Comprehensive Guide to Matplotlib.axis.Axis.get_gid() Function in Python
Matplotlib.axis.Axis.get_gid() function in Python is an essential method for retrieving the group id of an Axis object in Matplotlib. This function plays a crucial role in managing and identifying specific elements within a plot. In this comprehensive guide, we’ll explore the Matplotlib.axis.Axis.get_gid() function in depth, covering its usage, applications, and providing numerous examples to illustrate its functionality.
Understanding the Matplotlib.axis.Axis.get_gid() Function
The Matplotlib.axis.Axis.get_gid() function is a method associated with the Axis class in Matplotlib. Its primary purpose is to retrieve the group id (gid) of an Axis object. The group id is a string that can be used to identify and group related elements in a plot. This function is particularly useful when working with complex plots that contain multiple axes or when you need to manipulate specific axes programmatically.
Let’s start with a simple example to demonstrate how to use the Matplotlib.axis.Axis.get_gid() function:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Set a group id for the axis
ax.set_gid("how2matplotlib.com_axis1")
# Retrieve the group id using get_gid()
gid = ax.get_gid()
print(f"The group id of the axis is: {gid}")
plt.show()
Output:
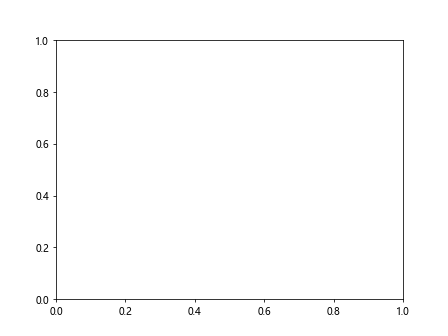
In this example, we create a figure and an axis, set a group id for the axis using the set_gid()
method, and then retrieve it using the get_gid()
function. The group id is then printed to the console.
Practical Applications of Matplotlib.axis.Axis.get_gid()
The Matplotlib.axis.Axis.get_gid() function has several practical applications in data visualization and plot management. Let’s explore some of these applications:
1. Identifying Specific Axes in Multi-Axis Plots
When working with plots that contain multiple axes, the Matplotlib.axis.Axis.get_gid() function can be used to identify and manipulate specific axes. Here’s an example:
import matplotlib.pyplot as plt
# Create a figure with multiple subplots
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(8, 6))
# Set group ids for each axis
ax1.set_gid("how2matplotlib.com_top_axis")
ax2.set_gid("how2matplotlib.com_bottom_axis")
# Plot some data
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax2.plot([1, 2, 3, 4], [4, 3, 2, 1])
# Retrieve and print the group ids
print(f"Top axis group id: {ax1.get_gid()}")
print(f"Bottom axis group id: {ax2.get_gid()}")
plt.tight_layout()
plt.show()
Output:
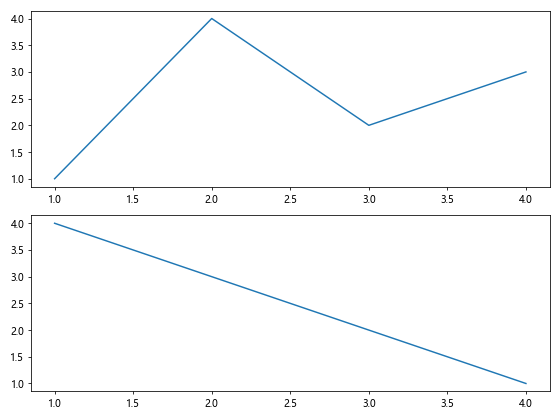
In this example, we create a figure with two subplots, set different group ids for each axis, and then retrieve and print these ids using the Matplotlib.axis.Axis.get_gid() function.
2. Customizing Axis Properties Based on Group ID
The Matplotlib.axis.Axis.get_gid() function can be used in conjunction with conditional statements to apply custom properties to specific axes. Here’s an example:
import matplotlib.pyplot as plt
# Create a figure with multiple subplots
fig, (ax1, ax2, ax3) = plt.subplots(3, 1, figsize=(8, 8))
# Set group ids for each axis
ax1.set_gid("how2matplotlib.com_red_axis")
ax2.set_gid("how2matplotlib.com_green_axis")
ax3.set_gid("how2matplotlib.com_blue_axis")
# Function to customize axis based on group id
def customize_axis(ax):
gid = ax.get_gid()
if gid == "how2matplotlib.com_red_axis":
ax.set_facecolor("#ffcccc")
elif gid == "how2matplotlib.com_green_axis":
ax.set_facecolor("#ccffcc")
elif gid == "how2matplotlib.com_blue_axis":
ax.set_facecolor("#ccccff")
# Apply customization to each axis
for ax in (ax1, ax2, ax3):
customize_axis(ax)
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
plt.tight_layout()
plt.show()
Output:
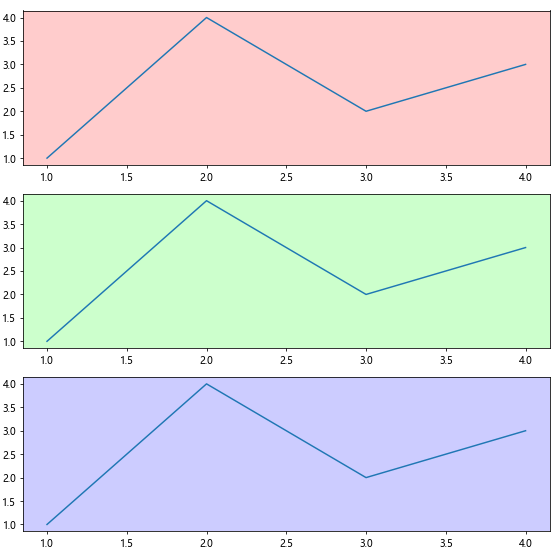
In this example, we create three subplots and assign different group ids to each. We then define a function that uses the Matplotlib.axis.Axis.get_gid() function to retrieve the group id and apply custom background colors based on the id.
Advanced Usage of Matplotlib.axis.Axis.get_gid()
The Matplotlib.axis.Axis.get_gid() function can be used in more advanced scenarios to enhance plot management and interactivity. Let’s explore some of these advanced use cases:
1. Dynamic Axis Manipulation
The Matplotlib.axis.Axis.get_gid() function can be used to dynamically manipulate axes based on user input or other conditions. Here’s an example:
import matplotlib.pyplot as plt
# Create a figure with multiple subplots
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(8, 6))
# Set group ids for each axis
ax1.set_gid("how2matplotlib.com_axis1")
ax2.set_gid("how2matplotlib.com_axis2")
# Plot some data
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3])
ax2.plot([1, 2, 3, 4], [4, 3, 2, 1])
# Function to toggle axis visibility
def toggle_axis_visibility(event):
if event.key == '1':
ax = fig.get_axes()[0]
ax.set_visible(not ax.get_visible())
elif event.key == '2':
ax = fig.get_axes()[1]
ax.set_visible(not ax.get_visible())
plt.draw()
# Connect the key press event to the function
fig.canvas.mpl_connect('key_press_event', toggle_axis_visibility)
plt.tight_layout()
plt.show()
Output:
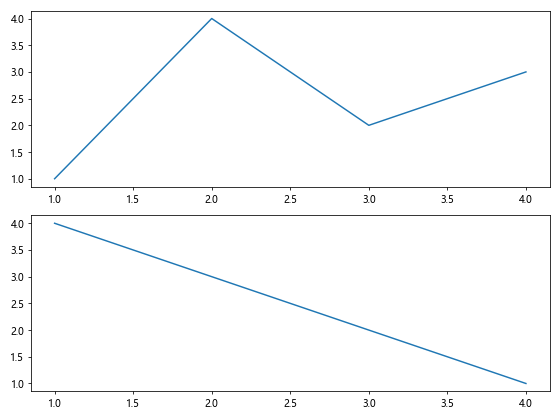
In this example, we create two subplots and assign group ids to each. We then define a function that toggles the visibility of each axis based on user input. The Matplotlib.axis.Axis.get_gid() function could be used within this function to identify which axis to manipulate.
2. Axis Grouping and Batch Operations
The Matplotlib.axis.Axis.get_gid() function can be used to group axes and perform batch operations on them. Here’s an example:
import matplotlib.pyplot as plt
# Create a figure with multiple subplots
fig, axes = plt.subplots(2, 2, figsize=(10, 8))
# Flatten the axes array for easier iteration
axes = axes.flatten()
# Set group ids for each axis
for i, ax in enumerate(axes):
ax.set_gid(f"how2matplotlib.com_axis{i+1}")
# Plot some data
for ax in axes:
ax.plot([1, 2, 3, 4], [1, 4, 2, 3])
# Function to apply a common operation to a group of axes
def apply_to_group(axes, group_prefix, operation):
for ax in axes:
if ax.get_gid().startswith(group_prefix):
operation(ax)
# Example operation: change y-axis limits
def change_ylim(ax):
ax.set_ylim(0, 5)
# Apply the operation to axes with group ids starting with "how2matplotlib.com_axis"
apply_to_group(axes, "how2matplotlib.com_axis", change_ylim)
plt.tight_layout()
plt.show()
Output:
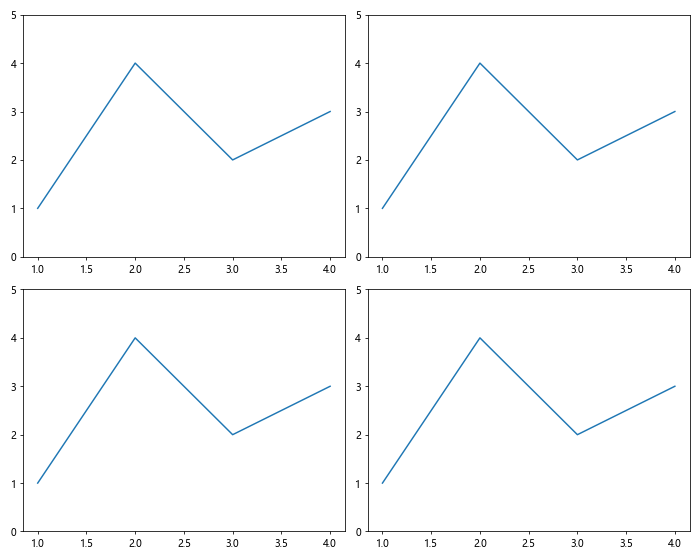
In this example, we create a 2×2 grid of subplots and assign unique group ids to each. We then define a function that applies a common operation to axes based on their group id prefix. The Matplotlib.axis.Axis.get_gid() function is used to retrieve the group id and determine which axes to operate on.
Best Practices for Using Matplotlib.axis.Axis.get_gid()
When working with the Matplotlib.axis.Axis.get_gid() function, it’s important to follow some best practices to ensure efficient and maintainable code:
- Use descriptive group ids: Choose group ids that clearly describe the purpose or content of the axis. This makes it easier to understand and maintain your code.
Be consistent with naming conventions: Establish a consistent naming convention for your group ids across your project.
Document your group ids: Maintain a list or documentation of the group ids used in your project and their purposes.
Use group ids sparingly: Only use group ids when necessary for specific identification or grouping purposes. Overuse can lead to cluttered and hard-to-maintain code.
Combine with other Matplotlib functions: Use the Matplotlib.axis.Axis.get_gid() function in combination with other Matplotlib functions to create more powerful and flexible visualizations.
Let’s look at an example that demonstrates these best practices: