How to Use Matplotlib Cursor Widget
Matplotlib Cursor Widget is a powerful tool for interactive data exploration in Matplotlib, one of the most popular plotting libraries in Python. This article will provide an in-depth look at how to use the Matplotlib Cursor Widget effectively in your data visualization projects. We’ll cover everything from basic usage to advanced techniques, with plenty of examples along the way.
Introduction to Matplotlib Cursor Widget
The Matplotlib Cursor Widget is a feature that allows users to interact with plots by displaying cursor coordinates and other information as they move the mouse over the plot. This widget is particularly useful for precise data reading and analysis, making it an essential tool for many data scientists and researchers.
Let’s start with a simple example of how to implement a basic Matplotlib Cursor Widget:
import matplotlib.pyplot as plt
from matplotlib.widgets import Cursor
fig, ax = plt.subplots(figsize=(8, 6))
ax.set_title("Basic Matplotlib Cursor Widget - how2matplotlib.com")
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
cursor = Cursor(ax, useblit=True, color='red', linewidth=1)
plt.show()
Output:
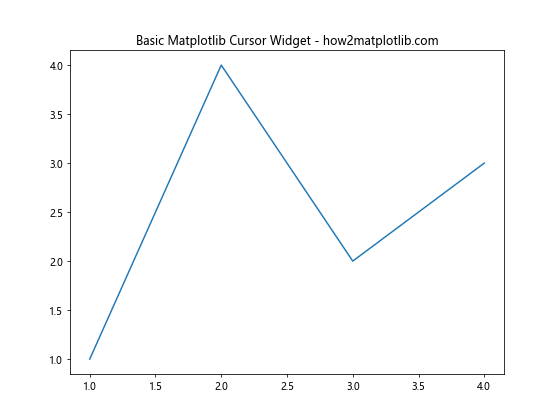
In this example, we create a simple line plot and add a Cursor widget to it. The Cursor is initialized with the axis object (ax), and we set useblit=True
for better performance. The color and linewidth of the cursor can be customized as shown.
Understanding Cursor Parameters
The Matplotlib Cursor Widget comes with several parameters that allow you to customize its behavior and appearance. Let’s explore some of these parameters:
useblit
: This boolean parameter determines whether to use blitting for drawing the cursor. Blitting can significantly improve performance, especially for complex plots.horizOn
andvertOn
: These boolean parameters control whether to draw the horizontal and vertical lines of the cursor, respectively.color
: Sets the color of the cursor lines.linewidth
: Determines the width of the cursor lines.linestyle
: Sets the style of the cursor lines (e.g., solid, dashed, dotted).
Here’s an example demonstrating the use of these parameters: