How to Master Matplotlib Cmap Colors: A Comprehensive Guide
Matplotlib cmap colors are an essential aspect of data visualization in Python. This comprehensive guide will explore the various ways to use colormaps (cmaps) and colors in Matplotlib, providing detailed explanations and practical examples. Whether you’re a beginner or an experienced data scientist, this article will help you enhance your visualizations using Matplotlib cmap colors.
Understanding Matplotlib Cmap Colors
Matplotlib cmap colors refer to the color mapping functionality provided by the Matplotlib library. Colormaps are used to represent data values as colors in visualizations such as heatmaps, scatter plots, and 3D surfaces. They allow you to map numerical data to a range of colors, making it easier to interpret and analyze complex datasets.
Let’s start with a simple example to demonstrate the use of Matplotlib cmap colors:
import matplotlib.pyplot as plt
import numpy as np
# Generate sample data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a scatter plot with a colormap
plt.scatter(x, y, c=y, cmap='viridis')
plt.colorbar(label='Sin(x)')
plt.title('Scatter plot with Matplotlib cmap colors - how2matplotlib.com')
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
Output:
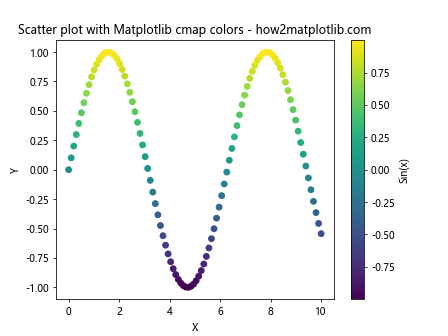
In this example, we create a scatter plot where the color of each point is determined by its y-value. The cmap='viridis'
parameter specifies the colormap to use, and plt.colorbar()
adds a color scale to the plot.
Types of Matplotlib Cmap Colors
Matplotlib offers a wide variety of colormaps to choose from. These can be broadly categorized into sequential, diverging, and qualitative colormaps. Let’s explore each type with examples:
Sequential Colormaps
Sequential colormaps are ideal for representing data that progresses from low to high values. They typically use a gradient of colors that increase in intensity or brightness.
import matplotlib.pyplot as plt
import numpy as np
# Generate sample data
data = np.random.rand(10, 10)
# Create a heatmap with a sequential colormap
plt.imshow(data, cmap='Blues')
plt.colorbar(label='Value')
plt.title('Heatmap with Sequential Colormap - how2matplotlib.com')
plt.show()
Output:
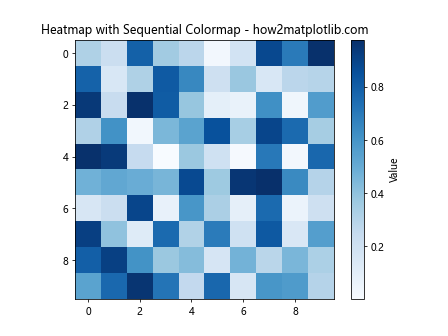
In this example, we use the ‘Blues’ colormap to create a heatmap. The colormap ranges from light blue (low values) to dark blue (high values), making it easy to identify patterns in the data.
Diverging Colormaps
Diverging colormaps are useful for data that has a meaningful midpoint, such as temperature anomalies or correlation coefficients. They typically use two different hues that diverge from a neutral color at the midpoint.
import matplotlib.pyplot as plt
import numpy as np
# Generate sample data
x = np.linspace(-1, 1, 100)
y = np.linspace(-1, 1, 100)
X, Y = np.meshgrid(x, y)
Z = X * Y
# Create a contour plot with a diverging colormap
plt.contourf(X, Y, Z, cmap='RdBu', levels=20)
plt.colorbar(label='X * Y')
plt.title('Contour plot with Diverging Colormap - how2matplotlib.com')
plt.xlabel('X')
plt.ylabel('Y')
plt.show()
Output:
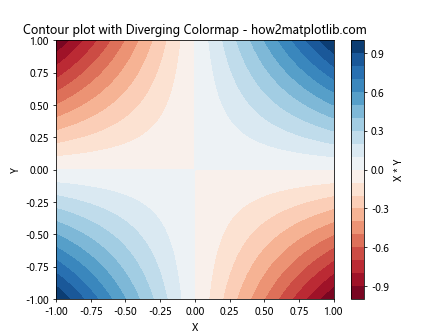
This example uses the ‘RdBu’ (Red-Blue) diverging colormap to visualize the product of X and Y. The colormap transitions from red (negative values) through white (values near zero) to blue (positive values).
Qualitative Colormaps
Qualitative colormaps are designed for categorical data, where colors are used to distinguish between different categories rather than represent numerical values.
import matplotlib.pyplot as plt
import numpy as np
# Generate sample data
categories = ['A', 'B', 'C', 'D', 'E']
values = np.random.rand(5, 5)
# Create a bar plot with a qualitative colormap
plt.bar(categories, values.mean(axis=1), color=plt.cm.Set3(np.linspace(0, 1, 5)))
plt.title('Bar plot with Qualitative Colormap - how2matplotlib.com')
plt.xlabel('Category')
plt.ylabel('Mean Value')
plt.show()
Output:
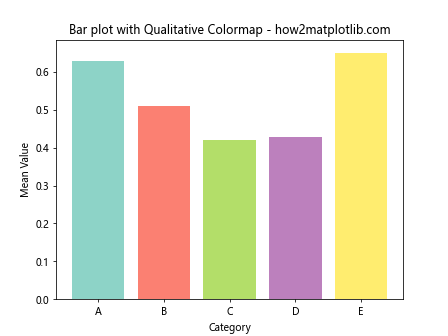
In this example, we use the ‘Set3’ qualitative colormap to assign distinct colors to different categories in a bar plot.
Creating Custom Matplotlib Cmap Colors
While Matplotlib provides many built-in colormaps, you can also create custom colormaps to suit your specific needs. Here’s an example of how to create a custom colormap:
import matplotlib.pyplot as plt
import matplotlib.colors as mcolors
import numpy as np
# Define custom colors
colors = ['#FF0000', '#00FF00', '#0000FF'] # Red, Green, Blue
# Create a custom colormap
n_bins = 100
cmap = mcolors.LinearSegmentedColormap.from_list('custom_cmap', colors, N=n_bins)
# Generate sample data
data = np.random.rand(10, 10)
# Create a heatmap with the custom colormap
plt.imshow(data, cmap=cmap)
plt.colorbar(label='Value')
plt.title('Heatmap with Custom Colormap - how2matplotlib.com')
plt.show()
Output:
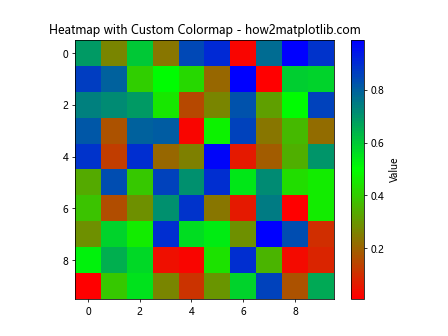
This example demonstrates how to create a custom colormap that transitions from red to green to blue. The LinearSegmentedColormap.from_list()
function is used to create the colormap from a list of colors.
Manipulating Matplotlib Cmap Colors
Matplotlib provides various ways to manipulate colormaps to achieve desired effects. Let’s explore some common techniques:
Reversing Colormaps
You can reverse any colormap by appending ‘_r’ to its name:
import matplotlib.pyplot as plt
import numpy as np
# Generate sample data
data = np.random.rand(10, 10)
# Create two subplots
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 5))
# Plot with original colormap
im1 = ax1.imshow(data, cmap='viridis')
ax1.set_title('Original Viridis - how2matplotlib.com')
plt.colorbar(im1, ax=ax1)
# Plot with reversed colormap
im2 = ax2.imshow(data, cmap='viridis_r')
ax2.set_title('Reversed Viridis - how2matplotlib.com')
plt.colorbar(im2, ax=ax2)
plt.tight_layout()
plt.show()
Output:
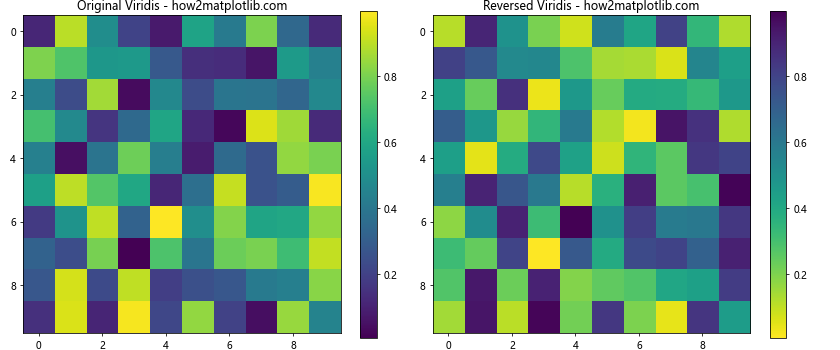
This example shows the ‘viridis’ colormap and its reversed version ‘viridis_r’ side by side.
Truncating Colormaps
You can use a subset of a colormap by truncating it:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.colors import LinearSegmentedColormap
# Generate sample data
data = np.random.rand(10, 10)
# Create a truncated colormap
cmap = plt.get_cmap('viridis')
truncated_cmap = LinearSegmentedColormap.from_list('trunc_viridis', cmap(np.linspace(0.2, 0.8, 100)))
# Create a heatmap with the truncated colormap
plt.imshow(data, cmap=truncated_cmap)
plt.colorbar(label='Value')
plt.title('Heatmap with Truncated Colormap - how2matplotlib.com')
plt.show()
Output:
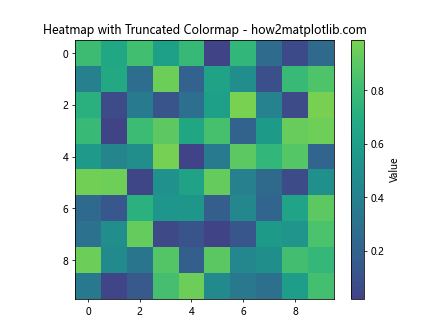
This example demonstrates how to create a truncated version of the ‘viridis’ colormap, using only the middle 60% of the original colormap.
Advanced Techniques with Matplotlib Cmap Colors
Let’s explore some advanced techniques for working with Matplotlib cmap colors:
Discrete Colormaps
Sometimes, you may want to use a discrete set of colors instead of a continuous colormap. Here’s how to create a discrete colormap:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.colors import BoundaryNorm, ListedColormap
# Generate sample data
data = np.random.randint(0, 5, (10, 10))
# Create a discrete colormap
cmap = ListedColormap(['#FF0000', '#00FF00', '#0000FF', '#FFFF00', '#FF00FF'])
bounds = [0, 1, 2, 3, 4, 5]
norm = BoundaryNorm(bounds, cmap.N)
# Create a heatmap with the discrete colormap
plt.imshow(data, cmap=cmap, norm=norm)
plt.colorbar(label='Category', ticks=[0.5, 1.5, 2.5, 3.5, 4.5], boundaries=bounds)
plt.title('Heatmap with Discrete Colormap - how2matplotlib.com')
plt.show()
Output:
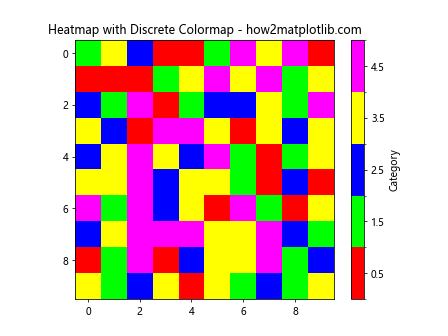
This example creates a discrete colormap with five distinct colors, each representing a different category in the data.
Combining Colormaps
You can create more complex colormaps by combining existing ones:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.colors import LinearSegmentedColormap
# Generate sample data
data = np.random.rand(10, 10)
# Combine two colormaps
cmap1 = plt.get_cmap('viridis')
cmap2 = plt.get_cmap('plasma')
colors1 = cmap1(np.linspace(0, 1, 128))
colors2 = cmap2(np.linspace(0, 1, 128))
colors = np.vstack((colors1, colors2))
combined_cmap = LinearSegmentedColormap.from_list('combined_cmap', colors)
# Create a heatmap with the combined colormap
plt.imshow(data, cmap=combined_cmap)
plt.colorbar(label='Value')
plt.title('Heatmap with Combined Colormap - how2matplotlib.com')
plt.show()
Output:
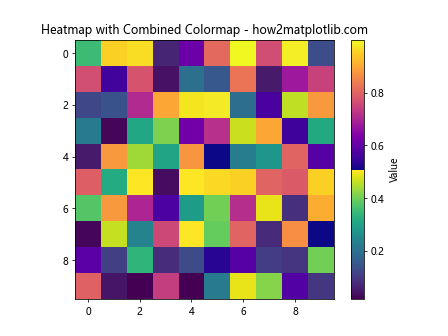
This example demonstrates how to create a new colormap by combining the ‘viridis’ and ‘plasma’ colormaps.
Best Practices for Using Matplotlib Cmap Colors
When working with Matplotlib cmap colors, it’s important to follow some best practices to ensure your visualizations are effective and accessible:
- Choose appropriate colormaps: Use sequential colormaps for continuous data, diverging colormaps for data with a meaningful midpoint, and qualitative colormaps for categorical data.
Consider color blindness: Use colormaps that are perceptually uniform and color-blind friendly, such as ‘viridis’, ‘plasma’, or ‘cividis’.
Provide context: Always include a colorbar with appropriate labels to help viewers interpret the colors in your visualization.
Be consistent: Use consistent colormaps across related visualizations to make comparisons easier.
Avoid rainbow colormaps: Rainbow colormaps like ‘jet’ can be misleading and are generally not recommended for scientific visualizations.
Let’s implement these best practices in an example: