Comprehensive Guide to Matplotlib.artist.Artist.is_transform_set() in Python
Matplotlib.artist.Artist.is_transform_set() in Python is a crucial method in the Matplotlib library that allows developers to check whether a transform has been set for an Artist object. This function is essential for managing and manipulating graphical elements in Matplotlib plots. In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.artist.Artist.is_transform_set(), its usage, and provide numerous examples to help you master this powerful tool.
Understanding Matplotlib.artist.Artist.is_transform_set() in Python
Matplotlib.artist.Artist.is_transform_set() is a method that belongs to the Artist class in Matplotlib. It returns a boolean value indicating whether a transform has been set for the Artist object. This method is particularly useful when working with complex visualizations that involve multiple layers and transformations.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.is_transform_set():
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.2)
ax.add_patch(circle)
print(f"Is transform set for circle? {circle.is_transform_set()}")
plt.title("How2Matplotlib.com: Checking Transform")
plt.show()
Output:
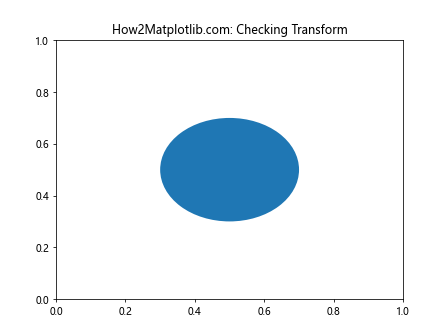
In this example, we create a circle patch and add it to the axes. Then, we use the is_transform_set() method to check if a transform has been set for the circle. The result will be printed to the console.
The Importance of Transforms in Matplotlib
Transforms play a crucial role in Matplotlib as they define how the coordinates of an Artist object are mapped to the final plot. Understanding and properly using transforms is essential for creating accurate and visually appealing visualizations.
Here’s an example that demonstrates the importance of transforms:
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
fig, ax = plt.subplots()
rect = plt.Rectangle((0.2, 0.2), 0.3, 0.4, fill=False)
ax.add_patch(rect)
print(f"Is transform set for rectangle? {rect.is_transform_set()}")
# Apply a transform
t = transforms.Affine2D().rotate_deg(45) + ax.transData
rect.set_transform(t)
print(f"Is transform set after rotation? {rect.is_transform_set()}")
plt.title("How2Matplotlib.com: Applying Transforms")
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.show()
Output:
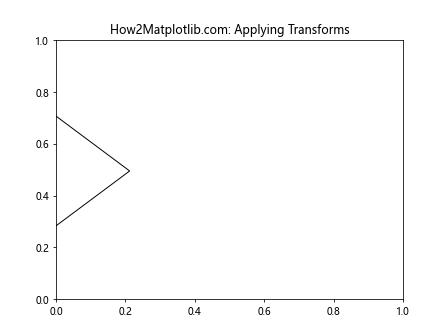
In this example, we create a rectangle and check if a transform is set. Then, we apply a rotation transform and check again. This demonstrates how Matplotlib.artist.Artist.is_transform_set() can be used to verify the application of transforms.
Using Matplotlib.artist.Artist.is_transform_set() with Different Artist Types
Matplotlib.artist.Artist.is_transform_set() can be used with various types of Artist objects, including patches, lines, and text. Let’s explore how to use this method with different Artist types:
Patches
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.2)
rectangle = patches.Rectangle((0.1, 0.1), 0.3, 0.3)
ax.add_patch(circle)
ax.add_patch(rectangle)
print(f"Is transform set for circle? {circle.is_transform_set()}")
print(f"Is transform set for rectangle? {rectangle.is_transform_set()}")
plt.title("How2Matplotlib.com: Checking Transforms for Patches")
plt.show()
Output:
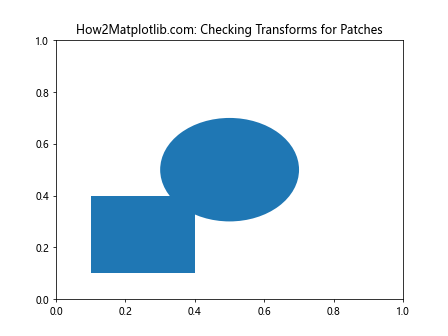
This example demonstrates how to use Matplotlib.artist.Artist.is_transform_set() with different types of patches.
Lines
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line = ax.plot([0, 1], [0, 1])[0]
print(f"Is transform set for line? {line.is_transform_set()}")
plt.title("How2Matplotlib.com: Checking Transform for Line")
plt.show()
Output:
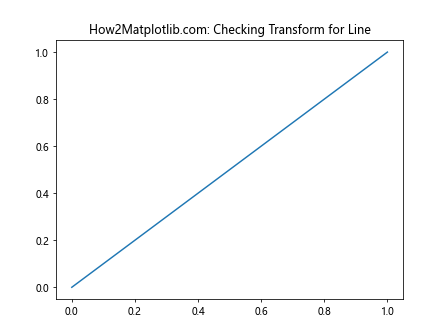
Here, we create a line and check if a transform is set for it using Matplotlib.artist.Artist.is_transform_set().
Text
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
text = ax.text(0.5, 0.5, "How2Matplotlib.com", ha='center', va='center')
print(f"Is transform set for text? {text.is_transform_set()}")
plt.title("How2Matplotlib.com: Checking Transform for Text")
plt.show()
Output:
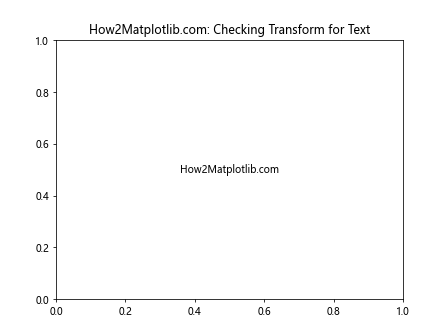
This example shows how to use Matplotlib.artist.Artist.is_transform_set() with text objects.
Combining Transforms and Checking with Matplotlib.artist.Artist.is_transform_set()
Matplotlib allows you to combine multiple transforms to create complex transformations. Let’s see how we can use Matplotlib.artist.Artist.is_transform_set() to verify these combined transforms:
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
fig, ax = plt.subplots()
rect = plt.Rectangle((0.2, 0.2), 0.3, 0.4, fill=False)
ax.add_patch(rect)
print(f"Is transform set initially? {rect.is_transform_set()}")
# Combine transforms
t1 = transforms.Affine2D().rotate_deg(45)
t2 = transforms.Affine2D().scale(1.5)
t3 = ax.transData
combined_transform = t1 + t2 + t3
rect.set_transform(combined_transform)
print(f"Is transform set after combination? {rect.is_transform_set()}")
plt.title("How2Matplotlib.com: Combined Transforms")
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.show()
Output:
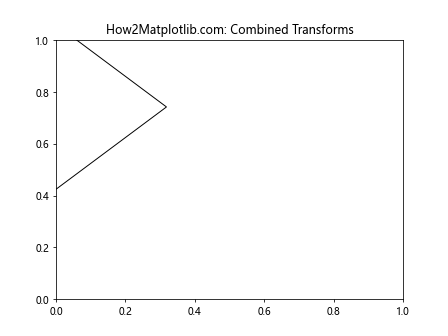
In this example, we combine rotation, scaling, and data transforms, then use Matplotlib.artist.Artist.is_transform_set() to verify if the combined transform has been set.
Resetting Transforms and Using Matplotlib.artist.Artist.is_transform_set()
Sometimes, you may want to reset a transform to its default state. Let’s see how we can use Matplotlib.artist.Artist.is_transform_set() to verify this process:
import matplotlib.pyplot as plt
import matplotlib.transforms as transforms
fig, ax = plt.subplots()
circle = plt.Circle((0.5, 0.5), 0.2, fill=False)
ax.add_patch(circle)
print(f"Is transform set initially? {circle.is_transform_set()}")
# Apply a transform
t = transforms.Affine2D().scale(2) + ax.transData
circle.set_transform(t)
print(f"Is transform set after scaling? {circle.is_transform_set()}")
# Reset transform
circle.set_transform(ax.transData)
print(f"Is transform set after reset? {circle.is_transform_set()}")
plt.title("How2Matplotlib.com: Resetting Transforms")
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.show()
Output:
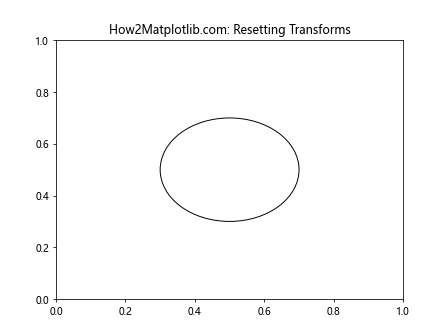
This example demonstrates how to reset a transform and use Matplotlib.artist.Artist.is_transform_set() to verify the reset.
Using Matplotlib.artist.Artist.is_transform_set() in Animation
Matplotlib.artist.Artist.is_transform_set() can be particularly useful when working with animations. Let’s create a simple animation and use this method to check the transform status:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
line, = ax.plot([], [], lw=2)
def init():
line.set_data([], [])
return line,
def animate(i):
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x + i/10)
line.set_data(x, y)
print(f"Frame {i}: Is transform set? {line.is_transform_set()}")
return line,
anim = animation.FuncAnimation(fig, animate, init_func=init, frames=50, interval=100, blit=True)
plt.title("How2Matplotlib.com: Animation and Transforms")
plt.show()
Output:
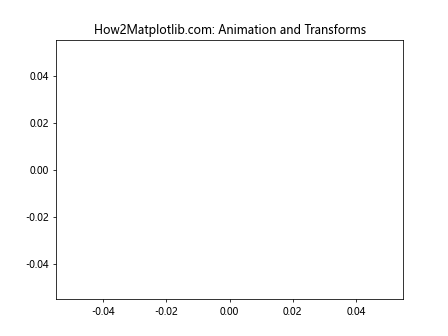
In this animation, we use Matplotlib.artist.Artist.is_transform_set() to check the transform status of the line in each frame.
Matplotlib.artist.Artist.is_transform_set() and Subplots
When working with subplots, it’s important to understand how transforms are applied. Let’s use Matplotlib.artist.Artist.is_transform_set() to explore this:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
circle1 = patches.Circle((0.5, 0.5), 0.2)
circle2 = patches.Circle((0.5, 0.5), 0.2)
ax1.add_patch(circle1)
ax2.add_patch(circle2)
print(f"Is transform set for circle1? {circle1.is_transform_set()}")
print(f"Is transform set for circle2? {circle2.is_transform_set()}")
plt.suptitle("How2Matplotlib.com: Transforms in Subplots")
plt.show()
Output:
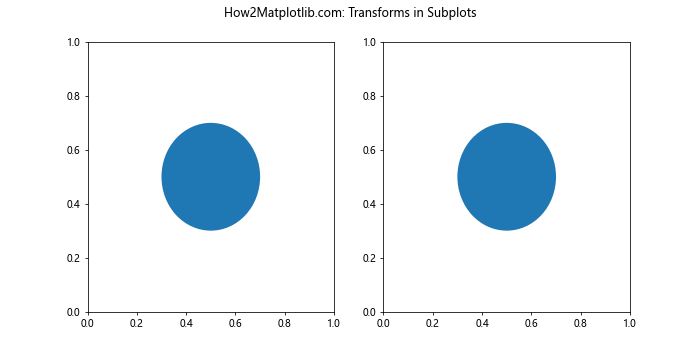
This example demonstrates how to use Matplotlib.artist.Artist.is_transform_set() with subplots.
Matplotlib.artist.Artist.is_transform_set() and Polar Plots
Polar plots use a different coordinate system, which affects how transforms are applied. Let’s explore this using Matplotlib.artist.Artist.is_transform_set():
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots(subplot_kw=dict(projection='polar'))
theta = np.linspace(0, 2*np.pi, 100)
r = np.sin(2*theta)
line, = ax.plot(theta, r)
print(f"Is transform set for polar line? {line.is_transform_set()}")
plt.title("How2Matplotlib.com: Transforms in Polar Plots")
plt.show()
Output:
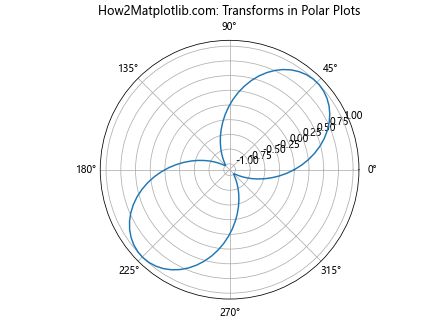
This example demonstrates how to use Matplotlib.artist.Artist.is_transform_set() with polar plots.
Matplotlib.artist.Artist.is_transform_set() and 3D Plots
3D plots introduce additional complexity to transforms. Let’s see how Matplotlib.artist.Artist.is_transform_set() works in this context:
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import numpy as np
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
surface = ax.plot_surface(X, Y, Z)
print(f"Is transform set for 3D surface? {surface.is_transform_set()}")
plt.title("How2Matplotlib.com: Transforms in 3D Plots")
plt.show()
Output:
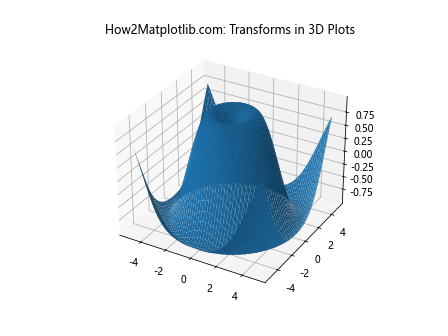
This example shows how to use Matplotlib.artist.Artist.is_transform_set() with 3D plots.
Conclusion
Matplotlib.artist.Artist.is_transform_set() in Python is a powerful tool for managing and verifying transforms in Matplotlib. Throughout this comprehensive guide, we’ve explored various aspects of this method, including its usage with different Artist types, combined transforms, animations, subplots, custom transforms, and specialized plot types like polar and 3D plots.