How to Use Matplotlib.artist.Artist.get_snap() in Python
Matplotlib.artist.Artist.get_snap() in Python is an essential method for working with artists in Matplotlib. This comprehensive guide will explore the various aspects of using get_snap() and its applications in creating visualizations. We’ll dive deep into the functionality, usage, and best practices of this method, providing numerous examples to illustrate its capabilities.
Understanding Matplotlib.artist.Artist.get_snap() in Python
Matplotlib.artist.Artist.get_snap() is a method that belongs to the Artist class in Matplotlib. It is used to retrieve the snap setting of an artist. The snap setting determines whether the artist should snap to pixel boundaries when rendering. This can be particularly useful for creating crisp and precise visualizations, especially when working with line plots or other geometric shapes.
Let’s start with a simple example to demonstrate how to use get_snap():
import matplotlib.pyplot as plt
import matplotlib.lines as lines
fig, ax = plt.subplots()
line = lines.Line2D([0, 1], [0, 1], label='how2matplotlib.com')
ax.add_line(line)
snap_setting = line.get_snap()
print(f"Snap setting: {snap_setting}")
plt.show()
Output:
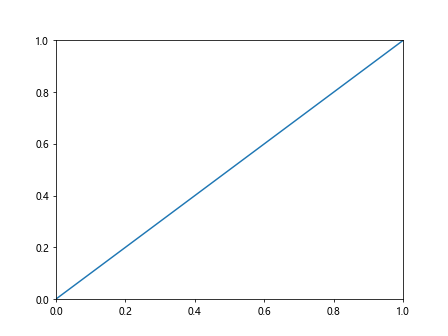
In this example, we create a simple line and add it to the plot. We then use get_snap() to retrieve the snap setting of the line. The snap setting is typically None by default, which means the artist will use the default snapping behavior.
Exploring the Functionality of get_snap()
The get_snap() method is a simple getter function that returns the current snap setting of an artist. It doesn’t take any arguments and returns either a boolean value or None. Let’s examine the possible return values:
- True: The artist will snap to pixel boundaries.
- False: The artist will not snap to pixel boundaries.
- None: The artist will use the default snapping behavior.
Here’s an example that demonstrates how to check the snap setting for different types of artists:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
line = plt.Line2D([0, 1], [0, 1], label='Line (how2matplotlib.com)')
circle = patches.Circle((0.5, 0.5), 0.2, label='Circle (how2matplotlib.com)')
rectangle = patches.Rectangle((0.2, 0.2), 0.3, 0.3, label='Rectangle (how2matplotlib.com)')
ax.add_line(line)
ax.add_patch(circle)
ax.add_patch(rectangle)
print(f"Line snap setting: {line.get_snap()}")
print(f"Circle snap setting: {circle.get_snap()}")
print(f"Rectangle snap setting: {rectangle.get_snap()}")
plt.legend()
plt.show()
Output:
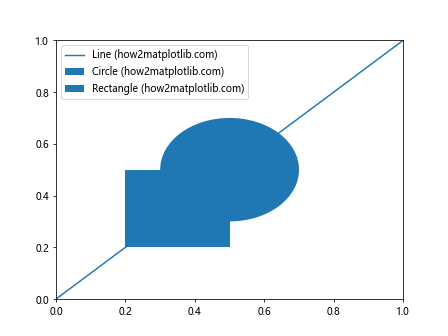
This example creates different types of artists (line, circle, and rectangle) and checks their snap settings using get_snap(). By default, all these artists will have a snap setting of None.
Using get_snap() with Different Artist Types
Matplotlib.artist.Artist.get_snap() can be used with various types of artists in Matplotlib. Let’s explore how to use it with different artist types and understand its behavior.
Lines
Lines are one of the most common artist types in Matplotlib. Here’s an example of using get_snap() with different line styles:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
line1 = ax.plot(x, y1, label='Sine (how2matplotlib.com)')[0]
line2 = ax.plot(x, y2, linestyle='--', label='Cosine (how2matplotlib.com)')[0]
print(f"Sine line snap setting: {line1.get_snap()}")
print(f"Cosine line snap setting: {line2.get_snap()}")
plt.legend()
plt.show()
Output:
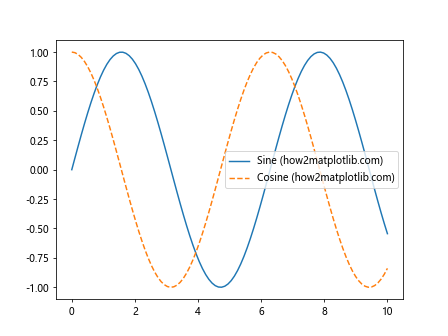
In this example, we create two lines with different styles and check their snap settings using get_snap(). Both lines will have a snap setting of None by default.
