Comprehensive Guide to Matplotlib.axis.Tick.update_from() Function in Python
Matplotlib.axis.Tick.update_from() function in Python is a powerful tool for updating tick properties in Matplotlib plots. This function allows you to efficiently copy properties from one tick object to another, making it easier to maintain consistency across your visualizations. In this comprehensive guide, we’ll explore the Matplotlib.axis.Tick.update_from() function in detail, providing numerous examples and explanations to help you master this essential feature of Matplotlib.
Understanding the Matplotlib.axis.Tick.update_from() Function
The Matplotlib.axis.Tick.update_from() function is a method of the Tick class in Matplotlib’s axis module. Its primary purpose is to update the properties of a tick object based on another tick object. This function is particularly useful when you want to maintain consistency between different ticks or when you need to apply a set of properties to multiple ticks efficiently.
Let’s start with a basic example to illustrate how the Matplotlib.axis.Tick.update_from() function works:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
tick1 = ax.xaxis.get_major_ticks()[0]
# Get the second x-axis tick
tick2 = ax.xaxis.get_major_ticks()[1]
# Update tick2 properties from tick1
tick2.update_from(tick1)
plt.legend()
plt.show()
Output:
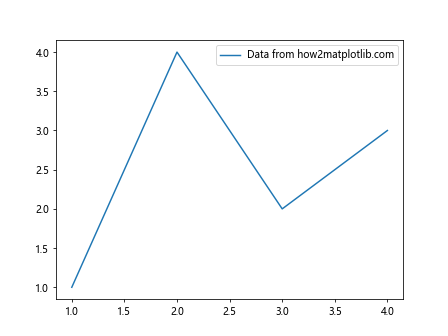
In this example, we create a simple plot and then use the Matplotlib.axis.Tick.update_from() function to update the properties of the second x-axis tick based on the first x-axis tick. This ensures that both ticks have the same properties.
Key Parameters of Matplotlib.axis.Tick.update_from()
The Matplotlib.axis.Tick.update_from() function has several important parameters that allow you to control which properties are updated. Let’s explore these parameters in detail:
other
: This is the source tick object from which properties will be copied.which
: A string or tuple specifying which properties to update. Options include ‘grid’, ‘tick1’, ‘tick2’, and ‘label’.
Here’s an example demonstrating the use of these parameters:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
tick1 = ax.xaxis.get_major_ticks()[0]
tick2 = ax.xaxis.get_major_ticks()[1]
# Update only the grid properties
tick2.update_from(tick1, which='grid')
plt.legend()
plt.show()
In this example, we update only the grid properties of tick2 based on tick1.
Updating Multiple Ticks with Matplotlib.axis.Tick.update_from()
One of the most powerful features of the Matplotlib.axis.Tick.update_from() function is its ability to update multiple ticks at once. This can be particularly useful when you want to apply a consistent style to all ticks on an axis.
Here’s an example of how to update all x-axis ticks based on the properties of the first tick:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4, 5], [1, 4, 2, 3, 5], label='Data from how2matplotlib.com')
# Get all x-axis ticks
x_ticks = ax.xaxis.get_major_ticks()
# Update all ticks based on the first tick
for tick in x_ticks[1:]:
tick.update_from(x_ticks[0])
plt.legend()
plt.show()
Output:
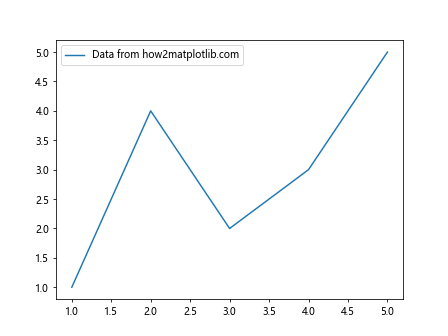
In this example, we iterate through all x-axis ticks (except the first one) and update their properties based on the first tick. This ensures that all ticks have consistent properties.
Customizing Tick Properties Before Using Matplotlib.axis.Tick.update_from()
Before using the Matplotlib.axis.Tick.update_from() function, you can customize the properties of the source tick. This allows you to create a template tick with the desired properties and then apply those properties to other ticks.
