Comprehensive Guide to Matplotlib.artist.Artist.get_animated() in Python
Matplotlib.artist.Artist.get_animated() in Python is a crucial method for managing animations in Matplotlib, one of the most popular data visualization libraries in Python. This function plays a significant role in determining whether an artist is animated or not, which is essential for creating dynamic and interactive visualizations. In this comprehensive guide, we’ll explore the ins and outs of Matplotlib.artist.Artist.get_animated() in Python, providing detailed explanations, numerous examples, and practical tips to help you master this powerful feature.
Understanding Matplotlib.artist.Artist.get_animated() in Python
Matplotlib.artist.Artist.get_animated() in Python is a method that belongs to the Artist class in Matplotlib. It returns a boolean value indicating whether the artist is animated or not. An animated artist is one that is redrawn for each frame in an animation, while a non-animated artist is only drawn once and then blitted onto each frame.
Let’s start with a simple example to demonstrate how to use Matplotlib.artist.Artist.get_animated() in Python:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
is_animated = line.get_animated()
print(f"Is the line animated? {is_animated}")
plt.title("Matplotlib.artist.Artist.get_animated() Example")
plt.legend()
plt.show()
Output:
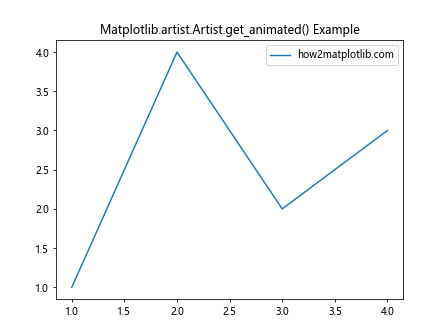
In this example, we create a simple line plot and then use the get_animated() method to check if the line artist is animated. By default, artists are not animated, so this will print “Is the line animated? False”.
Setting and Getting Animation Status with Matplotlib.artist.Artist.get_animated() in Python
To fully understand Matplotlib.artist.Artist.get_animated() in Python, it’s important to know how to set the animation status of an artist. This is done using the set_animated() method. Let’s look at an example that demonstrates both setting and getting the animation status:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
line, = ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='how2matplotlib.com')
print(f"Initial animation status: {line.get_animated()}")
line.set_animated(True)
print(f"After setting animated to True: {line.get_animated()}")
line.set_animated(False)
print(f"After setting animated to False: {line.get_animated()}")
plt.title("Setting and Getting Animation Status")
plt.legend()
plt.show()
Output:
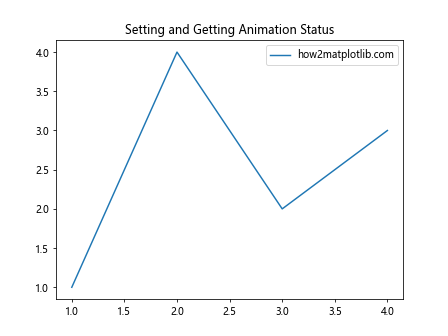
This example shows how to use set_animated() to change the animation status of the line artist, and then use get_animated() to verify the change.
Practical Applications of Matplotlib.artist.Artist.get_animated() in Python
Now that we understand the basics of Matplotlib.artist.Artist.get_animated() in Python, let’s explore some practical applications. One common use case is in creating animations where only certain elements of the plot change over time.
Here’s an example that creates a simple animation where a circle moves across the screen:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
fig, ax = plt.subplots()
circle, = ax.plot([], [], 'ro', label='how2matplotlib.com')
def init():
ax.set_xlim(0, 10)
ax.set_ylim(0, 10)
return circle,
def animate(frame):
circle.set_data(frame, 5)
return circle,
circle.set_animated(True)
print(f"Is the circle animated? {circle.get_animated()}")
ani = animation.FuncAnimation(fig, animate, frames=range(10), init_func=init, blit=True)
plt.title("Animated Circle using Matplotlib.artist.Artist.get_animated()")
plt.legend()
plt.show()
Output:
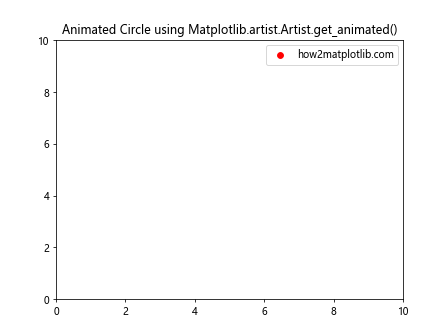
In this example, we set the circle artist to be animated and then use FuncAnimation to create the animation. The get_animated() method is used to verify that the circle is indeed set to be animated.
Optimizing Performance with Matplotlib.artist.Artist.get_animated() in Python
Matplotlib.artist.Artist.get_animated() in Python can be used to optimize the performance of your animations. By setting only the necessary artists to be animated, you can reduce the computational load and make your animations run more smoothly.
Here’s an example that demonstrates this concept:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
static_line, = ax.plot([], [], 'b-', label='Static (how2matplotlib.com)')
animated_line, = ax.plot([], [], 'r-', label='Animated (how2matplotlib.com)')
def init():
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
static_line.set_data(np.linspace(0, 2*np.pi, 100), np.sin(np.linspace(0, 2*np.pi, 100)))
return static_line, animated_line
def animate(frame):
animated_line.set_data(np.linspace(0, frame, 100), np.sin(np.linspace(0, frame, 100)))
return animated_line,
static_line.set_animated(False)
animated_line.set_animated(True)
print(f"Is static line animated? {static_line.get_animated()}")
print(f"Is animated line animated? {animated_line.get_animated()}")
ani = animation.FuncAnimation(fig, animate, frames=np.linspace(0, 2*np.pi, 100), init_func=init, blit=True)
plt.title("Optimized Animation using Matplotlib.artist.Artist.get_animated()")
plt.legend()
plt.show()
Output:
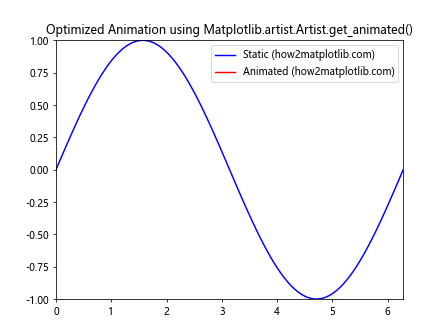
In this example, we have two lines: a static line that doesn’t change, and an animated line that grows over time. By setting only the animated line to be animated, we optimize the performance of our animation.
Advanced Techniques with Matplotlib.artist.Artist.get_animated() in Python
Matplotlib.artist.Artist.get_animated() in Python can be used in more advanced scenarios as well. For instance, you might want to dynamically change which artists are animated during the course of your animation. Here’s an example that demonstrates this:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
line1, = ax.plot([], [], 'r-', label='Line 1 (how2matplotlib.com)')
line2, = ax.plot([], [], 'b-', label='Line 2 (how2matplotlib.com)')
def init():
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
return line1, line2
def animate(frame):
if frame < np.pi:
line1.set_animated(True)
line2.set_animated(False)
line1.set_data(np.linspace(0, frame, 100), np.sin(np.linspace(0, frame, 100)))
return line1,
else:
line1.set_animated(False)
line2.set_animated(True)
line2.set_data(np.linspace(0, frame, 100), np.cos(np.linspace(0, frame, 100)))
return line2,
ani = animation.FuncAnimation(fig, animate, frames=np.linspace(0, 2*np.pi, 100), init_func=init, blit=True)
plt.title("Dynamic Animation Control with Matplotlib.artist.Artist.get_animated()")
plt.legend()
plt.show()
Output:
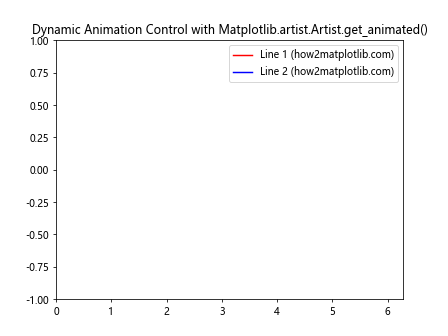
In this example, we switch which line is animated halfway through the animation. This demonstrates how you can use get_animated() and set_animated() to dynamically control your animations.
Troubleshooting with Matplotlib.artist.Artist.get_animated() in Python
When working with animations in Matplotlib, you might encounter issues related to the animation status of your artists. Matplotlib.artist.Artist.get_animated() in Python can be a valuable tool for troubleshooting these issues. Here's an example that demonstrates how to use get_animated() to debug an animation:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
line, = ax.plot([], [], 'r-', label='how2matplotlib.com')
def init():
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
return line,
def animate(frame):
line.set_data(np.linspace(0, frame, 100), np.sin(np.linspace(0, frame, 100)))
return line,
ani = animation.FuncAnimation(fig, animate, frames=np.linspace(0, 2*np.pi, 100), init_func=init, blit=True)
print(f"Is the line animated? {line.get_animated()}")
if not line.get_animated():
print("Warning: Line is not set to be animated. This may cause performance issues.")
line.set_animated(True)
print(f"Line animation status after correction: {line.get_animated()}")
plt.title("Troubleshooting with Matplotlib.artist.Artist.get_animated()")
plt.legend()
plt.show()
Output:
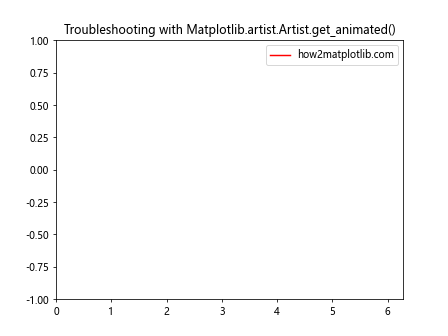
In this example, we use get_animated() to check if our line is set to be animated. If it's not, we print a warning and correct the issue by setting it to be animated.
Combining Matplotlib.artist.Artist.get_animated() with Other Animation Properties
Matplotlib.artist.Artist.get_animated() in Python works in conjunction with other animation properties and methods. Understanding how these interact can help you create more complex and efficient animations. Let's look at an example that combines get_animated() with the zorder property:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, ax = plt.subplots()
background, = ax.plot([], [], 'b-', label='Background (how2matplotlib.com)')
foreground, = ax.plot([], [], 'r-', label='Foreground (how2matplotlib.com)')
def init():
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
background.set_data(np.linspace(0, 2*np.pi, 100), np.sin(np.linspace(0, 2*np.pi, 100)))
return background, foreground
def animate(frame):
foreground.set_data(np.linspace(0, frame, 100), 0.5 * np.sin(np.linspace(0, frame, 100)))
return foreground,
background.set_animated(False)
background.set_zorder(1)
foreground.set_animated(True)
foreground.set_zorder(2)
print(f"Is background animated? {background.get_animated()}")
print(f"Is foreground animated? {foreground.get_animated()}")
print(f"Background zorder: {background.get_zorder()}")
print(f"Foreground zorder: {foreground.get_zorder()}")
ani = animation.FuncAnimation(fig, animate, frames=np.linspace(0, 2*np.pi, 100), init_func=init, blit=True)
plt.title("Combining Matplotlib.artist.Artist.get_animated() with zorder")
plt.legend()
plt.show()
Output:
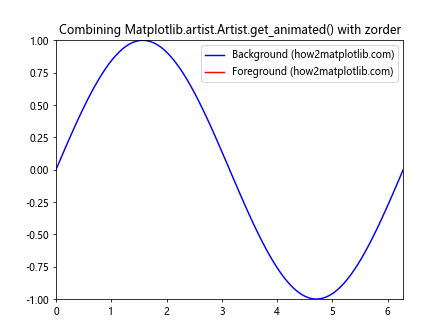
In this example, we use get_animated() in combination with the zorder property to create a layered animation where only the foreground element is animated.
Matplotlib.artist.Artist.get_animated() in Custom Animation Classes
When creating custom animation classes, Matplotlib.artist.Artist.get_animated() in Python can be a valuable tool for managing which elements of your plot are animated. Here's an example of a custom animation class that uses get_animated():
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
class CustomAnimator:
def __init__(self, ax):
self.ax = ax
self.line, = ax.plot([], [], 'r-', label='how2matplotlib.com')
self.line.set_animated(True)
def init(self):
self.ax.set_xlim(0, 2*np.pi)
self.ax.set_ylim(-1, 1)
return self.line,
def __call__(self, frame):
if self.line.get_animated():
self.line.set_data(np.linspace(0, frame, 100), np.sin(np.linspace(0, frame, 100)))
return self.line,
fig, ax = plt.subplots()
animator = CustomAnimator(ax)
ani = animation.FuncAnimation(fig, animator, frames=np.linspace(0, 2*np.pi, 100), init_func=animator.init, blit=True)
plt.title("Custom Animation Class using Matplotlib.artist.Artist.get_animated()")
plt.legend()
plt.show()
Output:
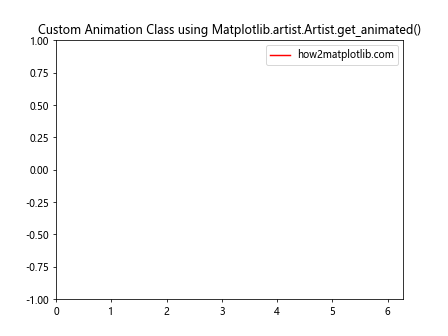
In this example, we create a CustomAnimator class that uses get_animated() to check whether the line should be updated in each frame.
Performance Considerations with Matplotlib.artist.Artist.get_animated() in Python
While Matplotlib.artist.Artist.get_animated() in Python is a powerful tool for managing animations, it's important to use it judiciously to ensure optimal performance. Here's an example that demonstrates the performance impact of animating different numbers of artists:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
import time
fig, ax = plt.subplots()
lines = [ax.plot([], [], label=f'Line {i} (how2matplotlib.com)')[0] for i in range(10)]
def init():
ax.set_xlim(0, 2*np.pi)
ax.set_ylim(-1, 1)
return lines
def animate(frame):
for i, line in enumerate(lines):
if line.get_animated():
line.set_data(np.linspace(0, frame, 100), np.sin(np.linspace(0, frame, 100) + i*np.pi/5))
return lines
def measure_performance(num_animated):
for i, line in enumerate(lines):
line.set_animated(i < num_animated)
start_time = time.time()
ani = animation.FuncAnimation(fig, animate, frames=np.linspace(0, 2*np.pi, 100), init_func=init, blit=True)
plt.draw()
plt.pause(1)
end_time = time.time()
return end_time - start_time
performance_results = [measure_performance(i) for i in range(11)]
plt.figure()
plt.plot(range(11), performance_results, 'bo-')
plt.xlabel('Number of Animated Lines')
plt.ylabel('Animation Time (seconds)')
plt.title('Performance Impact of Matplotlib.artist.Artist.get_animated()')
plt.show()
Output:
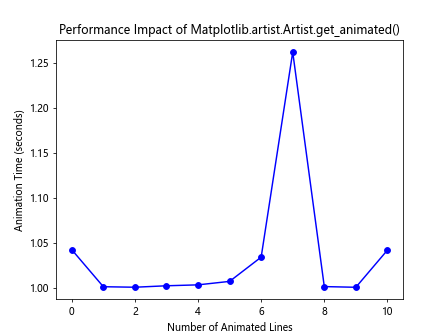
This example creates multiple lines and measures the performance impact of animating different numbers of them. It demonstrates how using get_animated() to selectively animate only necessary elements can improve performance.
Matplotlib.artist.Artist.get_animated() in Multi-Axis Plots
Matplotlib.artist.Artist.get_animated() in Python can be particularly useful when working with multi-axis plots. Here's an example that demonstrates how to use get_animated() to manage animations across multiple subplots:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import numpy as np
fig, (ax1, ax2) = plt.subplots(2, 1, figsize=(8, 8))
line1, = ax1.plot([], [], 'r-', label='Subplot 1 (how2matplotlib.com)')
line2, = ax2.plot([], [], 'b-', label='Subplot 2 (how2matplotlib.com)')
def init():
ax1.set_xlim(0, 2*np.pi)
ax1.set_ylim(-1, 1)
ax2.set_xlim(0, 2*np.pi)
ax2.set_ylim(-1, 1)
return line1, line2
def animate(frame):
line1.set_data(np.linspace(0, frame, 100), np.sin(np.linspace(0, frame, 100)))
line2.set_data(np.linspace(0, frame, 100), np.cos(np.linspace(0, frame, 100)))
return line1, line2
line1.set_animated(True)
line2.set_animated(True)
print(f"Is line1 animated? {line1.get_animated()}")
print(f"Is line2 animated? {line2.get_animated()}")
ani = animation.FuncAnimation(fig, animate, frames=np.linspace(0, 2*np.pi, 100), init_func=init, blit=True)
ax1.set_title("Subplot 1")
ax2.set_title("Subplot 2")
ax1.legend()
ax2.legend()
plt.tight_layout()
plt.show()
Output:
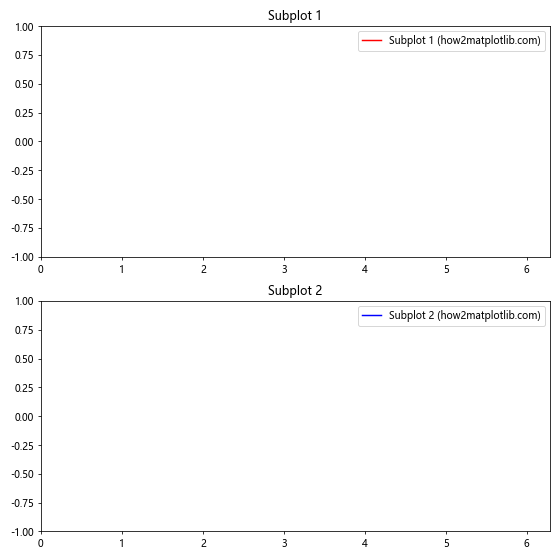
In this example, we create two subplots and animate a line in each. We use get_animated() to verify that both lines are set to be animated.
Matplotlib.artist.Artist.get_animated() in 3D Plots
Matplotlib.artist.Artist.get_animated() in Python can also be used with 3D plots. Here's an example that demonstrates how to create an animated 3D plot:
import matplotlib.pyplot as plt
import matplotlib.animation as animation
from mpl_toolkits.mplot3d import Axes3D
import numpy as np
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
def gen(n):
phi = 0
while phi < 2*np.pi:
yield np.array([np.cos(phi), np.sin(phi), phi])
phi += 2*np.pi/n
def update(num, data, line):
line.set_data(data[:2, :num])
line.set_3d_properties(data[2, :num])
return line
N = 100
data = np.array(list(gen(N))).T
line, = ax.plot(data[0, 0:1], data[1, 0:1], data[2, 0:1], label='how2matplotlib.com')
line.set_animated(True)
print(f"Is the 3D line animated? {line.get_animated()}")
ax.set_xlim(-1, 1)
ax.set_ylim(-1, 1)
ax.set_zlim(0, 10)
ax.set_title("3D Animation with Matplotlib.artist.Artist.get_animated()")
ani = animation.FuncAnimation(fig, update, N, fargs=(data, line), interval=10, blit=False)
plt.legend()
plt.show()
Output:
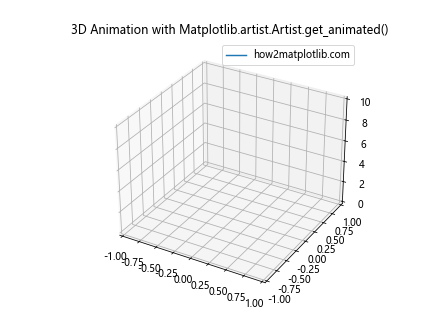
In this example, we create a 3D spiral animation and use get_animated() to verify that the line is set to be animated.
Conclusion
Matplotlib.artist.Artist.get_animated() in Python is a powerful tool for managing animations in Matplotlib. It allows you to control which elements of your plot are animated, potentially improving performance and creating more complex visualizations. By understanding how to use get_animated() in conjunction with other Matplotlib features like blitting, multi-axis plots, and 3D visualizations, you can create sophisticated and efficient animations.