Markers
Markers are often used in scatter plots or to highlight specific points on a line. Let’s see how get_snap() works with markers:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 20)
y = np.sin(x)
scatter = ax.scatter(x, y, label='Scatter (how2matplotlib.com)')
line_with_markers = ax.plot(x, y, 'ro-', label='Line with markers (how2matplotlib.com)')[0]
print(f"Scatter snap setting: {scatter.get_snap()}")
print(f"Line with markers snap setting: {line_with_markers.get_snap()}")
plt.legend()
plt.show()
Output:
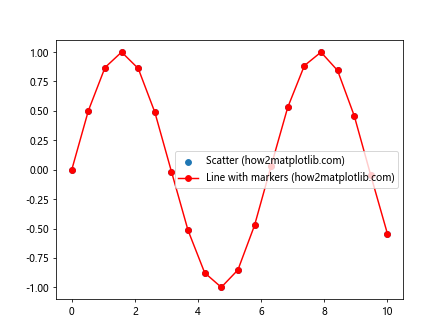
This example demonstrates how to use get_snap() with scatter plots and lines with markers. Both will have a snap setting of None by default.
Text
Text artists are used to add labels, titles, and annotations to plots. Let’s see how get_snap() behaves with text artists:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
text1 = ax.text(0.5, 0.5, 'Center Text (how2matplotlib.com)', ha='center', va='center')
text2 = ax.annotate('Annotation (how2matplotlib.com)', xy=(0.8, 0.8), xytext=(0.6, 0.6),
arrowprops=dict(arrowstyle='->'))
print(f"Text snap setting: {text1.get_snap()}")
print(f"Annotation snap setting: {text2.get_snap()}")
plt.show()
Output:
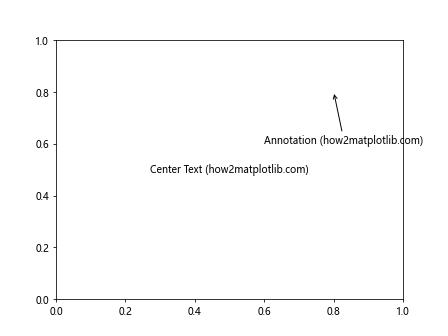
In this example, we create a regular text artist and an annotation, then check their snap settings using get_snap(). Both text artists will have a snap setting of None by default.
Combining get_snap() with Other Artist Methods
Matplotlib.artist.Artist.get_snap() can be used in conjunction with other artist methods to create more complex and customized visualizations. Let’s explore some examples of how to combine get_snap() with other methods.
Using get_snap() with set_snap()
The set_snap() method is the counterpart to get_snap() and allows you to set the snap setting for an artist. Here’s an example that demonstrates how to use these methods together:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.2, label='Circle (how2matplotlib.com)')
ax.add_patch(circle)
print(f"Initial snap setting: {circle.get_snap()}")
circle.set_snap(True)
print(f"After set_snap(True): {circle.get_snap()}")
circle.set_snap(False)
print(f"After set_snap(False): {circle.get_snap()}")
circle.set_snap(None)
print(f"After set_snap(None): {circle.get_snap()}")
plt.legend()
plt.show()
Output:
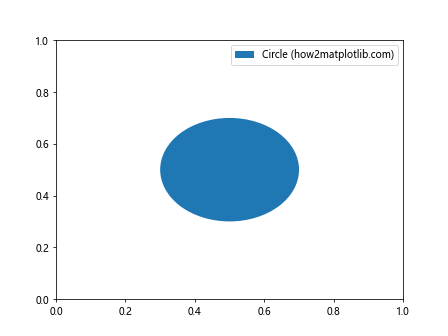
This example demonstrates how to use get_snap() to check the snap setting before and after using set_snap() to modify it.
Combining get_snap() with Visibility Settings
You can use get_snap() in combination with visibility settings to create dynamic visualizations. Here’s an example:
import matplotlib.pyplot as plt
import matplotlib.lines as lines
fig, ax = plt.subplots()
line1 = lines.Line2D([0, 1], [0, 1], label='Line 1 (how2matplotlib.com)')
line2 = lines.Line2D([0, 1], [1, 0], label='Line 2 (how2matplotlib.com)')
ax.add_line(line1)
ax.add_line(line2)
line2.set_visible(False)
print(f"Line 1 snap setting: {line1.get_snap()}, Visible: {line1.get_visible()}")
print(f"Line 2 snap setting: {line2.get_snap()}, Visible: {line2.get_visible()}")
plt.legend()
plt.show()
Output:
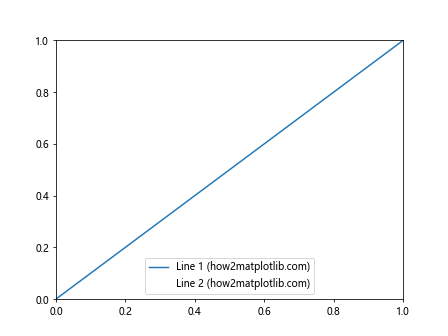
This example shows how to use get_snap() along with get_visible() to check both the snap setting and visibility of artists.
Advanced Usage of get_snap()
While the basic usage of Matplotlib.artist.Artist.get_snap() is straightforward, there are some advanced techniques and considerations to keep in mind when working with this method.
Using get_snap() in Custom Artist Classes
If you’re creating custom artist classes, you can implement the get_snap() method to provide custom snapping behavior. Here’s an example of a custom artist class that implements get_snap():
import matplotlib.pyplot as plt
from matplotlib.artist import Artist
class CustomArtist(Artist):
def __init__(self):
super().__init__()
self._snap = None
def draw(self, renderer):
# Custom drawing logic here
pass
def get_snap(self):
return self._snap
def set_snap(self, snap):
self._snap = snap
fig, ax = plt.subplots()
custom_artist = CustomArtist()
ax.add_artist(custom_artist)
print(f"Custom artist snap setting: {custom_artist.get_snap()}")
custom_artist.set_snap(True)
print(f"After set_snap(True): {custom_artist.get_snap()}")
plt.show()
Output:
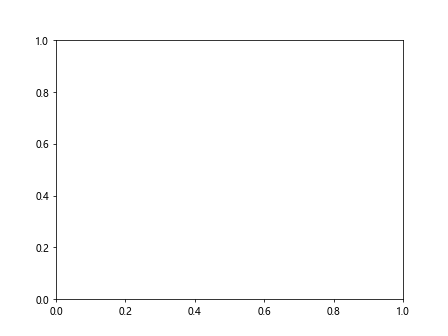
This example demonstrates how to create a custom artist class that implements the get_snap() method, allowing you to control the snapping behavior of your custom artists.
Using get_snap() with Compound Artists
Compound artists are artists that contain other artists. When working with compound artists, it’s important to understand how get_snap() behaves. Let’s look at an example using a legend, which is a compound artist:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
line1 = ax.plot(x, y1, label='Sine (how2matplotlib.com)')[0]
line2 = ax.plot(x, y2, label='Cosine (how2matplotlib.com)')[0]
legend = ax.legend()
print(f"Legend snap setting: {legend.get_snap()}")
print(f"Legend title snap setting: {legend.get_title().get_snap()}")
for text in legend.get_texts():
print(f"Legend text snap setting: {text.get_snap()}")
plt.show()
Output:
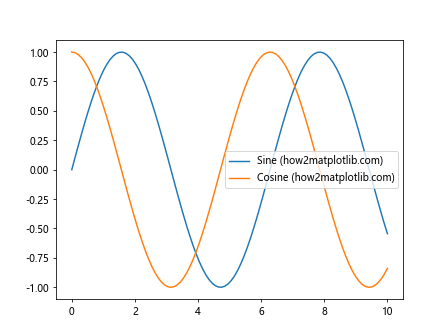
This example demonstrates how to use get_snap() with a legend, which is a compound artist containing other artists like text and lines.
Best Practices for Using get_snap()
When working with Matplotlib.artist.Artist.get_snap(), there are several best practices to keep in mind to ensure optimal results and maintainable code.
Consistency in Snap Settings
It’s generally a good idea to maintain consistency in snap settings across similar artists in your visualization. Here’s an example that demonstrates this practice:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle1 = patches.Circle((0.3, 0.3), 0.1, label='Circle 1 (how2matplotlib.com)')
circle2 = patches.Circle((0.7, 0.7), 0.1, label='Circle 2 (how2matplotlib.com)')
ax.add_patch(circle1)
ax.add_patch(circle2)
# Set consistent snap settings
circle1.set_snap(True)
circle2.set_snap(True)
print(f"Circle 1 snap setting: {circle1.get_snap()}")
print(f"Circle 2 snap setting: {circle2.get_snap()}")
plt.legend()
plt.show()
Output:
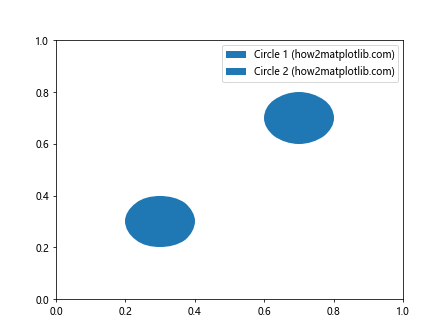
This example shows how to set consistent snap settings for similar artists, making it easier to manage and understand your visualization’s behavior.
Checking Snap Settings Before Rendering
It’s a good practice to check the snap settings of your artists before rendering the final plot. This can help you catch any unexpected settings and ensure your visualization looks as intended. Here’s an example:
import matplotlib.pyplot as plt
import matplotlib.lines as lines
fig, ax = plt.subplots()
line1 = lines.Line2D([0, 1], [0, 1], label='Line 1 (how2matplotlib.com)')
line2 = lines.Line2D([0, 1], [1, 0], label='Line 2 (how2matplotlib.com)')
ax.add_line(line1)
ax.add_line(line2)
# Check snap settings before rendering
for artist in ax.get_children():
if isinstance(artist, lines.Line2D):
print(f"{artist.get_label()} snap setting: {artist.get_snap()}")
plt.legend()
plt.show()
Output:
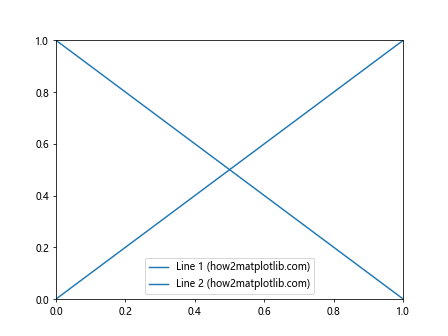
This example demonstrates how to check the snap settings of all Line2D artists in the axes before rendering the final plot.
Troubleshooting Common Issues with get_snap()
When working with Matplotlib.artist.Artist.get_snap(), you may encounter some common issues. Let’s explore these issues and how to resolve them.
Unexpected Snap Settings
Sometimes, you might find that an artist’s snap setting is not what you expected. This can happen if you’ve inadvertently changed the setting or if you’re working with artists created by other functions. Here’s an example of how to diagnose and fix this issue:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
rect = patches.Rectangle((0.2, 0.2), 0.6, 0.6, label='Rectangle (how2matplotlib.com)')
ax.add_patch(rect)
print(f"Initial snap setting: {rect.get_snap()}")
# Oops, we accidentally set the snap to False
rect.set_snap(False)
print(f"Unexpected snap setting: {rect.get_snap()}")
# Fix the issue by setting it back to None (default behavior)
rect.set_snap(None)
print(f"Corrected snap setting: {rect.get_snap()}")
plt.legend()
plt.show()
Output:
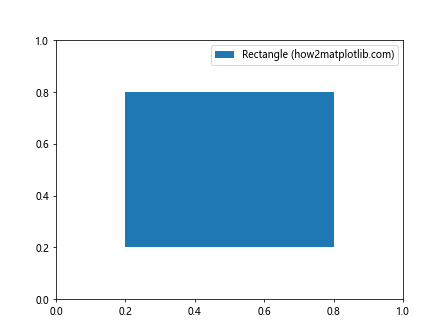
This example shows how to identify an unexpected snap setting and correct it.
Inconsistent Behavior Across Matplotlib Versions
Matplotlib’s behavior regarding snapping can sometimes change between versions. It’s important to be aware of these changes and adjust your code accordingly. Here’s an example that demonstrates how to handle version-specific behavior:
import matplotlib.pyplot as plt
import matplotlib.lines as lines
import matplotlib
fig, ax = plt.subplots()
line = lines.Line2D([0, 1], [0, 1], label='Line (how2matplotlib.com)')
ax.add_line(line)
print(f"Matplotlib version: {matplotlib.__version__}")
print(f"Default snap setting: {line.get_snap()}")
# Version-specific handling
if matplotlib.__version__ < '3.0':
# Older versions might have different default behavior
line.set_snap(True)
else:
# Newer versions use None as default
line.set_snap(None)
print(f"Adjusted snap setting: {line.get_snap()}")
plt.legend()
plt.show()
Output:
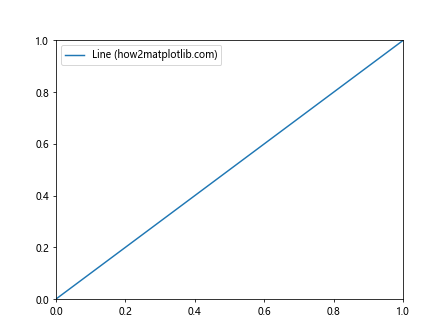
This example shows how to handle version-specific differences in snap behavior by checking the Matplotlib version and adjusting the snap setting accordingly.
Optimizing Performance with get_snap()
While Matplotlib.artist.Artist.get_snap() itself doesn't have a significant impact on performance, the snap setting it retrieves can affect rendering performance. Let's explore some ways to optimize performance when working with snap settings.
Balancing Quality and Performance
Snapping can improve the visual quality of your plots, but it may also impact rendering performance, especially for complex visualizations with many artists. Here's an example that demonstrates how to balance quality and performance:
import matplotlib.pyplot as plt
import numpy as np
import time
fig, (ax1, ax2) = plt.subplots(1, 2,figsize=(12, 5))
x = np.linspace(0, 10, 1000)
y = np.sin(x)
# Plot with snapping enabled
start_time = time.time()
line1 = ax1.plot(x, y, label='Snapping enabled (how2matplotlib.com)')[0]
line1.set_snap(True)
ax1.set_title('Snapping Enabled')
end_time = time.time()
print(f"Time with snapping: {end_time - start_time:.4f} seconds")
# Plot with snapping disabled
start_time = time.time()
line2 = ax2.plot(x, y, label='Snapping disabled (how2matplotlib.com)')[0]
line2.set_snap(False)
ax2.set_title('Snapping Disabled')
end_time = time.time()
print(f"Time without snapping: {end_time - start_time:.4f} seconds")
plt.tight_layout()
plt.show()
Output:
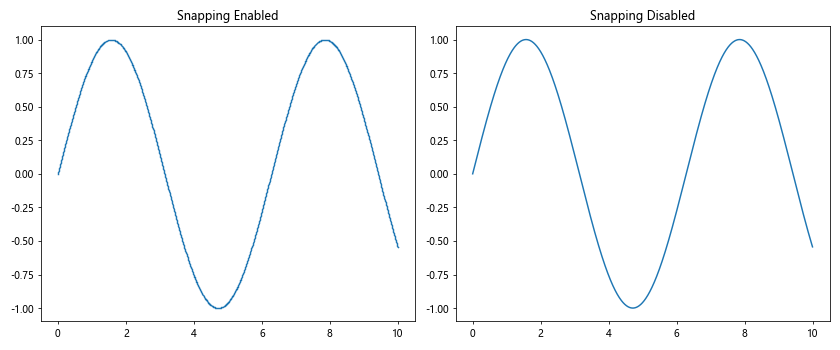
This example compares the rendering time of a plot with snapping enabled and disabled. While the difference may be negligible for simple plots, it can become noticeable for more complex visualizations.
