Subplot Title
In this article, we will discuss how to set titles for subplots in Matplotlib. Subplots are a powerful way to display multiple plots within the same figure. Adding titles to individual subplots can help clarify the information being presented and make the plots more informative.
Setting Subplot Titles
In Matplotlib, we can set titles for subplots using the set_title
method. This method allows us to specify the title for a specific subplot by passing the title as a parameter.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Setting title for the first subplot
ax[0].set_title('First Subplot Title')
# Setting title for the second subplot
ax[1].set_title('Second Subplot Title')
plt.show()
Output:
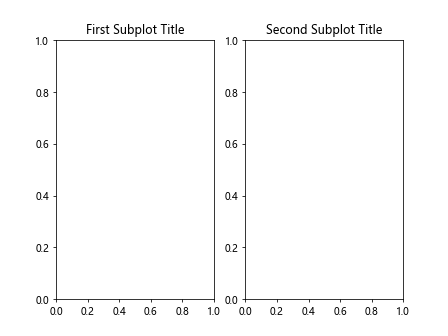
Adding Titles to Multiple Subplots
If we have multiple subplots in a single figure, we can iterate over the axes and set titles for each subplot individually.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(2, 2)
# Titles for subplots
titles = ['First Subplot Title', 'Second Subplot Title', 'Third Subplot Title', 'Fourth Subplot Title']
# Setting titles for each subplot
for i in range(4):
ax[i // 2, i % 2].set_title(titles[i])
plt.show()
Output:
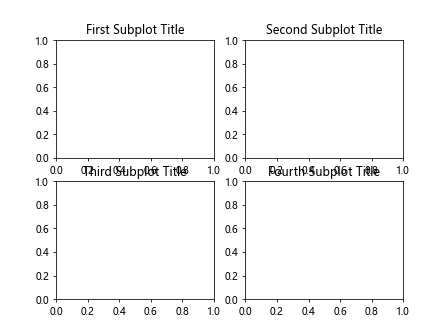
Customizing Subplot Titles
We can customize the appearance of subplot titles by changing the font size, font weight, font style, and color.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Customizing title for the first subplot
ax[0].set_title('Subplot Title', fontsize=16, fontweight='bold', fontstyle='italic', color='blue')
# Customizing title for the second subplot
ax[1].set_title('Subplot Title', fontsize=14, fontweight='normal', fontstyle='normal', color='green')
plt.show()
Output:
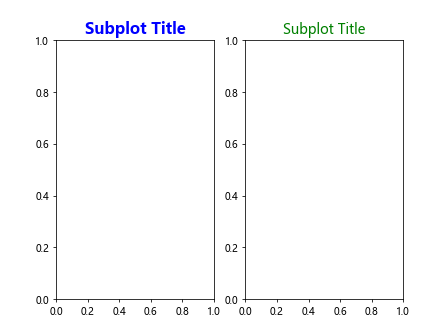
Using Main Title and Subtitles
In addition to individual subplot titles, we can also add a main title for the entire figure and subtitles for each subplot.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Main title for the figure
fig.suptitle('Main Figure Title', fontsize=18, fontweight='bold')
# Subtitles for each subplot
ax[0].set_title('First Subplot', fontsize=14)
ax[1].set_title('Second Subplot', fontsize=14)
plt.show()
Output:
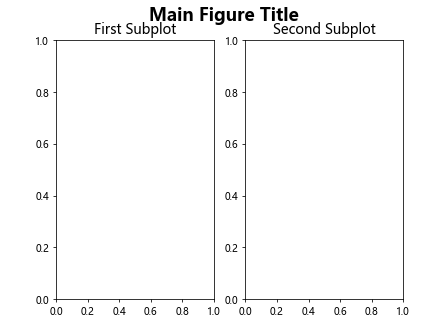
Setting Position of Subplot Titles
We can adjust the position of subplot titles by specifying the x
and y
coordinates of the title within the subplot.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Setting title position for the first subplot
ax[0].set_title('Subplot Title', x=0.5, y=0.9)
# Setting title position for the second subplot
ax[1].set_title('Subplot Title', x=0.1, y=0.1)
plt.show()
Output:
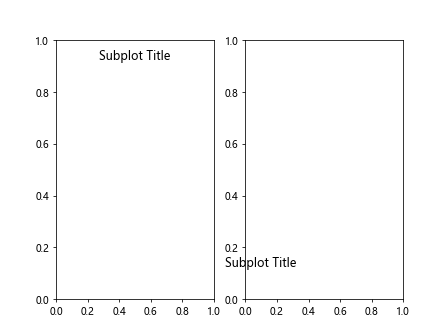
Using LaTeX in Subplot Titles
Matplotlib supports the use of LaTeX math expressions in subplot titles, allowing us to display mathematical symbols and equations.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Using LaTeX in title for the first subplot
ax[0].set_title(r'$\frac{a}{b}$', fontsize=14)
# Using LaTeX in title for the second subplot
ax[1].set_title(r'$e^{i\pi} + 1 = 0$', fontsize=14)
plt.show()
Output:
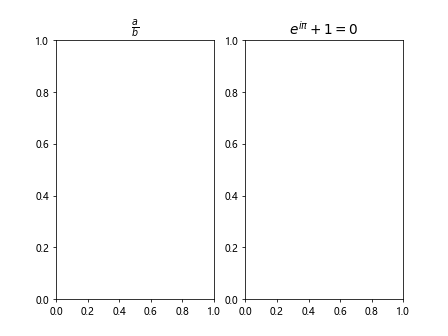
Rotating Subplot Titles
We can rotate subplot titles to be displayed vertically or horizontally by setting the rotation angle.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Rotating title for the first subplot vertically
ax[0].set_title('Vertical Title', rotation=90)
# Rotating title for the second subplot horizontally
ax[1].set_title('Horizontal Title', rotation=0)
plt.show()
Output:
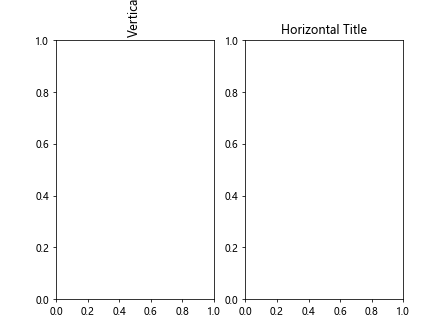
Multiline Subplot Titles
To create multiline titles for subplots, we can use a newline character \n
to separate lines of text.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Multiline title for the first subplot
ax[0].set_title('First Line\nSecond Line', fontsize=14)
# Multiline title for the second subplot
ax[1].set_title('Multiple\nLines\nTitle', fontsize=14)
plt.show()
Output:
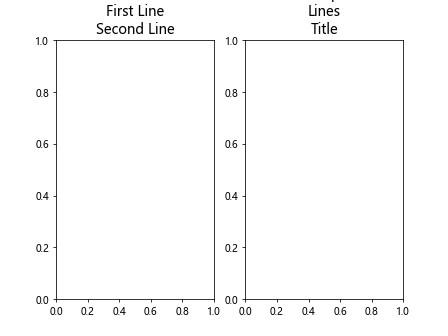
Wrapping Subplot Titles
If a title is too long to fit within the subplot, we can wrap the text by setting the wrap
parameter to True
.
import matplotlib.pyplot as plt
# Creating subplots
fig, ax = plt.subplots(1, 2)
# Long title for the first subplot
ax[0].set_title('Very Long Subplot Title That Needs to Be Wrapped', wrap=True)
# Another long title for the second subplot
ax[1].set_title('This is a Very Long Subplot Title That Also Needs Wrapping', wrap=True)
plt.show()
Output:
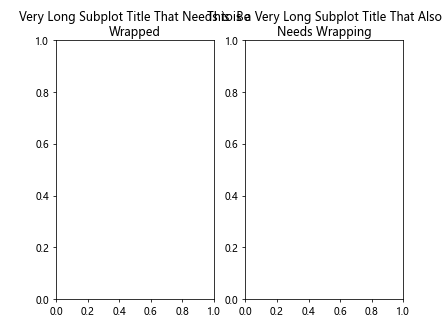
Conclusion
In this article, we have explored how to set titles for subplots in Matplotlib. By adding titles to subplots, we can enhance the visual clarity of our plots and provide additional context to the viewer. With the ability to customize the appearance, position, and content of subplot titles, we can create informative and visually appealing figures for data visualization. Next time you create subplots in Matplotlib, remember to add descriptive titles to help convey the information effectively.