How to Use Matplotlib.axes.Axes.is_transform_set() in Python
Matplotlib.axes.Axes.is_transform_set() in Python is an essential method for working with transformations in Matplotlib. This function is used to check whether a transform has been set for an Axes object. In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.axes.Axes.is_transform_set(), providing detailed explanations and practical examples to help you master this powerful tool.
Understanding Matplotlib.axes.Axes.is_transform_set()
Matplotlib.axes.Axes.is_transform_set() is a method that belongs to the Axes class in Matplotlib. It returns a boolean value indicating whether a transform has been set for the Axes object. This method is particularly useful when you need to determine if any custom transformations have been applied to your plot.
Let’s start with a simple example to demonstrate how to use Matplotlib.axes.Axes.is_transform_set():
import matplotlib.pyplot as plt
# Create a figure and an axes object
fig, ax = plt.subplots()
# Check if a transform is set
is_transform_set = ax.is_transform_set()
print(f"Is transform set? {is_transform_set}")
plt.title("Matplotlib.axes.Axes.is_transform_set() Example - how2matplotlib.com")
plt.show()
Output:
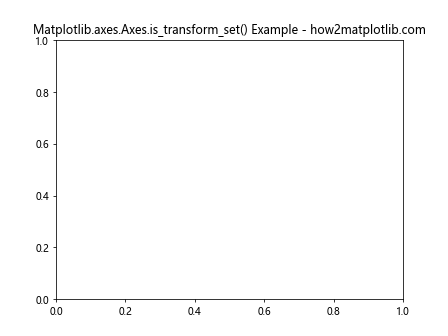
In this example, we create a figure and an axes object using plt.subplots(). We then call the is_transform_set() method on the axes object to check if a transform has been set. By default, no custom transform is set, so the method will return False.
When to Use Matplotlib.axes.Axes.is_transform_set()
Matplotlib.axes.Axes.is_transform_set() is particularly useful in scenarios where you need to:
- Verify if any custom transformations have been applied to your plot
- Conditionally apply transformations based on whether one has already been set
- Debug issues related to unexpected plot appearances due to transformations
Let’s explore a more complex example where we use Matplotlib.axes.Axes.is_transform_set() in conjunction with other Matplotlib functions:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a figure and an axes object
fig, ax = plt.subplots()
# Plot the data
ax.plot(x, y, label='Original')
# Check if a transform is set
is_transform_set_before = ax.is_transform_set()
print(f"Is transform set before? {is_transform_set_before}")
# Apply a custom transform
transform = Affine2D().rotate_deg(45) + ax.transData
ax.plot(x, y, transform=transform, label='Rotated')
# Check if a transform is set after applying the custom transform
is_transform_set_after = ax.is_transform_set()
print(f"Is transform set after? {is_transform_set_after}")
plt.legend()
plt.title("Matplotlib.axes.Axes.is_transform_set() Example - how2matplotlib.com")
plt.show()
Output:
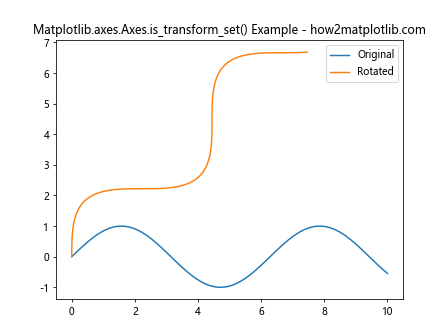
In this example, we first plot a sine wave using the original data. We then check if a transform is set using Matplotlib.axes.Axes.is_transform_set(). Next, we apply a custom transform to rotate the plot by 45 degrees and plot the transformed data. Finally, we check again if a transform is set. This demonstrates how Matplotlib.axes.Axes.is_transform_set() can be used to verify the application of transformations.
Exploring Different Types of Transformations
Matplotlib supports various types of transformations that can be applied to plots. Let’s explore some of these transformations and how Matplotlib.axes.Axes.is_transform_set() can be used in conjunction with them.
Scaling Transformation
Scaling transformations allow you to change the scale of your plot. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a figure and an axes object
fig, ax = plt.subplots()
# Plot the original data
ax.plot(x, y, label='Original')
# Check if a transform is set
is_transform_set_before = ax.is_transform_set()
print(f"Is transform set before? {is_transform_set_before}")
# Apply a scaling transformation
scale_factor = 2
transform = Affine2D().scale(scale_factor) + ax.transData
ax.plot(x, y, transform=transform, label=f'Scaled (x{scale_factor})')
# Check if a transform is set after applying the scaling transformation
is_transform_set_after = ax.is_transform_set()
print(f"Is transform set after? {is_transform_set_after}")
plt.legend()
plt.title("Scaling Transformation Example - how2matplotlib.com")
plt.show()
Output:
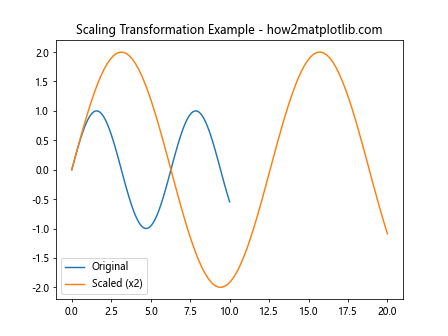
In this example, we apply a scaling transformation to double the size of the plot. We use Matplotlib.axes.Axes.is_transform_set() to check if a transform is set before and after applying the scaling transformation.
Translation Transformation
Translation transformations allow you to move your plot. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a figure and an axes object
fig, ax = plt.subplots()
# Plot the original data
ax.plot(x, y, label='Original')
# Check if a transform is set
is_transform_set_before = ax.is_transform_set()
print(f"Is transform set before? {is_transform_set_before}")
# Apply a translation transformation
dx, dy = 2, 1
transform = Affine2D().translate(dx, dy) + ax.transData
ax.plot(x, y, transform=transform, label=f'Translated ({dx}, {dy})')
# Check if a transform is set after applying the translation transformation
is_transform_set_after = ax.is_transform_set()
print(f"Is transform set after? {is_transform_set_after}")
plt.legend()
plt.title("Translation Transformation Example - how2matplotlib.com")
plt.show()
Output:
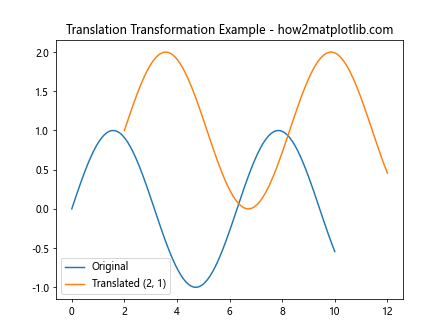
In this example, we apply a translation transformation to move the plot by 2 units along the x-axis and 1 unit along the y-axis. We use Matplotlib.axes.Axes.is_transform_set() to check if a transform is set before and after applying the translation transformation.
Rotation Transformation
Rotation transformations allow you to rotate your plot. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a figure and an axes object
fig, ax = plt.subplots()
# Plot the original data
ax.plot(x, y, label='Original')
# Check if a transform is set
is_transform_set_before = ax.is_transform_set()
print(f"Is transform set before? {is_transform_set_before}")
# Apply a rotation transformation
angle = 45
transform = Affine2D().rotate_deg(angle) + ax.transData
ax.plot(x, y, transform=transform, label=f'Rotated ({angle}°)')
# Check if a transform is set after applying the rotation transformation
is_transform_set_after = ax.is_transform_set()
print(f"Is transform set after? {is_transform_set_after}")
plt.legend()
plt.title("Rotation Transformation Example - how2matplotlib.com")
plt.show()
Output:
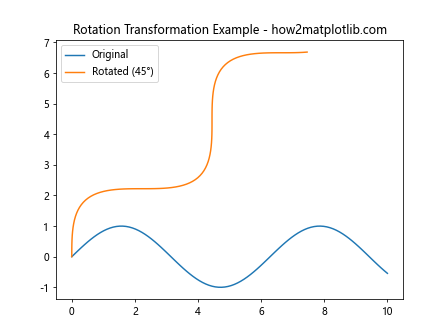
In this example, we apply a rotation transformation to rotate the plot by 45 degrees. We use Matplotlib.axes.Axes.is_transform_set() to check if a transform is set before and after applying the rotation transformation.
Combining Multiple Transformations
Matplotlib allows you to combine multiple transformations to create more complex effects. Let’s see how we can use Matplotlib.axes.Axes.is_transform_set() when working with combined transformations:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a figure and an axes object
fig, ax = plt.subplots()
# Plot the original data
ax.plot(x, y, label='Original')
# Check if a transform is set
is_transform_set_before = ax.is_transform_set()
print(f"Is transform set before? {is_transform_set_before}")
# Apply multiple transformations
scale_factor = 1.5
angle = 30
dx, dy = 1, 0.5
transform = (
Affine2D()
.scale(scale_factor)
.rotate_deg(angle)
.translate(dx, dy)
) + ax.transData
ax.plot(x, y, transform=transform, label='Combined Transform')
# Check if a transform is set after applying the combined transformation
is_transform_set_after = ax.is_transform_set()
print(f"Is transform set after? {is_transform_set_after}")
plt.legend()
plt.title("Combined Transformations Example - how2matplotlib.com")
plt.show()
Output:
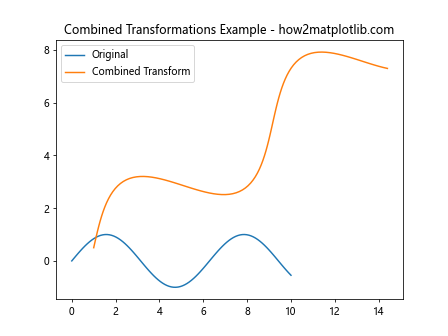
In this example, we combine scaling, rotation, and translation transformations. We use Matplotlib.axes.Axes.is_transform_set() to check if a transform is set before and after applying the combined transformation.
Using Matplotlib.axes.Axes.is_transform_set() with Different Plot Types
Matplotlib.axes.Axes.is_transform_set() can be used with various types of plots. Let’s explore how to use it with different plot types:
Scatter Plot
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.random.rand(50)
y = np.random.rand(50)
# Create a figure and an axes object
fig, ax = plt.subplots()
# Create a scatter plot
ax.scatter(x, y, label='Original')
# Check if a transform is set
is_transform_set_before = ax.is_transform_set()
print(f"Is transform set before? {is_transform_set_before}")
# Apply a transformation
transform = Affine2D().scale(2) + ax.transData
ax.scatter(x, y, transform=transform, label='Transformed')
# Check if a transform is set after applying the transformation
is_transform_set_after = ax.is_transform_set()
print(f"Is transform set after? {is_transform_set_after}")
plt.legend()
plt.title("Scatter Plot with Transformation - how2matplotlib.com")
plt.show()
Output:
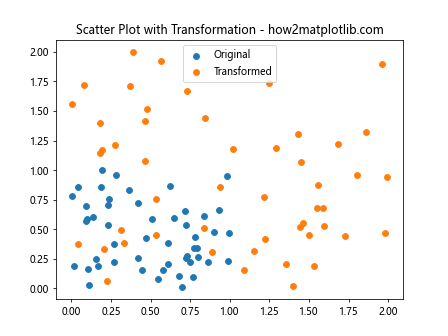
In this example, we create a scatter plot and apply a scaling transformation to it. We use Matplotlib.axes.Axes.is_transform_set() to check if a transform is set before and after applying the transformation.
Bar Plot
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
categories = ['A', 'B', 'C', 'D']
values = [3, 7, 2, 5]
# Create a figure and an axes object
fig, ax = plt.subplots()
# Create a bar plot
ax.bar(categories, values, label='Original')
# Check if a transform is set
is_transform_set_before = ax.is_transform_set()
print(f"Is transform set before? {is_transform_set_before}")
# Apply a transformation
transform = Affine2D().translate(0.5, 0) + ax.transData
ax.bar(categories, values, transform=transform, alpha=0.5, label='Transformed')
# Check if a transform is set after applying the transformation
is_transform_set_after = ax.is_transform_set()
print(f"Is transform set after? {is_transform_set_after}")
plt.legend()
plt.title("Bar Plot with Transformation - how2matplotlib.com")
plt.show()
Output:
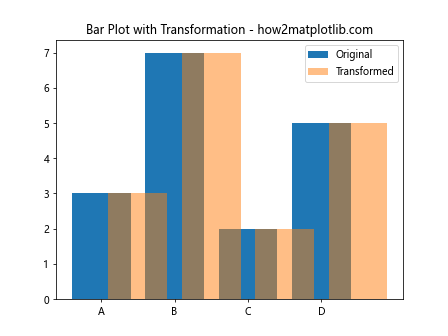
In this example, we create a bar plot and apply a translation transformation to it. We use Matplotlib.axes.Axes.is_transform_set() to check if a transform is set before and after applying the transformation.
Advanced Usage of Matplotlib.axes.Axes.is_transform_set()
Now that we’ve covered the basics, let’s explore some advanced usage scenarios for Matplotlib.axes.Axes.is_transform_set().
Conditional Transformation Application
You can use Matplotlib.axes.Axes.is_transform_set() to conditionally apply transformations based on whether one has already been set:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.linspace(0, 10, 100)
y = np.sin(x)
# Create a figure and an axes object
fig, ax = plt.subplots()
# Plot the original data
ax.plot(x, y, label='Original')
# Check if a transform is set
if not ax.is_transform_set():
print("No transform set. Applying a new transform.")
transform = Affine2D().scale(1.5) + ax.transData
ax.plot(x, y, transform=transform, label='Transformed')
else:
print("A transform is already set. Skipping new transform.")
plt.legend()
plt.title("Conditional Transformation Example - how2matplotlib.com")
plt.show()
Output:
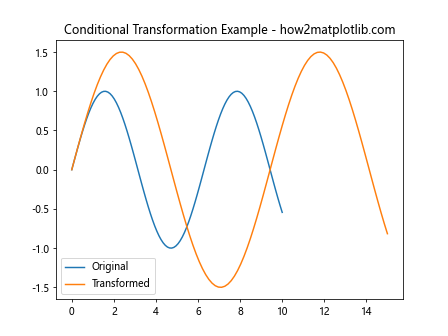
In this example, we check if a transform is set using Matplotlib.axes.Axes.is_transform_set(). If no transform is set, we apply a new transformation. This approach can be useful when you want to avoid overwriting existing transformations.
Working with Multiple Axes
When working with multiple axes, you may need to check and manage transformations for each axes independently. Here’s an example of how to use Matplotlib.axes.Axes.is_transform_set() with multiple axes:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.transforms import Affine2D
# Create data
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
# Create a figure with two subplots
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 5))
# Plot data on the first axes
ax1.plot(x, y1, label='Sin')
transform1 = Affine2D().scale(1.5) + ax1.transData
ax1.plot(x, y1, transform=transform1, label='Transformed Sin')
# Plot data on the second axes
ax2.plot(x, y2, label='Cos')
# Check if transforms are set for both axes
is_transform_set_ax1 = ax1.is_transform_set()
is_transform_set_ax2 = ax2.is_transform_set()
print(f"Is transform set for ax1? {is_transform_set_ax1}")
print(f"Is transform set for ax2? {is_transform_set_ax2}")
# Add legends and titles
ax1.legend()
ax2.legend()
ax1.set_title("Sine Wave - how2matplotlib.com")
ax2.set_title("Cosine Wave - how2matplotlib.com")
plt.suptitle("Working with Multiple Axes - how2matplotlib.com")
plt.tight_layout()
plt.show()
Output:
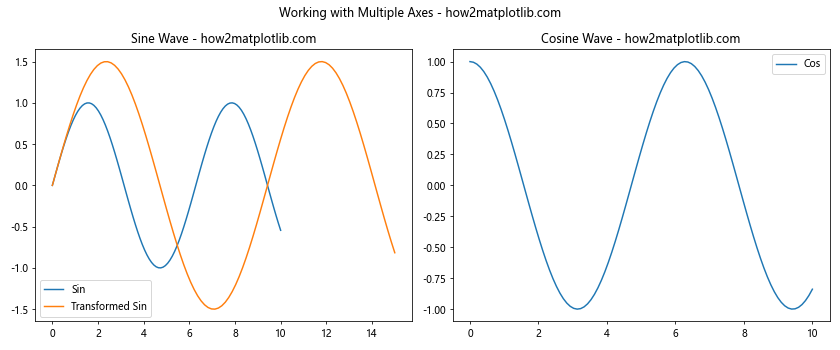
In this example, we create two subplots and apply a transformation to only one of them. We then use Matplotlib.axes.Axes.is_transform_set() to check if transforms are set for both axes independently.
Common Pitfalls and How to Avoid Them
When working with Matplotlib.axes.Axes.is_transform_set(), there are a few common pitfalls that you should be aware of:
- Assuming all axes have the same transformation: When working with multiple axes, remember that each axes object has its own transformation. Always check the transformation for each axes individually using Matplotlib.axes.Axes.is_transform_set().
Forgetting to update the transform after changes: If you modify the plot or apply new transformations, make sure to check the transform status again using Matplotlib.axes.Axes.is_transform_set().
Ignoring the return value: Always pay attention to the boolean value returned by Matplotlib.axes.Axes.is_transform_set(). It’s easy to overlook this information, which can lead to unexpected behavior in your plots.
Here’s an example that demonstrates how to avoid these pitfalls: