How to Plot the Power Spectral Density Using Matplotlib in Python
Plot the power spectral density using Matplotlib – Python is a powerful technique for analyzing frequency content in signals. This article will provide a detailed exploration of how to plot the power spectral density (PSD) using Matplotlib in Python. We’ll cover various aspects of PSD plotting, from basic concepts to advanced techniques, and provide numerous examples to help you master this essential skill in signal processing and data visualization.
Understanding Power Spectral Density
Before we dive into plotting the power spectral density using Matplotlib in Python, it’s crucial to understand what PSD is and why it’s important. Power spectral density represents the distribution of power across different frequencies in a signal. It’s a fundamental tool in signal processing, helping analysts identify dominant frequencies, noise characteristics, and other spectral features of a signal.
To plot the power spectral density using Matplotlib in Python, we first need to compute the PSD from our signal data. There are several methods to estimate PSD, including periodogram, Welch’s method, and multitaper methods. In this article, we’ll focus on using Matplotlib to visualize these PSD estimates.
Let’s start with a basic example of how to plot the power spectral density using Matplotlib in Python:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
# Compute PSD using Welch's method
f, Pxx = signal.welch(sig, fs=1000, nperseg=256)
# Plot the power spectral density
plt.figure(figsize=(10, 6))
plt.semilogy(f, Pxx)
plt.title('Power Spectral Density - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.grid(True)
plt.show()
Output:
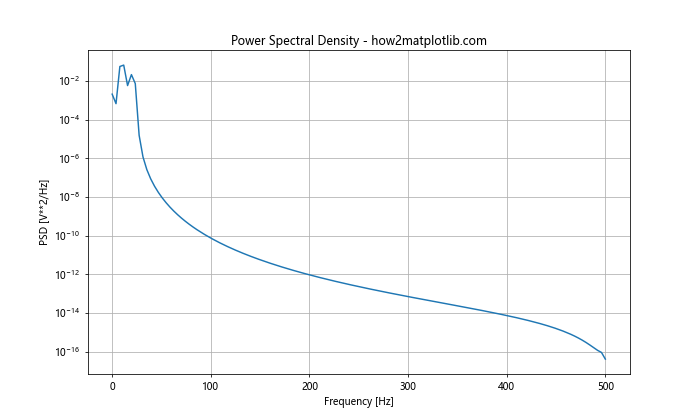
In this example, we generate a simple signal composed of two sine waves, compute its PSD using Welch’s method, and then plot the power spectral density using Matplotlib in Python. The semilogy
function is used to plot the PSD on a logarithmic scale, which is common practice for spectral analysis.
Customizing PSD Plots with Matplotlib
When you plot the power spectral density using Matplotlib in Python, there are numerous ways to customize your plots to make them more informative and visually appealing. Let’s explore some of these customization options:
Changing Line Styles and Colors
You can modify the appearance of your PSD plot by changing line styles and colors:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
# Compute PSD
f, Pxx = signal.welch(sig, fs=1000, nperseg=256)
# Plot the power spectral density with custom style
plt.figure(figsize=(10, 6))
plt.semilogy(f, Pxx, 'r--', linewidth=2)
plt.title('Customized PSD Plot - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.grid(True, linestyle=':', alpha=0.7)
plt.show()
Output:
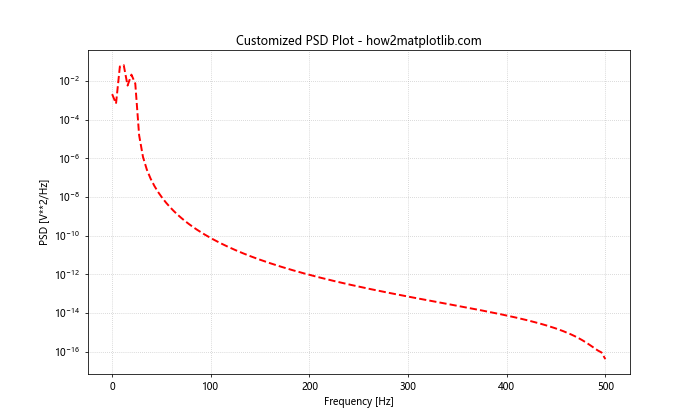
In this example, we use a red dashed line ('r--'
) with increased line width to plot the power spectral density using Matplotlib in Python. We also customize the grid style for better visibility.
Adding Multiple PSD Plots
Often, you may want to compare the power spectral density of multiple signals. Here’s how you can plot multiple PSDs on the same graph:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate two sample signals
t = np.linspace(0, 1, 1000, endpoint=False)
sig1 = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
sig2 = np.sin(2 * np.pi * 15 * t) + 0.3 * np.sin(2 * np.pi * 25 * t)
# Compute PSDs
f1, Pxx1 = signal.welch(sig1, fs=1000, nperseg=256)
f2, Pxx2 = signal.welch(sig2, fs=1000, nperseg=256)
# Plot multiple power spectral densities
plt.figure(figsize=(10, 6))
plt.semilogy(f1, Pxx1, 'b-', label='Signal 1')
plt.semilogy(f2, Pxx2, 'r--', label='Signal 2')
plt.title('Comparison of PSDs - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.legend()
plt.grid(True)
plt.show()
Output:
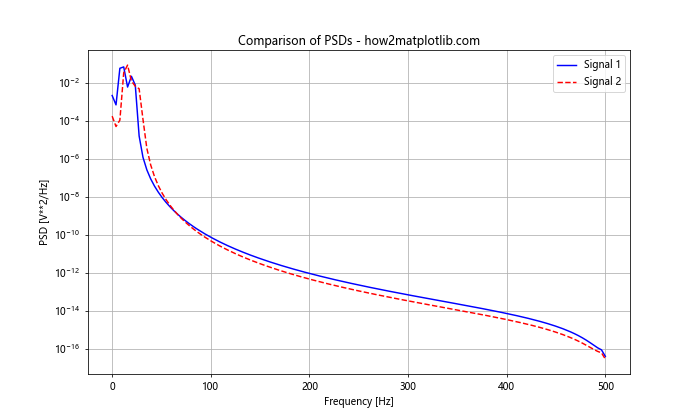
This example demonstrates how to plot the power spectral density using Matplotlib in Python for two different signals, allowing for easy comparison of their frequency content.
Advanced PSD Plotting Techniques
As you become more comfortable with plotting the power spectral density using Matplotlib in Python, you can explore more advanced techniques to enhance your visualizations and analysis.
Using Different PSD Estimation Methods
Matplotlib can be used to visualize PSDs computed using different estimation methods. Let’s compare periodogram and Welch’s method:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal
np.random.seed(0)
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.random.randn(len(t))
# Compute PSDs using different methods
f_welch, Pxx_welch = signal.welch(sig, fs=1000, nperseg=256)
f_periodogram, Pxx_periodogram = signal.periodogram(sig, fs=1000)
# Plot the power spectral densities
plt.figure(figsize=(10, 6))
plt.semilogy(f_welch, Pxx_welch, 'b-', label='Welch')
plt.semilogy(f_periodogram, Pxx_periodogram, 'r--', label='Periodogram')
plt.title('Comparison of PSD Estimation Methods - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.legend()
plt.grid(True)
plt.show()
Output:
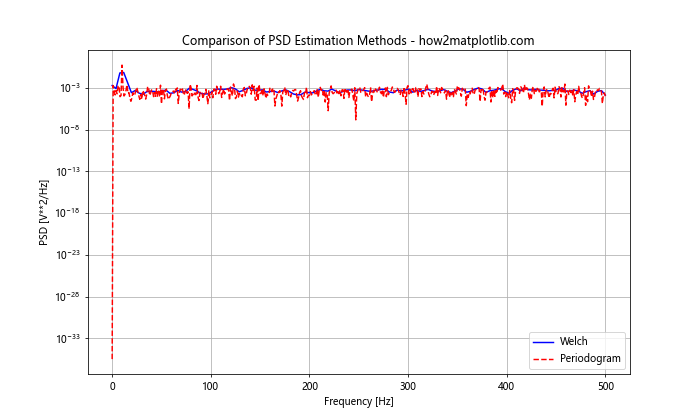
This example shows how to plot the power spectral density using Matplotlib in Python for both Welch’s method and the periodogram method, allowing for a comparison of their results.
