How to Adjust Marker Size in Matplotlib
Matplotlib is a comprehensive library for creating static, animated, and interactive visualizations in Python. One of the key features of Matplotlib is its ability to control and customize almost every element of a plot, including the size of markers in scatter plots, line plots, and more. This article provides a detailed guide on how to adjust marker sizes in Matplotlib, complete with a variety of examples to illustrate different techniques.
Introduction to Marker Size
In Matplotlib, markers are visual symbols that can be used in plots to represent data points. The size of these markers can be crucial for making plots more readable and visually appealing. Adjusting marker size can help in highlighting specific points, making patterns more noticeable, or simply making the plot look better tailored to specific presentation needs.
Basic Example of Adjusting Marker Size
Let’s start with a basic example of how to adjust the size of markers in a scatter plot.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a scatter plot
plt.scatter(x, y, s=100) # s is the size of the marker
plt.title("Basic Scatter Plot with Marker Size - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Output:
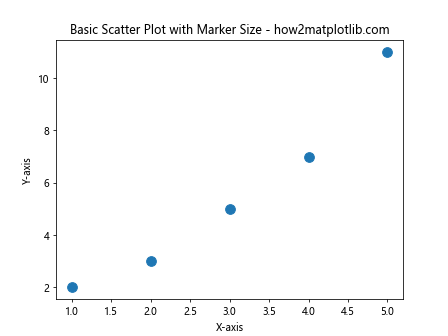
Dynamically Adjusting Marker Sizes
You can adjust the marker sizes dynamically based on data. For instance, you might want the size of each marker to correspond to another variable in your dataset.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
sizes = [30, 60, 90, 120, 150]
# Create a scatter plot
plt.scatter(x, y, s=sizes)
plt.title("Scatter Plot with Dynamic Marker Sizes - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Output:
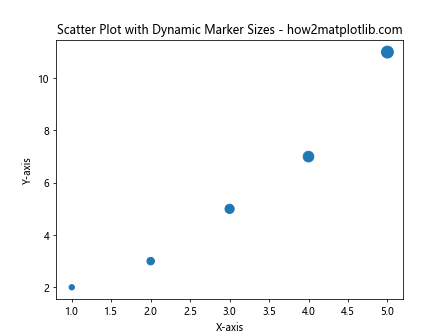
Using Marker Size to Encode Data
Marker size can be used to encode additional data. This technique can provide a third dimension of information.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
sizes = [20 * n for n in y] # Calculate sizes based on y values
# Create a scatter plot
plt.scatter(x, y, s=sizes)
plt.title("Marker Size Encoding Data - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Output:
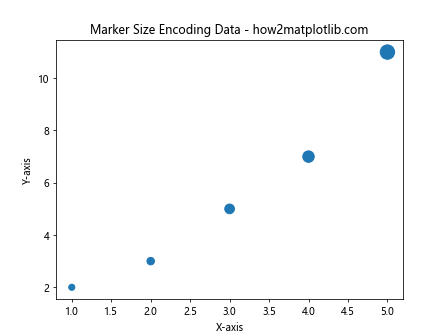
Adjusting Marker Size in Line Plots
While less common, you can also adjust marker sizes in line plots.
import matplotlib.pyplot as plt
# Sample data
x = [1, 2, 3, 4, 5]
y = [2, 3, 5, 7, 11]
# Create a line plot with markers
plt.plot(x, y, marker='o', markersize=10) # Set marker size with markersize
plt.title("Line Plot with Marker Size - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Output:
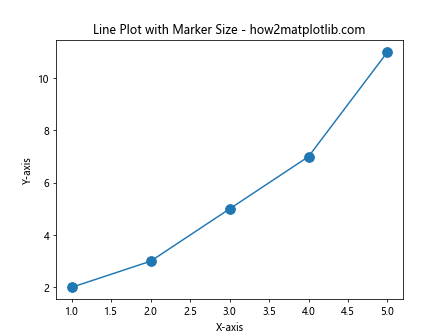
Customizing Marker Size Based on a Function
You can customize marker sizes based on a mathematical function or any custom logic.
import matplotlib.pyplot as plt
import numpy as np
# Sample data
x = np.random.rand(50)
y = np.random.rand(50)
sizes = np.exp(x * 10) # Exponential function for size
# Create a scatter plot
plt.scatter(x, y, s=sizes)
plt.title("Custom Function for Marker Sizes - how2matplotlib.com")
plt.xlabel("X-axis")
plt.ylabel("Y-axis")
plt.show()
Output:
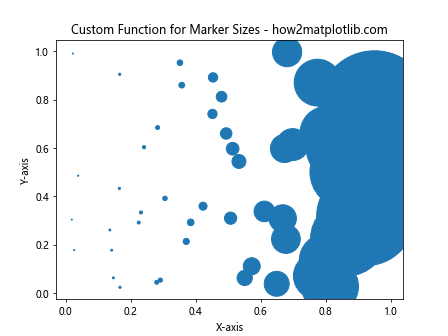
Conclusion
Adjusting marker sizes in Matplotlib is a powerful way to enhance the visual appeal and the informativeness of your plots. Whether you are working with scatter plots, line plots, or any other kind of plot, understanding how to manipulate marker sizes allows for better data visualization and storytelling. This guide has shown various methods to adjust marker sizes, from simple static values to dynamic sizes based on data or functions. By integrating these techniques into your plotting routine, you can create more effective and attractive visualizations.
Remember, the examples provided here are just the starting point. Matplotlib offers extensive customization options that can be explored further in the official Matplotlib documentation at Matplotlib’s website.