Here’s an example of how to customize tick properties before updating:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(14)
template_tick.label1.set_color('red')
template_tick.tick1line.set_linewidth(2)
# Update all other ticks based on the template
for tick in ax.xaxis.get_major_ticks()[1:]:
tick.update_from(template_tick)
plt.legend()
plt.show()
Output:
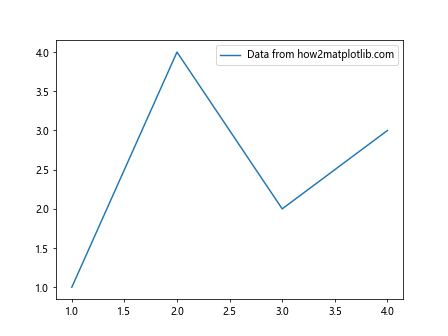
In this example, we customize the font size, color, and line width of the first tick, and then use it as a template to update all other ticks.
Using Matplotlib.axis.Tick.update_from() with Different Axis Types
The Matplotlib.axis.Tick.update_from() function can be used with different types of axes, including x-axis, y-axis, and even polar axes. Let’s explore how to use this function with different axis types:
Updating Y-axis Ticks
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first y-axis tick
template_tick = ax.yaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('blue')
# Update all other y-axis ticks
for tick in ax.yaxis.get_major_ticks()[1:]:
tick.update_from(template_tick)
plt.legend()
plt.show()
Output:
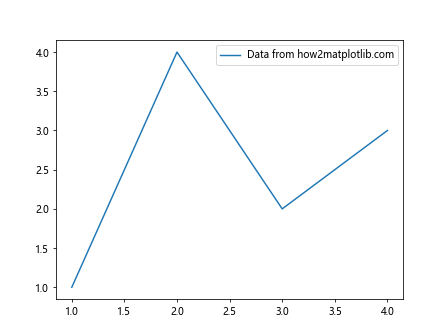
In this example, we update the y-axis ticks based on a customized template tick.
Updating Polar Axis Ticks
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(subplot_kw=dict(projection='polar'))
theta = np.linspace(0, 2*np.pi, 8, endpoint=False)
radii = np.random.rand(8)
ax.plot(theta, radii, label='Data from how2matplotlib.com')
# Get the first radial tick
template_tick = ax.yaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(10)
template_tick.label1.set_color('green')
# Update all other radial ticks
for tick in ax.yaxis.get_major_ticks()[1:]:
tick.update_from(template_tick)
plt.legend()
plt.show()
Output:
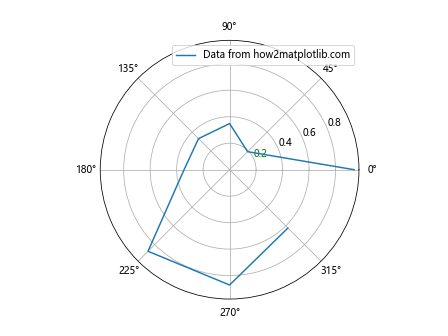
This example demonstrates how to update ticks in a polar plot using the Matplotlib.axis.Tick.update_from() function.
Advanced Usage of Matplotlib.axis.Tick.update_from()
Now that we’ve covered the basics, let’s explore some advanced usage scenarios for the Matplotlib.axis.Tick.update_from() function.
Selective Property Updates
You can use the which
parameter to selectively update specific properties of a tick. Here’s an example that updates only the label properties:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
# Customize the template tick label
template_tick.label1.set_fontsize(14)
template_tick.label1.set_color('purple')
# Update only the label properties of other ticks
for tick in ax.xaxis.get_major_ticks()[1:]:
tick.update_from(template_tick, which='label')
plt.legend()
plt.show()
In this example, we update only the label properties of the ticks, leaving other properties unchanged.
Combining Multiple Property Updates
You can update multiple properties at once by passing a tuple to the which
parameter:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('orange')
template_tick.tick1line.set_linewidth(2)
template_tick.tick1line.set_color('green')
# Update label and tick1 properties of other ticks
for tick in ax.xaxis.get_major_ticks()[1:]:
tick.update_from(template_tick, which=('label', 'tick1'))
plt.legend()
plt.show()
This example demonstrates how to update both label and tick line properties simultaneously.
Handling Exceptions with Matplotlib.axis.Tick.update_from()
When using the Matplotlib.axis.Tick.update_from() function, it’s important to handle potential exceptions that may occur. Here’s an example of how to handle exceptions when updating tick properties:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('red')
# Update other ticks with exception handling
for tick in ax.xaxis.get_major_ticks()[1:]:
try:
tick.update_from(template_tick)
except AttributeError as e:
print(f"Error updating tick: {e}")
# Handle the error or continue to the next tick
plt.legend()
plt.show()
Output:
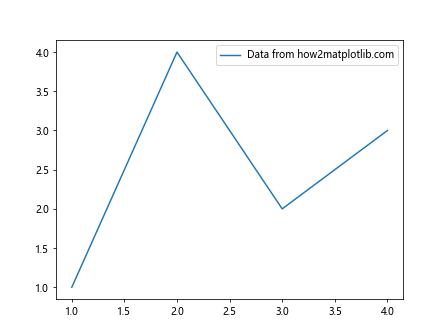
In this example, we use a try-except block to catch and handle any AttributeError that may occur during the update process.
Combining Matplotlib.axis.Tick.update_from() with Other Tick Customizations
The Matplotlib.axis.Tick.update_from() function can be combined with other tick customization techniques to create highly customized plots. Here’s an example that demonstrates this:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('blue')
template_tick.tick1line.set_linewidth(2)
# Update other ticks
for tick in ax.xaxis.get_major_ticks()[1:]:
tick.update_from(template_tick)
# Additional customizations
ax.tick_params(axis='x', rotation=45)
ax.set_xticks([1, 2, 3, 4])
ax.set_xticklabels(['A', 'B', 'C', 'D'])
plt.legend()
plt.tight_layout()
plt.show()
Output:
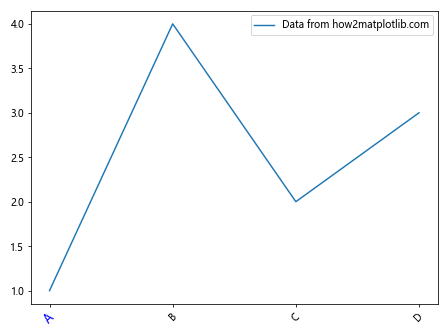
In this example, we combine the Matplotlib.axis.Tick.update_from() function with other tick customization techniques such as rotating tick labels and setting custom tick positions and labels.
Using Matplotlib.axis.Tick.update_from() in Subplots
The Matplotlib.axis.Tick.update_from() function can be particularly useful when working with subplots, allowing you to maintain consistency across multiple plots. Here’s an example:
import matplotlib.pyplot as plt
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 4))
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data 1 from how2matplotlib.com')
ax2.plot([1, 2, 3, 4], [3, 1, 4, 2], label='Data 2 from how2matplotlib.com')
# Get the first x-axis tick from the first subplot
template_tick = ax1.xaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('red')
# Update ticks in both subplots
for ax in (ax1, ax2):
for tick in ax.xaxis.get_major_ticks():
tick.update_from(template_tick)
ax1.legend()
ax2.legend()
plt.tight_layout()
plt.show()
Output:
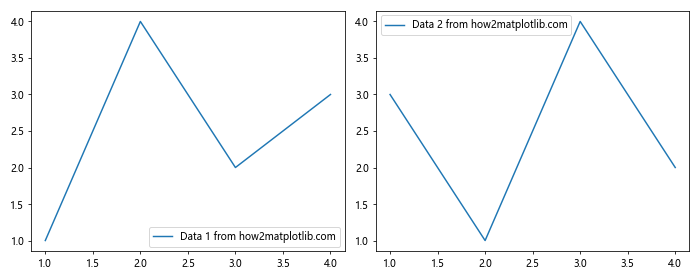
This example demonstrates how to use a single template tick to update ticks across multiple subplots, ensuring consistency in tick appearance.
Animating Tick Updates with Matplotlib.axis.Tick.update_from()
The Matplotlib.axis.Tick.update_from() function can also be used in animations to create dynamic tick updates. Here’s an example of how to create a simple animation that updates tick properties:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
def animate(frame):
# Update template tick properties
template_tick.label1.set_fontsize(10 + frame)
template_tick.label1.set_color(plt.cm.viridis(frame / 10))
# Update all ticks
for tick in ax.xaxis.get_major_ticks():
tick.update_from(template_tick)
return line,
ani = animation.FuncAnimation(fig, animate, frames=10, interval=500, blit=True)
plt.legend()
plt.show()
Output:
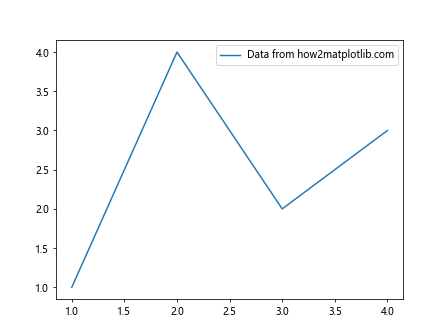
In this example, we create an animation that gradually changes the font size and color of the tick labels using the Matplotlib.axis.Tick.update_from() function.
Performance Considerations for Matplotlib.axis.Tick.update_from()
While the Matplotlib.axis.Tick.update_from() function is a powerful tool for updating tick properties, it’s important to consider performance when working with large numbers of ticks or frequent updates. Here are some tips to optimize performance:
- Update only the necessary properties by using the
which
parameter. - Avoid updating ticks in tight loops or high-frequency animations.
- Consider using vectorized operations for large-scale tick updates.
Here’s an example that demonstrates a more efficient way to update a large number of ticks:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
# Create a plot with many data points
x = np.linspace(0, 10, 1000)
y = np.sin(x)
ax.plot(x, y, label='Data from how2matplotlib.com')
# Get all x-axis ticks
x_ticks = ax.xaxis.get_major_ticks()
# Create a template tick
template_tick = x_ticks[0]
template_tick.label1.set_fontsize(10)
template_tick.label1.set_color('red')
# Efficiently update all ticks
for tick in x_ticks:
tick.update_from(template_tick, which='label')
plt.legend()
plt.show()
In this example, we update only the label properties of a large number of ticks, which is more efficient than updating all properties.
Troubleshooting Common Issues with Matplotlib.axis.Tick.update_from()
When using the Matplotlib.axis.Tick.update_from() function, you may encounter some common issues. Here are a few problems and their solutions:
- Ticks not updating: Ensure that you’re calling
plt.draw()
orplt.show()
after updating the ticks. - AttributeError: Make sure you’re using compatible tick objects and updating properties that exist on both ticks.
- Unexpected behavior: Double-check that you’re updating the correct axis (x or y) and the correct tick object.
Here’s an example that demonstrates how to handle these issues:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('blue')
# Update other ticks with error handling
for tick in ax.xaxis.get_major_ticks()[1:]:
try:
tick.update_from(template_tick)
except AttributeError as e:
print(f"Error updating tick: {e}")
except Exception as e:
print(f"Unexpected error: {e}")
plt.legend()
plt.draw() # Ensure updates are applied
plt.show()
Output:
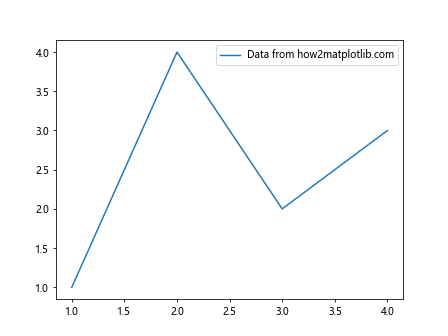
This example includes error handling and explicitly calls plt.draw()
to ensure the updates are applied.
Integrating Matplotlib.axis.Tick.update_from() with Other Matplotlib Features
The Matplotlib.axis.Tick.update_from() function can be integrated with other Matplotlib features to create more complex and customized visualizations. Here are a few examples:
Combining with Custom Tick Formatters
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [100, 400, 200, 300], label='Data from how2matplotlib.com')
# Create a custom tick formatter
def currency_formatter(x, pos):
return f'${x:,.0f}'
# Apply the formatter to the y-axis
ax.yaxis.set_major_formatter(ticker.FuncFormatter(currency_formatter))
# Get the first y-axis tick
template_tick = ax.yaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('green')
# Update other ticks
for tick in ax.yaxis.get_major_ticks()[1:]:
tick.update_from(template_tick)
plt.legend()
plt.show()
Output:
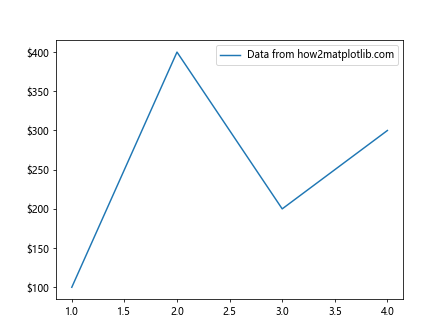
This example combines a custom tick formatter with the Matplotlib.axis.Tick.update_from() function to create currency-formatted tick labels with consistent styling.
Using with Logarithmic Scales
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.logspace(0, 3, 50)
y = x**2
ax.loglog(x, y, label='Data from how2matplotlib.com')
# Get the first x-axis tick
template_tick = ax.xaxis.get_major_ticks()[0]
# Customize the template tick
template_tick.label1.set_fontsize(10)
template_tick.label1.set_color('purple')
# Update other ticks
for tick in ax.xaxis.get_major_ticks()[1:]:
tick.update_from(template_tick)
plt.legend()
plt.show()
Output:
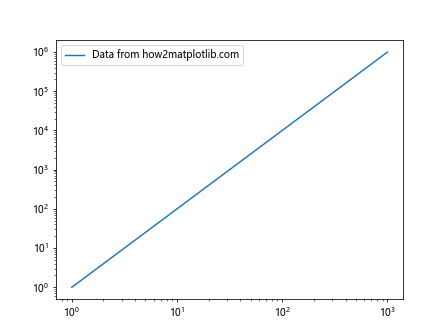
This example demonstrates how to use the Matplotlib.axis.Tick.update_from() function with logarithmic scales.
Best Practices for Using Matplotlib.axis.Tick.update_from()
To make the most of the Matplotlib.axis.Tick.update_from() function, consider the following best practices:
- Create a template tick with all desired properties before updating other ticks.
- Use the
which
parameter to update only necessary properties for better performance. - Combine with other Matplotlib customization techniques for more flexible visualizations.
- Handle exceptions to ensure robustness in your code.
- Use vectorized operations when working with large numbers of ticks.
Here’s an example that incorporates these best practices:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y = np.sin(x)
ax.plot(x, y, label='Data from how2matplotlib.com')
# Create a template tick with desired properties
template_tick = ax.xaxis.get_major_ticks()[0]
template_tick.label1.set_fontsize(12)
template_tick.label1.set_color('red')
template_tick.tick1line.set_linewidth(2)
# Update other ticks efficiently
for tick in ax.xaxis.get_major_ticks()[1:]:
try:
tick.update_from(template_tick, which=('label', 'tick1'))
except AttributeError as e:
print(f"Error updating tick: {e}")
# Additional customizations
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.grid(True, linestyle='--', alpha=0.7)
plt.legend()
plt.tight_layout()
plt.show()
This example demonstrates best practices for using the Matplotlib.axis.Tick.update_from() function, including efficient updating, error handling, and integration with other customization techniques.
Conclusion
The Matplotlib.axis.Tick.update_from() function is a powerful tool for updating tick properties in Matplotlib plots. By mastering this function, you can create consistent and visually appealing visualizations with ease. Remember to consider performance, handle exceptions, and combine it with other Matplotlib features for the best results. With the knowledge and examples provided in this comprehensive guide, you’re now well-equipped to use the Matplotlib.axis.Tick.update_from() function effectively in your Python data visualization projects.