot with Masked Array using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
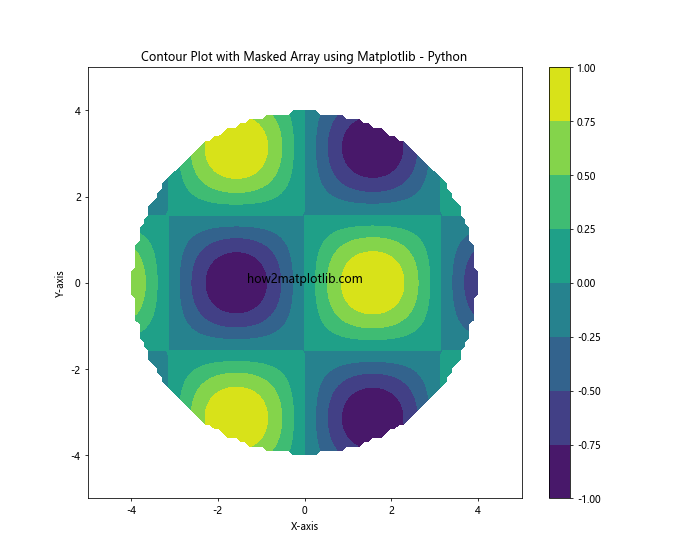
In this example, we create a mask based on the distance from the origin and apply it to our Z data. The resulting contour plot will only show data within a circular region.
Customizing Contour Plot Appearance
Matplotlib offers numerous options to customize the appearance of your contour plots. Let’s explore some of these options.
Changing Line Styles and Colors
You can customize the appearance of contour lines by changing their style and color.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
plt.figure(figsize=(10, 8))
contour = plt.contour(X, Y, Z, colors='k', linestyles=['solid', 'dashed', 'dotted'])
plt.clabel(contour, inline=True, fontsize=8)
plt.colorbar(contour)
plt.title('Contour Plot with Custom Line Styles using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
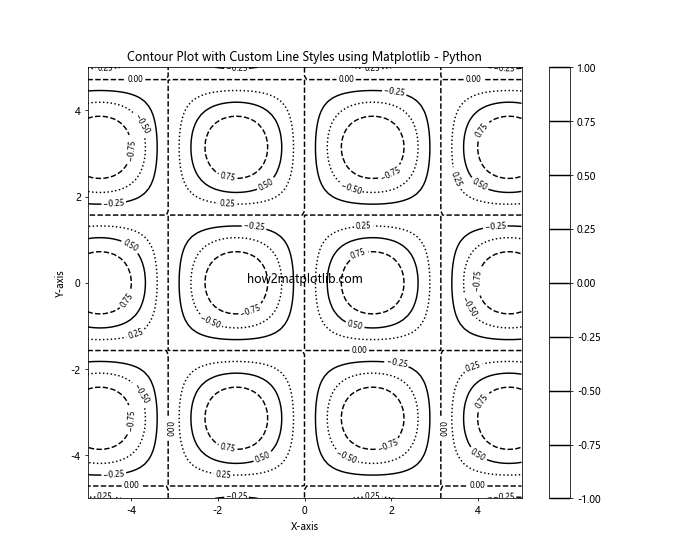
In this example, we set the contour lines to black (‘k’) and cycle through different line styles (solid, dashed, and dotted) for each contour level.
Adding a Color Bar with Custom Ticks
You can customize the color bar to better represent your data.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = X**2 + Y**2
plt.figure(figsize=(10, 8))
contour = plt.contourf(X, Y, Z, levels=np.linspace(0, 50, 11))
cbar = plt.colorbar(contour, ticks=np.linspace(0, 50, 6))
cbar.set_label('Value', rotation=270, labelpad=15)
plt.title('Contour Plot with Custom Color Bar using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
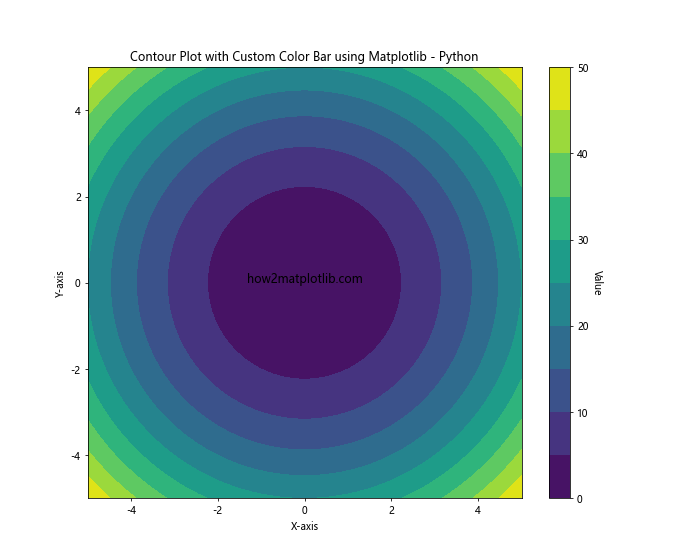
In this example, we create a custom color bar with specific ticks and add a label to it.
Contour Plots for Real-World Data
Contour plots are often used to visualize real-world data. Let’s look at an example of how to create a contour plot for temperature data.
import numpy as np
import matplotlib.pyplot as plt
# Simulating temperature data
np.random.seed(0)
x = np.linspace(0, 10, 20)
y = np.linspace(0, 10, 20)
X, Y = np.meshgrid(x, y)
Z = 20 + 5 * np.random.randn(*X.shape) + X + Y
plt.figure(figsize=(12, 8))
contour = plt.contourf(X, Y, Z, cmap='RdYlBu_r', levels=15)
plt.colorbar(contour, label='Temperature (°C)')
plt.title('Temperature Contour Map using Matplotlib - Python')
plt.xlabel('Longitude')
plt.ylabel('Latitude')
plt.text(5, 5, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
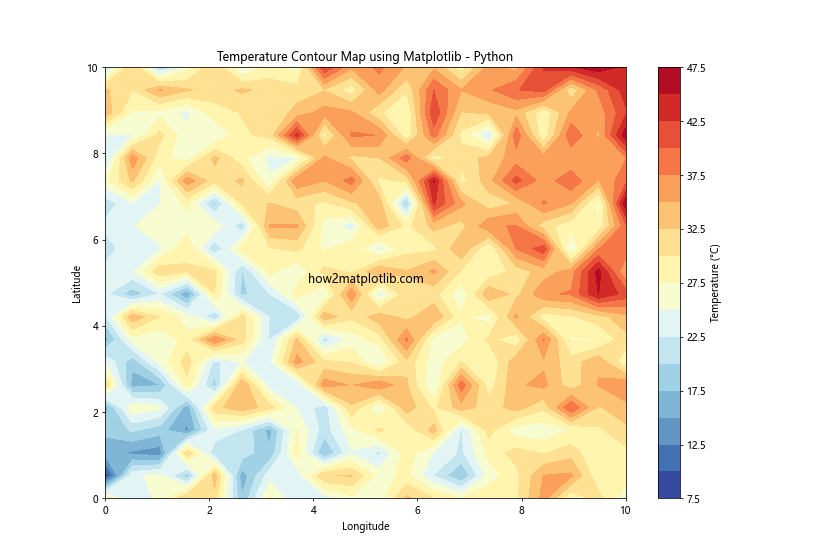
In this example, we simulate temperature data and create a contour plot to visualize it. The ‘RdYlBu_r’ colormap is used to represent temperature, with blue for cooler areas and red for warmer areas.
Combining Contour Plots with Other Plot Types
Contour plots can be combined with other types of plots to create more informative visualizations.
