How to Use Matplotlib.artist.Artist.get_clip_on() in Python
Matplotlib.artist.Artist.get_clip_on() in Python is an essential method for managing the clipping behavior of artists in Matplotlib. This function is part of the Artist class, which is the base class for all visible elements in a Matplotlib figure. Understanding how to use get_clip_on() effectively can greatly enhance your ability to create and manipulate visualizations in Python using Matplotlib.
In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.artist.Artist.get_clip_on() in Python, providing detailed explanations, numerous examples, and practical tips to help you master this important function.
What is Matplotlib.artist.Artist.get_clip_on() in Python?
Matplotlib.artist.Artist.get_clip_on() in Python is a method that returns a boolean value indicating whether the artist uses clipping. Clipping is the process of limiting the drawing of an artist to a specific region of the figure or axes. When clipping is enabled, any part of the artist that falls outside the specified clipping region is not rendered.
The get_clip_on() method is particularly useful when you need to check the current clipping status of an artist. This information can be valuable when you’re troubleshooting visualization issues or when you want to ensure that your artists are being rendered as expected.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.get_clip_on() in Python:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Create a simple line plot
line, = ax.plot([0, 1, 2, 3, 4], [0, 1, 4, 9, 16], label='how2matplotlib.com')
# Check if clipping is enabled for the line
is_clipping_on = line.get_clip_on()
print(f"Is clipping enabled for the line? {is_clipping_on}")
plt.title("Matplotlib.artist.Artist.get_clip_on() Example")
plt.legend()
plt.show()
Output:
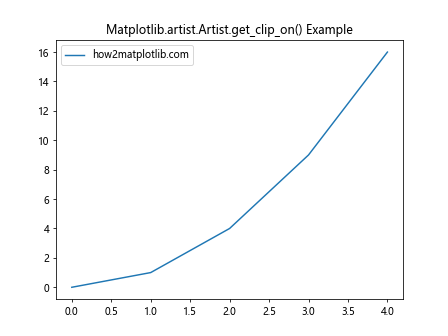
In this example, we create a simple line plot and then use the get_clip_on() method to check if clipping is enabled for the line artist. The result is printed to the console.
Understanding the Return Value of get_clip_on()
When you call Matplotlib.artist.Artist.get_clip_on() in Python, it returns a boolean value:
- True: Indicates that clipping is enabled for the artist.
- False: Indicates that clipping is disabled for the artist.
By default, most artists have clipping enabled. However, it’s important to note that the actual clipping behavior may depend on other factors, such as the artist’s position relative to the axes or figure boundaries.
Let’s look at another example that demonstrates how to check the clipping status of multiple artists:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Create multiple artists
line, = ax.plot([0, 1, 2, 3, 4], [0, 1, 4, 9, 16], label='Line')
scatter = ax.scatter([1, 2, 3], [2, 4, 6], label='Scatter')
text = ax.text(2, 8, 'how2matplotlib.com', fontsize=12)
# Check clipping status for each artist
print(f"Line clipping: {line.get_clip_on()}")
print(f"Scatter clipping: {scatter.get_clip_on()}")
print(f"Text clipping: {text.get_clip_on()}")
plt.title("Checking Clipping Status with get_clip_on()")
plt.legend()
plt.show()
Output:
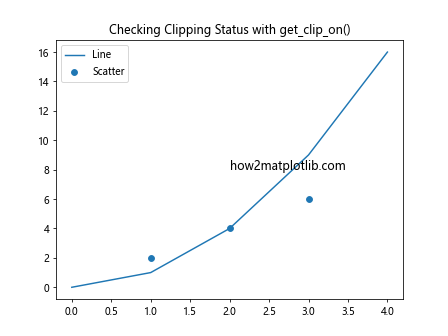
This example creates a line plot, a scatter plot, and a text annotation. We then use get_clip_on() to check the clipping status of each artist and print the results.
When to Use Matplotlib.artist.Artist.get_clip_on() in Python
Matplotlib.artist.Artist.get_clip_on() in Python is particularly useful in several scenarios:
- Debugging: When you’re troubleshooting visualization issues, checking the clipping status can help you understand why certain elements might not be visible.
Conditional Logic: You can use the clipping status in conditional statements to apply different styling or behavior based on whether an artist is being clipped.
Ensuring Consistency: When working with complex visualizations, you may want to ensure that all artists have consistent clipping settings.
Custom Visualization Tools: If you’re building custom visualization tools or wrappers around Matplotlib, get_clip_on() can be used to query and report on the state of artists.
Let’s look at an example that demonstrates using get_clip_on() in conditional logic: