Comprehensive Guide to Using Matplotlib.axis.Tick.get_snap() in Python for Data Visualization
Matplotlib.axis.Tick.get_snap() in Python is an essential method for fine-tuning the appearance of tick marks in Matplotlib plots. This article will delve deep into the functionality, usage, and practical applications of the get_snap() method, providing you with a comprehensive understanding of how to leverage this powerful tool in your data visualization projects.
Understanding Matplotlib.axis.Tick.get_snap() in Python
Matplotlib.axis.Tick.get_snap() in Python is a method that belongs to the Tick class in Matplotlib’s axis module. This method is used to retrieve the snap setting for a specific tick mark on an axis. The snap setting determines whether the tick mark should be adjusted to align with pixel boundaries, which can result in crisper and more visually appealing plots.
To better understand how Matplotlib.axis.Tick.get_snap() in Python works, let’s start with a simple example:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
ax.legend()
# Get the first x-axis tick
tick = ax.xaxis.get_major_ticks()[0]
# Get the snap setting for the tick
snap_setting = tick.get_snap()
print(f"Snap setting for the first x-axis tick: {snap_setting}")
plt.show()
Output:
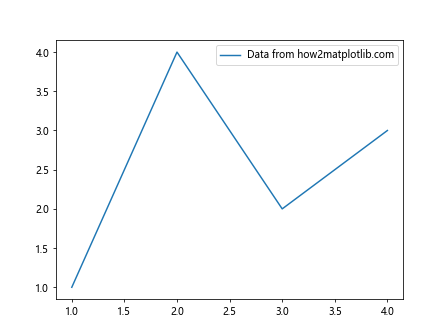
In this example, we create a simple line plot and then retrieve the first major tick on the x-axis. We use the Matplotlib.axis.Tick.get_snap() in Python method to get the snap setting for this tick. The result will be printed to the console, showing whether snapping is enabled for this particular tick.
The Importance of Matplotlib.axis.Tick.get_snap() in Python
Understanding and utilizing Matplotlib.axis.Tick.get_snap() in Python is crucial for creating high-quality, professional-looking visualizations. By controlling the snap setting of individual ticks, you can ensure that your plots are rendered with precision and clarity, especially when working with high-resolution displays or when preparing figures for publication.
Let’s explore another example that demonstrates the importance of Matplotlib.axis.Tick.get_snap() in Python:
import matplotlib.pyplot as plt
import numpy as np
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 5))
x = np.linspace(0, 10, 100)
y = np.sin(x)
ax1.plot(x, y, label='Sine wave from how2matplotlib.com')
ax1.set_title('Default Tick Snapping')
ax1.legend()
ax2.plot(x, y, label='Sine wave from how2matplotlib.com')
ax2.set_title('Custom Tick Snapping')
ax2.legend()
# Modify snap setting for x-axis ticks in the second subplot
for tick in ax2.xaxis.get_major_ticks():
tick.set_snap(False)
# Print snap settings for both subplots
for i, ax in enumerate([ax1, ax2], 1):
print(f"Subplot {i} x-axis tick snap settings:")
for j, tick in enumerate(ax.xaxis.get_major_ticks()):
print(f" Tick {j}: {tick.get_snap()}")
plt.tight_layout()
plt.show()
Output:
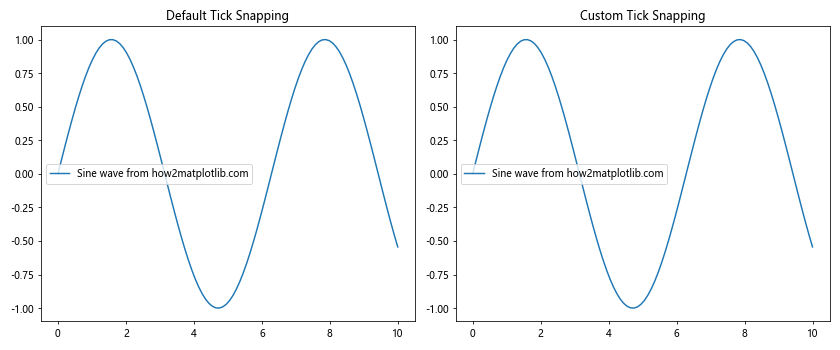
In this example, we create two subplots with identical sine waves. For the second subplot, we modify the snap setting of the x-axis ticks using the set_snap()
method. We then use Matplotlib.axis.Tick.get_snap() in Python to retrieve and print the snap settings for both subplots, allowing us to compare the differences.
Advanced Usage of Matplotlib.axis.Tick.get_snap() in Python
Matplotlib.axis.Tick.get_snap() in Python can be particularly useful when working with complex plots or when you need to fine-tune the appearance of your visualizations. Let’s explore some advanced scenarios where this method can be beneficial.
Customizing Tick Snapping for Different Axis Scales
When working with different axis scales, such as logarithmic or symlog scales, you may want to adjust the snap settings accordingly. Here’s an example that demonstrates how to use Matplotlib.axis.Tick.get_snap() in Python with different scales:
import matplotlib.pyplot as plt
import numpy as np
fig, (ax1, ax2, ax3) = plt.subplots(1, 3, figsize=(15, 5))
x = np.logspace(0, 2, 100)
y = x**2
ax1.plot(x, y, label='Linear scale (how2matplotlib.com)')
ax1.set_title('Linear Scale')
ax1.set_xscale('linear')
ax1.legend()
ax2.plot(x, y, label='Log scale (how2matplotlib.com)')
ax2.set_title('Log Scale')
ax2.set_xscale('log')
ax2.legend()
ax3.plot(x, y, label='Symlog scale (how2matplotlib.com)')
ax3.set_title('Symlog Scale')
ax3.set_xscale('symlog')
ax3.legend()
# Print snap settings for all subplots
for i, ax in enumerate([ax1, ax2, ax3], 1):
print(f"Subplot {i} x-axis tick snap settings:")
for j, tick in enumerate(ax.xaxis.get_major_ticks()):
print(f" Tick {j}: {tick.get_snap()}")
plt.tight_layout()
plt.show()
Output:
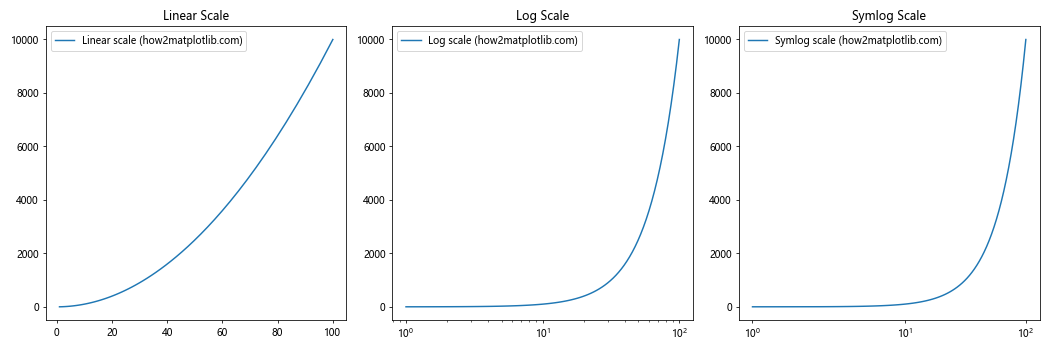
In this example, we create three subplots with different x-axis scales: linear, logarithmic, and symlog. We then use Matplotlib.axis.Tick.get_snap() in Python to retrieve and print the snap settings for each tick on the x-axis of each subplot. This allows us to observe how the snap settings may vary depending on the axis scale.
Combining Matplotlib.axis.Tick.get_snap() with Other Tick Properties
Matplotlib.axis.Tick.get_snap() in Python can be used in conjunction with other tick properties to create highly customized and visually appealing plots. Let’s explore an example that combines snap settings with tick label rotation and alignment:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(figsize=(10, 6))
x = np.arange(0, 10, 0.1)
y = np.sin(x)
ax.plot(x, y, label='Sine wave from how2matplotlib.com')
ax.set_title('Customized Tick Properties')
ax.legend()
# Customize x-axis ticks
for i, tick in enumerate(ax.xaxis.get_major_ticks()):
if i % 2 == 0:
tick.set_snap(True)
tick.label1.set_rotation(45)
else:
tick.set_snap(False)
tick.label1.set_rotation(-45)
tick.label1.set_ha('right')
# Print snap settings and rotation for x-axis ticks
for i, tick in enumerate(ax.xaxis.get_major_ticks()):
snap = tick.get_snap()
rotation = tick.label1.get_rotation()
print(f"Tick {i}: Snap = {snap}, Rotation = {rotation}")
plt.tight_layout()
plt.show()
Output:
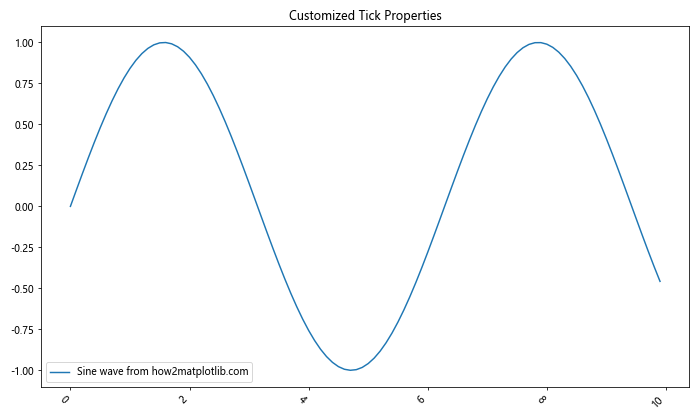
In this example, we create a sine wave plot and customize the x-axis ticks by alternating their snap settings and label rotations. We use Matplotlib.axis.Tick.get_snap() in Python to retrieve the snap settings for each tick and print them along with the corresponding label rotations.
Practical Applications of Matplotlib.axis.Tick.get_snap() in Python
Now that we have a solid understanding of how Matplotlib.axis.Tick.get_snap() in Python works, let’s explore some practical applications where this method can be particularly useful.
Creating Publication-Quality Figures
When preparing figures for academic publications or professional reports, it’s crucial to ensure that your plots are rendered with the highest possible quality. Matplotlib.axis.Tick.get_snap() in Python can help you achieve this by allowing you to fine-tune the appearance of tick marks. Here’s an example that demonstrates how to create a publication-quality figure:
import matplotlib.pyplot as plt
import numpy as np
plt.rcParams['font.family'] = 'serif'
plt.rcParams['font.size'] = 12
plt.rcParams['axes.linewidth'] = 1.5
fig, ax = plt.subplots(figsize=(8, 6), dpi=300)
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
ax.plot(x, y1, label='Sine (how2matplotlib.com)', linewidth=2)
ax.plot(x, y2, label='Cosine (how2matplotlib.com)', linewidth=2)
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
ax.set_title('Publication-Quality Figure')
ax.legend()
# Customize tick properties
for axis in [ax.xaxis, ax.yaxis]:
for tick in axis.get_major_ticks():
tick.set_snap(True)
tick.label1.set_fontweight('bold')
# Print snap settings for all ticks
for axis_name, axis in [('X-axis', ax.xaxis), ('Y-axis', ax.yaxis)]:
print(f"{axis_name} tick snap settings:")
for i, tick in enumerate(axis.get_major_ticks()):
print(f" Tick {i}: {tick.get_snap()}")
plt.tight_layout()
plt.savefig('publication_quality_figure.png', dpi=300, bbox_inches='tight')
plt.show()
Output:
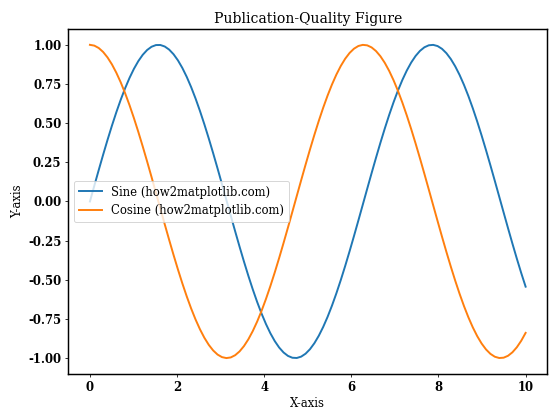
In this example, we create a high-resolution figure with customized font properties and line widths. We use Matplotlib.axis.Tick.get_snap() in Python to ensure that all tick marks are snapped to pixel boundaries, resulting in a crisp and professional appearance. We also print the snap settings for all ticks to verify our customizations.
Handling Large Datasets with Matplotlib.axis.Tick.get_snap()
When working with large datasets, it’s important to optimize the rendering of your plots to maintain performance. Matplotlib.axis.Tick.get_snap() in Python can be used in conjunction with other techniques to create efficient visualizations of large datasets. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(figsize=(10, 6))
# Generate a large dataset
np.random.seed(42)
n_points = 1000000
x = np.random.randn(n_points)
y = np.random.randn(n_points)
# Plot the data using a 2D histogram
hist, xedges, yedges = np.histogram2d(x, y, bins=100)
extent = [xedges[0], xedges[-1], yedges[0], yedges[-1]]
im = ax.imshow(hist.T, extent=extent, origin='lower', cmap='viridis')
ax.set_title(f'2D Histogram of {n_points} Points (how2matplotlib.com)')
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
# Add a colorbar
cbar = plt.colorbar(im)
cbar.set_label('Count')
# Customize tick properties
for axis in [ax.xaxis, ax.yaxis]:
for tick in axis.get_major_ticks():
tick.set_snap(True)
# Print snap settings for all ticks
for axis_name, axis in [('X-axis', ax.xaxis), ('Y-axis', ax.yaxis)]:
print(f"{axis_name} tick snap settings:")
for i, tick in enumerate(axis.get_major_ticks()):
print(f" Tick {i}: {tick.get_snap()}")
plt.tight_layout()
plt.show()
Output:
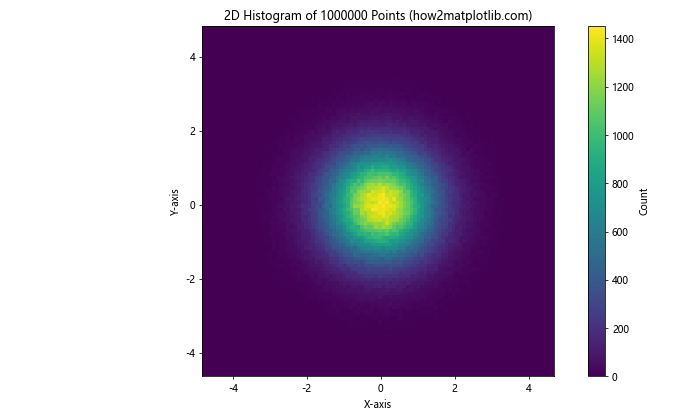
In this example, we generate a large dataset with one million points and visualize it using a 2D histogram. We use Matplotlib.axis.Tick.get_snap() in Python to ensure that all tick marks are snapped to pixel boundaries, which can help improve the overall appearance of the plot when dealing with dense data.
Troubleshooting Common Issues with Matplotlib.axis.Tick.get_snap() in Python
While Matplotlib.axis.Tick.get_snap() in Python is a powerful tool, you may encounter some issues when using it. Here are some common problems and their solutions:
Issue: Tick Snapping Not Working as Expected
In some cases, you may find that setting the snap property doesn’t seem to have any effect on the tick appearance.
Solution:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(figsize=(10, 6))
x = np.linspace(0, 10, 100)
y = np.cos(x)
ax.plot(x, y, label='Cosine wave (how2matplotlib.com)')
ax.set_title('Effective Tick Snapping')
ax.legend()
# Ensure effective tick snapping
ax.minorticks_on()
ax.tick_params(which='both', width=1.5, length=4, direction='in')
for axis in [ax.xaxis, ax.yaxis]:
for tick in axis.get_major_ticks() + axis.get_minor_ticks():
tick.set_snap(True)
# Print snap settings for all ticks
for axis_name, axis in [('X-axis', ax.xaxis), ('Y-axis', ax.yaxis)]:
print(f"{axis_name} tick snap settings:")
for i, tick in enumerate(axis.get_major_ticks() + axis.get_minor_ticks()):
print(f" Tick {i}: {tick.get_snap()}")
plt.tight_layout()
plt.show()
Output:
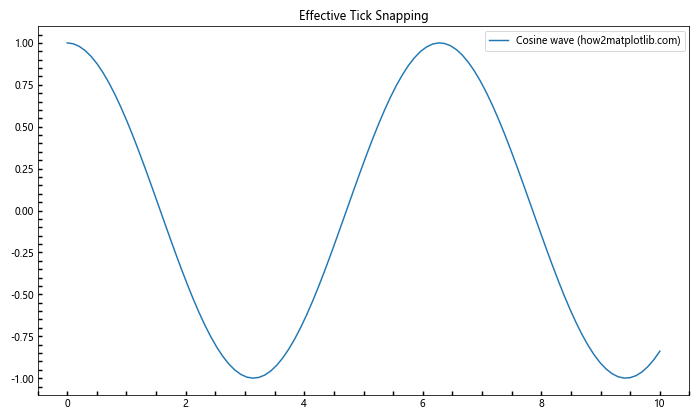
In this example, we enable minor ticks and adjust the tick parameters to make the snapping effect more noticeable. We also apply the snap setting to both major and minor ticks.
Advanced Techniques with Matplotlib.axis.Tick.get_snap() in Python
Now that we’ve covered the basics and troubleshooting, let’s explore some advanced techniques using Matplotlib.axis.Tick.get_snap() in Python.
Technique 1: Dynamic Tick Snapping
You can create plots with dynamic tick snapping that changes based on user interaction or data updates.
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.widgets import CheckButtons
fig, ax = plt.subplots(figsize=(10, 6))
x = np.linspace(0, 10, 100)
y = np.sin(x)
line, = ax.plot(x, y, label='Sine wave (how2matplotlib.com)')
ax.set_title('Dynamic Tick Snapping')
ax.legend()
# Create check buttons for toggling snap
ax_check = plt.axes([0.02, 0.9, 0.15, 0.1])
check = CheckButtons(ax_check, ['Snap Ticks'], [True])
def toggle_snap(label):
snap_enabled = check.get_status()[0]
for axis in [ax.xaxis, ax.yaxis]:
for tick in axis.get_major_ticks():
tick.set_snap(snap_enabled)
# Print current snap settings
print("Current tick snap settings:")
for axis_name, axis in [('X-axis', ax.xaxis), ('Y-axis', ax.yaxis)]:
print(f" {axis_name}:")
for i, tick in enumerate(axis.get_major_ticks()):
print(f" Tick {i}: {tick.get_snap()}")
fig.canvas.draw_idle()
check.on_clicked(toggle_snap)
plt.tight_layout()
plt.show()
Output:
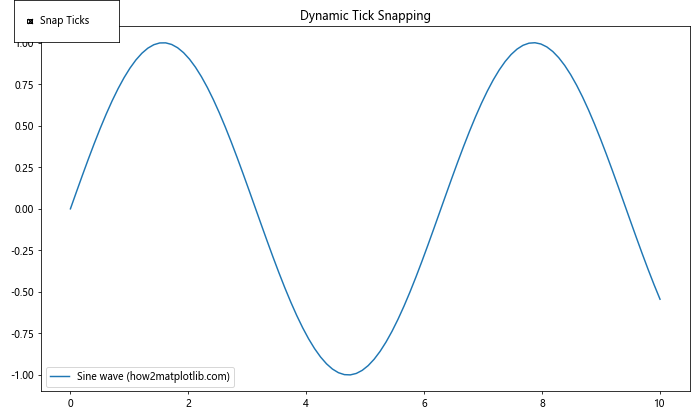
This example creates a plot with a checkbox that allows the user to toggle tick snapping on and off dynamically.
Technique 2: Custom Tick Snapping Logic
You can create custom logic for tick snapping based on specific conditions or data properties.
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(figsize=(10, 6))
x = np.linspace(0, 10, 100)
y = np.exp(-0.5 * x) * np.sin(2 * np.pi * x)
ax.plot(x, y, label='Damped sine wave (how2matplotlib.com)')
ax.set_title('Custom Tick Snapping Logic')
ax.legend()
def custom_snap_logic(tick_loc):
# Snap ticks to nearest 0.5 if they're close
return abs(tick_loc - round(tick_loc * 2) / 2) < 0.1
# Apply custom snap logic
for axis in [ax.xaxis, ax.yaxis]:
for tick in axis.get_major_ticks():
tick_loc = tick.get_loc()
should_snap = custom_snap_logic(tick_loc)
tick.set_snap(should_snap)
# Print snap settings for all ticks
for axis_name, axis in [('X-axis', ax.xaxis), ('Y-axis', ax.yaxis)]:
print(f"{axis_name} tick snap settings:")
for i, tick in enumerate(axis.get_major_ticks()):
print(f" Tick {i}: Location = {tick.get_loc():.2f}, Snap = {tick.get_snap()}")
plt.tight_layout()
plt.show()
Output:
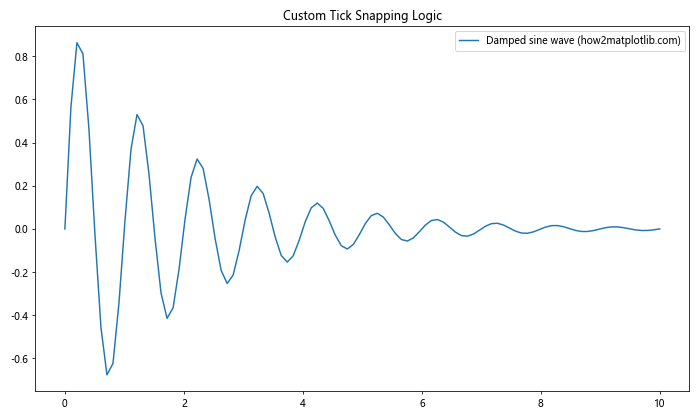
In this example, we define a custom snapping logic that snaps ticks to the nearest 0.5 if they're within a certain threshold.
Integrating Matplotlib.axis.Tick.get_snap() with Other Matplotlib Features
Matplotlib.axis.Tick.get_snap() in Python can be effectively combined with other Matplotlib features to create more complex and informative visualizations. Let's explore some examples of how to integrate this method with other Matplotlib functionalities.
Combining with Subplots and GridSpec
You can use Matplotlib.axis.Tick.get_snap() in Python in conjunction with subplots and GridSpec to create complex layouts with customized tick snapping.
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.gridspec import GridSpec
fig = plt.figure(figsize=(12, 8))
gs = GridSpec(2, 2, figure=fig)
ax1 = fig.add_subplot(gs[0, 0])
ax2 = fig.add_subplot(gs[0, 1])
ax3 = fig.add_subplot(gs[1, :])
x = np.linspace(0, 10, 100)
ax1.plot(x, np.sin(x), label='Sine (how2matplotlib.com)')
ax1.set_title('Subplot 1')
ax1.legend()
ax2.plot(x, np.cos(x), label='Cosine (how2matplotlib.com)')
ax2.set_title('Subplot 2')
ax2.legend()
ax3.plot(x, np.tan(x), label='Tangent (how2matplotlib.com)')
ax3.set_title('Subplot 3')
ax3.set_ylim(-5, 5)
ax3.legend()
# Customize tick snapping for each subplot
for ax in [ax1, ax2, ax3]:
for axis in [ax.xaxis, ax.yaxis]:
for tick in axis.get_major_ticks():
tick.set_snap(True)
# Print snap settings for all subplots
for i, ax in enumerate([ax1, ax2, ax3], 1):
print(f"Subplot {i} tick snap settings:")
for axis_name, axis in [('X-axis', ax.xaxis), ('Y-axis', ax.yaxis)]:
print(f" {axis_name}:")
for j, tick in enumerate(axis.get_major_ticks()):
print(f" Tick {j}: {tick.get_snap()}")
plt.tight_layout()
plt.show()
Output:
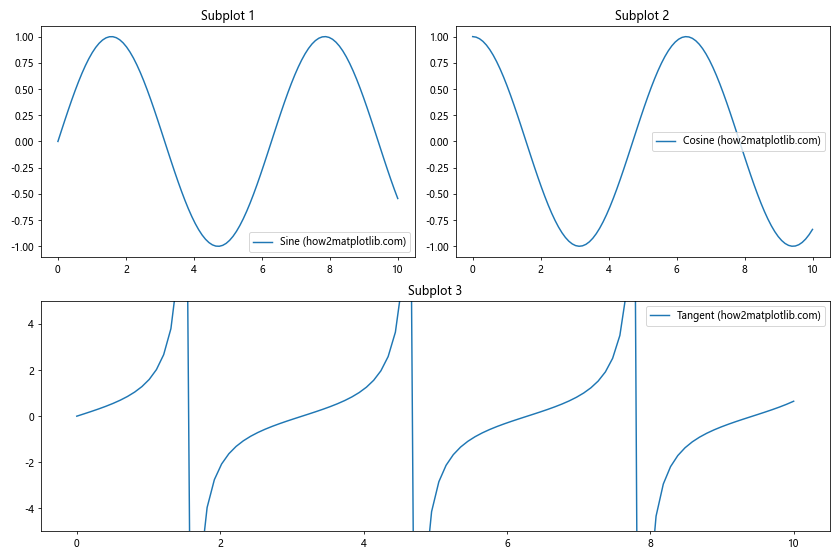
This example demonstrates how to use Matplotlib.axis.Tick.get_snap() in Python with a complex layout created using GridSpec.
Integrating with Custom Projections
You can also use Matplotlib.axis.Tick.get_snap() in Python with custom projections, such as polar plots or 3D plots.
import matplotlib.pyplot as plt
import numpy as np
fig = plt.figure(figsize=(12, 5))
# Polar plot
ax1 = fig.add_subplot(121, projection='polar')
theta = np.linspace(0, 2*np.pi, 100)
r = np.sin(4*theta)
ax1.plot(theta, r, label='r = sin(4θ) (how2matplotlib.com)')
ax1.set_title('Polar Plot')
ax1.legend()
# 3D plot
ax2 = fig.add_subplot(122, projection='3d')
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
ax2.plot_surface(X, Y, Z, cmap='viridis')
ax2.set_title('3D Surface Plot (how2matplotlib.com)')
# Customize tick snapping for both plots
for ax in [ax1, ax2]:
for axis in ax.get_xaxis(), ax.get_yaxis():
if hasattr(axis, 'get_major_ticks'): # Check if the axis has ticks
for tick in axis.get_major_ticks():
tick.set_snap(True)
# Print snap settings for both plots
for i, ax in enumerate([ax1, ax2], 1):
print(f"Plot {i} tick snap settings:")
for axis_name, axis in [('X-axis', ax.get_xaxis()), ('Y-axis', ax.get_yaxis())]:
if hasattr(axis, 'get_major_ticks'):
print(f" {axis_name}:")
for j, tick in enumerate(axis.get_major_ticks()):
print(f" Tick {j}: {tick.get_snap()}")
else:
print(f" {axis_name}: No ticks available")
plt.tight_layout()
plt.show()
Output:
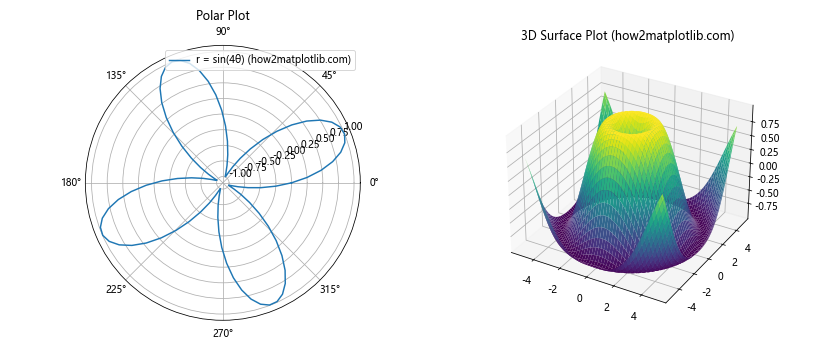
This example shows how to apply Matplotlib.axis.Tick.get_snap() in Python to both a polar plot and a 3D surface plot.
Conclusion
In this comprehensive guide, we've explored the various aspects of Matplotlib.axis.Tick.get_snap() in Python, from basic usage to advanced techniques and integration with other Matplotlib features. By mastering this method, you can create more visually appealing and precise plots, especially when working on publication-quality figures or complex visualizations.