Specialized PSD Plots
As you delve deeper into plotting the power spectral density using Matplotlib in Python, you may encounter the need for more specialized types of PSD plots. Let’s explore some of these advanced techniques.
Spectrogram: Time-Varying PSD
A spectrogram is a visual representation of the spectrum of frequencies in a signal as they vary with time. It’s essentially a series of PSD plots stacked together to show how the frequency content of a signal changes over time. Here’s how you can create a spectrogram using Matplotlib:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a chirp signal
t = np.linspace(0, 10, 1000)
w = signal.chirp(t, f0=1, f1=50, t1=10, method='linear')
# Compute spectrogram
f, t, Sxx = signal.spectrogram(w, fs=100)
# Plot the spectrogram
plt.figure(figsize=(10, 6))
plt.pcolormesh(t, f, 10 * np.log10(Sxx), shading='gouraud')
plt.title('Spectrogram - how2matplotlib.com')
plt.ylabel('Frequency [Hz]')
plt.xlabel('Time [sec]')
plt.colorbar(label='PSD [dB]')
plt.show()
Output:
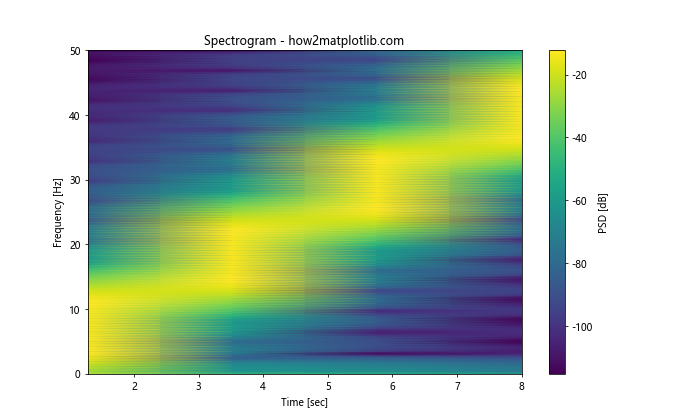
This example shows how to plot the power spectral density using Matplotlib in Python as a spectrogram, which is particularly useful for analyzing signals with time-varying frequency content.
Coherence: PSD-Based Correlation
Coherence is a statistic that can be used to examine the relation between two signals in the frequency domain. It’s based on the PSD of the signals and their cross-spectral density. Here’s how you can plot coherence using Matplotlib:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate two related signals
t = np.linspace(0, 1, 1000, endpoint=False)
sig1 = np.sin(2 * np.pi * 10 * t) + 0.5 * np.random.randn(len(t))
sig2 = np.sin(2 * np.pi * 10 * t + np.pi/4) + 0.5 * np.random.randn(len(t))
# Compute coherence
f, Cxy = signal.coherence(sig1, sig2, fs=1000, nperseg=256)
# Plot the coherence
plt.figure(figsize=(10, 6))
plt.semilogy(f, Cxy)
plt.title('Coherence Plot - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('Coherence')
plt.grid(True)
plt.show()
Output:
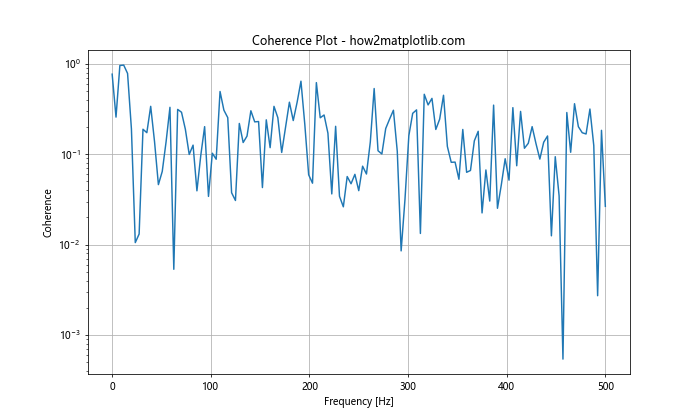
This example demonstrates how to plot the power spectral density-based coherence using Matplotlib in Python, which can reveal frequency-dependent relationships between two signals.
