Comprehensive Guide to Using Matplotlib.axis.Axis.get_url() Function in Python
Matplotlib.axis.Axis.get_url() in function Python is an essential method for retrieving URL information associated with an axis in Matplotlib plots. This function plays a crucial role in creating interactive visualizations and linking plot elements to external resources. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.get_url() function in depth, covering its usage, applications, and best practices.
Understanding Matplotlib.axis.Axis.get_url()
Matplotlib.axis.Axis.get_url() is a method that belongs to the Axis class in Matplotlib. It allows you to retrieve the URL associated with an axis object. This function is particularly useful when working with interactive plots or when you want to link specific axis elements to external resources.
Let’s start with a simple example to demonstrate how to use Matplotlib.axis.Axis.get_url():
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Set a URL for the x-axis
ax.xaxis.set_url("https://how2matplotlib.com")
# Get the URL from the x-axis
url = ax.xaxis.get_url()
print(f"The URL associated with the x-axis is: {url}")
plt.show()
Output:
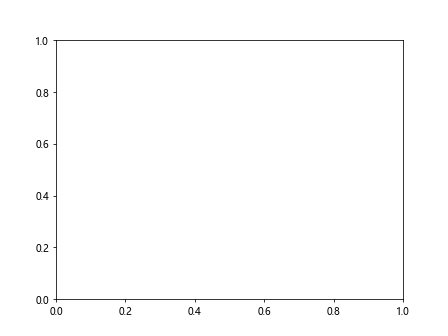
In this example, we first create a figure and axis using plt.subplots()
. We then set a URL for the x-axis using the set_url()
method. Finally, we use the get_url()
method to retrieve the URL and print it.
Applications of Matplotlib.axis.Axis.get_url()
Matplotlib.axis.Axis.get_url() has various applications in data visualization and interactive plotting. Let’s explore some common use cases:
1. Creating Interactive Plots
One of the primary applications of Matplotlib.axis.Axis.get_url() is in creating interactive plots where users can click on axis elements to access additional information or resources.
import matplotlib.pyplot as plt
from matplotlib.backends.backend_tkagg import FigureCanvasTkAgg
import tkinter as tk
def on_click(event):
if event.inaxes:
url = event.inaxes.xaxis.get_url()
if url:
print(f"Clicked on axis with URL: {url}")
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_url("https://how2matplotlib.com")
root = tk.Tk()
canvas = FigureCanvasTkAgg(fig, master=root)
canvas.draw()
canvas.get_tk_widget().pack()
canvas.mpl_connect('button_press_event', on_click)
root.mainloop()
In this example, we create an interactive plot using Tkinter. When the user clicks on the x-axis, the associated URL is printed.
2. Linking Axis Elements to Documentation
Matplotlib.axis.Axis.get_url() can be used to link axis elements to relevant documentation or explanatory resources.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_url("https://how2matplotlib.com/x-axis")
ax.yaxis.set_url("https://how2matplotlib.com/y-axis")
x_url = ax.xaxis.get_url()
y_url = ax.yaxis.get_url()
print(f"X-axis documentation: {x_url}")
print(f"Y-axis documentation: {y_url}")
plt.show()
Output:
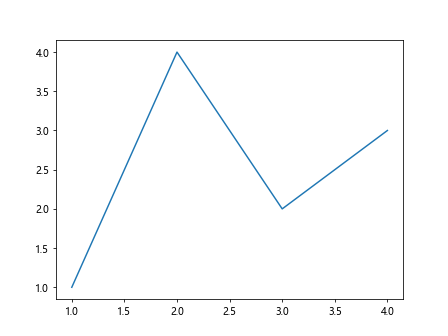
This example demonstrates how to associate different URLs with the x-axis and y-axis, which could be used to link to specific documentation pages for each axis.
3. Dynamic URL Assignment
Matplotlib.axis.Axis.get_url() can be used in conjunction with dynamic URL assignment based on plot data or user interactions.
import matplotlib.pyplot as plt
def update_url(event):
if event.inaxes:
x, y = event.xdata, event.ydata
new_url = f"https://how2matplotlib.com/data?x={x:.2f}&y={y:.2f}"
event.inaxes.xaxis.set_url(new_url)
print(f"Updated URL: {event.inaxes.xaxis.get_url()}")
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
fig.canvas.mpl_connect('motion_notify_event', update_url)
plt.show()
Output:
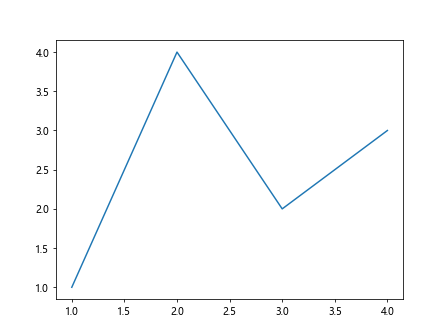
In this example, we dynamically update the x-axis URL based on the mouse position over the plot. The get_url()
method is then used to retrieve and print the updated URL.
Advanced Usage of Matplotlib.axis.Axis.get_url()
Let’s explore some advanced techniques and best practices for using Matplotlib.axis.Axis.get_url() in your Python projects.
1. Combining with Other Axis Properties
Matplotlib.axis.Axis.get_url() can be used in combination with other axis properties to create more informative and interactive plots.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax.xaxis.set_label_text("X-axis")
ax.xaxis.set_url("https://how2matplotlib.com/x-axis")
ax.yaxis.set_label_text("Y-axis")
ax.yaxis.set_url("https://how2matplotlib.com/y-axis")
print(f"X-axis label: {ax.xaxis.get_label_text()}")
print(f"X-axis URL: {ax.xaxis.get_url()}")
print(f"Y-axis label: {ax.yaxis.get_label_text()}")
print(f"Y-axis URL: {ax.yaxis.get_url()}")
plt.show()
Output:
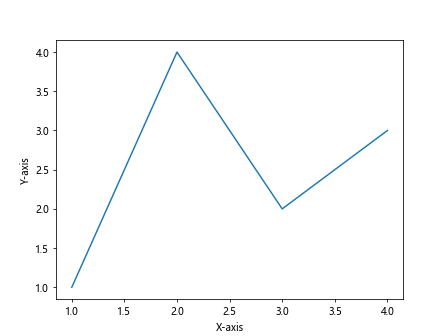
This example demonstrates how to combine get_url()
with other axis properties like labels to create a more comprehensive axis configuration.
2. URL Encoding and Decoding
When working with URLs in Matplotlib.axis.Axis.get_url(), it’s important to handle URL encoding and decoding properly, especially when dealing with special characters or query parameters.
import matplotlib.pyplot as plt
from urllib.parse import quote, unquote
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Encode the URL
encoded_url = quote("https://how2matplotlib.com/search?q=Matplotlib axis")
ax.xaxis.set_url(encoded_url)
# Retrieve and decode the URL
retrieved_url = ax.xaxis.get_url()
decoded_url = unquote(retrieved_url)
print(f"Encoded URL: {retrieved_url}")
print(f"Decoded URL: {decoded_url}")
plt.show()
Output:
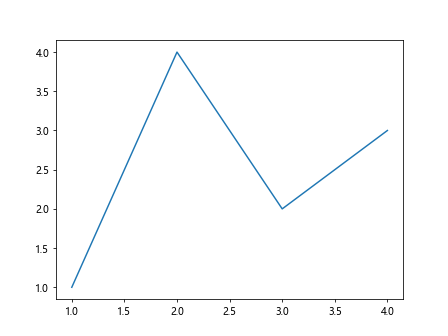
This example shows how to use URL encoding and decoding when working with Matplotlib.axis.Axis.get_url() to ensure proper handling of special characters in URLs.
3. Error Handling
When using Matplotlib.axis.Axis.get_url(), it’s important to implement proper error handling to deal with cases where the URL might not be set or accessible.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
try:
url = ax.xaxis.get_url()
if url is None:
print("No URL set for the x-axis")
else:
print(f"X-axis URL: {url}")
except AttributeError:
print("get_url() method not available for this axis object")
plt.show()
Output:
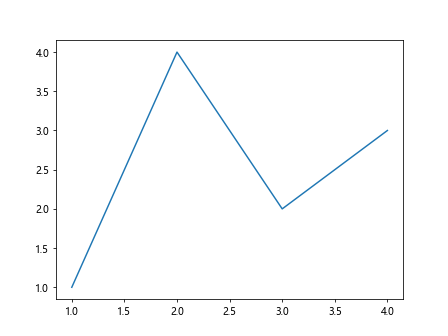
This example demonstrates how to handle cases where the URL might not be set or the get_url()
method might not be available for a particular axis object.
Best Practices for Using Matplotlib.axis.Axis.get_url()
When working with Matplotlib.axis.Axis.get_url() in your Python projects, consider the following best practices:
- Consistency: Maintain consistency in URL assignment across your plots to ensure a uniform user experience.
Documentation: Clearly document the purpose and expected format of URLs associated with axis elements.
Security: Be cautious when using user-provided or dynamically generated URLs to prevent security vulnerabilities.
Performance: Avoid excessive use of
get_url()
in performance-critical sections of your code, as it may impact rendering speed for complex plots.Accessibility: Consider providing alternative ways to access linked resources for users who may not be able to interact with the plot directly.
Let’s look at an example that incorporates these best practices: