Matplotlib imshow Grayscale
Matplotlib is a powerful library in Python for creating visualizations. One of the commonly used functions in Matplotlib is imshow()
, which allows us to display images. This article focuses on using imshow()
to display grayscale images.
Grayscale images are images that contain shades of gray ranging from black to white but lack any color information. Each pixel in a grayscale image is represented by a single value between 0 and 255, where 0 represents black and 255 represents white. These values determine the intensity or brightness of each pixel.
Setting up Matplotlib
Before we start exploring imshow()
for grayscale images, let’s set up Matplotlib by importing the necessary libraries and configuring the inline backend for Jupyter notebooks:
import numpy as np
import matplotlib.pyplot as plt
%matplotlib inline
Example 1: Displaying a Grayscale Image
In this example, we will display a grayscale image using imshow()
.
# Create a grayscale image
image = np.random.randint(0, 256, size=(100, 100))
# Display the grayscale image
plt.imshow(image, cmap='gray')
plt.axis('off')
plt.show()
Output:
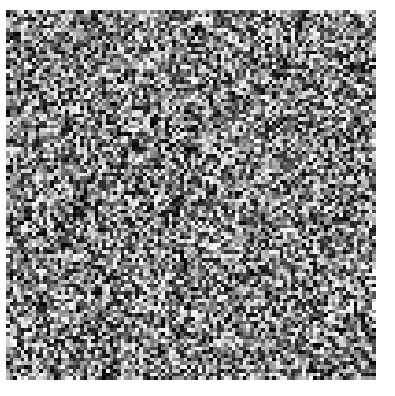
In the above example, we first create a random grayscale image using numpy
. The image
variable represents the pixel values of the grayscale image. We then use imshow()
to display the image. The cmap='gray'
argument specifies that we want to display the image in grayscale. We also turn off the axis labels using plt.axis('off')
. Finally, we call plt.show()
to display the image.
Example 2: Changing Color Map
By default, imshow()
uses the colormap viridis
to display images. However, we can change the colormap to customize the appearance of the image.
# Create a grayscale image
image = np.random.randint(0, 256, size=(100, 100))
# Display the grayscale image with a different colormap
plt.imshow(image, cmap='inferno')
plt.axis('off')
plt.show()
Output:
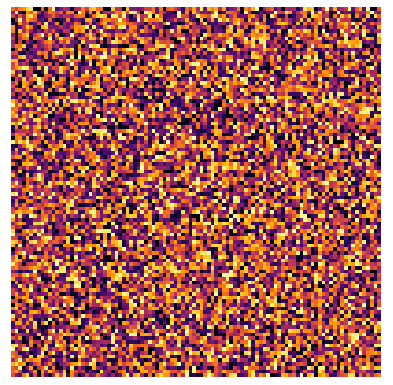
In the above example, we use the cmap='inferno'
argument in imshow()
to change the colormap. Here, we use the inferno
colormap, which provides a different color scheme for the grayscale image.
Example 3: Rescaling Pixel Values
Sometimes, the pixel values of grayscale images are not scaled properly, leading to incorrect display results. In such cases, we need to rescale the pixel values to ensure proper visualization.
# Create a grayscale image
image = np.random.randint(100, 200, size=(100, 100))
# Rescale the pixel values to the range [0, 255]
rescaled_image = ((image - np.min(image)) / (np.max(image) - np.min(image))) * 255
# Display the rescaled grayscale image
plt.imshow(rescaled_image, cmap='gray')
plt.axis('off')
plt.show()
Output:
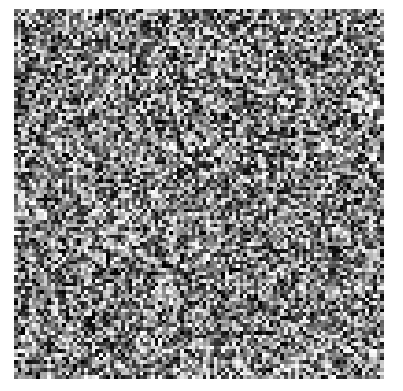
In the above example, we create a grayscale image with pixel values ranging from 100 to 200. The rescaled_image
variable represents the rescaled pixel values, ensuring that they are in the range [0, 255]. Finally, we use imshow()
to display the rescaled grayscale image.
Example 4: Adjusting Brightness/Contrast
We can adjust the brightness and contrast of grayscale images using the imshow()
function.
# Create a grayscale image
image = np.random.randint(0, 256, size=(100, 100))
# Adjust the brightness and contrast of the grayscale image
adjusted_image = image * 1.5 + 50
# Display the adjusted grayscale image
plt.imshow(adjusted_image, cmap='gray')
plt.axis('off')
plt.show()
Output:
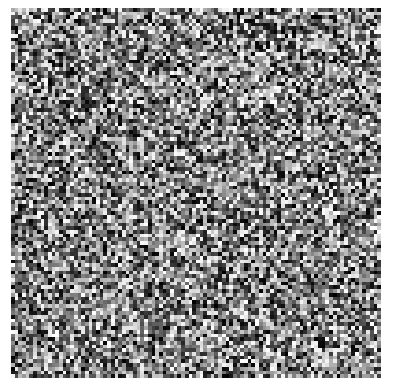
In the above example, we multiply each pixel value of the grayscale image by 1.5 and then add 50 to adjust the brightness and contrast. The resulting image, adjusted_image
, is then displayed using imshow()
.
Example 5: Showing Colorbar
We can add a colorbar to the grayscale image to provide a reference for interpreting the intensity values.
# Create a grayscale image
image = np.random.randint(0, 256, size=(100, 100))
# Display the grayscale image with a colorbar
plt.imshow(image, cmap='gray')
plt.axis('off')
plt.colorbar()
plt.show()
Output:
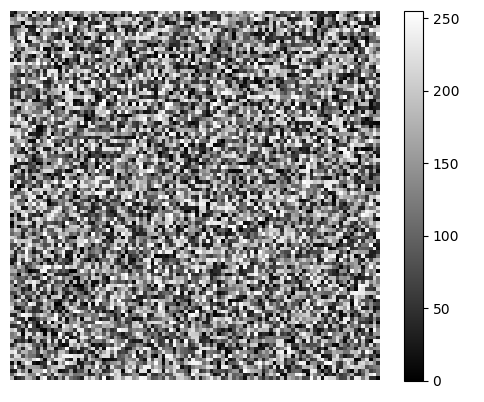
In the above example, after displaying the grayscale image using imshow()
, we add a colorbar to it using plt.colorbar()
. The colorbar shows a range of intensity values from 0 to 255, enabling us to interpret the pixel intensity in the image.
Example 6: Displaying Multiple Grayscale Images
We can display multiple grayscale images in a single plot using imshow()
.
# Create grayscale images
image1 = np.random.randint(0, 256, size=(100, 100))
image2 = np.random.randint(0, 256, size=(100, 100))
# Display multiple grayscale images
fig, ax = plt.subplots(1, 2)
ax[0].imshow(image1, cmap='gray')
ax[0].axis('off')
ax[1].imshow(image2, cmap='gray')
ax[1].axis('off')
plt.show()
Output:
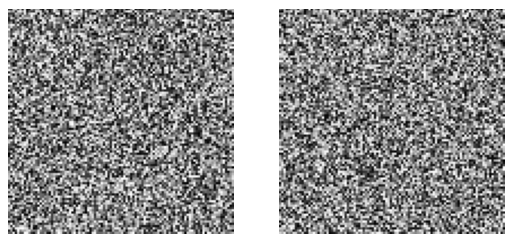
In the above example, we create two grayscale images, image1
and image2
. We then use plt.subplots()
to create a subplot with two axes, ax[0]
and ax[1]
. Finally, we use imshow()
to display each grayscale image in its respective axis.
Example 7: Adding Titles and Labels
We can add titles and labels to the grayscale image plot to provide additional information.
# Create a grayscale image
image = np.random.randint(0, 256, size=(100, 100))
# Display the grayscale image with a title and labels
plt.imshow(image, cmap='gray')
plt.axis('off')
plt.title('Grayscale Image')
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
Output:
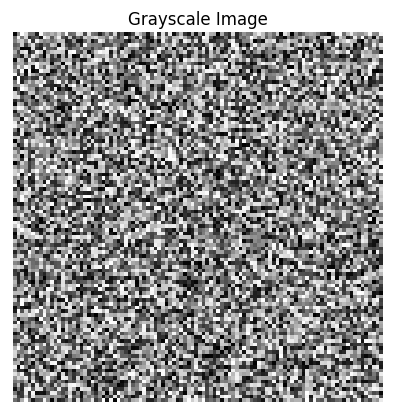
In the above example, we add a title to the plot using plt.title()
, and the labels for the x and y axes using plt.xlabel()
and plt.ylabel()
, respectively.
Example 8: Saving the Grayscale Image
We can save the grayscale image plot to a file using plt.savefig()
.
# Create a grayscale image
image = np.random.randint(0, 256, size=(100, 100))
# Display the grayscale image and save it to a file
plt.imshow(image, cmap='gray')
plt.axis('off')
plt.savefig('grayscale_image.png')
plt.show()
Output:
A file named grayscale_image.png
is saved in the current directory.
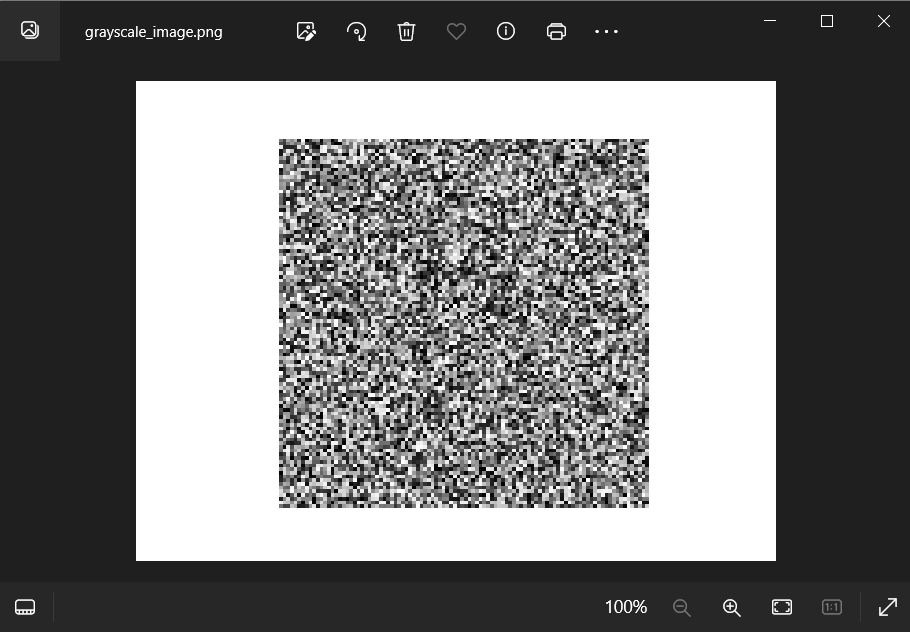
In the above example, after displaying the image using imshow()
, we use plt.savefig()
to save the image to a file called grayscale_image.png
. The file is saved in the current working directory.
Example 9: Zooming in the Grayscale Image
We can zoom in on specific regions of the grayscale image using imshow()
.
# Create a grayscale image
image = np.random.randint(0, 256, size=(100, 100))
# Zoom in on a specific region of the grayscale image
zoomed_image = image[30:50, 40:60]
# Display the zoomed-in grayscale image
plt.imshow(zoomed_image, cmap='gray')
plt.axis('off')
plt.show()
Output:
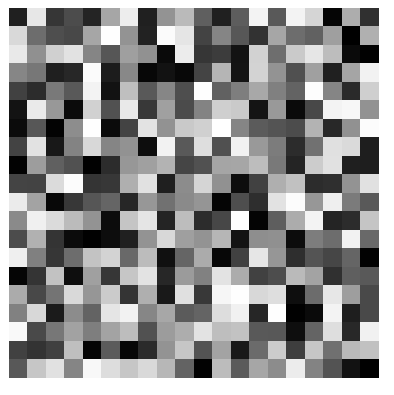
In the above example, we create a grayscale image and then extract a specific region from it using slicing. The resulting zoomed-in image, zoomed_image
, is then displayed using imshow()
.
Example 10: Overlaying Grayscale Image on a Background
We can overlay a grayscale image on a background image or another plot using imshow()
.
# Create a grayscale image
grayscale_image = np.random.randint(0, 256, size=(100, 100))
# Create a background image
background_image = np.zeros((100, 100, 3), dtype=np.uint8) # Black background image
# Overlay the grayscale image on the background image
background_image[..., 0] = grayscale_image # Assign grayscale image to the blue channel
# Display the overlayed image
plt.imshow(background_image)
plt.axis('off')
plt.show()
Output:
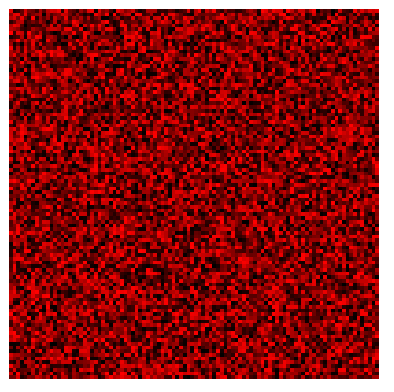
In the above example, we first create a grayscale image and a background image. The background image is initialized as a black image with dimensions (100, 100, 3), where the third dimension represents the RGB channels. We then overlay the grayscale image on the background image by assigning the grayscale image to the blue channel (background_image[..., 0]
). Finally, we display the overlayed image using imshow()
.
As demonstrated in the above examples, imshow()
in Matplotlib is a versatile tool that allows us to visualize grayscale images with customizable options. Whether adjusting brightness/contrast, adding colorbars, or overlaying images, imshow()
provides the necessary flexibility to create informative and visually appealing grayscale image visualizations.
The provided code examples give a glimpse into the capabilities of imshow()
for grayscale images. By modifying the parameters and exploring further, users can unlock a wide range of image visualization possibilities using this powerful Matplotlib function.