Customizing PSD Plot Aesthetics
When you plot the power spectral density using Matplotlib in Python, paying attention to the aesthetics of your plot can greatly enhance its readability and impact. Let’s explore some ways to improve the visual appeal of your PSD plots.
Using a Custom Color Palette
You can use custom color palettes to make your PSD plots more visually appealing or to conform to specific style guidelines:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
from matplotlib.colors import LinearSegmentedColormap
# Generate a sample signal
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
# Compute spectrogram
f, t, Sxx = signal.spectrogram(sig, fs=1000)
# Create a custom colormap
colors = ['#000033', '#0000CC', '#0099FF', '#33CCFF', '#FFFF00', '#FF9900', '#FF0000']
n_bins = 100
cmap = LinearSegmentedColormap.from_list('custom', colors, N=n_bins)
# Plot the spectrogram with custom colormap
plt.figure(figsize=(10, 6))
plt.pcolormesh(t, f, 10 * np.log10(Sxx), cmap=cmap, shading='gouraud')
plt.title('Spectrogram with Custom Colormap - how2matplotlib.com')
plt.ylabel('Frequency [Hz]')
plt.xlabel('Time [sec]')
plt.colorbar(label='PSD [dB]')
plt.show()
Output:
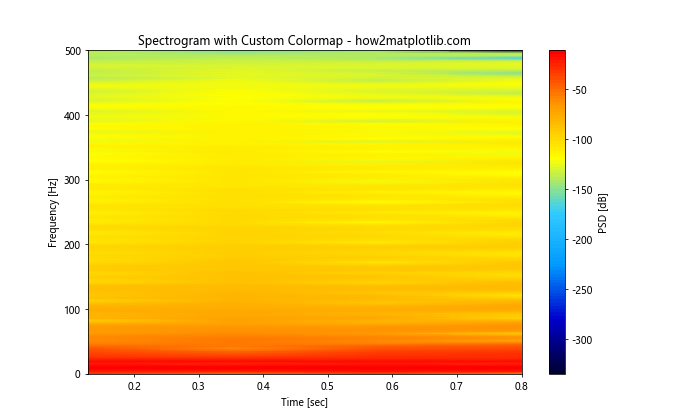
This example shows how to plot the power spectral density using Matplotlib in Python with a custom color palette, which can make your spectrogram more visually striking or easier to interpret.
Adding Annotations to PSD Plots
Annotations can be useful for highlighting specific features in your PSD plot:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
# Compute PSD
f, Pxx = signal.welch(sig, fs=1000, nperseg=256)
# Plot the power spectral density
plt.figure(figsize=(10, 6))
plt.semilogy(f, Pxx)
plt.title('Annotated PSD Plot - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.grid(True)
# Add annotations
plt.annotate('10 Hz Peak', xy=(10, Pxx[np.argmax(f >= 10)]),
xytext=(15, 1e-4), arrowprops=dict(facecolor='black', shrink=0.05))
plt.annotate('20 Hz Peak', xy=(20, Pxx[np.argmax(f >= 20)]),
xytext=(25, 1e-5), arrowprops=dict(facecolor='black', shrink=0.05))
plt.show()
Output:
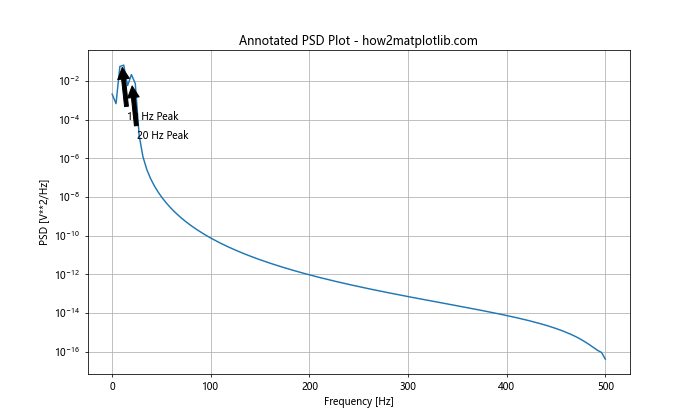
This example demonstrates how to plot the power spectral density using Matplotlib in Python with added annotations to highlight specific frequency peaks.
Handling Real-World Data
When you plot the power spectral density using Matplotlib in Python for real-world data, you may need to handle various challenges such as noise, trends, and multiple channels.
Detrending Before PSD Computation
Often, real-world signals have trends that can affect PSD estimation. Detrending can help mitigate this issue:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal with a trend
t = np.linspace(0, 10, 1000)
trend = 0.1 * t
sig = np.sin(2 * np.pi * 1 * t) + 0.5 * np.sin(2 * np.pi * 2 * t) + trend
# Compute PSD with and without detrending
f_trend, Pxx_trend = signal.welch(sig, fs=100, nperseg=256)
f_detrend, Pxx_detrend = signal.welch(signal.detrend(sig), fs=100, nperseg=256)
# Plot the power spectral densities
plt.figure(figsize=(10, 6))
plt.semilogy(f_trend, Pxx_trend, 'r--', label='With Trend')
plt.semilogy(f_detrend, Pxx_detrend, 'b-', label='Detrended')
plt.title('PSD: Effect of Detrending - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.legend()
plt.grid(True)
plt.show()
Output:
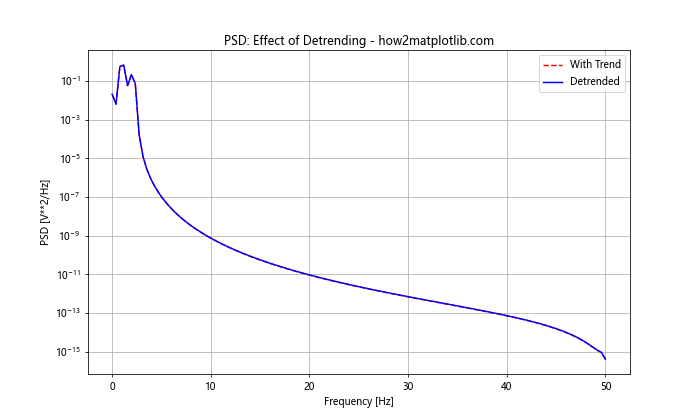
This example illustrates how to plot the power spectral density using Matplotlib in Python for both the original signal with a trend and the detrended signal, showing the importance of detrending in PSD analysis.
Handling Multi-Channel Data
When dealing with multi-channel data, you may want to plot the PSD for each channel:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate multi-channel data
t = np.linspace(0, 1, 1000, endpoint=False)
ch1 = np.sin(2 * np.pi * 10 * t) + 0.5 * np.random.randn(len(t))
ch2 = np.sin(2 * np.pi * 20 * t) + 0.5 * np.random.randn(len(t))
ch3 = np.sin(2 * np.pi * 30 * t) + 0.5 * np.random.randn(len(t))
# Compute PSDs
f, Pxx_list = signal.welch([ch1, ch2, ch3], fs=1000, nperseg=256)
# Plot the power spectral densities
plt.figure(figsize=(10, 6))
for i, Pxx in enumerate(Pxx_list):
plt.semilogy(f, Pxx, label=f'Channel {i+1}')
plt.title('Multi-Channel PSD Plot - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.legend()
plt.grid(True)
plt.show()
Output:
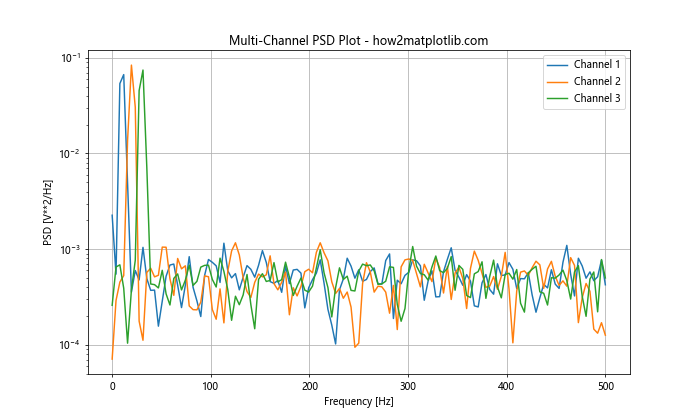
This example demonstrates how to plot the power spectral density using Matplotlib in Python for multiple channels of data, allowing for easy comparison between channels.
Advanced PSD Analysis Techniques
As you become more proficient in plotting the power spectral density using Matplotlib in Python, you may want to explore more advanced analysis techniques.
Windowing in PSD Estimation
The choice of window function can significantly affect your PSD estimate. Let’s compare different window functions:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
# Compute PSDs with different windows
f, Pxx_hann = signal.welch(sig, fs=1000, window='hann', nperseg=256)
f, Pxx_hamming = signal.welch(sig, fs=1000, window='hamming', nperseg=256)
f, Pxx_blackman = signal.welch(sig, fs=1000, window='blackman', nperseg=256)
# Plot the power spectral densities
plt.figure(figsize=(10, 6))
plt.semilogy(f, Pxx_hann, 'b-', label='Hann')
plt.semilogy(f, Pxx_hamming, 'r--', label='Hamming')
plt.semilogy(f, Pxx_blackman, 'g-.', label='Blackman')
plt.title('PSD: Effect of Window Functions - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.legend()
plt.grid(True)
plt.show()
Output:
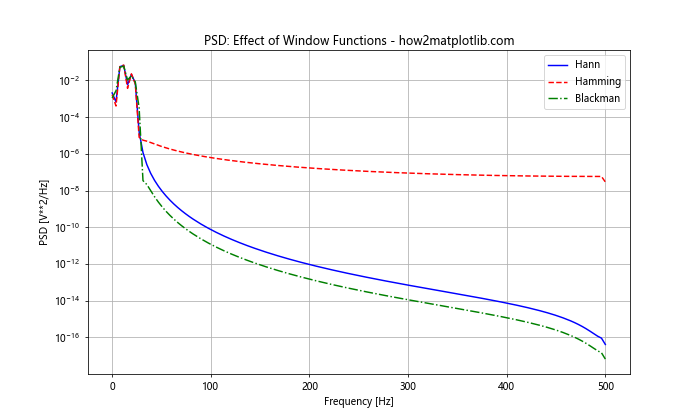
This example shows how to plot the power spectral density using Matplotlib in Python with different window functions, illustrating their effect on the PSD estimate.
Logarithmic Frequency Axis
For signals with a wide frequency range, using a logarithmic frequency axis can be beneficial:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal with wide frequency range
t = np.linspace(0, 1, 10000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 1000 * t)
# Compute PSD
f, Pxx = signal.welch(sig, fs=10000, nperseg=1024)
# Plot the power spectral density with logarithmic frequency axis
plt.figure(figsize=(10, 6))
plt.semilogx(f, 10 * np.log10(Pxx))
plt.title('PSD with Logarithmic Frequency Axis - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [dB/Hz]')
plt.grid(True)
plt.show()
Output:
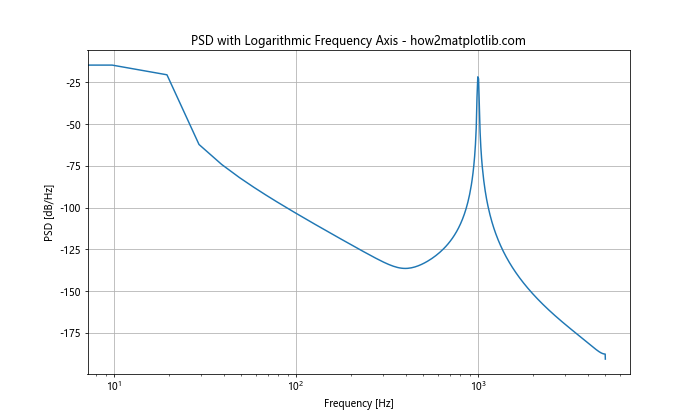
This example demonstrates how to plot the power spectral density using Matplotlib in Python with a logarithmic frequency axis, which can be useful for signals with wide frequency ranges.
Comparing Different PSD Estimation Methods
When you plot the power spectral density using Matplotlib in Python, it’s often insightful to compare different PSD estimation methods. Let’s look at how to do this:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal
np.random.seed(0)
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.random.randn(len(t))
# Compute PSDs using different methods
f_welch, Pxx_welch = signal.welch(sig, fs=1000, nperseg=256)
f_periodogram, Pxx_periodogram = signal.periodogram(sig, fs=1000)
f_lombscargle = np.linspace(0.1, 50, 500)
Pxx_lombscargle = signal.lombscargle(t, sig, f_lombscargle)
# Plot the power spectral densities
plt.figure(figsize=(10, 6))
plt.semilogy(f_welch, Pxx_welch, 'b-', label='Welch')
plt.semilogy(f_periodogram, Pxx_periodogram, 'r--', label='Periodogram')
plt.semilogy(f_lombscargle, Pxx_lombscargle, 'g-.', label='Lomb-Scargle')
plt.title('Comparison of PSD Estimation Methods - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.legend()
plt.grid(True)
plt.show()
Output:
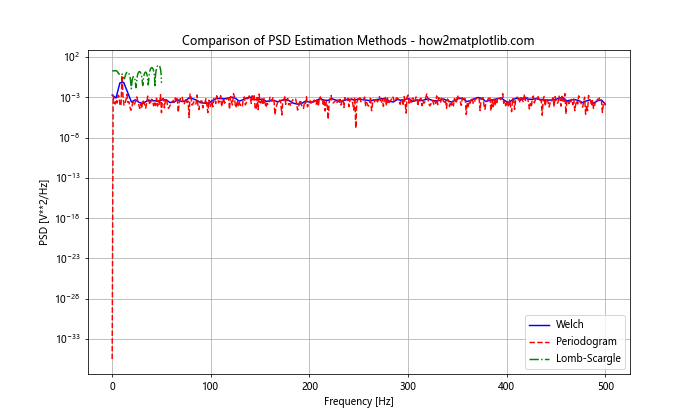
This example shows how to plot the power spectral density using Matplotlib in Python for three different PSD estimation methods: Welch’s method, the periodogram method, and the Lomb-Scargle periodogram. Each method has its strengths and is suitable for different types of data.
Visualizing PSD in 3D
Sometimes, it can be insightful to visualize the power spectral density in three dimensions, especially when dealing with time-varying signals. Here’s how you can create a 3D surface plot of a spectrogram:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
from mpl_toolkits.mplot3d import Axes3D
# Generate a chirp signal
t = np.linspace(0, 10, 1000)
w = signal.chirp(t, f0=1, f1=50, t1=10, method='linear')
# Compute spectrogram
f, t, Sxx = signal.spectrogram(w, fs=100)
# Create 3D surface plot
fig = plt.figure(figsize=(10, 8))
ax = fig.add_subplot(111, projection='3d')
T, F = np.meshgrid(t, f)
surf = ax.plot_surface(T, F, 10 * np.log10(Sxx), cmap='viridis')
ax.set_title('3D Spectrogram - how2matplotlib.com')
ax.set_xlabel('Time [sec]')
ax.set_ylabel('Frequency [Hz]')
ax.set_zlabel('PSD [dB]')
fig.colorbar(surf, shrink=0.5, aspect=5)
plt.show()
Output:
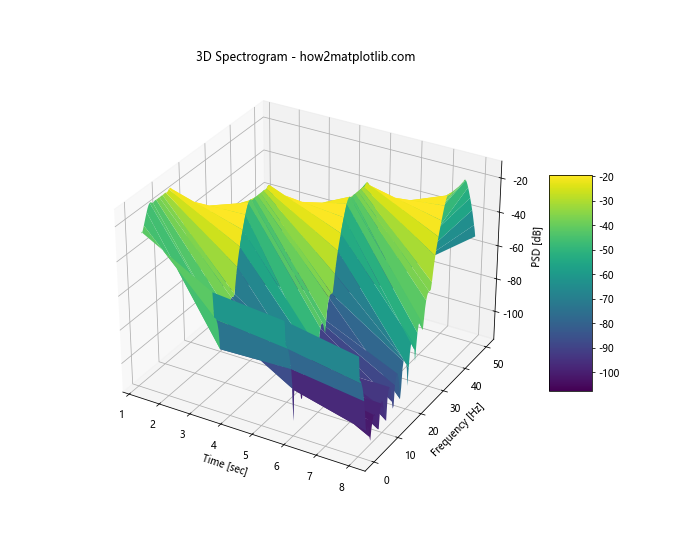
This example demonstrates how to plot the power spectral density using Matplotlib in Python as a 3D surface, providing a unique perspective on how the frequency content of a signal changes over time.
Handling Missing Data in PSD Analysis
When dealing with real-world data, you may encounter missing values. Here’s how you can handle missing data when plotting the power spectral density using Matplotlib in Python:
import numpy as np
import matplotlib.pyplot as plt
from scipy import signal
# Generate a sample signal with missing data
t = np.linspace(0, 1, 1000, endpoint=False)
sig = np.sin(2 * np.pi * 10 * t) + 0.5 * np.sin(2 * np.pi * 20 * t)
mask = np.random.choice([0, 1], size=len(sig), p=[0.1, 0.9])
sig_with_gaps = sig * mask
# Interpolate missing data
t_valid = t[mask == 1]
sig_valid = sig_with_gaps[mask == 1]
sig_interp = np.interp(t, t_valid, sig_valid)
# Compute PSDs
f_orig, Pxx_orig = signal.welch(sig, fs=1000, nperseg=256)
f_gaps, Pxx_gaps = signal.welch(sig_with_gaps, fs=1000, nperseg=256)
f_interp, Pxx_interp = signal.welch(sig_interp, fs=1000, nperseg=256)
# Plot the power spectral densities
plt.figure(figsize=(10, 6))
plt.semilogy(f_orig, Pxx_orig, 'b-', label='Original')
plt.semilogy(f_gaps, Pxx_gaps, 'r--', label='With Gaps')
plt.semilogy(f_interp, Pxx_interp, 'g-.', label='Interpolated')
plt.title('PSD: Handling Missing Data - how2matplotlib.com')
plt.xlabel('Frequency [Hz]')
plt.ylabel('PSD [V**2/Hz]')
plt.legend()
plt.grid(True)
plt.show()
Output:
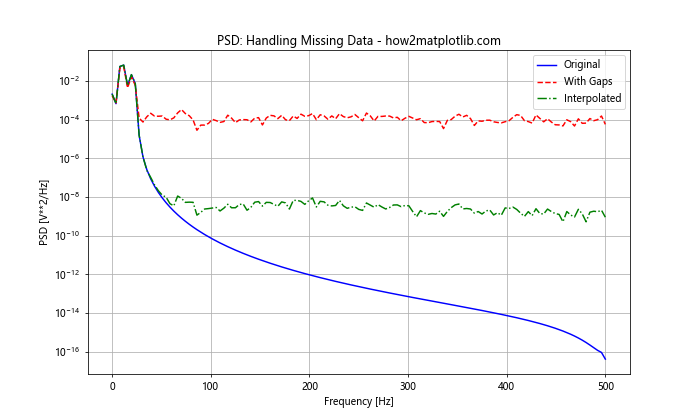
This example illustrates how to plot the power spectral density using Matplotlib in Python for a signal with missing data, comparing the original signal, the signal with gaps, and an interpolated version of the signal.
Conclusion
In this comprehensive guide, we’ve explored numerous aspects of how to plot the power spectral density using Matplotlib in Python. We’ve covered basic PSD plotting, customization techniques, advanced analysis methods, and ways to handle real-world data challenges. By mastering these techniques, you’ll be well-equipped to perform detailed spectral analysis on a wide variety of signals.
Remember that when you plot the power spectral density using Matplotlib in Python, you’re not just creating a visualization – you’re providing a powerful tool for understanding the frequency content of your signals. Whether you’re working in signal processing, audio analysis, vibration analysis, or any other field that deals with time-series data, the ability to effectively plot and interpret power spectral density is an invaluable skill.