Selective Snapping
To optimize performance while maintaining visual quality, you can selectively enable snapping for only the most important elements of your plot. Here's an example:
import matplotlib.pyplot as plt
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 1000)
y1 = np.sin(x)
y2 = np.cos(x)
# Main plot line with snapping enabled
line1 = ax.plot(x, y1, label='Main line (how2matplotlib.com)')[0]
line1.set_snap(True)
# Secondary plot line with snapping disabled
line2 = ax.plot(x, y2, label='Secondary line (how2matplotlib.com)')[0]
line2.set_snap(False)
# Grid lines with snapping disabled
ax.grid(True)
for grid_line in ax.get_xgridlines() + ax.get_ygridlines():
grid_line.set_snap(False)
print(f"Main line snap setting: {line1.get_snap()}")
print(f"Secondary line snap setting: {line2.get_snap()}")
print(f"Grid line snap setting: {ax.get_xgridlines()[0].get_snap()}")
plt.legend()
plt.show()
Output:
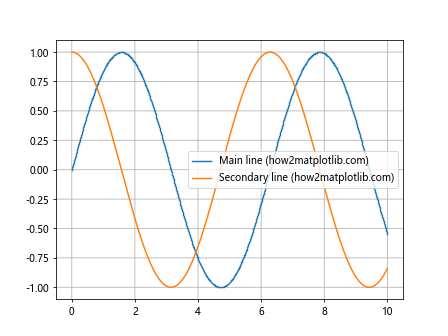
This example demonstrates how to selectively enable snapping for the main plot elements while disabling it for less critical elements to balance visual quality and performance.
Integrating get_snap() with Interactive Plots
Matplotlib.artist.Artist.get_snap() can be particularly useful when working with interactive plots. Let's explore some examples of how to integrate get_snap() with interactive features.
Toggle Snapping with a Button
You can create an interactive button to toggle snapping on and off for specific artists. Here's an example:
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y = np.sin(x)
line, = ax.plot(x, y, label='Sine wave (how2matplotlib.com)')
def toggle_snap(event):
current_snap = line.get_snap()
new_snap = not current_snap if isinstance(current_snap, bool) else True
line.set_snap(new_snap)
snap_status.set_text(f"Snap: {new_snap}")
fig.canvas.draw_idle()
ax_button = plt.axes([0.8, 0.05, 0.1, 0.075])
button = Button(ax_button, 'Toggle Snap')
button.on_clicked(toggle_snap)
ax_status = plt.axes([0.8, 0.15, 0.1, 0.075])
snap_status = ax_status.text(0.5, 0.5, f"Snap: {line.get_snap()}", ha='center', va='center')
ax_status.axis('off')
plt.show()
Output:
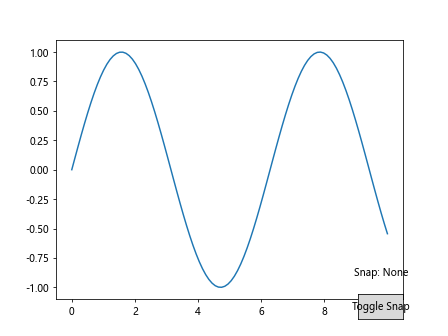
This example creates a button that toggles the snap setting for the plot line and displays the current snap status.
Displaying Snap Settings in a Tooltip
You can use tooltips to display the snap settings of artists when hovering over them. Here's an example using the mpld3
library:
import matplotlib.pyplot as plt
import mpld3
import numpy as np
fig, ax = plt.subplots()
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
line1 = ax.plot(x, y1, label='Sine (how2matplotlib.com)')[0]
line2 = ax.plot(x, y2, label='Cosine (how2matplotlib.com)')[0]
line1.set_snap(True)
line2.set_snap(False)
tooltip1 = mpld3.plugins.LineLabelTooltip(line1, label=f"Sine - Snap: {line1.get_snap()}")
tooltip2 = mpld3.plugins.LineLabelTooltip(line2, label=f"Cosine - Snap: {line2.get_snap()}")
mpld3.plugins.connect(fig, tooltip1, tooltip2)
plt.legend()
mpld3.show()
This example uses the mpld3
library to create interactive tooltips that display the snap settings of the plot lines when hovering over them.
Conclusion
In this comprehensive guide, we've explored the Matplotlib.artist.Artist.get_snap() method in Python, covering its functionality, usage, and best practices. We've seen how get_snap() can be used with various artist types, combined with other methods, and integrated into interactive visualizations.
Key takeaways include:
- The get_snap() method returns the current snap setting of an artist, which can be True, False, or None.
- Snap settings can affect both the visual quality and rendering performance of your plots.
- Consistency in snap settings across similar artists can help maintain a cohesive look in your visualizations.
- Checking snap settings before rendering can help catch unexpected behavior.
- Version-specific handling may be necessary when working with different Matplotlib versions.
- Selective snapping can be used to balance visual quality and performance.
- get_snap() can be integrated with interactive features to create dynamic visualizations.