Comprehensive Guide to Matplotlib.artist.Artist.get_clip_box() in Python: Mastering Clipping Regions
Matplotlib.artist.Artist.get_clip_box() in Python is an essential method for managing and retrieving clipping regions in Matplotlib plots. This comprehensive guide will delve deep into the intricacies of using get_clip_box() to enhance your data visualization skills. We’ll explore various aspects of this method, providing detailed explanations and practical examples to help you master its usage.
Understanding Matplotlib.artist.Artist.get_clip_box() in Python
Matplotlib.artist.Artist.get_clip_box() in Python is a method that returns the clipping box of an artist object. The clipping box defines the region within which the artist is visible. This method is crucial for controlling the visibility of plot elements and managing complex visualizations.
Let’s start with a simple example to demonstrate the basic usage of get_clip_box():
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.2, fill=False)
ax.add_patch(circle)
clip_box = circle.get_clip_box()
print(f"Clip box: {clip_box}")
plt.title("How2matplotlib.com - Basic get_clip_box() Example")
plt.show()
Output:
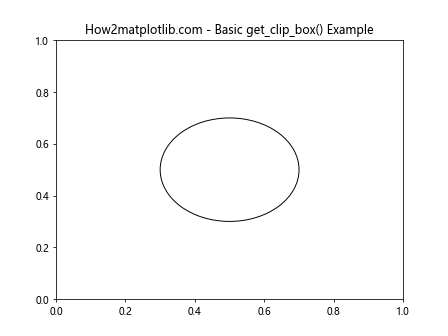
In this example, we create a circle patch and add it to the axes. We then use get_clip_box() to retrieve the clipping box of the circle. The returned value represents the bounding box of the entire axes.
Exploring the Return Value of Matplotlib.artist.Artist.get_clip_box() in Python
When you call Matplotlib.artist.Artist.get_clip_box() in Python, it returns a Bbox object representing the clipping box. The Bbox object contains information about the bounding box, including its coordinates and dimensions.
Let’s examine the structure of the returned Bbox object:
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.2, fill=False)
ax.add_patch(circle)
clip_box = circle.get_clip_box()
print(f"Clip box type: {type(clip_box)}")
print(f"Clip box bounds: {clip_box.bounds}")
print(f"Clip box x0: {clip_box.x0}")
print(f"Clip box y0: {clip_box.y0}")
print(f"Clip box x1: {clip_box.x1}")
print(f"Clip box y1: {clip_box.y1}")
plt.title("How2matplotlib.com - Exploring get_clip_box() Return Value")
plt.show()
Output:
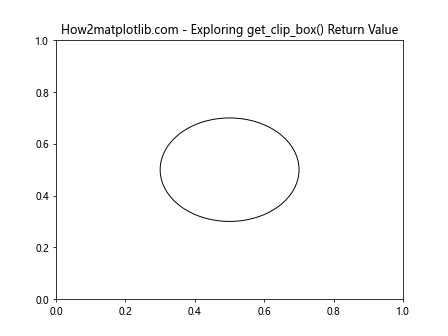
This example demonstrates how to access various properties of the Bbox object returned by get_clip_box(). Understanding these properties is essential for working with clipping regions effectively.
Using Matplotlib.artist.Artist.get_clip_box() in Python with Different Artist Types
Matplotlib.artist.Artist.get_clip_box() in Python can be used with various types of artists, including lines, patches, and text objects. Let’s explore how to use get_clip_box() with different artist types:
Lines
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line, = ax.plot([0, 1, 2], [0, 1, 0], label="How2matplotlib.com")
clip_box = line.get_clip_box()
print(f"Line clip box: {clip_box}")
plt.title("How2matplotlib.com - get_clip_box() with Lines")
plt.legend()
plt.show()
Output:
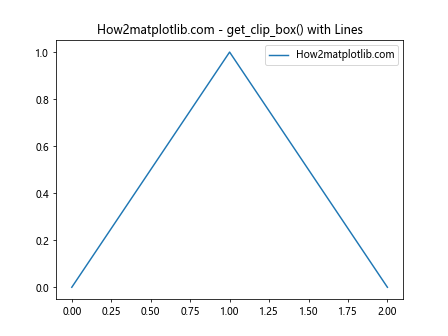
Patches
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
rect = patches.Rectangle((0.2, 0.2), 0.6, 0.6, fill=False, label="How2matplotlib.com")
ax.add_patch(rect)
clip_box = rect.get_clip_box()
print(f"Rectangle clip box: {clip_box}")
plt.title("How2matplotlib.com - get_clip_box() with Patches")
plt.legend()
plt.show()
Output:
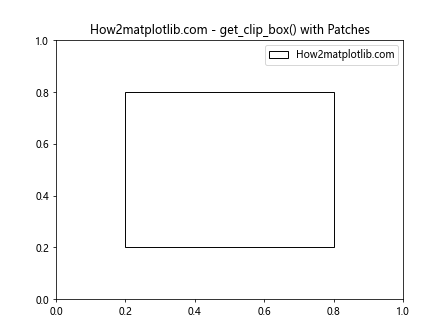
Text
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
text = ax.text(0.5, 0.5, "How2matplotlib.com", ha="center", va="center")
clip_box = text.get_clip_box()
print(f"Text clip box: {clip_box}")
plt.title("How2matplotlib.com - get_clip_box() with Text")
plt.show()
Output:
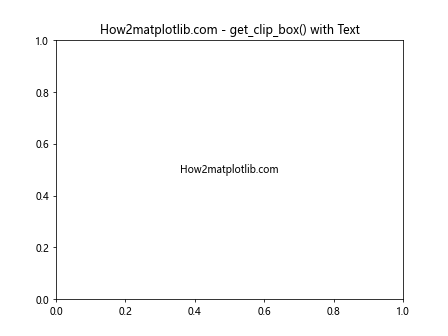
These examples demonstrate how to use Matplotlib.artist.Artist.get_clip_box() in Python with different types of artists, showcasing its versatility in managing clipping regions across various plot elements.
Combining Matplotlib.artist.Artist.get_clip_box() in Python with Other Clipping Methods
Matplotlib.artist.Artist.get_clip_box() in Python can be used in conjunction with other clipping methods to create more complex and precise clipping behaviors. Let’s explore some examples of combining get_clip_box() with other clipping techniques:
Using get_clip_box() with set_clip_path()
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
rect = patches.Rectangle((0.2, 0.2), 0.6, 0.6, fill=False)
ax.add_patch(rect)
original_clip_box = rect.get_clip_box()
print(f"Original clip box: {original_clip_box}")
clip_path = patches.Circle((0.5, 0.5), 0.3)
rect.set_clip_path(clip_path)
updated_clip_box = rect.get_clip_box()
print(f"Updated clip box: {updated_clip_box}")
plt.title("How2matplotlib.com - get_clip_box() with set_clip_path()")
plt.show()
Output:
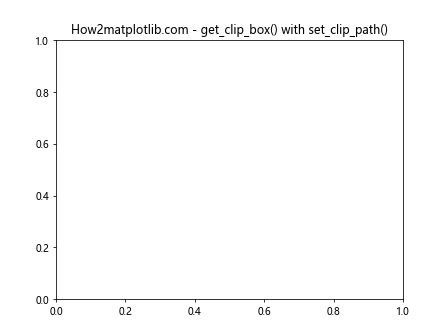
These examples demonstrate how Matplotlib.artist.Artist.get_clip_box() in Python can be used in combination with other clipping methods to achieve more advanced clipping effects.
Common Pitfalls and Troubleshooting with Matplotlib.artist.Artist.get_clip_box() in Python
While using Matplotlib.artist.Artist.get_clip_box() in Python, you may encounter some common issues. Let’s discuss these pitfalls and how to troubleshoot them:
Incorrect Clipping Box
Sometimes, the clipping box returned by get_clip_box() may not match your expectations. This can happen if the artist’s transform is not properly set or if the figure layout has changed.
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
rect = patches.Rectangle((0.2, 0.2), 0.6, 0.6, fill=False)
ax.add_patch(rect)
clip_box = rect.get_clip_box()
print(f"Initial clip box: {clip_box}")
# Change the figure size
fig.set_size_inches(8, 6)
updated_clip_box = rect.get_clip_box()
print(f"Updated clip box: {updated_clip_box}")
plt.title("How2matplotlib.com - Incorrect Clipping Box")
plt.show()
Output:
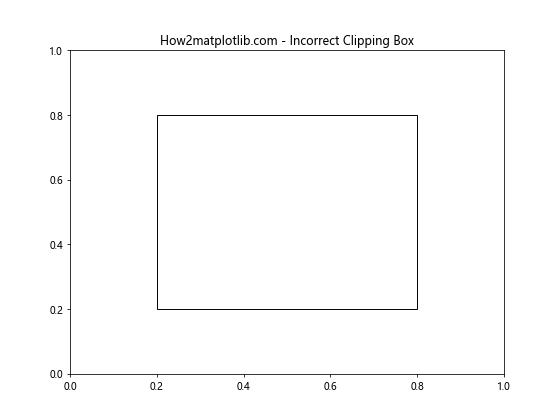
To resolve this issue, make sure to update the artist’s transform or redraw the figure after making changes to the layout.
Clipping Box Not Affecting Visibility
In some cases, you might find that the clipping box returned by get_clip_box() doesn’t seem to affect the visibility of the artist. This can occur if the artist has a custom clipping path set.
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.3, fill=False)
ax.add_patch(circle)
clip_box = circle.get_clip_box()
print(f"Clip box: {clip_box}")
# Set a custom clipping path
clip_path = patches.Rectangle((0.2, 0.2), 0.6, 0.6)
circle.set_clip_path(clip_path)
updated_clip_box = circle.get_clip_box()
print(f"Updated clip box: {updated_clip_box}")
plt.title("How2matplotlib.com - Clipping Box Not Affecting Visibility")
plt.show()
Output:
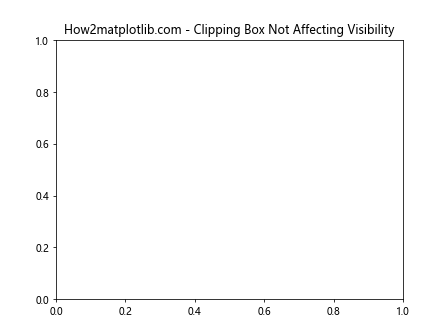
To address this issue, check if the artist has a custom clipping path set and consider using set_clip_path(None) to remove it if necessary.
Future Developments and Trends in Matplotlib.artist.Artist.get_clip_box() in Python
As Matplotlib continues to evolve, we can expect to see improvements and new features related to Matplotlib.artist.Artist.get_clip_box() in Python. Some potential future developments and trends include:
- Enhanced integration with interactive plotting libraries
- Improved performance for handling large datasets
- More advanced clipping techniques, such as non-rectangular clipping regions
- Better support for 3D plotting and clipping
While we can’t predict exactly how get_clip_box() will change in the future, it’s likely that it will continue to be an important tool for managing plot element visibility and clipping in Matplotlib.
Conclusion: Mastering Matplotlib.artist.Artist.get_clip_box() in Python
In this comprehensive guide, we’ve explored Matplotlib.artist.Artist.get_clip_box() in Python from various angles, covering its basic usage, advanced techniques, common pitfalls, and integration with data analysis workflows. By mastering get_clip_box(), you can gain greater control over your Matplotlib visualizations and create more polished and professional-looking plots.