Introduction to Matplotlib Palette
When it comes to data visualization, having a good color palette is crucial. Matplotlib, one of the most popular libraries for creating graphs and plots in Python, offers a variety of built-in color palettes to choose from. In this article, we will explore how to use these palettes in Matplotlib to create visually appealing plots.
Default Color Palette
Matplotlib has a default color palette that is used when no specific colors are specified. Let’s take a look at an example:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.show()
Output:
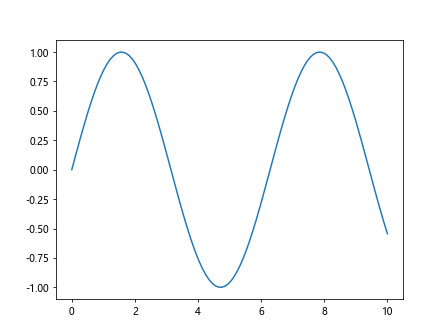
In the example above, Matplotlib uses the default color for the line plot. If you want to change the default color, you can do so by specifying a color using the color
parameter.
Using Built-In Palettes
Matplotlib provides several built-in color palettes that you can use to customize the colors in your plots. Let’s take a look at some examples:
Viridis Palette
The Viridis color palette is a perceptually uniform color map that is widely used for data visualization. You can use it in Matplotlib like this:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.scatter(x, y, c=y, cmap='viridis')
plt.colorbar()
plt.show()
Output:
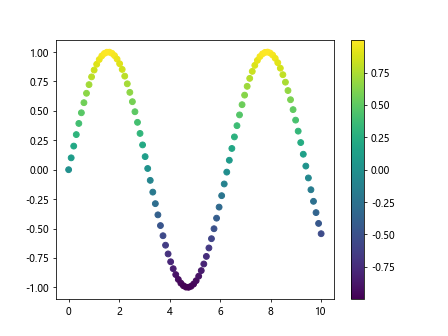
Plasma Palette
The Plasma color palette is another popular choice for data visualization. You can use it in Matplotlib like this:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.scatter(x, y, c=y, cmap='plasma')
plt.colorbar()
plt.show()
Output:
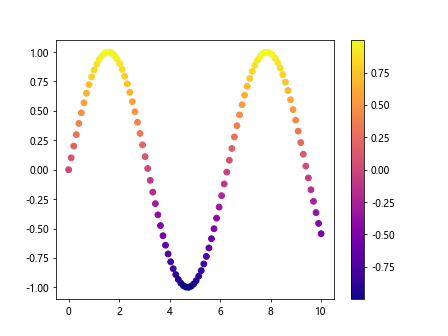
Inferno Palette
The Inferno color palette is known for its high contrast colors, making it suitable for highlighting differences in data. You can use it in Matplotlib like this:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.scatter(x, y, c=y, cmap='inferno')
plt.colorbar()
plt.show()
Output:
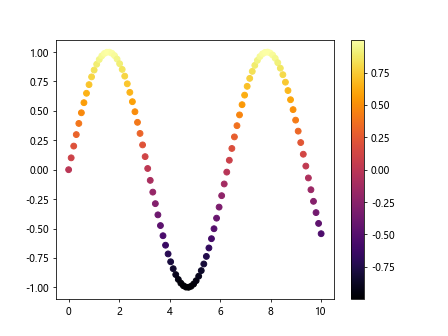
Magma Palette
The Magma color palette is similar to the Inferno palette but with a different color scheme. You can use it in Matplotlib like this:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.scatter(x, y, c=y, cmap='magma')
plt.colorbar()
plt.show()
Output:
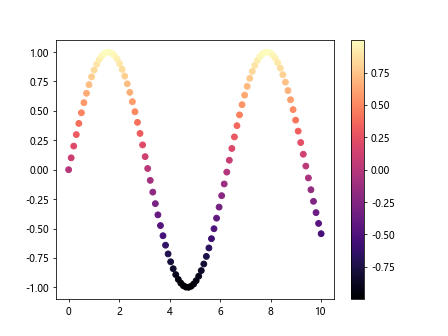
Jet Palette
The Jet color palette is a rainbow color map that was widely used in the past but is now discouraged due to its poor perceptual uniformity. You can use it in Matplotlib like this:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.scatter(x, y, c=y, cmap='jet')
plt.colorbar()
plt.show()
Output:
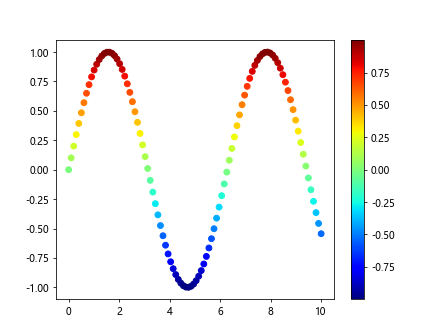
Customizing Palettes
In addition to the built-in color palettes, you can also create custom palettes in Matplotlib. Let’s take a look at an example:
import matplotlib.pyplot as plt
import numpy as np
colors = ['red', 'green', 'blue', 'orange', 'purple']
plt.bar(range(5), range(5), color=colors)
plt.show()
Output:
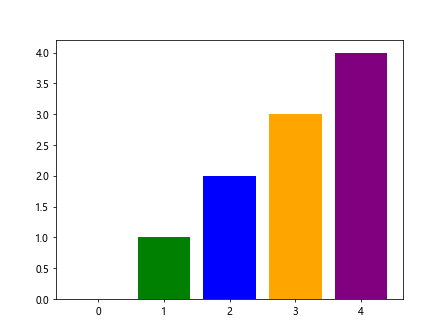
In the example above, we created a custom color palette using a list of color names and applied it to a bar plot.
Conclusion
In this article, we explored how to use color palettes in Matplotlib to create visually appealing plots. We looked at the default color palette, built-in palettes such as Viridis, Plasma, Inferno, Magma, and Jet, as well as how to create custom palettes. By choosing the right color palette for your data visualization, you can effectively convey information and make your plots more engaging. Experiment with different palettes to find the one that works best for your data and audience.