Comprehensive Guide to Using Matplotlib.artist.Artist.get_url() in Python for Data Visualization
Matplotlib.artist.Artist.get_url() in Python is a powerful method that allows you to retrieve the URL associated with an Artist object in Matplotlib. This function is an essential part of the Matplotlib library, which is widely used for creating static, animated, and interactive visualizations in Python. In this comprehensive guide, we’ll explore the various aspects of Matplotlib.artist.Artist.get_url() and how it can enhance your data visualization projects.
Understanding Matplotlib.artist.Artist.get_url() in Python
Matplotlib.artist.Artist.get_url() in Python is a method that belongs to the Artist class in Matplotlib. The Artist class is the base class for all visible elements in a Matplotlib figure. This method allows you to retrieve the URL associated with an Artist object, which can be useful for creating interactive plots or adding hyperlinks to specific elements in your visualizations.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.get_url() in Python:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Create a text object with a URL
text = ax.text(0.5, 0.5, 'Visit how2matplotlib.com', ha='center', va='center')
text.set_url('https://how2matplotlib.com')
# Get the URL using get_url()
url = text.get_url()
print(f"The URL associated with the text object is: {url}")
plt.show()
Output:
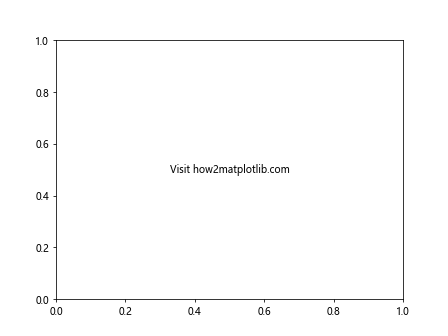
In this example, we create a text object with a URL using the set_url()
method, and then retrieve the URL using get_url()
. This demonstrates the basic usage of Matplotlib.artist.Artist.get_url() in Python.
The Importance of Matplotlib.artist.Artist.get_url() in Data Visualization
Matplotlib.artist.Artist.get_url() in Python plays a crucial role in creating interactive and informative visualizations. By associating URLs with various elements in your plots, you can enhance the user experience and provide additional context or resources related to your data.
Here are some key reasons why Matplotlib.artist.Artist.get_url() in Python is important:
- Interactive visualizations: By adding URLs to plot elements, you can create clickable areas that lead to external resources or additional information.
Data exploration: URLs can be used to link to detailed datasets or explanations, allowing users to dive deeper into the data being visualized.
Documentation: You can use URLs to link to documentation or methodology explanations, providing transparency and context for your visualizations.
Cross-referencing: URLs can be used to create connections between different visualizations or related content.
Let’s look at an example that demonstrates how Matplotlib.artist.Artist.get_url() in Python can be used to create an interactive plot: