Comprehensive Guide to Matplotlib.artist.Artist.get_children() in Python
Matplotlib.artist.Artist.get_children() in Python is a powerful method that allows you to access and manipulate the child artists of a given Artist object. This function is an essential tool for developers working with Matplotlib, as it provides a way to navigate the hierarchical structure of plots and access individual elements for customization. In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.artist.Artist.get_children(), its usage, and practical applications in various plotting scenarios.
Understanding Matplotlib.artist.Artist.get_children() in Python
Matplotlib.artist.Artist.get_children() is a method that returns a list of child Artist objects associated with a given Artist. These child artists can include various elements of a plot, such as lines, text, axes, and more. By using this method, you can access and modify these child elements, allowing for fine-grained control over your visualizations.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.get_children():
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot a line
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
# Get the children of the axis
children = ax.get_children()
# Print the number of children
print(f"Number of children: {len(children)}")
# Print the types of children
for child in children:
print(type(child))
plt.show()
Output:
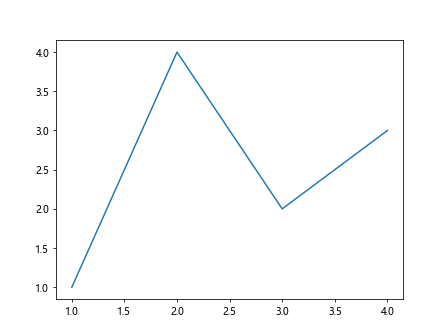
In this example, we create a simple line plot and then use Matplotlib.artist.Artist.get_children() to retrieve the children of the axis. We print the number of children and their types to get an idea of what elements are present in the plot.
Exploring the Hierarchy of Artists in Matplotlib
To fully understand Matplotlib.artist.Artist.get_children(), it’s important to grasp the hierarchical structure of Matplotlib plots. At the top level, we have the Figure, which contains one or more Axes. Each Axis can contain various child artists such as lines, text, and other plot elements.
Let’s explore this hierarchy using Matplotlib.artist.Artist.get_children():
import matplotlib.pyplot as plt
# Create a figure with two subplots
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
# Plot data on both axes
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
ax2.scatter([1, 2, 3, 4], [3, 1, 4, 2], label='how2matplotlib.com')
# Get children of the figure
fig_children = fig.get_children()
print("Figure children:")
for child in fig_children:
print(f"- {type(child)}")
# Get children of the first axis
ax1_children = ax1.get_children()
print("\nAxis 1 children:")
for child in ax1_children:
print(f"- {type(child)}")
plt.show()
Output:
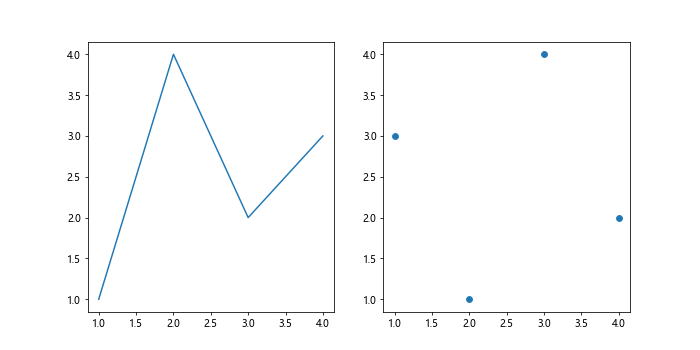
This example demonstrates how Matplotlib.artist.Artist.get_children() can be used to explore the hierarchy of a figure with multiple subplots. We print the types of children for both the figure and the first axis to illustrate the different levels of the hierarchy.
Manipulating Child Artists with Matplotlib.artist.Artist.get_children()
One of the most powerful applications of Matplotlib.artist.Artist.get_children() is the ability to access and modify specific child artists. This can be particularly useful when you want to change properties of plot elements after they’ve been created.
Here’s an example that demonstrates how to modify the properties of a line plot using Matplotlib.artist.Artist.get_children():
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot a line
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
# Get the children of the axis
children = ax.get_children()
# Find the Line2D object (the plotted line)
line = next(child for child in children if isinstance(child, plt.Line2D))
# Modify the line properties
line.set_color('red')
line.set_linewidth(3)
line.set_linestyle('--')
plt.show()
Output:
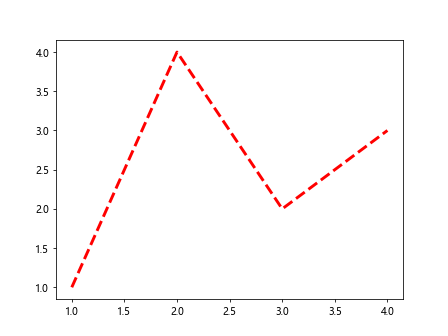
In this example, we use Matplotlib.artist.Artist.get_children() to access the Line2D object representing our plotted line. We then modify its color, line width, and line style. This demonstrates how Matplotlib.artist.Artist.get_children() can be used to make targeted modifications to specific plot elements.
Working with Text Artists using Matplotlib.artist.Artist.get_children()
Text elements in Matplotlib plots are also accessible through Matplotlib.artist.Artist.get_children(). This can be useful for modifying labels, titles, and other text elements after they’ve been created.
Here’s an example that shows how to access and modify text artists:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot data and add labels
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
ax.set_title("My Plot")
ax.set_xlabel("X-axis")
ax.set_ylabel("Y-axis")
# Get the children of the axis
children = ax.get_children()
# Find and modify text elements
for child in children:
if isinstance(child, plt.Text):
if child.get_text() == "My Plot":
child.set_color('red')
child.set_fontsize(16)
elif child.get_text() == "X-axis":
child.set_color('blue')
elif child.get_text() == "Y-axis":
child.set_color('green')
plt.show()
Output:
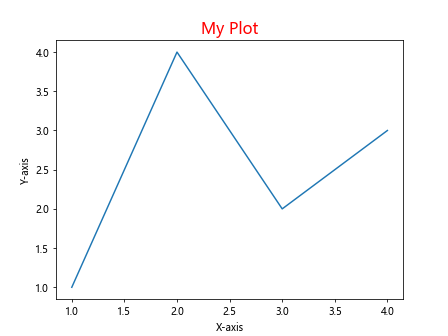
This example demonstrates how to use Matplotlib.artist.Artist.get_children() to access and modify text elements in a plot. We change the color and font size of the title, and the colors of the x and y-axis labels.
Accessing and Modifying Legend Artists with Matplotlib.artist.Artist.get_children()
Legends in Matplotlib are also accessible through Matplotlib.artist.Artist.get_children(). This can be useful for customizing legend appearance or modifying individual legend entries.
Here’s an example that demonstrates how to access and modify legend artists:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot multiple lines
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Line 1 - how2matplotlib.com')
ax.plot([1, 2, 3, 4], [3, 1, 4, 2], label='Line 2 - how2matplotlib.com')
# Add a legend
legend = ax.legend()
# Get the children of the legend
legend_children = legend.get_children()
# Modify legend text
for child in legend_children:
if isinstance(child, plt.Text):
child.set_fontsize(12)
child.set_color('navy')
# Modify legend patches (color swatches)
for child in legend.legendHandles:
child.set_linewidth(3)
plt.show()
Output:
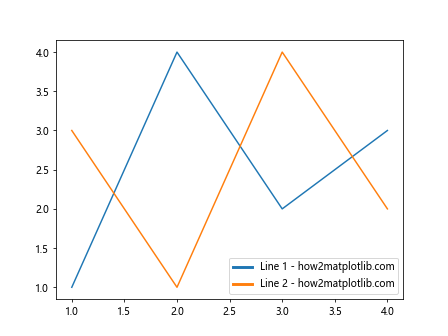
In this example, we use Matplotlib.artist.Artist.get_children() to access the children of the legend. We then modify the font size and color of the legend text, as well as the line width of the legend color swatches.
Using Matplotlib.artist.Artist.get_children() with Subplots
When working with subplots, Matplotlib.artist.Artist.get_children() can be particularly useful for accessing and modifying elements across multiple axes. Here’s an example that demonstrates this:
import matplotlib.pyplot as plt
# Create a figure with multiple subplots
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
# Plot data on both axes
ax1.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Axis 1 - how2matplotlib.com')
ax2.scatter([1, 2, 3, 4], [3, 1, 4, 2], label='Axis 2 - how2matplotlib.com')
# Add titles to both axes
ax1.set_title("Subplot 1")
ax2.set_title("Subplot 2")
# Get children of the figure
fig_children = fig.get_children()
# Modify properties of all axes
for child in fig_children:
if isinstance(child, plt.Axes):
# Change background color
child.set_facecolor('#f0f0f0')
# Modify title
title = child.get_title()
child.set_title(f"Modified: {title}", color='red')
plt.show()
Output:
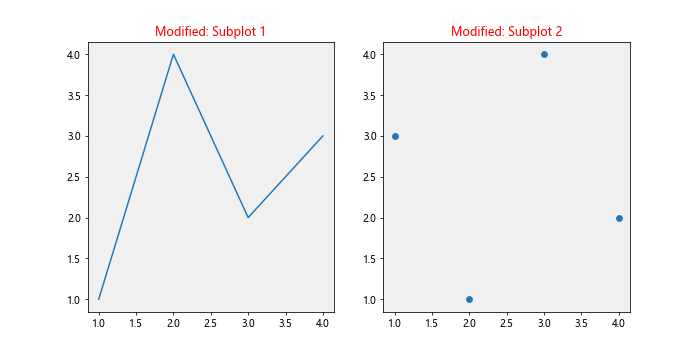
This example shows how to use Matplotlib.artist.Artist.get_children() to access and modify properties of multiple subplots simultaneously. We change the background color of all axes and modify their titles.
Modifying Tick Labels with Matplotlib.artist.Artist.get_children()
Matplotlib.artist.Artist.get_children() can also be used to access and modify tick labels. Here’s an example that demonstrates how to change the properties of tick labels:
import matplotlib.pyplot as plt
# Create a figure and axis
fig, ax = plt.subplots()
# Plot some data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
# Get the x-axis tick labels
x_tick_labels = ax.get_xticklabels()
# Modify the x-axis tick labels
for label in x_tick_labels:
label.set_rotation(45)
label.set_color('red')
label.set_fontweight('bold')
# Get the y-axis tick labels
y_tick_labels = ax.get_yticklabels()
# Modify the y-axis tick labels
for label in y_tick_labels:
label.set_color('blue')
label.set_fontsize(12)
plt.show()
Output:
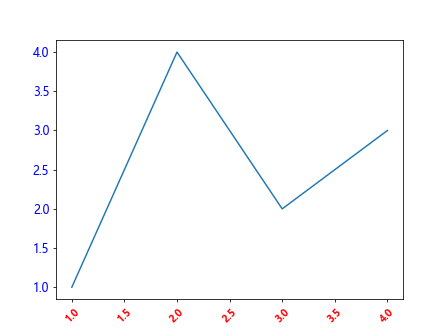
In this example, we use Matplotlib.artist.Artist.get_children() indirectly through the get_xticklabels() and get_yticklabels() methods, which return lists of Text artists. We then modify the properties of these tick labels, such as rotation, color, and font size.
Handling Animations with Matplotlib.artist.Artist.get_children()
Matplotlib.artist.Artist.get_children() can be particularly useful when working with animations. It allows you to access and modify plot elements dynamically. Here’s an example that demonstrates a simple animation using Matplotlib.artist.Artist.get_children():
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
# Create a figure and axis
fig, ax = plt.subplots()
# Initialize the plot
line, = ax.plot([], [], label='how2matplotlib.com')
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
# Animation function
def animate(frame):
x = np.linspace(0, 2*np.pi, 100)
y = np.sin(x + frame/10)
line.set_data(x, y)
# Get the children of the axis
children = ax.get_children()
# Find and modify the title
for child in children:
if isinstance(child, plt.Text) and child.get_position()[1] > 1:
child.set_text(f"Frame: {frame}")
return line,
# Create the animation
anim = animation.FuncAnimation(fig, animate, frames=100, interval=50, blit=True)
plt.show()
Output:
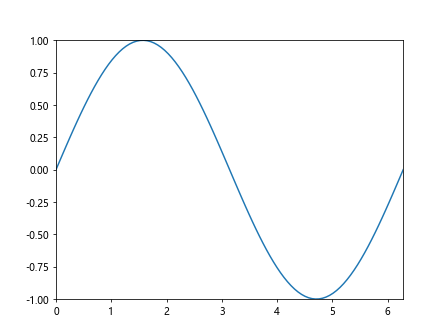
In this example, we use Matplotlib.artist.Artist.get_children() within the animation function to access and modify the title of the plot dynamically. This demonstrates how Matplotlib.artist.Artist.get_children() can be used in more complex, interactive visualizations.
Best Practices for Using Matplotlib.artist.Artist.get_children()
When working with Matplotlib.artist.Artist.get_children(), it’s important to keep some best practices in mind:
- Type checking: Always check the type of the child artists you’re working with to ensure you’re modifying the correct elements.
2.2. Performance considerations: Avoid calling Matplotlib.artist.Artist.get_children() repeatedly in loops, as it can impact performance. Instead, call it once and store the results if you need to access the children multiple times.
- Error handling: Be prepared to handle cases where the expected child artists may not be present. Use try-except blocks or conditional statements to gracefully handle these situations.
Documentation: When using Matplotlib.artist.Artist.get_children() in your code, add comments explaining what specific children you’re targeting and why. This will make your code more maintainable.
Combine with other methods: Matplotlib.artist.Artist.get_children() works well in combination with other Matplotlib methods. Don’t hesitate to use it alongside other techniques for manipulating plots.
Here’s an example that demonstrates these best practices: