Matplotlib: Making Titles Bold
Matplotlib is a popular plotting library in Python that allows you to create various types of plots and graphs. One common customization that users often want to make is to make the titles of their plots bold for better visibility and emphasis. In this article, we will explore how to make titles bold in Matplotlib using different methods and examples.
Method 1: Using Matplotlib’s FontProperties
class
import matplotlib.pyplot as plt
from matplotlib.font_manager import FontProperties
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title('Bold Title Example', fontproperties=FontProperties(weight='bold'))
plt.show()
Output:
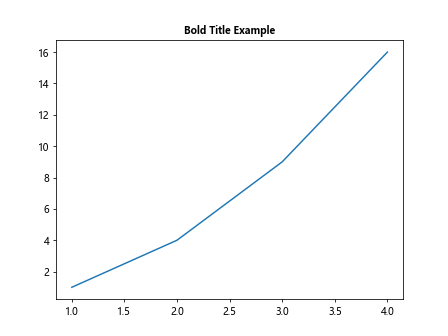
Method 2: Using set_fontweight
method
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title('Bold Title Example')
plt.gca().title.set_fontweight('bold')
plt.show()
Output:
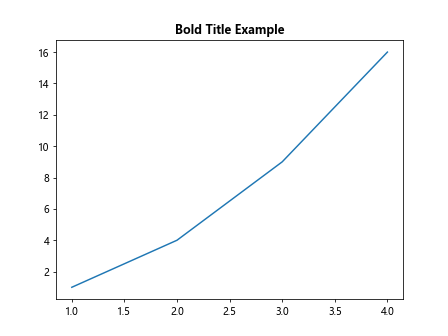
Method 3: Using set_fontweight
method and weight
parameter
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
title_obj = plt.title('Bold Title Example')
plt.setp(title_obj, fontweight='bold')
plt.show()
Output:
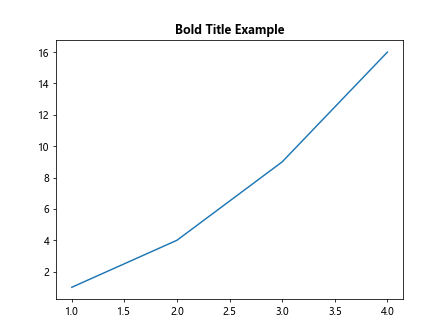
Method 4: Using fontweight
parameter in title
function
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.title('Bold Title Example', fontweight='bold')
plt.show()
Output:
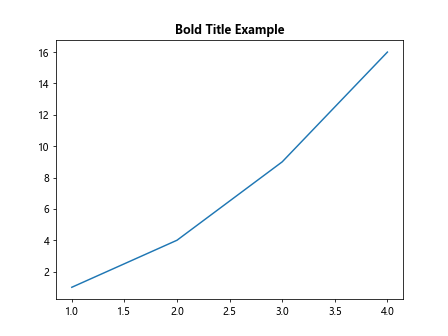
Method 5: Using Matplotlib’s text
function
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.text(2, 18, 'Bold Title Example', weight='bold')
plt.show()
Output:
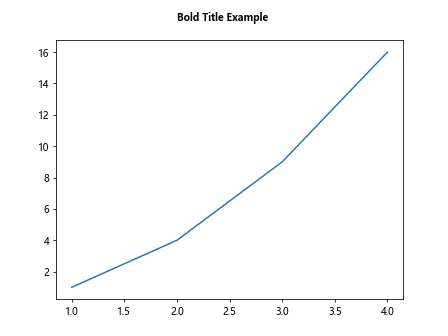
Method 6: Using set_text
method of title object
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
title_obj = plt.title('Bold Title Example')
title_obj.set_text('Bold Title Example')
title_obj.set_fontweight('bold')
plt.show()
Output:
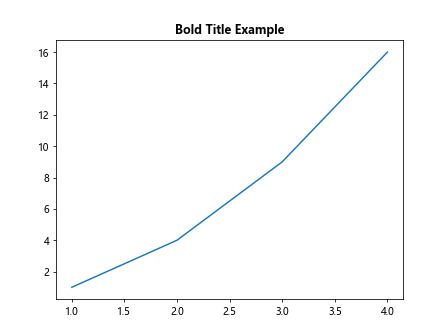
Method 7: Using set_fontweight
method of title object
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
title_obj = plt.title('Bold Title Example')
title_obj.set_fontweight('bold')
plt.show()
Output:
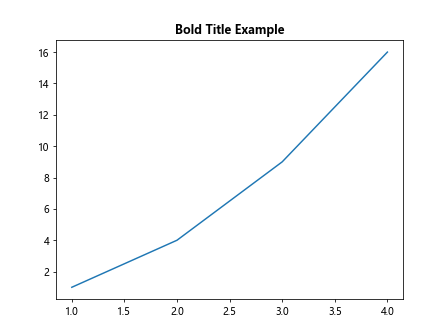
Method 8: Using set_fontweight
method with get_text
function
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
title_obj = plt.title('Bold Title Example')
title_obj.set_fontweight('bold')
title_obj.set_text(title_obj.get_text())
plt.show()
Output:
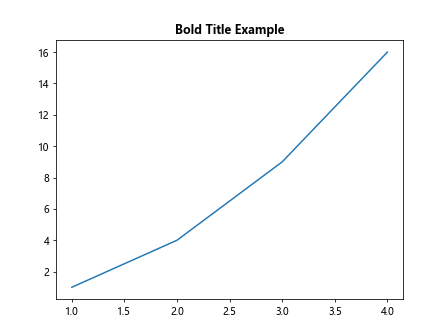
Method 9: Using set_fontweight
method with set_text
function
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
title_obj = plt.title('Bold Title Example')
title_obj.set_fontweight('bold')
title_obj.set_text('Bold Title Example')
plt.show()
Output:
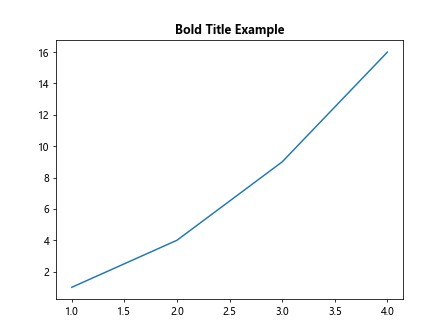
Method 10: Using set_text
method with set_fontweight
function
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
title_obj = plt.title('Bold Title Example')
title_obj.set_text('Bold Title Example')
title_obj.set_fontweight('bold')
plt.show()
Output:
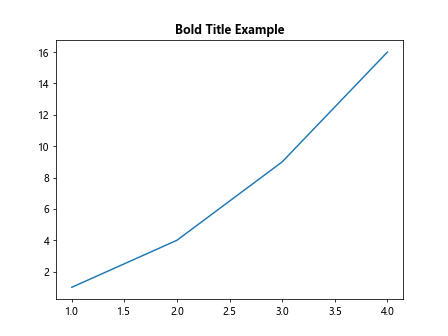
In this article, we have explored various methods to make titles bold in Matplotlib. Whether you use the FontProperties
class, set_fontweight
method, fontweight
parameter, text
function, or a combination of these methods, you can easily customize the appearance of your plot titles to make them stand out. Experiment with these methods to find the best approach for your plotting needs!