Matplotlib Title Font Size
Matplotlib is a popular Python library used for creating high-quality visualizations and graphs. It provides a wide range of options to customize the appearance of plots, including the font size of the title. The title of a plot is essential to convey the main idea or purpose of the visualization to the audience. In this article, we will explore different ways to set the font size of the title in Matplotlib and provide code examples to demonstrate their usage.
Setting the Font Size of the Title
There are multiple approaches to set the font size of the title in Matplotlib. We will discuss each of them in detail.
Method 1: Using title
Function
One of the simplest ways to set the font size of the title is by using the title
function and specifying the fontsize
parameter. This parameter allows us to define the font size in points.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
plt.plot(x, y)
plt.title("Title with Font Size", fontsize=16)
plt.show()
Output:
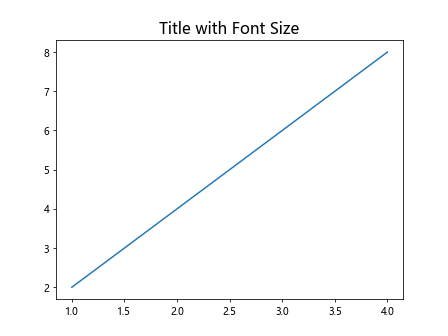
In the above example, we create a simple line plot using plot
function. Then, we set the title using the title
function and specify the fontsize
parameter as 16
. This will set the font size of the title to 16 points.
Method 2: Using set_title
Function
Another way to set the font size of the title is by using the set_title
function on the Axes
object. This method allows us to customize various aspects of the title, including the font size.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
fig, ax = plt.subplots()
ax.plot(x, y)
ax.set_title("Title with Font Size", fontsize=16)
plt.show()
Output:
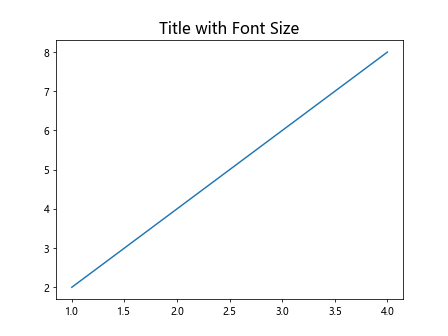
In the above example, we create a figure and an axes using subplots
function. Then, we plot the data on the axes using plot
function. Finally, we set the title using the set_title
function and specify the fontsize
parameter as 16
. This will set the font size of the title to 16 points.
Method 3: Using suptitle
Function
If you want to set the font size of the main title of the figure rather than the title of a specific subplot, you can use the suptitle
function in Matplotlib.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
fig, ax = plt.subplots()
ax.plot(x, y)
plt.suptitle("Main Title with Font Size", fontsize=16)
plt.show()
Output:
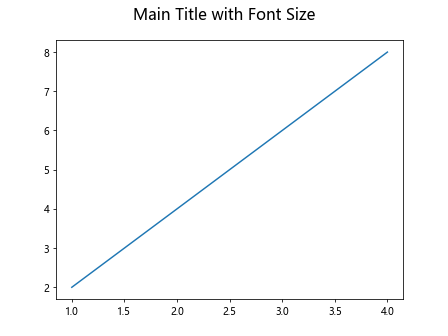
In the above example, we create a figure and an axes using subplots
function. Then, we plot the data on the axes using plot
function. Finally, we set the main title using the suptitle
function and specify the fontsize
parameter as 16
. This will set the font size of the main title to 16 points.
Method 4: Using FontProperties
Object
Matplotlib also allows us to set the font size of the title using a FontProperties
object. This method provides more control over various font-related attributes, including the size.
import matplotlib.pyplot as plt
from matplotlib.font_manager import FontProperties
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
font = FontProperties()
font.set_size(16)
plt.plot(x, y)
plt.title("Title with Font Properties", fontproperties=font)
plt.show()
Output:
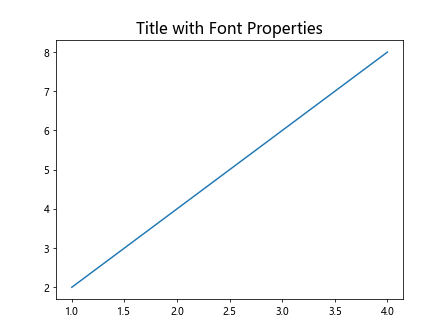
In the above example, we create a simple line plot using plot
function. Then, we set the title using the title
function and specify the fontproperties
parameter as font
, which is a FontProperties
object with a font size of 16
points.
Method 5: Using rcParams
Configuration
Matplotlib provides the rcParams
configuration option, which allows us to set various default settings for our plots. We can use this option to set the font size of the title globally.
import matplotlib.pyplot as plt
import matplotlib as mpl
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
mpl.rcParams['axes.titlesize'] = 16
plt.plot(x, y)
plt.title("Title with rcParams Configuration")
plt.show()
Output:
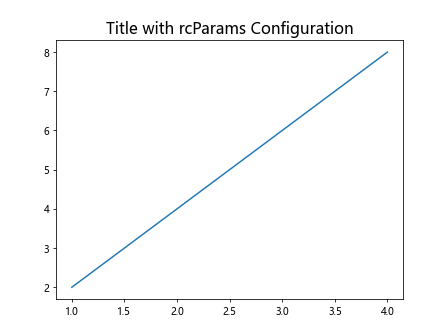
In the above example, we use the rcParams
dictionary from matplotlib
module to set the axes.titlesize
parameter to 16
. This will change the default font size of the title for all the plots in the script.
Method 6: Using title
as Method Chaining
Another way to set the font size of the title is by using method chaining with the title
function. This allows us to set multiple attributes of the title in a single line of code.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
plt.plot(x, y).title("Title with Font Size", fontsize=16)
plt.show()
In the above example, we create a simple line plot using plot
function. Then, we use method chaining with the title
function to set the font size of the title to 16
points.
Method 7: Using pyplot.rc
Configuration
Similar to rcParams
, Matplotlib provides the pyplot.rc
function to configure default settings for the current script. We can use this function to set the font size of the title.
import matplotlib.pyplot as plt
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
plt.rc('axes', titlesize=16)
plt.plot(x, y)
plt.title("Title with pyplot.rc Configuration")
plt.show()
Output:
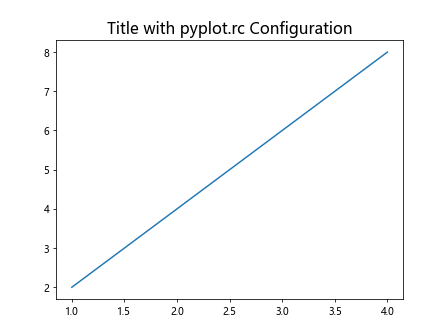
In the above example, we use the pyplot.rc
function to set the titlesize
parameter of the axes
to 16
. This will change the default font size of the title for all the plots in the script.
Method 8: Using FontProperties
as Method Argument
Although we have already discussed using a FontProperties
object to set the font size of the title, it is also possible to directly pass the FontProperties
object as a method argument.
import matplotlib.pyplot as plt
from matplotlib.font_manager import FontProperties
x = [1, 2, 3, 4]
y = [2, 4, 6, 8]
font = FontProperties(size=16)
plt.plot(x, y)
plt.title("Title with Font Properties", fontproperties=font)
plt.show()
Output:
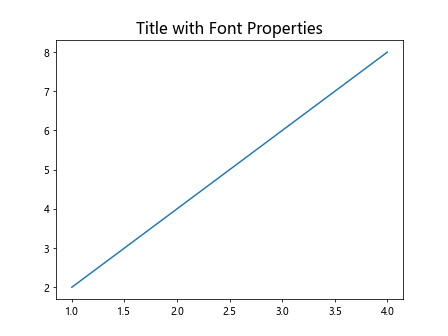
In the above example, we create a simple line plot using plot
function. Then, we set the title using the title
function and pass the font
object as the fontproperties
argument. The font
object is a FontProperties
object with a font size of 16
points.