Comprehensive Guide to Matplotlib.artist.Artist.get_visible() in Python
Matplotlib.artist.Artist.get_visible() in Python is a crucial method for managing the visibility of artists in Matplotlib plots. This function is an essential tool for developers working with data visualization in Python, allowing them to dynamically control which elements are displayed in their plots. In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.artist.Artist.get_visible(), providing detailed explanations and practical examples to help you master this powerful feature.
Understanding Matplotlib.artist.Artist.get_visible() in Python
Matplotlib.artist.Artist.get_visible() in Python is a method that belongs to the Artist class in Matplotlib. This method is used to retrieve the visibility status of an artist object. An artist in Matplotlib can be any graphical element in a plot, such as lines, markers, text, or shapes. The get_visible() method returns a boolean value indicating whether the artist is currently visible or not.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.get_visible() in Python:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot a line
line, = ax.plot([0, 1, 2, 3], [0, 1, 0, 1], label='how2matplotlib.com')
# Get the visibility status of the line
is_visible = line.get_visible()
print(f"Is the line visible? {is_visible}")
plt.show()
Output:
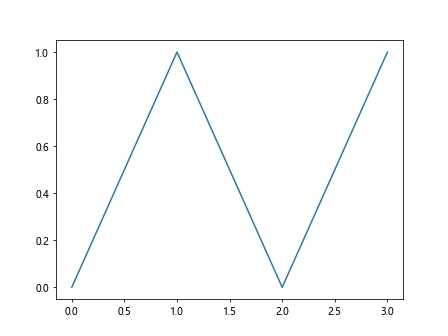
In this example, we create a simple line plot and then use the get_visible() method to check if the line is visible. By default, all artists are visible when created, so this will return True.
The Importance of Matplotlib.artist.Artist.get_visible() in Python
Matplotlib.artist.Artist.get_visible() in Python plays a crucial role in creating interactive and dynamic visualizations. It allows developers to query the current visibility state of any artist object, which is particularly useful when working with complex plots or when implementing user interactions.
Here’s an example that demonstrates the importance of Matplotlib.artist.Artist.get_visible() in Python:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
# Create multiple lines
line1, = ax.plot([0, 1, 2, 3], [0, 1, 0, 1], label='Line 1 - how2matplotlib.com')
line2, = ax.plot([0, 1, 2, 3], [1, 0, 1, 0], label='Line 2 - how2matplotlib.com')
# Toggle visibility of line2
line2.set_visible(False)
# Check visibility of both lines
print(f"Is line1 visible? {line1.get_visible()}")
print(f"Is line2 visible? {line2.get_visible()}")
plt.legend()
plt.show()
Output:
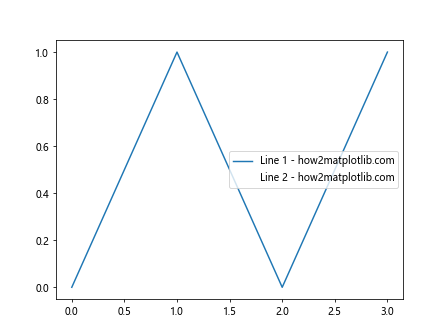
In this example, we create two lines and then hide one of them using the set_visible() method. We then use get_visible() to check the visibility status of both lines, demonstrating how this method can be used to manage multiple artists in a plot.
Practical Applications of Matplotlib.artist.Artist.get_visible() in Python
Matplotlib.artist.Artist.get_visible() in Python has numerous practical applications in data visualization. It’s particularly useful when creating interactive plots, implementing custom legend behavior, or managing complex visualizations with multiple layers.
Let’s explore some practical applications:
1. Creating Toggle Buttons for Plot Elements
import matplotlib.pyplot as plt
from matplotlib.widgets import CheckButtons
fig, ax = plt.subplots()
# Create multiple lines
x = range(10)
line1, = ax.plot(x, [i**2 for i in x], label='Square - how2matplotlib.com')
line2, = ax.plot(x, [i**3 for i in x], label='Cube - how2matplotlib.com')
# Create check buttons
rax = plt.axes([0.05, 0.4, 0.1, 0.15])
check = CheckButtons(rax, ('Square', 'Cube'), (True, True))
def func(label):
if label == 'Square':
line1.set_visible(not line1.get_visible())
elif label == 'Cube':
line2.set_visible(not line2.get_visible())
plt.draw()
check.on_clicked(func)
plt.show()
Output:
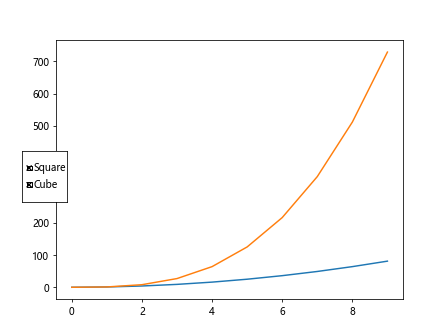
In this example, we create a plot with two lines and add check buttons to toggle their visibility. The get_visible() method is used to determine the current visibility state of each line when toggling.
2. Implementing Custom Legend Behavior
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
# Create multiple lines
line1, = ax.plot([0, 1, 2, 3], [0, 1, 0, 1], label='Line 1 - how2matplotlib.com')
line2, = ax.plot([0, 1, 2, 3], [1, 0, 1, 0], label='Line 2 - how2matplotlib.com')
# Create a custom legend
legend = ax.legend()
# Function to toggle visibility when clicking on legend
def on_pick(event):
legend = event.artist
isVisible = legend.get_visible()
legend.set_visible(not isVisible)
for line in [line1, line2]:
if line.get_label() == legend.get_text():
line.set_visible(not line.get_visible())
fig.canvas.draw()
# Make legend pickable
for legend_line in legend.get_lines():
legend_line.set_picker(5)
fig.canvas.mpl_connect('pick_event', on_pick)
plt.show()
Output:
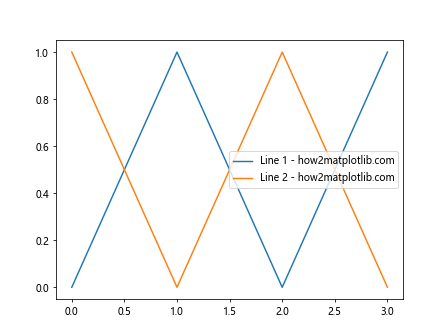
This example demonstrates how to create a custom legend where clicking on a legend item toggles the visibility of the corresponding line. The get_visible() method is used to check the current visibility state of both the legend items and the lines.
Advanced Techniques with Matplotlib.artist.Artist.get_visible() in Python
Matplotlib.artist.Artist.get_visible() in Python can be combined with other Matplotlib features to create more complex and interactive visualizations. Let’s explore some advanced techniques:
1. Animating Visibility Changes
import matplotlib.pyplot as plt
import matplotlib.animation as animation
fig, ax = plt.subplots()
# Create multiple lines
x = range(10)
lines = [ax.plot(x, [i**n for i in x], label=f'Line {n} - how2matplotlib.com')[0] for n in range(1, 5)]
def animate(frame):
for i, line in enumerate(lines):
line.set_visible((frame + i) % len(lines) < 2)
return lines
ani = animation.FuncAnimation(fig, animate, frames=20, interval=500, blit=True)
plt.legend()
plt.show()
Output:
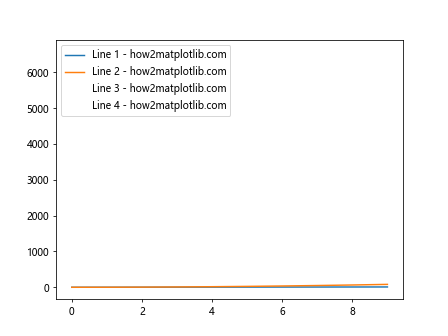
This example creates an animation where the visibility of multiple lines changes over time. The get_visible() method is implicitly used by the animation framework to determine which artists need to be redrawn.
2. Implementing a Visibility Stack
import matplotlib.pyplot as plt
class VisibilityStack:
def __init__(self, artists):
self.artists = artists
self.stack = []
def push(self):
self.stack.append([artist.get_visible() for artist in self.artists])
def pop(self):
if self.stack:
visibilities = self.stack.pop()
for artist, visibility in zip(self.artists, visibilities):
artist.set_visible(visibility)
fig, ax = plt.subplots()
# Create multiple lines
lines = [ax.plot([0, 1, 2, 3], [i, i+1, i, i+1], label=f'Line {i} - how2matplotlib.com')[0] for i in range(3)]
stack = VisibilityStack(lines)
# Push current visibility state
stack.push()
# Change visibility
lines[0].set_visible(False)
lines[2].set_visible(False)
# Push new visibility state
stack.push()
# Restore previous state
stack.pop()
for line in lines:
print(f"{line.get_label()} is visible: {line.get_visible()}")
plt.legend()
plt.show()
Output:
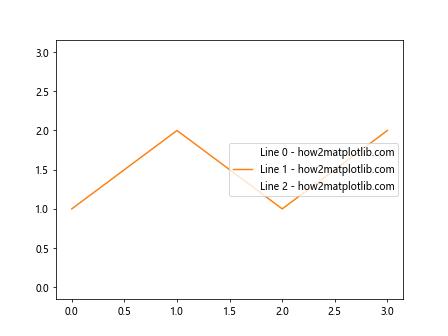
This advanced example implements a visibility stack that allows you to save and restore the visibility state of multiple artists. The get_visible() method is used to capture the current visibility state before making changes.
Best Practices for Using Matplotlib.artist.Artist.get_visible() in Python
When working with Matplotlib.artist.Artist.get_visible() in Python, it's important to follow some best practices to ensure your code is efficient and maintainable:
- Use get_visible() sparingly: Avoid calling get_visible() in tight loops or frequently executed code, as it may impact performance.
Combine with set_visible(): Often, get_visible() is used in conjunction with set_visible() to toggle visibility. Consider using a toggle function that encapsulates both operations.
Cache visibility states: If you need to frequently check the visibility of many artists, consider caching the visibility states in a separate data structure for quicker access.
Use with other Artist methods: Combine get_visible() with other Artist methods like get_label() or get_zorder() for more complex visibility management.
Handle visibility in batch: When working with many artists, try to handle visibility changes in batch operations rather than individually.
Here's an example demonstrating these best practices: