Comprehensive Guide to Matplotlib.axis.Axis.properties() Function in Python
Matplotlib.axis.Axis.properties() function in Python is a powerful tool for customizing and retrieving axis properties in Matplotlib plots. This function is an essential part of the Matplotlib library, which is widely used for creating static, animated, and interactive visualizations in Python. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.properties() function in depth, covering its usage, parameters, and various applications in data visualization.
Understanding the Basics of Matplotlib.axis.Axis.properties()
The Matplotlib.axis.Axis.properties() function is a method of the Axis class in Matplotlib. It allows users to get or set properties of an axis object. This function is particularly useful when you need to fine-tune the appearance of your plots or retrieve information about the current axis settings.
Let’s start with a simple example to demonstrate how to use the Matplotlib.axis.Axis.properties() function:
import matplotlib.pyplot as plt
# Create a simple plot
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Get the properties of the x-axis
x_axis_properties = ax.xaxis.properties()
# Print the properties
print("X-axis properties:", x_axis_properties)
plt.title("How to use Matplotlib.axis.Axis.properties() - how2matplotlib.com")
plt.show()
Output:
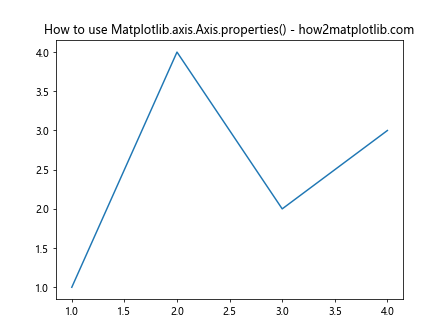
In this example, we create a simple line plot and then use the Matplotlib.axis.Axis.properties() function to retrieve the properties of the x-axis. The function returns a dictionary containing all the properties of the axis object.
Exploring the Return Value of Matplotlib.axis.Axis.properties()
The Matplotlib.axis.Axis.properties() function returns a dictionary containing various properties of the axis object. These properties include:
- Label
- Scale
- Limits
- Ticks
- Tick labels
- Grid lines
- Axis line
- Axis label
Let’s examine each of these properties in detail and see how we can modify them using the Matplotlib.axis.Axis.properties() function.
Label Property
The label property represents the text label for the axis. You can retrieve or set this property using the Matplotlib.axis.Axis.properties() function.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Get the current x-axis label
x_label = ax.xaxis.properties()['label']
print("Current x-axis label:", x_label)
# Set a new x-axis label
ax.xaxis.properties()['label'] = 'X-axis (how2matplotlib.com)'
plt.title("Matplotlib.axis.Axis.properties() - Label Example")
plt.show()
Output:
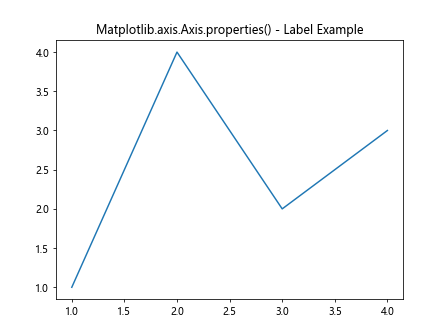
In this example, we first retrieve the current x-axis label using the Matplotlib.axis.Axis.properties() function. Then, we set a new label for the x-axis using the same function.
Scale Property
The scale property determines the scaling of the axis (e.g., linear, logarithmic). You can modify this property using the Matplotlib.axis.Axis.properties() function.
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.logspace(0, 2, 100)
ax.plot(x, x**2)
# Get the current x-axis scale
x_scale = ax.xaxis.properties()['scale']
print("Current x-axis scale:", x_scale)
# Set a logarithmic scale for the x-axis
ax.xaxis.properties()['scale'] = 'log'
plt.title("Matplotlib.axis.Axis.properties() - Scale Example (how2matplotlib.com)")
plt.show()
Output:
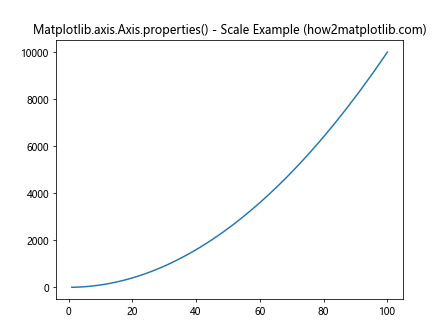
This example demonstrates how to retrieve the current scale of the x-axis and then set it to a logarithmic scale using the Matplotlib.axis.Axis.properties() function.
Tick Labels Property
The tick labels property controls the text labels associated with tick marks on the axis. You can customize these labels using the Matplotlib.axis.Axis.properties() function.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Get the current x-axis tick labels
x_tick_labels = ax.xaxis.properties()['ticklabels']
print("Current x-axis tick labels:", x_tick_labels)
# Set new x-axis tick labels
ax.xaxis.properties()['ticklabels'] = ['A', 'B', 'C', 'D']
plt.title("Matplotlib.axis.Axis.properties() - Tick Labels Example (how2matplotlib.com)")
plt.show()
Output:
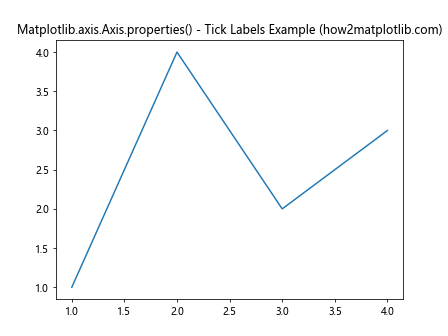
In this example, we retrieve the current x-axis tick labels and then set new custom labels using the Matplotlib.axis.Axis.properties() function.
Grid Lines Property
The grid lines property controls the appearance of grid lines on the plot. You can modify this property using the Matplotlib.axis.Axis.properties() function.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Get the current x-axis grid lines properties
x_grid_lines = ax.xaxis.properties()['grid']
print("Current x-axis grid lines properties:", x_grid_lines)
# Enable grid lines and set their properties
ax.xaxis.properties()['grid'] = {'visible': True, 'color': 'red', 'linestyle': '--'}
plt.title("Matplotlib.axis.Axis.properties() - Grid Lines Example (how2matplotlib.com)")
plt.show()
This example demonstrates how to retrieve the current grid lines properties and then enable and customize the grid lines using the Matplotlib.axis.Axis.properties() function.
Axis Line Property
The axis line property controls the appearance of the axis line itself. You can modify this property using the Matplotlib.axis.Axis.properties() function.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Get the current x-axis line properties
x_axis_line = ax.xaxis.properties()['axisline']
print("Current x-axis line properties:", x_axis_line)
# Customize the x-axis line
ax.xaxis.properties()['axisline'] = {'visible': True, 'color': 'blue', 'linewidth': 2}
plt.title("Matplotlib.axis.Axis.properties() - Axis Line Example (how2matplotlib.com)")
plt.show()
In this example, we retrieve the current x-axis line properties and then customize the appearance of the axis line using the Matplotlib.axis.Axis.properties() function.
Axis Label Property
The axis label property controls the appearance of the axis label. You can modify this property using the Matplotlib.axis.Axis.properties() function.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Get the current x-axis label properties
x_axis_label = ax.xaxis.properties()['label']
print("Current x-axis label properties:", x_axis_label)
# Customize the x-axis label
ax.xaxis.properties()['label'] = {'text': 'X-axis', 'fontsize': 14, 'color': 'green'}
plt.title("Matplotlib.axis.Axis.properties() - Axis Label Example (how2matplotlib.com)")
plt.show()
Output:
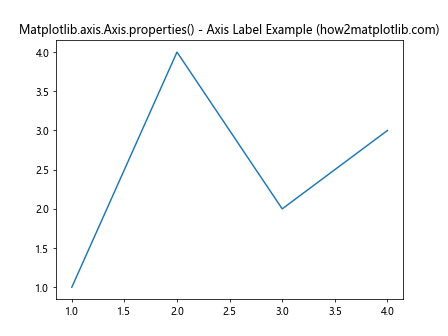
This example shows how to retrieve the current x-axis label properties and then customize the label using the Matplotlib.axis.Axis.properties() function.
Advanced Usage of Matplotlib.axis.Axis.properties()
Now that we’ve covered the basic properties that can be accessed and modified using the Matplotlib.axis.Axis.properties() function, let’s explore some advanced usage scenarios.
Combining Multiple Property Changes
You can use the Matplotlib.axis.Axis.properties() function to modify multiple properties at once, which can be useful for creating complex visualizations efficiently.
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
# Modify multiple properties at once
ax.xaxis.properties().update({
'label': {'text': 'X-axis (how2matplotlib.com)', 'fontsize': 14},
'lim': (0, 12),
'ticks': np.arange(0, 13, 2),
'grid': {'visible': True, 'color': 'gray', 'linestyle': ':'},
'scale': 'linear'
})
plt.title("Matplotlib.axis.Axis.properties() - Multiple Properties Example")
plt.show()
Output:
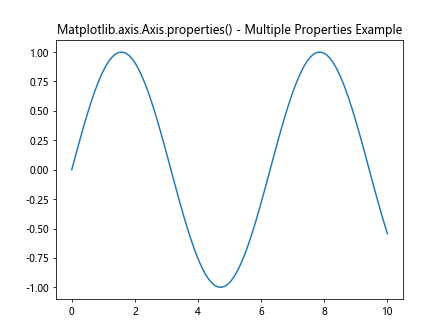
In this example, we use the update()
method to modify multiple properties of the x-axis at once, including the label, limits, ticks, grid, and scale.
Applying Properties to Both Axes
You can use the Matplotlib.axis.Axis.properties() function to apply similar properties to both the x-axis and y-axis simultaneously.
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
# Define common properties
common_properties = {
'label': {'fontsize': 12, 'color': 'blue'},
'grid': {'visible': True, 'color': 'gray', 'linestyle': '--'},
'ticks': {'color': 'red'}
}
# Apply properties to both axes
ax.xaxis.properties().update(common_properties)
ax.yaxis.properties().update(common_properties)
# Set specific labels for each axis
ax.xaxis.properties()['label']['text'] = 'X-axis (how2matplotlib.com)'
ax.yaxis.properties()['label']['text'] = 'Y-axis (how2matplotlib.com)'
plt.title("Matplotlib.axis.Axis.properties() - Both Axes Example")
plt.show()
This example demonstrates how to apply common properties to both the x-axis and y-axis using the Matplotlib.axis.Axis.properties() function, while still allowing for axis-specific customizations.
Creating Custom Tick Formatters
The Matplotlib.axis.Axis.properties() function can be used in conjunction with custom tick formatters to create more advanced axis labels.
import matplotlib.pyplot as plt
import numpy as np
def custom_formatter(x, pos):
return f"{x:.2f} units"
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x))
# Set custom tick formatter
ax.xaxis.properties()['major_formatter'] = plt.FuncFormatter(custom_formatter)
plt.title("Matplotlib.axis.Axis.properties() - Custom Tick Formatter Example (how2matplotlib.com)")
plt.show()
Output:
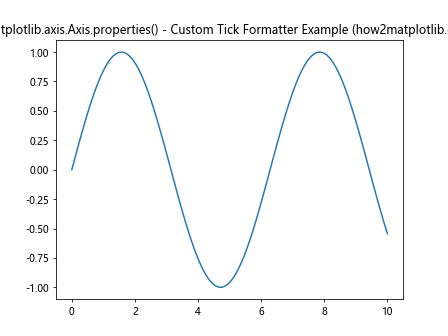
In this example, we define a custom tick formatter function and apply it to the x-axis using the Matplotlib.axis.Axis.properties() function.
Animating Axis Properties
The Matplotlib.axis.Axis.properties() function can be used in animations to create dynamic visualizations with changing axis properties.
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
line, = ax.plot(x, np.sin(x))
def update(frame):
ax.xaxis.properties()['lim'] = (frame, frame + 5)
return line,
ani = FuncAnimation(fig, update, frames=np.linspace(0, 5, 50), blit=True)
plt.title("Matplotlib.axis.Axis.properties() - Animation Example (how2matplotlib.com)")
plt.show()
Output:
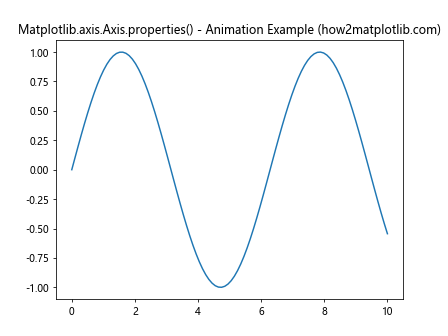
This example demonstrates how to use the Matplotlib.axis.Axis.properties() function in an animation to create a moving window effect by updating the x-axis limits in each frame.
Best Practices for Using Matplotlib.axis.Axis.properties()
When working with the Matplotlib.axis.Axis.properties() function, it’s important to follow some best practices to ensure your code is efficient, readable, and maintainable.
- Use property dictionaries: Instead of setting individual properties one by one, create a dictionary of properties and update them all at once using the
update()
method.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
x_axis_properties = {
'label': {'text': 'X-axis (how2matplotlib.com)', 'fontsize': 14},
'lim': (0, 5),
'ticks': [0, 1, 2, 3, 4, 5],
'grid': {'visible': True, 'color': 'gray', 'linestyle': ':'}
}
ax.xaxis.properties().update(x_axis_properties)
plt.title("Matplotlib.axis.Axis.properties() - Best Practices Example")
plt.show()
Output:
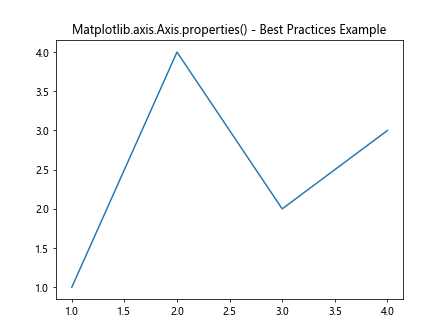
- Use consistent naming conventions: When working with multiple axes or subplots, use consistent naming conventions for your property dictionaries to improve code readability.
Separate style from data: Keep your data processing and plotting logic separate from your styling code. This makes it easier to maintain and update your visualizations.
Document your property changes: Add comments to explain why you’re making specific property changes, especially for complex visualizations or when working in a team.
Use property getters sparingly: While the Matplotlib.axis.Axis.properties() function can be used to retrieve property values, it’s often more efficient to use specific getter methods (e.g.,
get_label()
,get_ticks()
) when you only need a single property value.Be aware of performance: Modifying axis properties can be computationally expensive, especially when done frequently. Try to minimize property changes in performance-critical code, such as animations.
Use context managers: When making temporary changes to axis properties, consider using context managers to ensure the properties are reset after use.
import matplotlib.pyplot as plt
from contextlib import contextmanager
@contextmanager
def temporary_x_axis_properties(ax, **kwargs):
original_properties = ax.xaxis.properties().copy()
ax.xaxis.properties().update(kwargs)
yield
ax.xaxis.properties().update(original_properties)
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
with temporary_x_axis_properties(ax, lim=(0, 5), label={'text': 'Temporary X-axis (how2matplotlib.com)'}):
plt.title("Matplotlib.axis.Axis.properties() - Context Manager Example")
plt.show()
# The x-axis properties will be reset to their original values after the 'with' block
Output: