Comprehensive Guide to Using Matplotlib.artist.Artist.set_animated() in Python for Efficient Visualization
Matplotlib.artist.Artist.set_animated() in Python is a powerful method that plays a crucial role in creating efficient and dynamic visualizations. This article will delve deep into the intricacies of this function, exploring its various applications, benefits, and best practices. By the end of this comprehensive guide, you’ll have a thorough understanding of how to leverage Matplotlib.artist.Artist.set_animated() in Python to enhance your data visualization projects.
Understanding Matplotlib.artist.Artist.set_animated() in Python
Matplotlib.artist.Artist.set_animated() in Python is a method that belongs to the Artist class in Matplotlib. It is used to set the animated state of an artist object. When an artist is set to be animated, it tells Matplotlib that this particular artist will be updated frequently in an animation. This can significantly improve the performance of animations by allowing Matplotlib to optimize the rendering process.
Let’s start with a simple example to illustrate how to use Matplotlib.artist.Artist.set_animated() in Python:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 2*np.pi, 100)
line, = ax.plot(x, np.sin(x))
line.set_animated(True)
plt.title('How to use set_animated() - how2matplotlib.com')
plt.show()
Output:
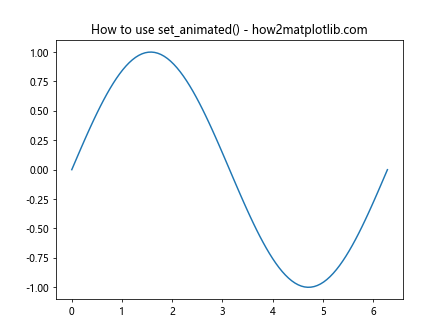
In this example, we create a simple sine wave plot and set the line artist to be animated using the set_animated() method. This tells Matplotlib that this line will be frequently updated in an animation.
The Importance of Matplotlib.artist.Artist.set_animated() in Python
Matplotlib.artist.Artist.set_animated() in Python is particularly important when creating animations or interactive plots. By setting an artist as animated, you’re essentially giving Matplotlib a hint that this artist will be frequently updated. This allows Matplotlib to optimize its rendering process, potentially leading to significant performance improvements.
Here’s an example that demonstrates the importance of using set_animated() in an animation:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 2*np.pi, 100)
line, = ax.plot(x, np.sin(x))
line.set_animated(True)
def animate(frame):
line.set_ydata(np.sin(x + frame/10))
return line,
ani = animation.FuncAnimation(fig, animate, frames=100, interval=50, blit=True)
plt.title('Animation with set_animated() - how2matplotlib.com')
plt.show()
Output:
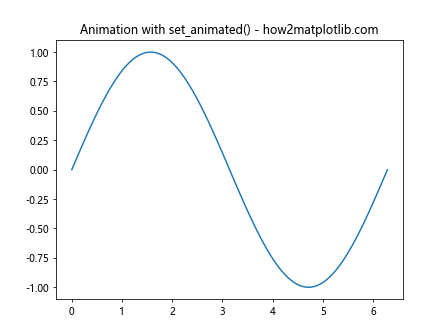
In this animation, we’re updating the y-data of the line in each frame. By setting the line as animated, we’re telling Matplotlib that this line will be updated frequently, allowing for more efficient rendering.
How Matplotlib.artist.Artist.set_animated() in Python Works
When you call Matplotlib.artist.Artist.set_animated() in Python with a value of True, you’re essentially telling Matplotlib that this artist will be updated frequently. This information is used by Matplotlib’s blitting system, which is an optimization technique for animations.
Blitting works by saving a background image of the static parts of the plot and only redrawing the parts that have changed. When an artist is set as animated, Matplotlib knows that this artist is likely to change frequently and can optimize its rendering accordingly.
Here’s an example that demonstrates how set_animated() works with blitting:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 2*np.pi, 100)
line, = ax.plot(x, np.sin(x))
line.set_animated(True)
def init():
ax.set_ylim(-1.1, 1.1)
return line,
def animate(frame):
line.set_ydata(np.sin(x + frame/10))
return line,
ani = animation.FuncAnimation(fig, animate, init_func=init, frames=100, interval=50, blit=True)
plt.title('Blitting with set_animated() - how2matplotlib.com')
plt.show()
Output:
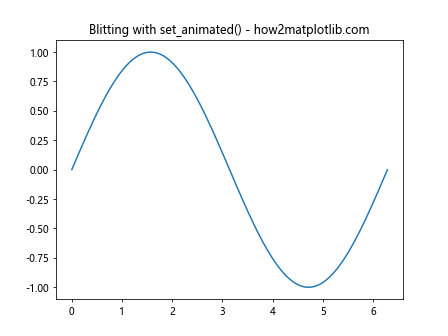
In this example, we’re using the FuncAnimation class with blitting enabled. The line is set as animated, which allows Matplotlib to optimize the animation rendering.
Benefits of Using Matplotlib.artist.Artist.set_animated() in Python
Using Matplotlib.artist.Artist.set_animated() in Python can provide several benefits:
- Improved Performance: By setting artists as animated, you can significantly improve the performance of animations, especially for complex plots with many elements.
Smoother Animations: The optimizations enabled by set_animated() can lead to smoother animations, particularly on less powerful hardware.
Reduced CPU Usage: By minimizing the amount of redrawing required, set_animated() can help reduce CPU usage during animations.
Better Control: It gives you more fine-grained control over which elements of your plot are updated frequently and which are static.
Here’s an example that demonstrates these benefits by creating a more complex animation: