Comprehensive Guide to Matplotlib.axis.Axis.set_gid() Function in Python
Matplotlib.axis.Axis.set_gid() function in Python is an essential tool for managing and organizing elements within Matplotlib plots. This function allows you to set a group id for an Axis object, which can be particularly useful when working with complex visualizations or when you need to manipulate specific elements of your plot programmatically. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.set_gid() function in depth, covering its usage, benefits, and providing numerous examples to help you master this powerful feature.
Understanding the Basics of Matplotlib.axis.Axis.set_gid()
The Matplotlib.axis.Axis.set_gid() function is a method of the Axis class in Matplotlib. It’s used to set a group id (gid) for an Axis object. This gid can be used later to identify and manipulate the axis or its elements. Let’s start with a simple example to illustrate how to use the Matplotlib.axis.Axis.set_gid() function:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_gid('how2matplotlib.com_x_axis')
ax.yaxis.set_gid('how2matplotlib.com_y_axis')
plt.show()
Output:
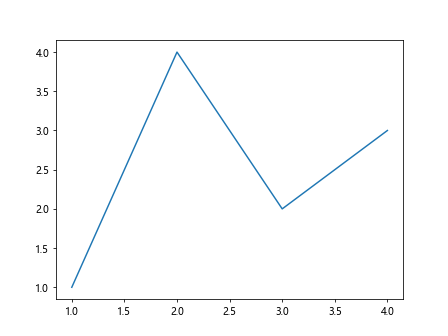
In this example, we create a simple line plot and use the Matplotlib.axis.Axis.set_gid() function to set group ids for both the x-axis and y-axis. The gids ‘how2matplotlib.com_x_axis’ and ‘how2matplotlib.com_y_axis’ can be used later to reference these specific axes.
Why Use Matplotlib.axis.Axis.set_gid()?
The Matplotlib.axis.Axis.set_gid() function provides several benefits when working with Matplotlib:
- Identification: It allows you to uniquely identify axes in complex plots with multiple subplots.
- Manipulation: You can easily manipulate specific axes using their gids.
- Styling: Group ids can be used for applying styles or transformations to specific axes.
- Interactivity: In interactive applications, gids can be used to handle user interactions with specific axes.
Let’s explore these benefits with more detailed examples.
Using Matplotlib.axis.Axis.set_gid() for Identification
When working with complex plots that have multiple subplots, the Matplotlib.axis.Axis.set_gid() function can be incredibly useful for identifying specific axes. Here’s an example:
import matplotlib.pyplot as plt
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax1.set_title('Plot 1')
ax1.xaxis.set_gid('how2matplotlib.com_plot1_x')
ax1.yaxis.set_gid('how2matplotlib.com_plot1_y')
ax2.plot([1, 2, 3, 4], [3, 2, 4, 1])
ax2.set_title('Plot 2')
ax2.xaxis.set_gid('how2matplotlib.com_plot2_x')
ax2.yaxis.set_gid('how2matplotlib.com_plot2_y')
plt.tight_layout()
plt.show()
Output:
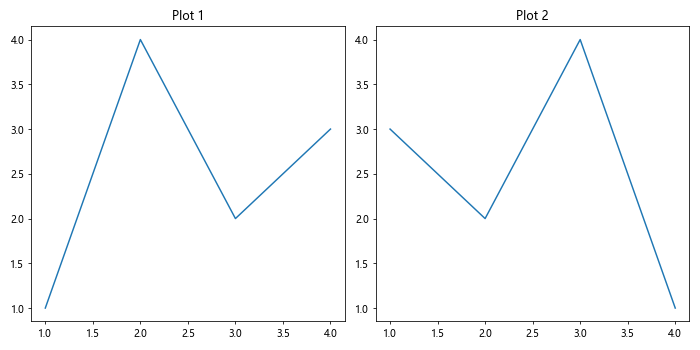
In this example, we create two subplots and use the Matplotlib.axis.Axis.set_gid() function to set unique gids for each axis. This makes it easy to identify and manipulate specific axes later in your code.
Manipulating Axes with Matplotlib.axis.Axis.set_gid()
Once you’ve set gids for your axes using the Matplotlib.axis.Axis.set_gid() function, you can easily manipulate them. Here’s an example that demonstrates how to change the color of a specific axis using its gid:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_gid('how2matplotlib.com_x_axis')
ax.yaxis.set_gid('how2matplotlib.com_y_axis')
# Change the color of the x-axis
for axis in fig.axes:
if axis.xaxis.get_gid() == 'how2matplotlib.com_x_axis':
axis.spines['bottom'].set_color('red')
plt.show()
Output:
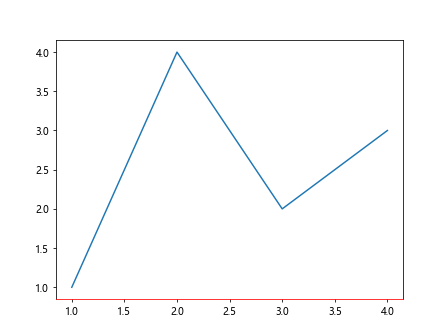
In this example, we use the gid set by Matplotlib.axis.Axis.set_gid() to identify the x-axis and change its color to red.
Styling Axes with Matplotlib.axis.Axis.set_gid()
The Matplotlib.axis.Axis.set_gid() function can also be used to apply styles to specific axes. Here’s an example that demonstrates how to change the font size of tick labels for a specific axis:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_gid('how2matplotlib.com_x_axis')
ax.yaxis.set_gid('how2matplotlib.com_y_axis')
# Change the font size of y-axis tick labels
for axis in fig.axes:
if axis.yaxis.get_gid() == 'how2matplotlib.com_y_axis':
axis.tick_params(axis='y', labelsize=14)
plt.show()
Output:
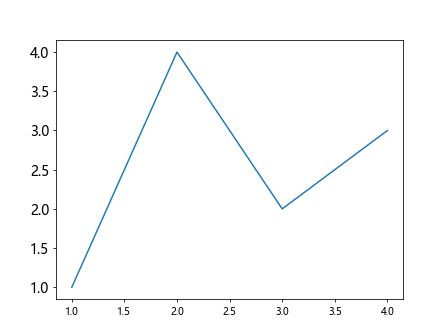
In this example, we use the gid set by Matplotlib.axis.Axis.set_gid() to identify the y-axis and increase the font size of its tick labels.
Using Matplotlib.axis.Axis.set_gid() for Interactivity
The Matplotlib.axis.Axis.set_gid() function can be particularly useful when creating interactive plots. Here’s an example that demonstrates how to create a plot where clicking on an axis changes its color:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_gid('how2matplotlib.com_x_axis')
ax.yaxis.set_gid('how2matplotlib.com_y_axis')
def on_click(event):
if event.inaxes:
if event.inaxes.xaxis.get_gid() == 'how2matplotlib.com_x_axis':
event.inaxes.spines['bottom'].set_color('red')
elif event.inaxes.yaxis.get_gid() == 'how2matplotlib.com_y_axis':
event.inaxes.spines['left'].set_color('blue')
fig.canvas.draw()
fig.canvas.mpl_connect('button_press_event', on_click)
plt.show()
Output:
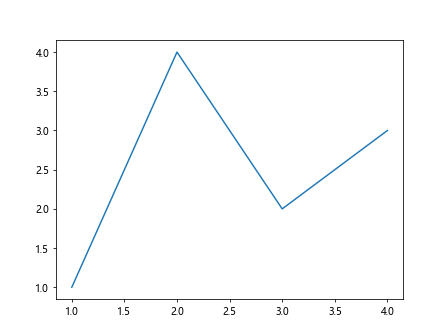
In this interactive example, we use the gids set by Matplotlib.axis.Axis.set_gid() to identify which axis was clicked and change its color accordingly.
Advanced Usage of Matplotlib.axis.Axis.set_gid()
The Matplotlib.axis.Axis.set_gid() function can be used in more advanced scenarios as well. Let’s explore some of these use cases.
Using Matplotlib.axis.Axis.set_gid() with Multiple Subplots
When working with multiple subplots, the Matplotlib.axis.Axis.set_gid() function can help you manage and manipulate axes across different subplots. Here’s an example:
import matplotlib.pyplot as plt
fig, ((ax1, ax2), (ax3, ax4)) = plt.subplots(2, 2, figsize=(10, 10))
axes = [ax1, ax2, ax3, ax4]
for i, ax in enumerate(axes, 1):
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.set_title(f'Plot {i}')
ax.xaxis.set_gid(f'how2matplotlib.com_plot{i}_x')
ax.yaxis.set_gid(f'how2matplotlib.com_plot{i}_y')
# Change color of all x-axes
for ax in fig.axes:
if 'x' in ax.xaxis.get_gid():
ax.spines['bottom'].set_color('red')
plt.tight_layout()
plt.show()
Output:
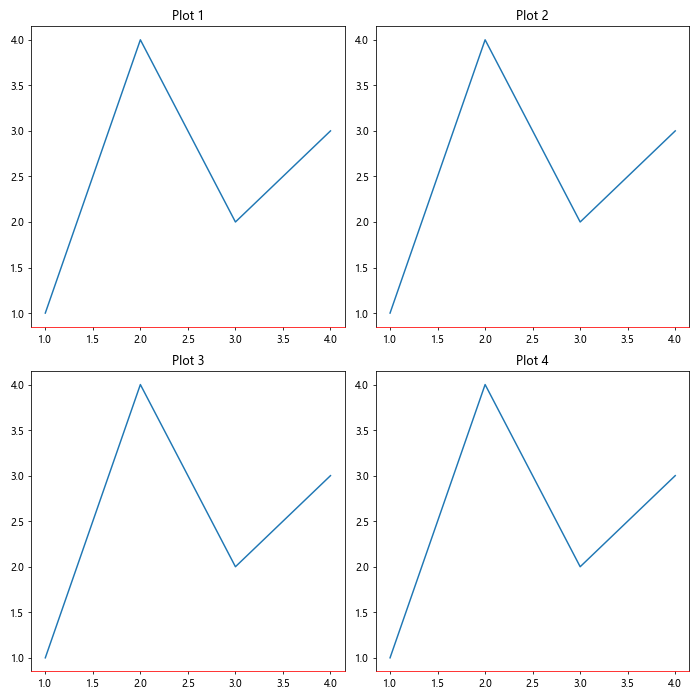
In this example, we create four subplots and use Matplotlib.axis.Axis.set_gid() to set unique gids for each axis. We then use these gids to change the color of all x-axes across all subplots.
Using Matplotlib.axis.Axis.set_gid() with Custom Tick Formatters
The Matplotlib.axis.Axis.set_gid() function can be combined with custom tick formatters to create more complex visualizations. Here’s an example:
import matplotlib.pyplot as plt
import matplotlib.ticker as ticker
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_gid('how2matplotlib.com_x_axis')
ax.yaxis.set_gid('how2matplotlib.com_y_axis')
def custom_formatter(x, pos):
return f'Value: {x:.2f}'
for axis in fig.axes:
if axis.yaxis.get_gid() == 'how2matplotlib.com_y_axis':
axis.yaxis.set_major_formatter(ticker.FuncFormatter(custom_formatter))
plt.show()
Output:
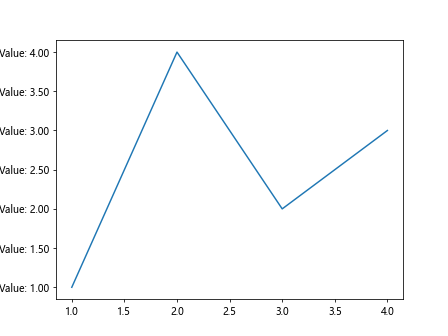
In this example, we use Matplotlib.axis.Axis.set_gid() to identify the y-axis and apply a custom tick formatter to it.
Using Matplotlib.axis.Axis.set_gid() with Logarithmic Scales
The Matplotlib.axis.Axis.set_gid() function can be useful when working with different scale types, such as logarithmic scales. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.logspace(0, 2, 100)
ax.semilogx(x, np.sqrt(x))
ax.xaxis.set_gid('how2matplotlib.com_log_x')
ax.yaxis.set_gid('how2matplotlib.com_linear_y')
# Add grid lines only to the x-axis
for axis in fig.axes:
if axis.xaxis.get_gid() == 'how2matplotlib.com_log_x':
axis.grid(True, axis='x', which='both', linestyle='--', color='gray')
plt.show()
Output:
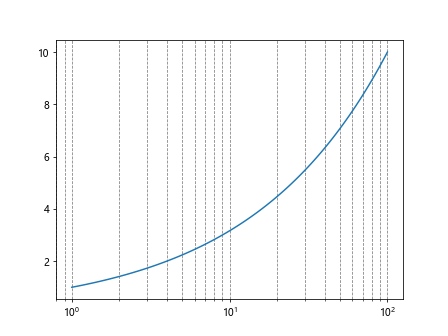
In this example, we create a semilog plot and use Matplotlib.axis.Axis.set_gid() to identify the logarithmic x-axis. We then use this gid to add grid lines only to the x-axis.
Best Practices for Using Matplotlib.axis.Axis.set_gid()
When using the Matplotlib.axis.Axis.set_gid() function, it’s important to follow some best practices to ensure your code is clean, efficient, and maintainable:
- Use descriptive gids: Choose gid names that clearly describe the axis or its purpose. For example, ‘how2matplotlib.com_temperature_x’ is more descriptive than ‘x1’.
Be consistent: Use a consistent naming convention for your gids across your project.
Document your gids: If you’re using many gids in a complex project, consider maintaining a list of gids and their purposes in your documentation.
Avoid hardcoding: Instead of hardcoding gid strings throughout your code, consider using constants or a configuration file.
Check for gid existence: Before manipulating an axis based on its gid, check if the gid exists to avoid errors.
Here’s an example that demonstrates these best practices: