Matplotlib Multiline Title
When creating plots using Matplotlib, it is often useful to provide titles that contain multiple lines of text. This can help to better explain the content of the plot or provide additional information to the viewer. In this article, we will explore how to create multiline titles in Matplotlib.
Example 1: Basic Multiline Title
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Multiline\nTitle\nExample")
plt.show()
Output:
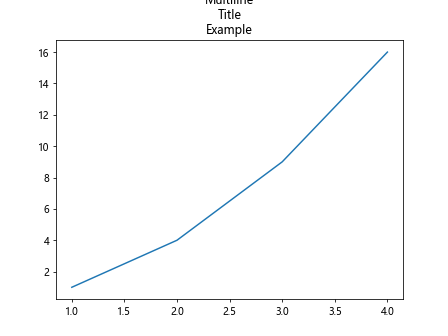
In this example, we create a simple plot and set a multiline title using the \n
character to create line breaks.
Example 2: Adjusting Title Font Properties
import matplotlib.pyplot as plt
from matplotlib.font_manager import FontProperties
font = FontProperties()
font.set_weight('bold')
font.set_size('large')
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Title with\nCustom Font", fontproperties=font)
plt.show()
Output:
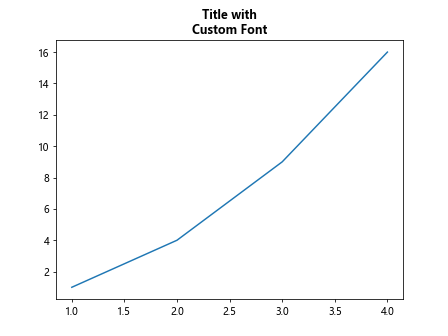
Here, we customize the font properties of the title using the FontProperties
class.
Example 3: Center Aligned Multiline Title
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Multiline\nCentered\nTitle", loc='center')
plt.show()
Output:
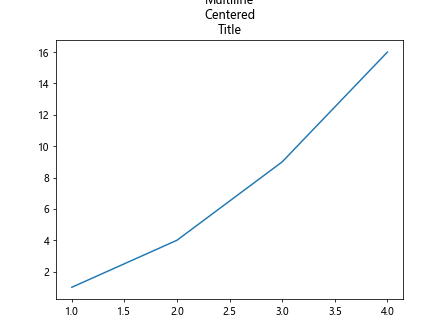
By setting the loc='center'
parameter in the title
function, we can create a center-aligned multiline title.
Example 4: Adding Subtitles to Plots
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Main Title", loc='left')
plt.suptitle("SubTitle Example")
plt.show()
Output:
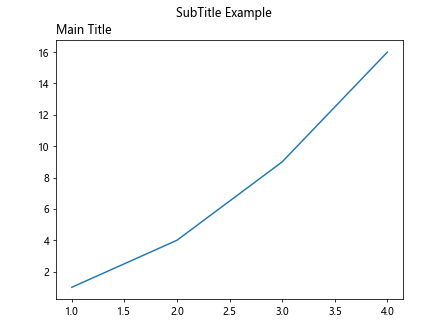
In this example, we add a subtitle to the plot using the suptitle
function.
Example 5: Multiline Title with Different Font Sizes
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Multiline\nTitle", fontsize=12, fontweight='bold')
plt.show()
Output:
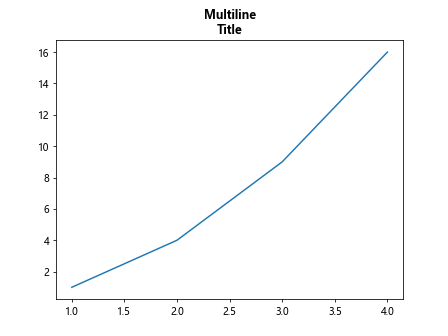
By specifying different font sizes and font weights in the title
function, we can create a multiline title with varied text properties.
Example 6: Multiline Title with Colored Text
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Colorful\nTitle", color='red')
plt.show()
Output:
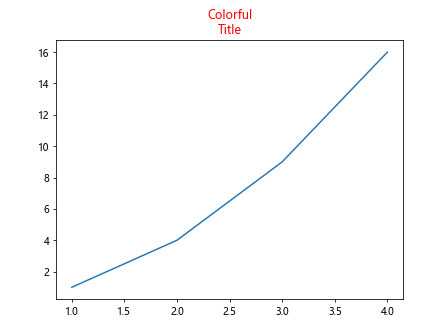
In this example, we set the color of the text in the multiline title to red using the color
parameter.
Example 7: Multiline Title with Background Color
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Title with\nBackground Color", backgroundcolor='lightgrey')
plt.show()
Output:
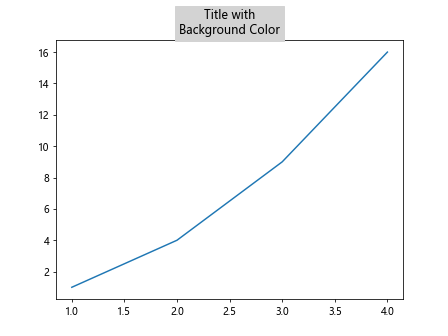
By specifying a backgroundcolor
in the title
function, we can create a multiline title with a colored background.
Example 8: Rotated Multiline Title
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Rotated\nTitle", rotation=45)
plt.show()
Output:
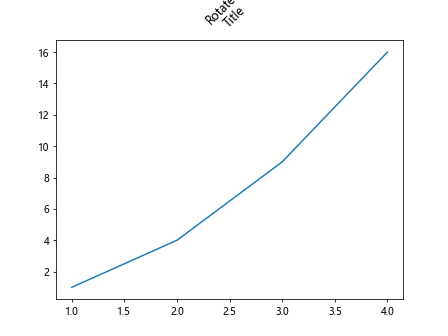
By setting the rotation
parameter in the title
function, we can rotate the multiline title text.
Example 9: Multiline Title with Different Alignments
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Title\nwith\nAlignment", loc='right')
plt.show()
Output:
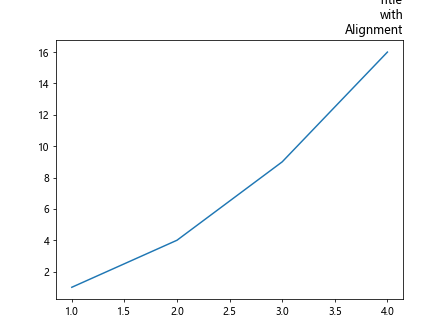
In this example, we set the alignment of the multiline title text to the right using the loc
parameter.
Example 10: Multiline Title with Padding
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title("Title with\nPadding", pad=20)
plt.show()
Output:
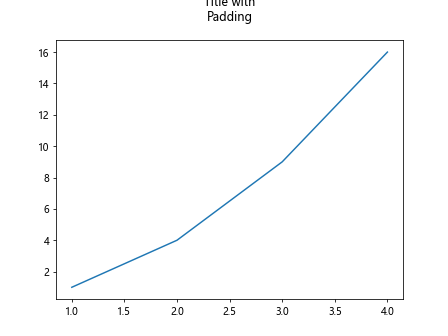
By specifying a padding value in the title
function, we can add space between the multiline title and the plot content.
Conclusion
In this article, we have explored various ways to create multiline titles in Matplotlib plots. By using different parameters and techniques, we can customize the appearance of titles to better convey information and enhance the overall presentation of our plots. Whether it’s adjusting font properties, adding subtitles, or changing alignments, Matplotlib provides a flexible and powerful tool for creating visually appealing plots with informative multiline titles.