Comprehensive Guide to Matplotlib.axes.Axes.get_transformed_clip_path_and_affine() in Python: How to Transform Clip Paths and Affines
Matplotlib.axes.Axes.get_transformed_clip_path_and_affine() in Python is a powerful method that allows you to retrieve the transformed clip path and affine transformation for an Axes object. This function is essential for advanced plotting and customization in Matplotlib, particularly when dealing with complex visualizations that require precise control over the clipping region and coordinate transformations. In this comprehensive guide, we’ll explore the ins and outs of get_transformed_clip_path_and_affine(), its usage, and provide numerous examples to help you master this important Matplotlib feature.
Understanding Matplotlib.axes.Axes.get_transformed_clip_path_and_affine()
Matplotlib.axes.Axes.get_transformed_clip_path_and_affine() is a method that returns a tuple containing two elements: the transformed clip path and the affine transformation. The clip path defines the region within which the plot elements are visible, while the affine transformation describes how the coordinates are transformed from the data space to the display space.
To better understand this method, let’s break down its components:
- Clip Path: A path that defines the boundary of the visible region in the plot.
- Affine Transformation: A linear transformation that preserves straight lines and parallelism.
The get_transformed_clip_path_and_affine() method is particularly useful when you need to perform custom drawing or when you want to apply additional transformations to your plot elements.
Basic Usage of get_transformed_clip_path_and_affine()
Let’s start with a basic example of how to use get_transformed_clip_path_and_affine():
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
ax.plot(np.random.rand(10), label='how2matplotlib.com')
clip_path, affine = ax.get_transformed_clip_path_and_affine()
print(f"Clip Path: {clip_path}")
print(f"Affine Transformation: {affine}")
plt.legend()
plt.title('Basic usage of get_transformed_clip_path_and_affine()')
plt.show()
Output:
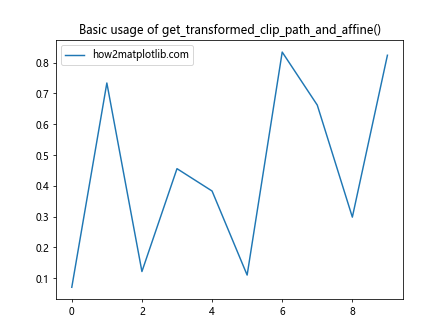
In this example, we create a simple plot and then call get_transformed_clip_path_and_affine() on the Axes object. The method returns the clip path and affine transformation, which we then print to the console.
Custom Clipping with get_transformed_clip_path_and_affine()
The clip path returned by get_transformed_clip_path_and_affine() can be used to create custom clipping regions for your plot elements. Here’s an example:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import numpy as np
fig, ax = plt.subplots()
ax.plot(np.random.rand(10), label='how2matplotlib.com')
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Create a custom clipping path
custom_clip = patches.Circle((0.5, 0.5), 0.3, transform=ax.transAxes)
# Apply the custom clipping path to a rectangle
rect = patches.Rectangle((0.2, 0.2), 0.6, 0.6, transform=ax.transAxes,
facecolor='red', alpha=0.5)
rect.set_clip_path(custom_clip)
ax.add_patch(rect)
plt.legend()
plt.title('Custom Clipping with get_transformed_clip_path_and_affine()')
plt.show()
Output:
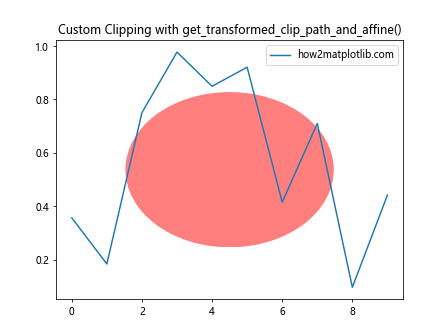
In this example, we create a custom circular clipping path and apply it to a rectangle patch. This demonstrates how you can use get_transformed_clip_path_and_affine() in conjunction with custom clipping paths to create more complex visualizations.
Working with Multiple Axes and get_transformed_clip_path_and_affine()
When working with multiple axes, get_transformed_clip_path_and_affine() can be particularly useful for ensuring consistent transformations across different subplots. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
ax1.plot(np.random.rand(10), label='how2matplotlib.com')
ax2.plot(np.random.rand(10), label='how2matplotlib.com')
clip_path1, affine1 = ax1.get_transformed_clip_path_and_affine()
clip_path2, affine2 = ax2.get_transformed_clip_path_and_affine()
# Apply the transformation from ax1 to a text in ax2
ax2.text(0.5, 0.5, 'Transformed Text', transform=affine1)
plt.legend()
plt.suptitle('Working with Multiple Axes and get_transformed_clip_path_and_affine()')
plt.show()
Output:
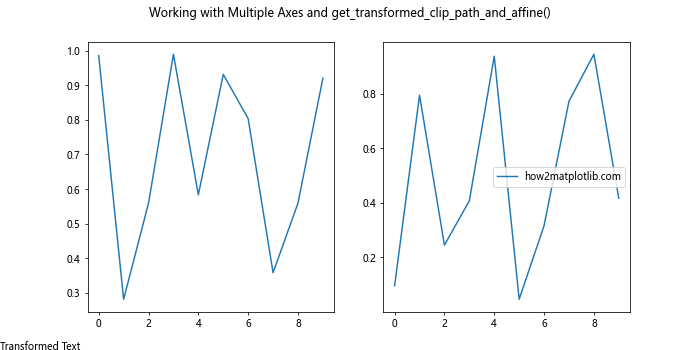
In this example, we create two subplots and use get_transformed_clip_path_and_affine() to retrieve the transformations for both. We then apply the transformation from the first axes to a text object in the second axes, demonstrating how you can maintain consistent coordinate systems across multiple subplots.
Animating with get_transformed_clip_path_and_affine()
get_transformed_clip_path_and_affine() can be used in animations to create dynamic transformations. Here’s an example of a simple animation using this method:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
line, = ax.plot([], [], label='how2matplotlib.com')
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
def animate(frame):
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x + frame/10)
line.set_data(x, y)
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Use the affine transformation to update the line's transform
line.set_transform(affine)
return line,
ani = animation.FuncAnimation(fig, animate, frames=100, interval=50, blit=True)
plt.legend()
plt.title('Animating with get_transformed_clip_path_and_affine()')
plt.show()
Output:
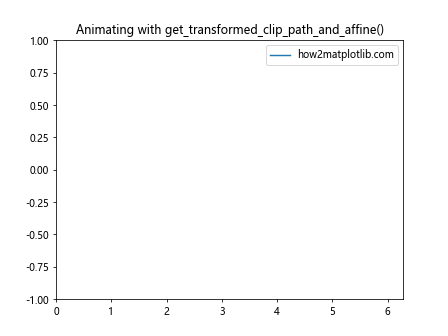
In this animation, we use get_transformed_clip_path_and_affine() to update the line’s transformation in each frame, creating a dynamic effect.
Advanced Clipping Techniques with get_transformed_clip_path_and_affine()
get_transformed_clip_path_and_affine() can be used for advanced clipping techniques, such as creating non-rectangular plot areas. Here’s an example:
import matplotlib.pyplot as plt
import matplotlib.path as mpath
import numpy as np
fig, ax = plt.subplots()
# Create a star-shaped path
star = mpath.Path.unit_regular_star(5, 0.5)
clip_path = mpath.Path(star.vertices * 0.5 + 0.5, star.codes)
# Get the transformed clip path and affine
_, affine = ax.get_transformed_clip_path_and_affine()
# Apply the star-shaped clip path
ax.set_clip_path(clip_path, transform=ax.transAxes)
# Plot some data
x = np.linspace(0, 1, 100)
ax.plot(x, np.sin(2*np.pi*x), label='how2matplotlib.com')
plt.legend()
plt.title('Advanced Clipping with get_transformed_clip_path_and_affine()')
plt.show()
Output:
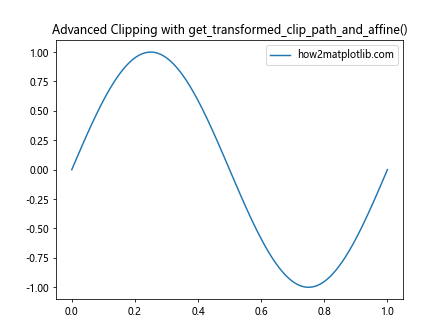
In this example, we create a star-shaped clipping path and apply it to the entire axes, resulting in a non-rectangular plot area.
Using get_transformed_clip_path_and_affine() with Custom Projections
get_transformed_clip_path_and_affine() can be particularly useful when working with custom projections. Here’s an example using a polar projection:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(subplot_kw=dict(projection='polar'))
# Plot some data
theta = np.linspace(0, 2*np.pi, 100)
r = np.sin(4*theta)
ax.plot(theta, r, label='how2matplotlib.com')
# Get the transformed clip path and affine
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Use the affine transformation to place a text in data coordinates
ax.text(np.pi/4, 0.5, 'Polar Text', transform=affine)
plt.legend()
plt.title('Using get_transformed_clip_path_and_affine() with Custom Projections')
plt.show()
Output:
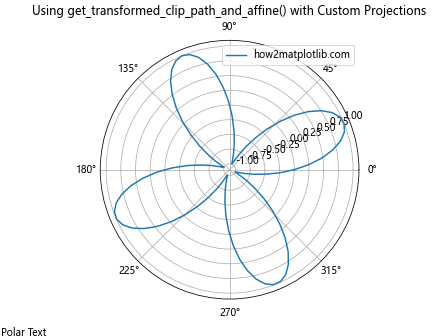
In this example, we use a polar projection and demonstrate how get_transformed_clip_path_and_affine() can be used to correctly place text in the transformed coordinate system.
Handling Logarithmic Scales with get_transformed_clip_path_and_affine()
When working with logarithmic scales, get_transformed_clip_path_and_affine() can help ensure correct transformations. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
# Set logarithmic scale for y-axis
ax.set_yscale('log')
# Plot some data
x = np.linspace(1, 10, 100)
ax.plot(x, x**2, label='how2matplotlib.com')
# Get the transformed clip path and affine
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Use the affine transformation to place a text
ax.text(5, 50, 'Log Scale Text', transform=affine)
plt.legend()
plt.title('Handling Logarithmic Scales with get_transformed_clip_path_and_affine()')
plt.show()
Output:
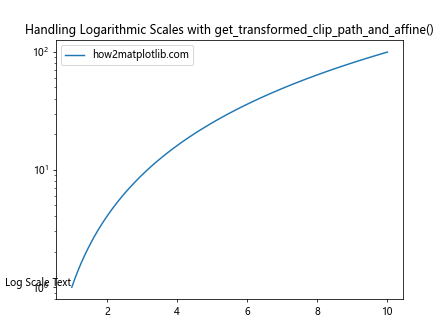
In this example, we use a logarithmic scale for the y-axis and demonstrate how get_transformed_clip_path_and_affine() correctly handles the transformation for text placement.
Creating Custom Legends with get_transformed_clip_path_and_affine()
get_transformed_clip_path_and_affine() can be used to create custom legend entries. Here’s an example:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import numpy as np
fig, ax = plt.subplots()
# Plot some data
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x), label='how2matplotlib.com')
# Get the transformed clip path and affine
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Create a custom legend entry
custom_line = patches.Rectangle((0, 0), 1, 1, facecolor='red')
custom_line.set_transform(affine)
ax.legend([custom_line], ['Custom Legend'])
plt.title('Creating Custom Legends with get_transformed_clip_path_and_affine()')
plt.show()
Output:
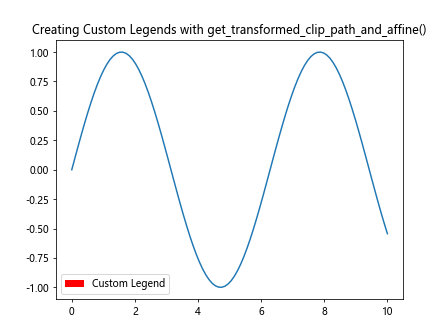
In this example, we create a custom legend entry using a Rectangle patch and apply the affine transformation from get_transformed_clip_path_and_affine() to ensure it’s correctly positioned in the legend.
Applying get_transformed_clip_path_and_affine() to 3D Plots
While get_transformed_clip_path_and_affine() is primarily used with 2D plots, it can also be applied to 3D plots. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits.mplot3d import Axes3D
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# Plot some 3D data
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
ax.plot_surface(X, Y, Z, cmap='viridis')
# Get the transformed clip path and affine
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Use the affine transformation to place a text in 3D space
ax.text(0, 0, 0, 'how2matplotlib.com', transform=affine)
plt.title('Applying get_transformed_clip_path_and_affine() to 3D Plots')
plt.show()
Output:
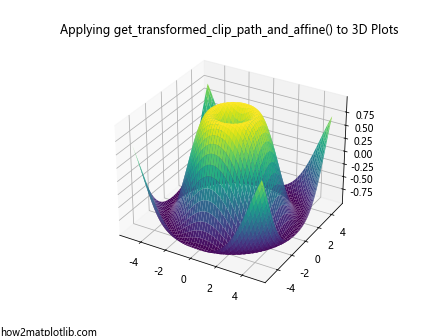
In this example, we create a 3D surface plot and use get_transformed_clip_path_and_affine() to correctly place text in the 3D space.
Using get_transformed_clip_path_and_affine() with Colorbars
get_transformed_clip_path_and_affine() can be useful when working with colorbars. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
# Create a heatmap
data = np.random.rand(10, 10)
im = ax.imshow(data, cmap='viridis')
# Add a colorbar
cbar = plt.colorbar(im)
# Get the transformed clip path and affine for the main axes
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Use the affine transformation to place text relative to the main axes
ax.text(5, 11, 'how2matplotlib.com', transform=affine)
plt.title('Using get_transformed_clip_path_and_affine() with Colorbars')
plt.show()
Output:
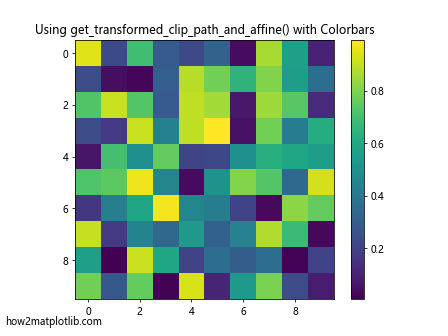
In this example, we create a heatmap with a colorbar and use get_transformed_clip_path_and_affine() to place text relative to the main axes, even though the colorbar has changed the overall figure layout.
Handling Aspect Ratios with get_transformed_clip_path_and_affine()
When working with plots that have specific aspect ratios, get_transformed_clip_path_and_affine() can help ensure correct transformations. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
# Plot some data
x = np.linspace(0, 10, 100)
ax.plot(x, np.sin(x), label='how2matplotlib.com')
# Set a specific aspect ratio
ax.set_aspect('equal')
# Get the transformed clip path and affine
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Use the affine transformation to place text
ax.text(5, 0, 'Equal Aspect Text', transform=affine)
plt.legend()
plt.title('Handling Aspect Ratios with get_transformed_clip_path_and_affine()')
plt.show()
Output:
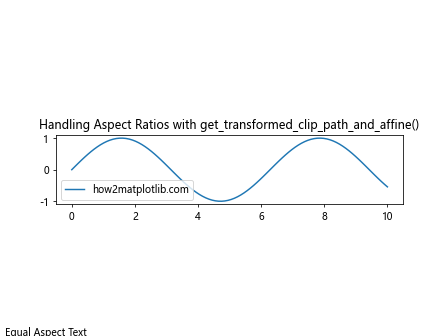
In this example, we set the aspect ratio of the plot to ‘equal’ and demonstrate how get_transformed_clip_path_and_affine() correctly handles the transformation for text placement.
Working with Polar Plots and get_transformed_clip_path_and_affine()
get_transformed_clip_path_and_affine() can be particularly useful when working with polar plots. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(subplot_kw=dict(projection='polar'))
# Plot some data
theta = np.linspace(0, 2*np.pi, 100)
r = 1 + 0.5 * np.sin(5*theta)
ax.plot(theta, r, label='how2matplotlib.com')
# Get the transformed clip path and affine
clip_path, affine = ax.get_transformed_clip_path_and_affine()
# Use the affine transformation to place text in polar coordinates
ax.text(np.pi/4, 1.5, 'Polar Text', transform=affine)
plt.legend()
plt.title('Working with Polar Plots and get_transformed_clip_path_and_affine()')
plt.show()
Output:
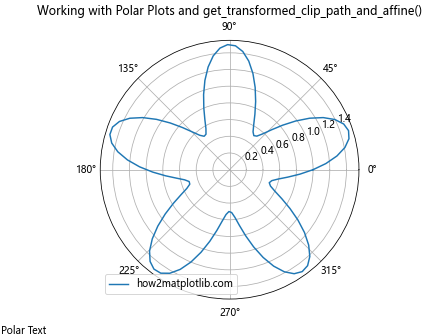
In this example, we create a polar plot and use get_transformed_clip_path_and_affine() to correctly place text in polar coordinates.
Applying get_transformed_clip_path_and_affine() to Subplots
When working with subplots, get_transformed_clip_path_and_affine() can be applied to each individual subplot. Here’s an example:
import matplotlib.pyplot as plt
import numpy as np
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
# Plot data in both subplots
x = np.linspace(0, 10, 100)
ax1.plot(x, np.sin(x), label='how2matplotlib.com')
ax2.plot(x, np.cos(x), label='how2matplotlib.com')
# Get transformed clip path and affine for each subplot
clip_path1, affine1 = ax1.get_transformed_clip_path_and_affine()
clip_path2, affine2 = ax2.get_transformed_clip_path_and_affine()
# Use the affine transformations to place text in each subplot
ax1.text(5, 0, 'Subplot 1', transform=affine1)
ax2.text(5, 0, 'Subplot 2', transform=affine2)
plt.legend()
plt.suptitle('Applying get_transformed_clip_path_and_affine() to Subplots')
plt.show()
Output:
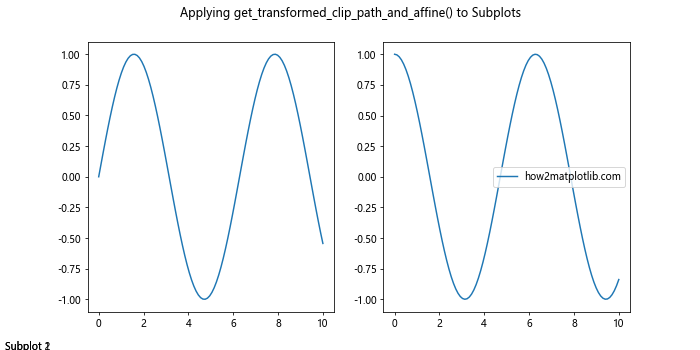
In this example, we create two subplots and use get_transformed_clip_path_and_affine() for each subplot to correctly place text in their respective coordinate systems.
Conclusion
Matplotlib.axes.Axes.get_transformed_clip_path_and_affine() in Python is a powerful tool for advanced plotting and customization in Matplotlib. Throughout this comprehensive guide, we’ve explored various aspects and applications of this method, including:
- Basic usage and understanding of get_transformed_clip_path_and_affine()
- Applying transformations to plot elements
- Custom clipping techniques
- Working with multiple axes
- Creating animations
- Advanced clipping techniques
- Combining transformations
- Using custom projections
- Handling logarithmic scales
- Creating custom legends
- Applying to 3D plots
- Working with colorbars
- Handling aspect ratios
- Working with polar plots
- Applying to subplots
By mastering get_transformed_clip_path_and_affine(), you can gain fine-grained control over your Matplotlib visualizations, enabling you to create more complex and customized plots. This method is particularly useful when you need to work with different coordinate systems, apply custom transformations, or create non-standard plot layouts.
Remember that while get_transformed_clip_path_and_affine() provides powerful capabilities, it’s important to use it judiciously and in conjunction with other Matplotlib features to create clear and effective visualizations. Always consider the needs of your audience and the purpose of your plot when applying advanced techniques.