Comprehensive Guide to Using Matplotlib.artist.Artist.remove_callback() in Python
Matplotlib.artist.Artist.remove_callback() in Python is an essential method for managing callbacks in Matplotlib, a popular data visualization library. This article will delve deep into the intricacies of this method, providing a thorough understanding of its usage, applications, and best practices. We’ll explore various aspects of Matplotlib.artist.Artist.remove_callback() in Python, including its syntax, parameters, return values, and practical examples.
Understanding Matplotlib.artist.Artist.remove_callback() in Python
Matplotlib.artist.Artist.remove_callback() in Python is a method that belongs to the Artist class in Matplotlib. It is used to remove a previously added callback from an artist object. Callbacks in Matplotlib are functions that are called when certain events occur, such as when a figure is drawn or when properties of an artist are changed. The ability to remove callbacks is crucial for managing the behavior of Matplotlib plots and preventing unwanted side effects.
Let’s start with a simple example to illustrate the basic usage of Matplotlib.artist.Artist.remove_callback() in Python:
import matplotlib.pyplot as plt
def callback(event):
print("Callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(callback)
line.remove_callback(cid)
plt.show()
Output:
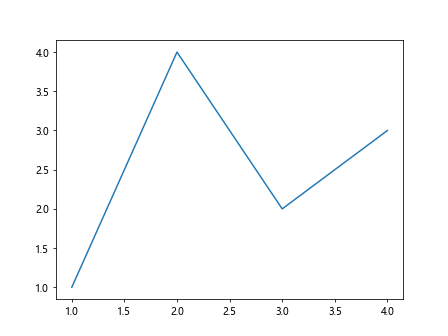
In this example, we first define a callback function that prints a message. We then create a plot with a single line and add the callback to the line artist. Finally, we use Matplotlib.artist.Artist.remove_callback() in Python to remove the callback. When you run this code, you’ll notice that the callback is not triggered because it has been removed.
Syntax and Parameters of Matplotlib.artist.Artist.remove_callback() in Python
The syntax for Matplotlib.artist.Artist.remove_callback() in Python is straightforward:
Artist.remove_callback(oid)
The method takes a single parameter:
oid
: This is the callback id returned byadd_callback()
. It’s used to identify which callback should be removed.
Matplotlib.artist.Artist.remove_callback() in Python returns None
. It modifies the artist object in-place by removing the specified callback.
Let’s look at a more detailed example:
import matplotlib.pyplot as plt
def callback1(event):
print("Callback 1 triggered by how2matplotlib.com")
def callback2(event):
print("Callback 2 triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid1 = line.add_callback(callback1)
cid2 = line.add_callback(callback2)
line.remove_callback(cid1) # Remove only callback1
plt.show()
Output:
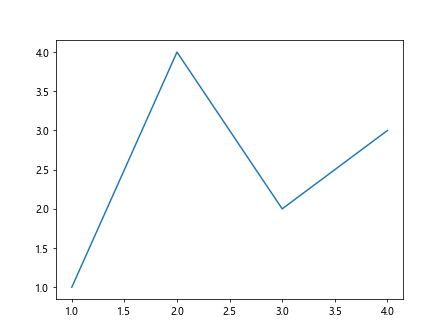
In this example, we add two callbacks to the line artist and then use Matplotlib.artist.Artist.remove_callback() in Python to remove only the first callback. When you run this code, only the second callback will be triggered.
Common Use Cases for Matplotlib.artist.Artist.remove_callback() in Python
Matplotlib.artist.Artist.remove_callback() in Python is particularly useful in several scenarios:
- Temporary callbacks: When you need to add a callback temporarily and remove it after a certain condition is met.
- Dynamic behavior: When you want to change the behavior of your plot dynamically during runtime.
- Cleanup: When you’re done with a callback and want to remove it to free up resources.
Let’s explore these use cases with examples:
Temporary Callbacks
import matplotlib.pyplot as plt
def temporary_callback(event):
print("Temporary callback triggered by how2matplotlib.com")
line.remove_callback(cid) # Remove itself after being called once
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(temporary_callback)
plt.show()
Output:
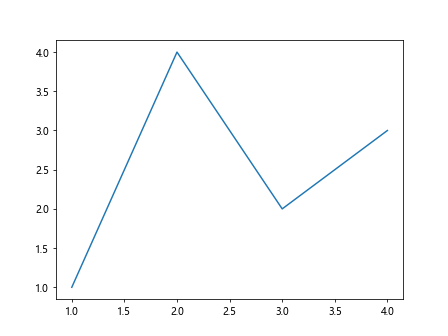
In this example, we create a temporary callback that removes itself after being called once. This is useful when you want a callback to be triggered only once.
Dynamic Behavior
import matplotlib.pyplot as plt
def callback_a(event):
print("Callback A triggered by how2matplotlib.com")
def callback_b(event):
print("Callback B triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid_a = line.add_callback(callback_a)
cid_b = line.add_callback(callback_b)
# Switch callbacks
line.remove_callback(cid_a)
line.add_callback(callback_a)
plt.show()
Output:
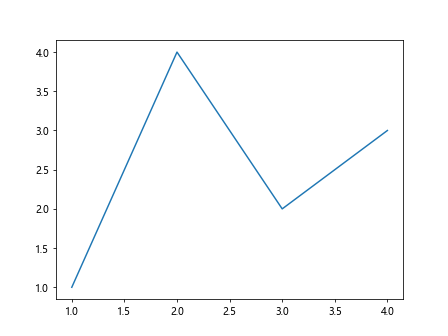
This example demonstrates how you can use Matplotlib.artist.Artist.remove_callback() in Python to dynamically change the order of callbacks.
Cleanup
import matplotlib.pyplot as plt
def cleanup_callback(event):
print("Cleanup callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(cleanup_callback)
# Do some operations...
line.remove_callback(cid) # Cleanup
plt.show()
Output:
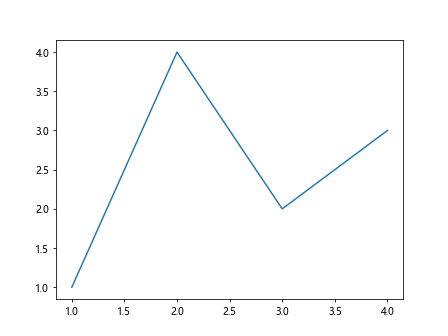
In this example, we add a callback and then remove it as part of a cleanup process. This is useful when you want to ensure that callbacks are properly removed when they’re no longer needed.
Advanced Usage of Matplotlib.artist.Artist.remove_callback() in Python
While the basic usage of Matplotlib.artist.Artist.remove_callback() in Python is straightforward, there are some advanced techniques and considerations to keep in mind:
Removing Multiple Callbacks
import matplotlib.pyplot as plt
def callback1(event):
print("Callback 1 triggered by how2matplotlib.com")
def callback2(event):
print("Callback 2 triggered by how2matplotlib.com")
def callback3(event):
print("Callback 3 triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cids = [line.add_callback(cb) for cb in [callback1, callback2, callback3]]
for cid in cids:
line.remove_callback(cid)
plt.show()
Output:
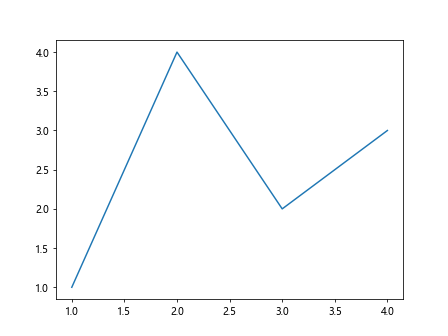
This example shows how to remove multiple callbacks efficiently using a loop.
Conditional Callback Removal
import matplotlib.pyplot as plt
def conditional_callback(event):
print("Conditional callback triggered by how2matplotlib.com")
if some_condition:
line.remove_callback(cid)
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
some_condition = True
cid = line.add_callback(conditional_callback)
plt.show()
Output:
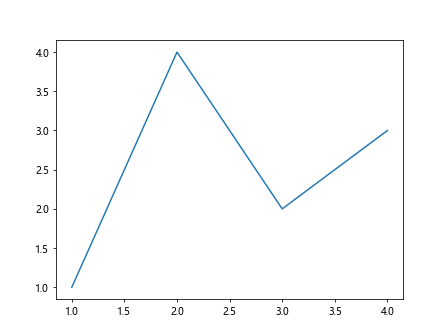
This example demonstrates how to remove a callback based on a condition within the callback itself.
Error Handling
import matplotlib.pyplot as plt
def error_prone_callback(event):
print("Error-prone callback triggered by how2matplotlib.com")
raise Exception("Simulated error")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(error_prone_callback)
try:
# Simulate callback triggering
line.stale = True
fig.canvas.draw()
except Exception as e:
print(f"Error occurred: {e}")
line.remove_callback(cid)
plt.show()
Output:
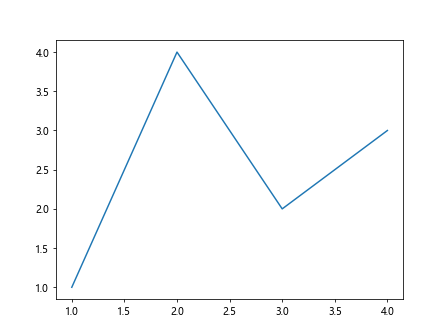
This example shows how to handle errors that might occur in callbacks and remove them if necessary.
Best Practices for Using Matplotlib.artist.Artist.remove_callback() in Python
When working with Matplotlib.artist.Artist.remove_callback() in Python, it’s important to follow some best practices to ensure your code is efficient, maintainable, and bug-free:
- Always store callback IDs: When adding callbacks, always store the returned callback ID. This is necessary for removing the callback later.
import matplotlib.pyplot as plt
def my_callback(event):
print("Callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(my_callback) # Store the callback ID
# Later, when you want to remove the callback
line.remove_callback(cid)
plt.show()
Output:
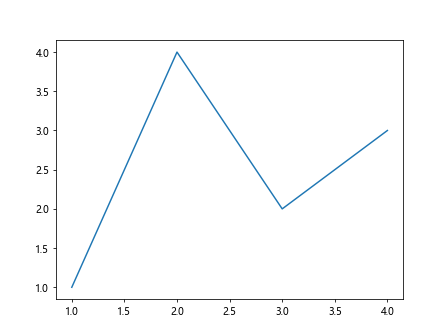
- Remove callbacks when they’re no longer needed: This helps prevent memory leaks and unexpected behavior.
import matplotlib.pyplot as plt
def temporary_callback(event):
print("Temporary callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(temporary_callback)
# After using the callback
line.remove_callback(cid)
plt.show()
Output:
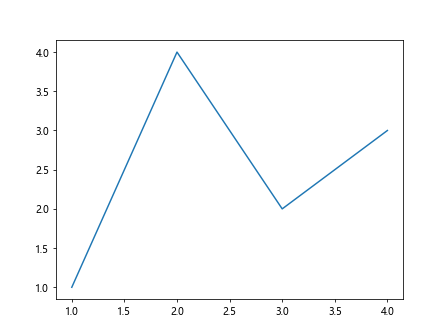
- Use try-except blocks when removing callbacks: This can help prevent errors if the callback has already been removed or if the callback ID is invalid.
import matplotlib.pyplot as plt
def my_callback(event):
print("Callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(my_callback)
try:
line.remove_callback(cid)
except ValueError:
print("Callback already removed or invalid ID")
plt.show()
Output:
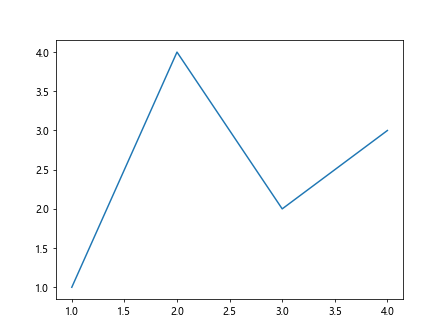
- Be careful with lambda functions: If you use lambda functions as callbacks, you won’t be able to remove them later because you can’t store their IDs.
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
# Bad practice: using lambda as callback
line.add_callback(lambda event: print("Lambda callback triggered by how2matplotlib.com"))
# Good practice: using named function as callback
def named_callback(event):
print("Named callback triggered by how2matplotlib.com")
cid = line.add_callback(named_callback)
line.remove_callback(cid) # This is possible with named functions
plt.show()
Output:
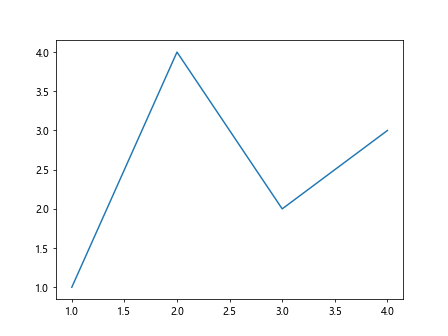
- Use meaningful names for callback functions: This makes your code more readable and maintainable.
import matplotlib.pyplot as plt
def update_title_on_click(event):
plt.title("Clicked! - how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = fig.canvas.mpl_connect('button_press_event', update_title_on_click)
plt.show()
Output:
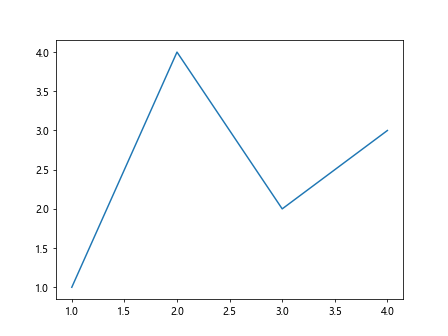
Common Pitfalls and How to Avoid Them
When using Matplotlib.artist.Artist.remove_callback() in Python, there are several common pitfalls that developers often encounter. Let’s discuss these and how to avoid them:
- Removing a non-existent callback: If you try to remove a callback that doesn’t exist, Matplotlib will raise a ValueError. Always check if the callback exists before removing it.
import matplotlib.pyplot as plt
def my_callback(event):
print("Callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(my_callback)
# Check if callback exists before removing
if cid in line.callbacks:
line.remove_callback(cid)
else:
print("Callback doesn't exist")
plt.show()
- Removing a callback multiple times: If you accidentally try to remove the same callback multiple times, it can lead to errors. Keep track of which callbacks have been removed.
import matplotlib.pyplot as plt
def my_callback(event):
print("Callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(my_callback)
# Remove callback and set cid to None
line.remove_callback(cid)
cid = None
# Check if cid is None before trying to remove again
if cid is not None:
line.remove_callback(cid)
plt.show()
Output:
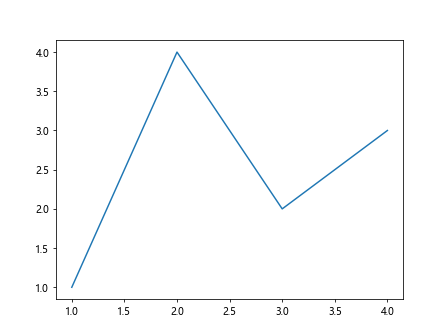
- Forgetting to remove callbacks: If you add callbacks but forget to remove them, it can lead to memory leaks and unexpected behavior. Always remove callbacks when they’re no longer needed.
import matplotlib.pyplot as plt
class CallbackManager:
def __init__(self):
self.callbacks = []
def add_callback(self, artist, callback):
cid = artist.add_callback(callback)
self.callbacks.append((artist, cid))
def remove_all_callbacks(self):
for artist, cid in self.callbacks:
artist.remove_callback(cid)
self.callbacks.clear()
manager = CallbackManager()
def my_callback(event):
print("Callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
manager.add_callback(line, my_callback)
# Later, when you're done with all callbacks
manager.remove_all_callbacks()
plt.show()
Output:
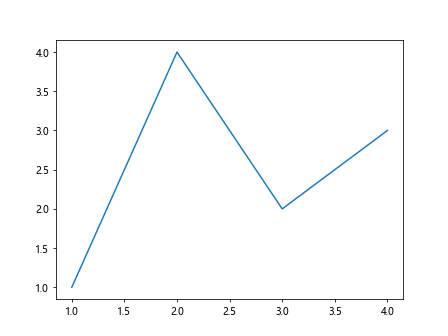
- Removing callbacks from the wrong artist: Make sure you’re removing the callback from the correct artist object.
import matplotlib.pyplot as plt
def callback1(event):
print("Callback 1 triggered by how2matplotlib.com")
def callback2(event):
print("Callback 2 triggered by how2matplotlib.com")
fig, (ax1, ax2) = plt.subplots(1, 2)
line1, = ax1.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
line2, = ax2.plot([1, 2, 3, 4], [3, 2, 4, 1], label='how2matplotlib.com')
cid1 = line1.add_callback(callback1)
cid2 = line2.add_callback(callback2)
# Correct: Remove callback from the right artist
line1.remove_callback(cid1)
line2.remove_callback(cid2)
# Incorrect: This would raise an error
# line1.remove_callback(cid2)
plt.show()
Output:
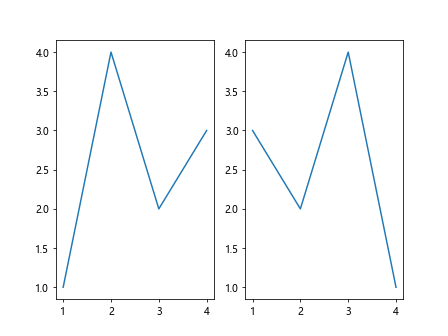
- Not handling exceptions when removing callbacks: Always wrap your remove_callback calls in try-except blocks to handle potential errors gracefully.
import matplotlib.pyplot as plt
def my_callback(event):
print("Callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid = line.add_callback(my_callback)
try:
line.remove_callback(cid)
except ValueError as e:
print(f"Error removing callback: {e}")
plt.show()
Output:
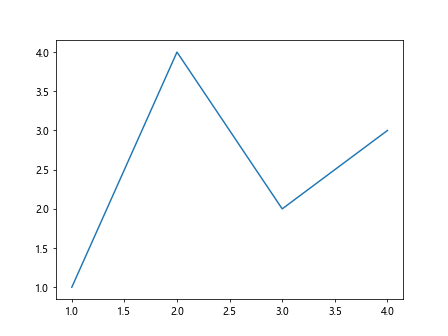
Advanced Techniques with Matplotlib.artist.Artist.remove_callback() in Python
Now that we’ve covered the basics and common pitfalls, let’s explore some advanced techniques for using Matplotlib.artist.Artist.remove_callback() in Python:
Callback Chaining
You can create a chain of callbacks where one callback adds or removes others based on certain conditions:
import matplotlib.pyplot as plt
def callback1(event):
print("Callback 1 triggered by how2matplotlib.com")
line.add_callback(callback2)
line.remove_callback(cid1)
def callback2(event):
print("Callback 2 triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
cid1 = line.add_callback(callback1)
plt.show()
Output:
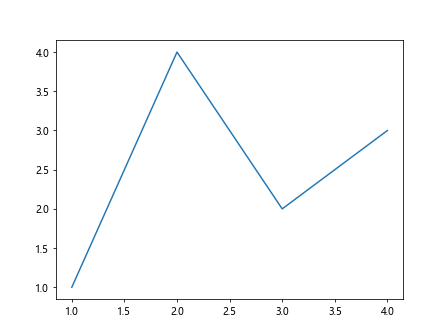
In this example, callback1 adds callback2 and then removes itself.
Dynamic Callback Management
You can create a system to dynamically manage callbacks based on the state of your application:
import matplotlib.pyplot as plt
class CallbackManager:
def __init__(self):
self.callbacks = {}
self.active_callbacks = set()
def add_callback(self, name, artist, callback):
cid = artist.add_callback(callback)
self.callbacks[name] = (artist, cid)
self.active_callbacks.add(name)
def remove_callback(self, name):
if name in self.active_callbacks:
artist, cid = self.callbacks[name]
artist.remove_callback(cid)
self.active_callbacks.remove(name)
def toggle_callback(self, name):
if name in self.active_callbacks:
self.remove_callback(name)
else:
artist, cid = self.callbacks[name]
artist.add_callback(cid)
self.active_callbacks.add(name)
manager = CallbackManager()
def callback1(event):
print("Callback 1 triggered by how2matplotlib.com")
def callback2(event):
print("Callback 2 triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
manager.add_callback('cb1', line, callback1)
manager.add_callback('cb2', line, callback2)
# Toggle callbacks on and off
manager.toggle_callback('cb1')
manager.toggle_callback('cb2')
plt.show()
Output:
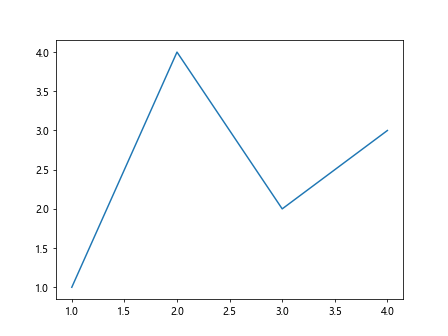
This CallbackManager class allows you to add, remove, and toggle callbacks dynamically.
Callback Prioritization
You can implement a system to prioritize callbacks and remove them based on their priority:
import matplotlib.pyplot as plt
from collections import OrderedDict
class PriorityCallbackManager:
def __init__(self):
self.callbacks = OrderedDict()
def add_callback(self, artist, callback, priority):
cid = artist.add_callback(callback)
self.callbacks[cid] = (artist, callback, priority)
self.callbacks = OrderedDict(sorted(self.callbacks.items(), key=lambda x: x[1][2], reverse=True))
def remove_lowest_priority(self):
if self.callbacks:
cid, (artist, _, _) = self.callbacks.popitem()
artist.remove_callback(cid)
manager = PriorityCallbackManager()
def callback1(event):
print("High priority callback triggered by how2matplotlib.com")
def callback2(event):
print("Medium priority callback triggered by how2matplotlib.com")
def callback3(event):
print("Low priority callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
manager.add_callback(line, callback1, 3)
manager.add_callback(line, callback2, 2)
manager.add_callback(line, callback3, 1)
# Remove the lowest priority callback
manager.remove_lowest_priority()
plt.show()
Output:
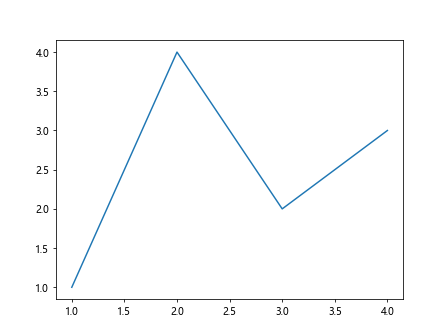
This PriorityCallbackManager class allows you to add callbacks with priorities and remove the lowest priority callback when needed.
Integrating Matplotlib.artist.Artist.remove_callback() with Other Matplotlib Features
Matplotlib.artist.Artist.remove_callback() in Python can be effectively integrated with other Matplotlib features to create more dynamic and interactive visualizations. Let’s explore some examples:
Combining with Event Handling
You can use Matplotlib.artist.Artist.remove_callback() in Python in conjunction with Matplotlib’s event handling system:
import matplotlib.pyplot as plt
def on_click(event):
if event.inaxes == ax:
print(f"Clicked at position ({event.xdata}, {event.ydata}) - how2matplotlib.com")
fig.canvas.mpl_disconnect(cid)
line.remove_callback(callback_id)
def line_callback(event):
print("Line callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
callback_id = line.add_callback(line_callback)
cid = fig.canvas.mpl_connect('button_press_event', on_click)
plt.show()
Output:
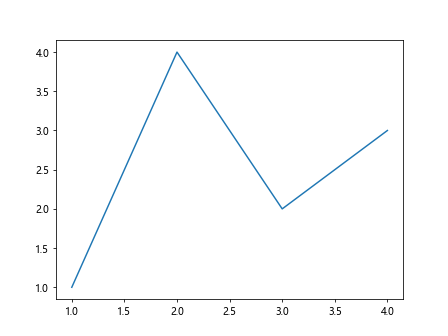
In this example, we combine event handling with Matplotlib.artist.Artist.remove_callback() in Python. When the user clicks on the plot, it disconnects the click event and removes the line callback.
Integrating with Animation
You can use Matplotlib.artist.Artist.remove_callback() in Python to create dynamic animations:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
line, = ax.plot([], [], label='how2matplotlib.com')
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
def init():
line.set_data([], [])
return line,
def animate(i):
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x + i/10.0)
line.set_data(x, y)
return line,
def callback(event):
print(f"Frame {event} animated by how2matplotlib.com")
callback_id = line.add_callback(callback)
anim = animation.FuncAnimation(fig, animate, init_func=init, frames=200, interval=20, blit=True)
# Remove callback after 100 frames
def remove_callback(frame):
if frame == 100:
line.remove_callback(callback_id)
print("Callback removed at frame 100")
anim.event_source.add_callback(remove_callback)
plt.show()
Output:
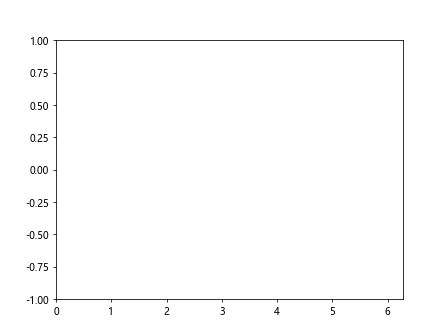
This example creates an animation and adds a callback to the line artist. The callback is then removed after 100 frames using Matplotlib.artist.Artist.remove_callback() in Python.
Working with Subplots
Matplotlib.artist.Artist.remove_callback() in Python can be used effectively with subplots:
import matplotlib.pyplot as plt
def callback1(event):
print("Callback 1 triggered by how2matplotlib.com")
def callback2(event):
print("Callback 2 triggered by how2matplotlib.com")
fig, (ax1, ax2) = plt.subplots(1, 2)
line1, = ax1.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
line2, = ax2.plot([1, 2, 3, 4], [3, 2, 4, 1], label='how2matplotlib.com')
cid1 = line1.add_callback(callback1)
cid2 = line2.add_callback(callback2)
def on_click(event):
if event.inaxes == ax1:
line1.remove_callback(cid1)
print("Callback removed from subplot 1")
elif event.inaxes == ax2:
line2.remove_callback(cid2)
print("Callback removed from subplot 2")
fig.canvas.mpl_connect('button_press_event', on_click)
plt.show()
Output:
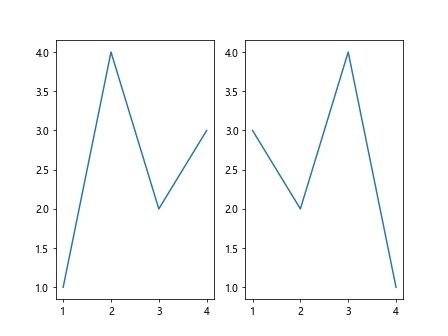
In this example, we create two subplots, each with its own callback. Clicking on a subplot removes the callback for that specific subplot using Matplotlib.artist.Artist.remove_callback() in Python.
Performance Considerations
When using Matplotlib.artist.Artist.remove_callback() in Python, it’s important to consider the performance implications, especially when dealing with large numbers of callbacks or frequent additions and removals:
- Avoid adding and removing callbacks frequently: Each call to add_callback() or remove_callback() involves modifying the artist’s internal data structures, which can be costly if done too often.
Use a callback manager: If you need to manage many callbacks, consider implementing a callback manager class that can efficiently handle additions and removals.
Be mindful of callback complexity: Complex callbacks can slow down your application, especially if they’re called frequently. Keep your callbacks as simple and efficient as possible.
Use conditional execution: Instead of adding and removing callbacks, you can use a flag to conditionally execute the callback code:
import matplotlib.pyplot as plt
class ConditionalCallback:
def __init__(self):
self.enabled = True
def __call__(self, event):
if self.enabled:
print("Conditional callback triggered by how2matplotlib.com")
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
callback = ConditionalCallback()
line.add_callback(callback)
# Instead of removing the callback, disable it
callback.enabled = False
plt.show()
Output: