Matplotlib Legend Facecolor
Matplotlib is a powerful library for creating static, animated, and interactive visualizations in Python. One commonly used feature in Matplotlib is the legend, which provides information about the plot elements being displayed. In this article, we will explore how to customize the facecolor of a legend in Matplotlib.
Basic Legend Facecolor
You can set the facecolor of a legend using the facecolor
parameter. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
plt.legend(facecolor='lightblue')
plt.show()
Output:
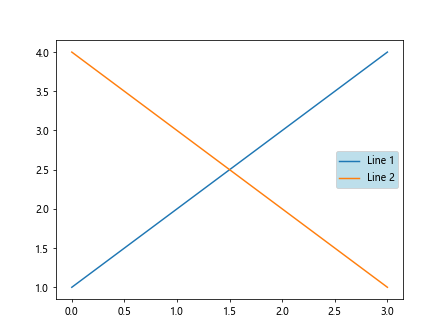
In the above code, the facecolor of the legend is set to ‘lightblue’. You can customize the facecolor by providing different color values such as ‘red’, ‘blue’, ‘#FFA500’, etc.
Legend Facecolor with Transparency
You can also set the transparency level of the legend facecolor using the alpha
parameter. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
plt.legend(facecolor='lightgreen', alpha=0.5)
plt.show()
In the above code, the facecolor of the legend is set to ‘lightgreen’ with 50% transparency.
Custom Legend with Rectangular Frame
You can create a custom legend with a rectangular frame and set the facecolor of the frame using the frame
and framealpha
parameters. Here is an example code snippet:
import matplotlib.pyplot as plt
line1, = plt.plot([1, 2, 3, 4], label='Line 1')
line2, = plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend([line1, line2], ['Line 1', 'Line 2'], facecolor='lightyellow', frame=True, framealpha=0.8)
plt.show()
In the above code, we create a custom legend with a rectangular frame that has a facecolor of ‘lightyellow’ and 80% transparency.
Legend Facecolor with Shadow
You can add a shadow to the legend box and customize the shadow properties such as color, offset, and transparency. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(facecolor='lightcoral', shadow=True, shadowcolor='gray', shadowoffset=(2, -2), shadowalpha=0.5)
plt.show()
In the above code, we add a shadow to the legend box with a shadow color of ‘gray’, offset of (2, -2), and 50% transparency.
Multiple Legends with Different Facecolors
You can create multiple legends with different facecolors in the same plot. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend1 = plt.legend(['Line 1'], facecolor='lightblue', loc='lower right')
legend2 = plt.legend(['Line 2'], facecolor='lightgreen', loc='upper left')
plt.gca().add_artist(legend1)
plt.show()
Output:
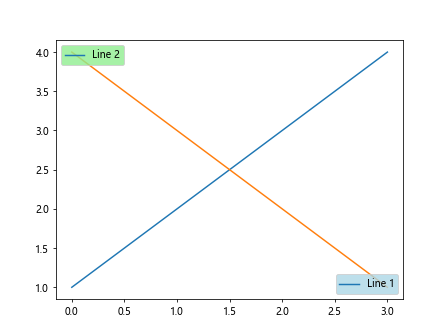
In the above code, we create two separate legends for ‘Line 1’ and ‘Line 2’ with different facecolors and positions in the plot.
Legend Facecolor with Border
You can add a border to the legend box and customize the border properties such as color, width, and transparency. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(facecolor='lightyellow', edgecolor='black', linewidth=2)
plt.show()
In the above code, we add a black border with a width of 2 to the legend box.
Legend Facecolor with Title
You can add a title to the legend box and customize the title properties such as color, font size, and position. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(facecolor='lightblue', title='Legend Title', title_fontsize='large', title_color='red', title_loc='left')
plt.show()
In the above code, we add a title to the legend box with a red color, large font size, and positioned to the left.
Custom Legend Label Facecolor
You can customize the facecolor of individual legend labels using the labelcolor
parameter. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(facecolor='lightgreen', labelcolor='purple')
plt.show()
Output:
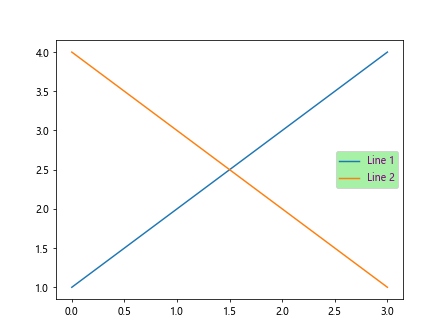
In the above code, we set the facecolor of the legend to ‘lightgreen’ and the color of the legend labels to ‘purple’.
Legend Facecolor with Horizontal Alignment
You can align the legend box horizontally with respect to the plot using the ncol
parameter. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(facecolor='lightyellow', ncol=2)
plt.show()
Output:
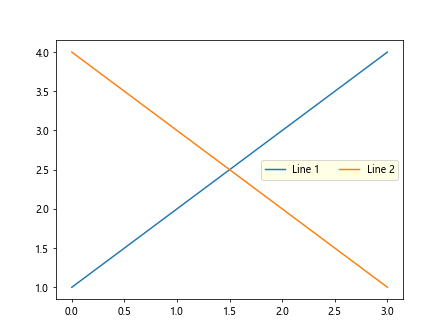
In the above code, we set the facecolor of the legend to ‘lightyellow’ and align the legend box with two columns horizontally.
Legend Facecolor with Custom Marker
You can add a custom marker to the legend box and customize its properties such as color, size, and transparency. Here is an example code snippet:
import matplotlib.pyplot as plt
from matplotlib.patches import Patch
line1, = plt.plot([1, 2, 3, 4], label='Line 1')
line2, = plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(handles=[Patch(facecolor='lightpink', linewidth=2)], labels=['Custom Marker'], loc='upper right')
plt.show()
Output:
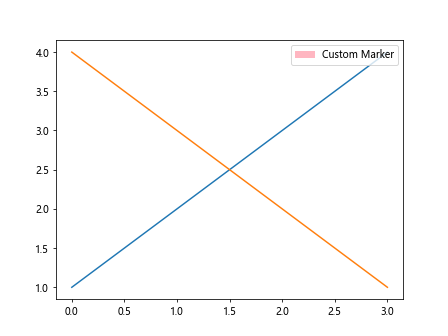
In the above code, we create a custom marker with a facecolor of ‘lightpink’ and a linewidth of 2 to be displayed in the legend box.
Legend Facecolor with Custom Text
You can add custom text to the legend box and customize its properties such as color, font size, and alignment. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(labels=['Custom Text'], prop={'size': 14, 'weight': 'bold', 'color': 'orange'}, loc='upper left')
plt.show()
In the above code, we add custom text with a font size of 14, bold weight, and orange color to the legend box.
Legend Facecolor with Clickable Legend
You can make the legend box clickable by adding an interactive element to it. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
def on_legend_click(event):
print(f'Legend {event.artist} clicked!')
legend = plt.legend(['Line 1', 'Line 2'])
legend.set_picker(True)
plt.gcf().canvas.mpl_connect('pick_event', on_legend_click)
plt.show()
Output:
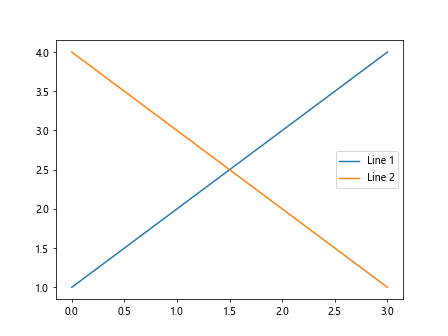
In the above code, we create a simple interactive plot with two lines and a legend that is clickable. Once the legend is clicked, it will print a message indicating which legend has been clicked.
Legend Facecolor with Marker Size
You can change the size of the markers displayed in the legend box using the markerscale
parameter. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1', marker='o')
plt.plot([4, 3, 2, 1], label='Line 2', marker='s')
legend = plt.legend(facecolor='lightblue', markerscale=2)
plt.show()
Output:
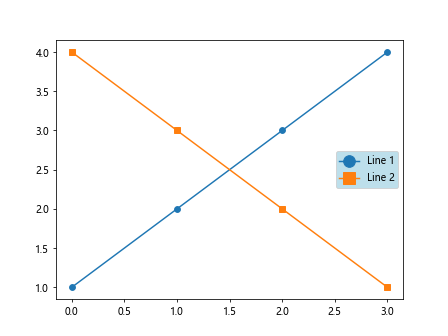
In the above code, we set the markerscale to 2, which doubles the size of the markers displayed in the legend box.
Legend Facecolor with Horizontal Spacing
You can adjust the horizontal spacing between legend labels using the columnspacing
parameter. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1', color='red')
plt.plot([4, 3, 2, 1], label='Line 2', color='blue')
legend = plt.legend(facecolor='lightgreen', ncol=2, columnspacing=0.5)
plt.show()
Output:
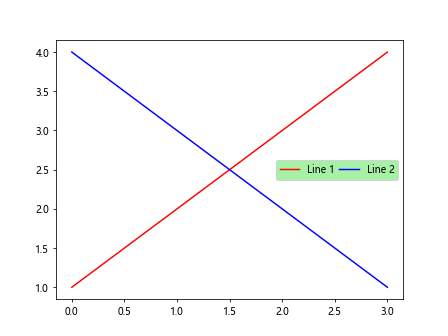
In the above code, we set the horizontal spacing between legend labels to 0.5, creating a more compact layout in the legend box.
Legend Facecolor with Title Position
You can specify the position of the legend title in the legend box using the title_loc
parameter. Here is an example code snippet:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], label='Line 1')
plt.plot([4, 3, 2, 1], label='Line 2')
legend = plt.legend(facecolor='lightblue', title='Legend Title', title_loc='right')
plt.show()
In the above code, we set the position of the legend title to be on the right side of the legend box.
Conclusion
In this article, we have explored various techniques for customizing the facecolor of legends in Matplotlib. By utilizing these techniques, you can create visually appealing and informative plots with customized legend boxes in your Python visualizations. Experiment with different facecolors, transparency levels, shadows, borders, titles, and other customizable options to enhance the presentation of your plots.
Remember that the examples provided in this article are just a starting point, and you can further customize the legends based on your specific requirements and design preferences. Matplotlib offers a wide range of options for customizing legends, allowing you to create unique and dynamic visualizations for your data analysis projects.