Contour Plot with Scatter Plot
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(X) * np.cos(Y)
# Generate random points for scatter plot
np.random.seed(0)
scatter_x = np.random.uniform(-5, 5, 50)
scatter_y = np.random.uniform(-5, 5, 50)
plt.figure(figsize=(10, 8))
contour = plt.contourf(X, Y, Z, cmap='viridis', alpha=0.7)
plt.scatter(scatter_x, scatter_y, c='red', s=20)
plt.colorbar(contour)
plt.title('Contour Plot with Scatter Plot using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
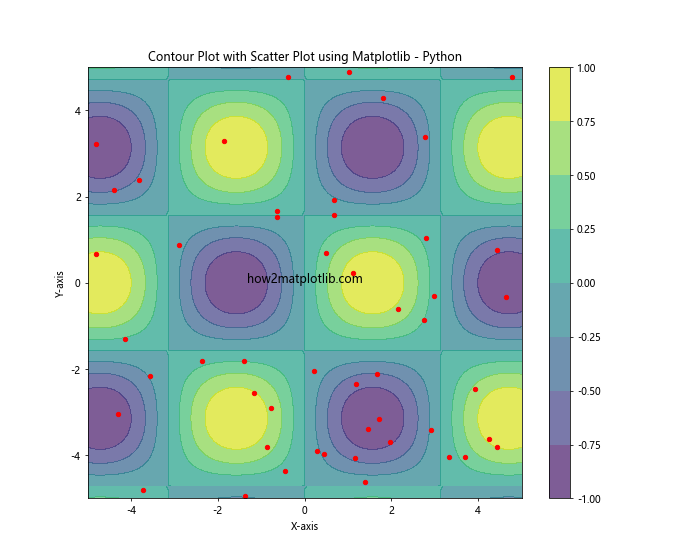
In this example, we combine a contour plot with a scatter plot to show both the overall trend and individual data points.
Contour Plot with Vector Field
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(-5, 5, 20)
y = np.linspace(-5, 5, 20)
X, Y = np.meshgrid(x, y)
Z = X*np.exp(-X**2 - Y**2)
U = -2*X*np.exp(-X**2 - Y**2)
V = -2*Y*np.exp(-X**2 - Y**2)
plt.figure(figsize=(10, 8))
contour = plt.contourf(X, Y, Z, cmap='viridis', alpha=0.7)
plt.quiver(X, Y, U, V, color='white', alpha=0.8)
plt.colorbar(contour)
plt.title('Contour Plot with Vector Field using Matplotlib - Python')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
plt.show()
Output:
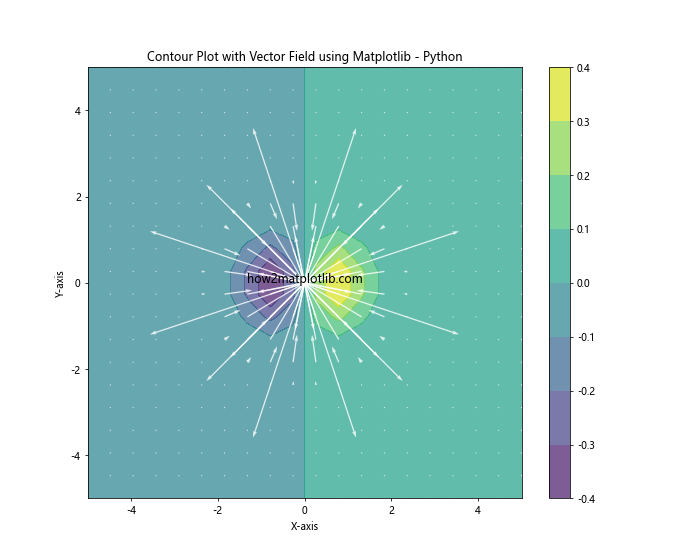
In this example, we combine a contour plot with a vector field to show both scalar and vector data in the same plot.
Animating Contour Plots
You can create animated contour plots to visualize how your data changes over time or with respect to a parameter.
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
fig, ax = plt.subplots(figsize=(10, 8))
contour = ax.contourf(X, Y, np.zeros_like(X))
plt.colorbar(contour)
ax.set_title('Animated Contour Plot using Matplotlib - Python')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
def update(frame):
Z = np.sin(X + frame/10) * np.cos(Y + frame/10)
ax.clear()
contour = ax.contourf(X, Y, Z, cmap='viridis')
ax.set_title(f'Animated Contour Plot (Frame {frame})')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.text(0, 0, 'how2matplotlib.com', fontsize=12, ha='center')
return contour
anim = FuncAnimation(fig, update, frames=100, interval=50)
plt.show()
Output:
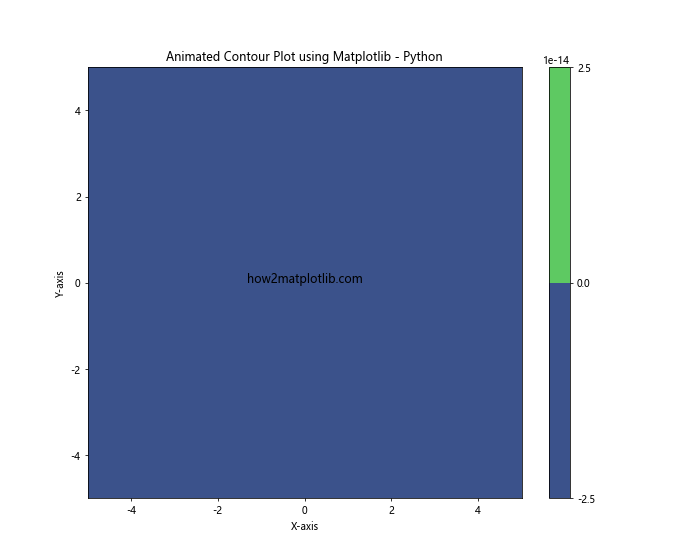
In this example, we create an animated contour plot that changes over time. The FuncAnimation
class from Matplotlib is used to create the animation.
Best Practices for Creating Contour Plots using Matplotlib – Python
When creating contour plots using Matplotlib in Python, keep these best practices in mind:
- Choose appropriate color maps: Select color maps that are suitable for your data and easy to interpret.
- Use an appropriate number of contour levels: Too few levels may not show enough detail, while too many can make the plot cluttered.
- Add labels and titles: Always include clear labels for axes, a title, and a color bar to make your plot easy to understand.
- Consider your audience: Tailor your plot’s complexity to your audience’s familiarity with the subject matter.
- Use logarithmic scales when appropriate: For data spanning multiple orders of magnitude, consider using log-scaled contour plots.
- Combine with other plot types: When relevant, combine contour plots with other visualizations to provide more context.
- Experiment with different projections: For certain types of data, polar or other projections might be more appropriate than Cartesian coordinates.
Troubleshooting Common Issues
When working with contour plots using Matplotlib in Python, you might encounter some common issues. Here are some tips to help you troubleshoot:
- Blank or incorrect plot: Ensure your data is properly formatted and doesn’t contain NaN or infinite values.
- Colorbar issues: If your colorbar doesn’t appear or looks incorrect, check that you’re passing the correct contour object to
plt.colorbar()
. - Memory errors: For large datasets, consider downsampling or using more efficient data structures.
- Contour lines not appearing: Make sure your data has sufficient variation to create contours. You might need to adjust the number of levels or use a different plotting function (e.g.,
contourf
instead ofcontour
). - Labeling issues: If contour labels are overlapping or misplaced, try adjusting the
inline
andfontsize
parameters inplt.clabel()
.
Conclusion
Contour plots are a powerful tool for visualizing three-dimensional data in two dimensions. Matplotlib provides a robust and flexible set of functions for creating contour plots in Python. From basic plots to advanced techniques like log-scaled and polar contour plots, Matplotlib offers a wide range of options to customize your visualizations.