How to Remove the Frame from a Matplotlib Figure in Python
How to remove the frame from a Matplotlib figure in Python is an essential skill for creating clean and professional-looking visualizations. This article will explore various techniques and methods to achieve this goal, providing detailed explanations and easy-to-understand code examples. By the end of this guide, you’ll be well-equipped to remove frames from your Matplotlib figures and enhance the overall appearance of your plots.
Understanding the Importance of Frame Removal in Matplotlib
Before diving into the specifics of how to remove the frame from a Matplotlib figure in Python, it’s crucial to understand why this technique is valuable. Removing the frame can:
- Enhance the visual appeal of your plots
- Reduce clutter and distractions
- Focus attention on the data itself
- Create a more minimalist and modern design
Let’s start with a basic example of a Matplotlib figure with a frame:
import matplotlib.pyplot as plt
# Create a simple plot
plt.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
plt.title('Plot with Frame')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
plt.legend()
# Display the plot
plt.show()
Output:
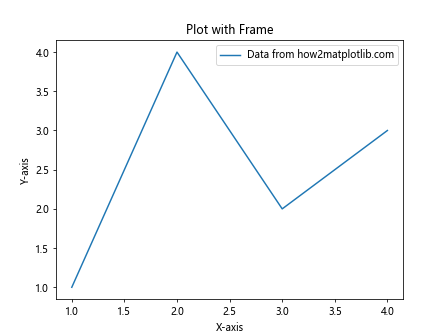
This code creates a simple line plot with a frame. Now, let’s explore various methods to remove this frame and create a cleaner visualization.
Method 1: Using plt.axis(‘off’) to Remove the Frame
One of the simplest ways to remove the frame from a Matplotlib figure in Python is by using the plt.axis('off')
command. This method turns off all axis lines, ticks, and labels, effectively removing the frame.
Here’s an example of how to remove the frame using this method:
import matplotlib.pyplot as plt
# Create a simple plot
plt.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
plt.title('Plot without Frame')
# Remove the frame
plt.axis('off')
# Display the plot
plt.show()
Output:
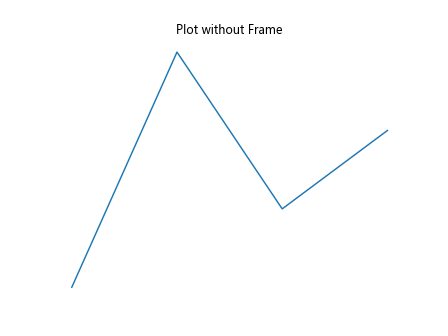
In this example, we’ve added the plt.axis('off')
line before displaying the plot. This command removes all axis elements, including the frame, ticks, and labels.
Method 2: Using spines to Remove the Frame
Another way to remove the frame from a Matplotlib figure in Python is by manipulating the spines. Spines are the lines that connect the axis tick marks and form the boundaries of the data area. By setting the visibility of all spines to False, we can effectively remove the frame.
Here’s an example:
import matplotlib.pyplot as plt
# Create a figure and axis objects
fig, ax = plt.subplots()
# Plot the data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
ax.set_title('Plot without Frame using Spines')
# Remove the frame
for spine in ax.spines.values():
spine.set_visible(False)
# Remove ticks
ax.tick_params(left=False, bottom=False, labelleft=False, labelbottom=False)
# Display the plot
plt.show()
Output:
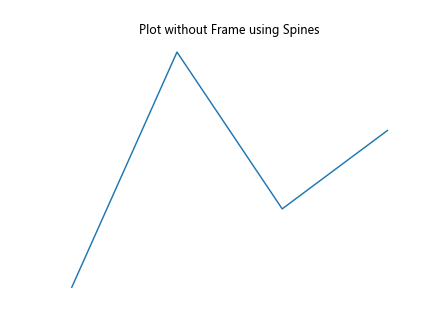
In this example, we iterate through all spines and set their visibility to False. We also remove the ticks and tick labels to complete the frameless look.
Method 3: Using ax.set_axis_off() to Remove the Frame
Similar to the plt.axis('off')
method, we can use ax.set_axis_off()
when working with Axes objects. This method is particularly useful when dealing with subplots or more complex figure layouts.
Here’s an example of how to remove the frame from a Matplotlib figure in Python using this method:
import matplotlib.pyplot as plt
# Create a figure and axis objects
fig, ax = plt.subplots()
# Plot the data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
ax.set_title('Plot without Frame using set_axis_off()')
# Remove the frame
ax.set_axis_off()
# Display the plot
plt.show()
Output:
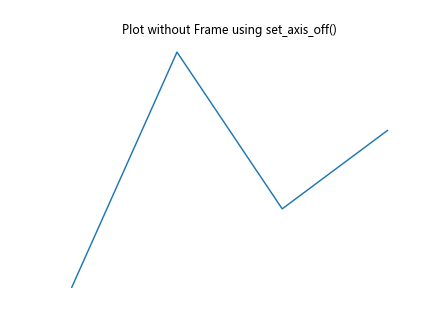
This method achieves the same result as plt.axis('off')
but is more suitable when working with specific Axes objects.
Method 4: Removing Specific Spines
Sometimes, you may want to remove only specific parts of the frame while keeping others. In this case, you can target individual spines and set their visibility to False.
Here’s an example of how to remove specific spines from a Matplotlib figure in Python:
import matplotlib.pyplot as plt
# Create a figure and axis objects
fig, ax = plt.subplots()
# Plot the data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
ax.set_title('Plot with Partial Frame')
# Remove top and right spines
ax.spines['top'].set_visible(False)
ax.spines['right'].set_visible(False)
# Display the plot
plt.show()
Output:
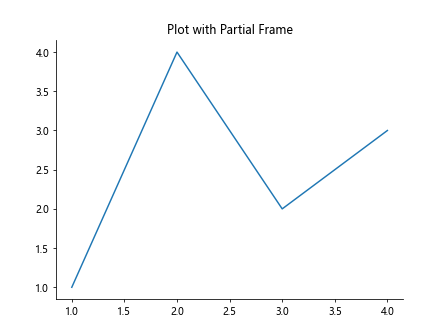
In this example, we’ve removed only the top and right spines, creating a plot with a partial frame.
Method 5: Using seaborn to Remove the Frame
Seaborn, a statistical data visualization library built on top of Matplotlib, provides an easy way to remove the frame from a Matplotlib figure in Python. The sns.despine()
function can be used to remove specific spines or all spines at once.
Here’s an example:
import matplotlib.pyplot as plt
import seaborn as sns
# Set the style to remove the frame
sns.set_style("whitegrid")
# Create a simple plot
plt.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
plt.title('Plot without Frame using Seaborn')
# Remove all spines
sns.despine(left=True, bottom=True, right=True, top=True)
# Display the plot
plt.show()
Output:
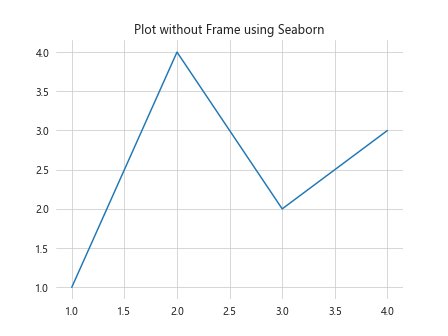
In this example, we first set the Seaborn style to “whitegrid” and then use sns.despine()
to remove all spines, effectively removing the frame.
Method 6: Customizing the Frame Removal
Sometimes, you may want to remove the frame but keep certain elements, such as axis labels or ticks. Here’s an example of how to achieve this level of customization:
import matplotlib.pyplot as plt
# Create a figure and axis objects
fig, ax = plt.subplots()
# Plot the data
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label='Data from how2matplotlib.com')
ax.set_title('Customized Frame Removal')
# Remove the frame
for spine in ax.spines.values():
spine.set_visible(False)
# Keep bottom and left ticks
ax.tick_params(left=True, bottom=True, labelleft=True, labelbottom=True)
# Set axis labels
ax.set_xlabel('X-axis')
ax.set_ylabel('Y-axis')
# Display the plot
plt.show()
Output:
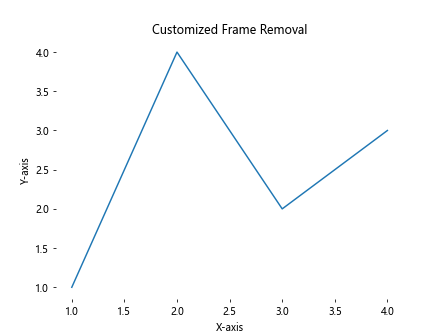
In this example, we remove the frame but keep the bottom and left ticks, as well as the axis labels, creating a clean yet informative plot.
Method 7: Removing the Frame in 3D Plots
Removing the frame from a 3D plot in Matplotlib requires a slightly different approach. Here’s an example of how to remove the frame from a Matplotlib figure in Python when working with 3D plots:
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import numpy as np
# Create a figure and 3D axis
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
# Generate some sample data
x = np.linspace(-5, 5, 100)
y = np.linspace(-5, 5, 100)
X, Y = np.meshgrid(x, y)
Z = np.sin(np.sqrt(X**2 + Y**2))
# Create the surface plot
surf = ax.plot_surface(X, Y, Z, cmap='viridis')
# Remove the frame
ax.set_axis_off()
# Set the title
ax.set_title('3D Plot without Frame (how2matplotlib.com)')
# Display the plot
plt.show()
Output:
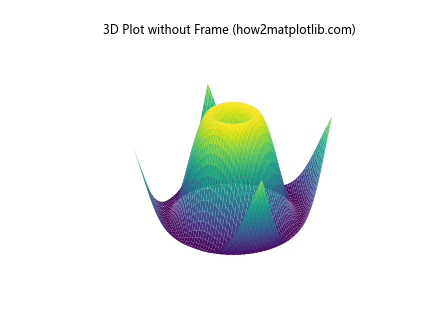
In this example, we use ax.set_axis_off()
to remove the frame from the 3D plot, creating a clean and modern look.
Method 8: Removing the Frame in Polar Plots
Polar plots are another type of visualization where removing the frame can enhance the overall appearance. Here’s an example of how to remove the frame from a Matplotlib figure in Python when working with polar plots:
import matplotlib.pyplot as plt
import numpy as np
# Create polar axes
fig, ax = plt.subplots(subplot_kw=dict(projection='polar'))
# Generate some sample data
r = np.linspace(0, 2, 100)
theta = 2 * np.pi * r
# Create the polar plot
ax.plot(theta, r)
# Remove the frame
ax.set_axis_off()
# Set the title
ax.set_title('Polar Plot without Frame (how2matplotlib.com)')
# Display the plot
plt.show()
Output:
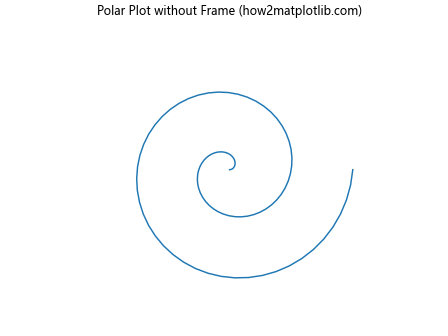
In this example, we use ax.set_axis_off()
to remove the frame from the polar plot, resulting in a clean and minimalist design.
Method 9: Removing the Frame in Subplots
When working with multiple subplots, you may want to remove the frame from all or specific subplots. Here’s an example of how to remove the frame from a Matplotlib figure in Python when dealing with subplots:
import matplotlib.pyplot as plt
# Create a figure with 2x2 subplots
fig, axs = plt.subplots(2, 2, figsize=(10, 10))
# Flatten the axs array for easier iteration
axs = axs.flatten()
# Plot data and remove frames
for i, ax in enumerate(axs):
ax.plot([1, 2, 3, 4], [1, 4, 2, 3], label=f'Data {i+1} from how2matplotlib.com')
ax.set_title(f'Subplot {i+1}')
ax.set_axis_off()
# Adjust the layout and display the plot
plt.tight_layout()
plt.show()
Output:
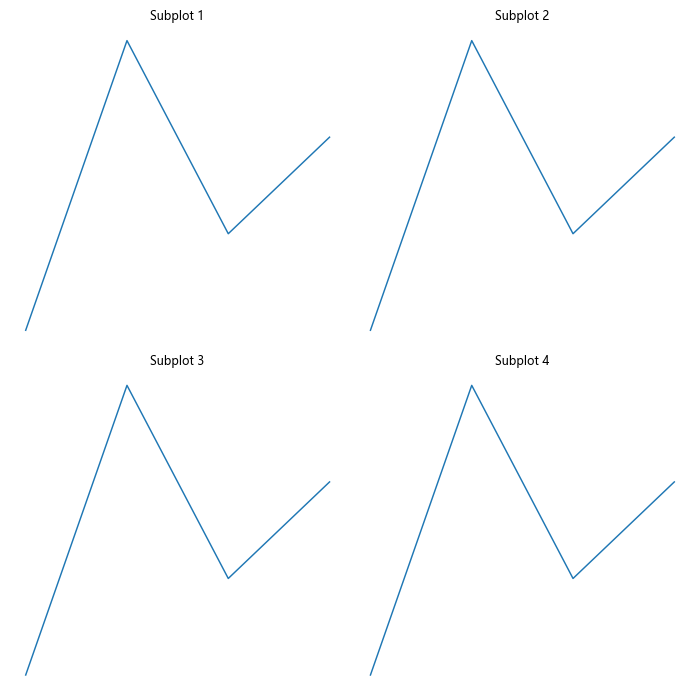
In this example, we create a 2×2 grid of subplots and remove the frame from each subplot using ax.set_axis_off()
.
Method 10: Removing the Frame in Histograms
Histograms are another type of plot where removing the frame can create a cleaner look. Here’s an example of how to remove the frame from a Matplotlib figure in Python when creating a histogram:
import matplotlib.pyplot as plt
import numpy as np
# Generate some sample data
data = np.random.normal(0, 1, 1000)
# Create the histogram
plt.hist(data, bins=30, edgecolor='black')
# Remove the frame
plt.axis('off')
# Set the title
plt.title('Histogram without Frame (how2matplotlib.com)')
# Display the plot
plt.show()
Output:
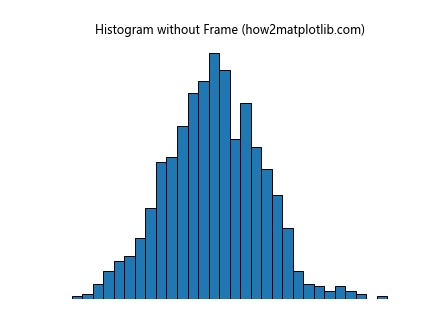
In this example, we use plt.axis('off')
to remove the frame from the histogram, creating a clean and focused visualization.
Best Practices for Removing Frames in Matplotlib
When removing the frame from a Matplotlib figure in Python, it’s important to keep a few best practices in mind:
- Consider the context: Not all plots benefit from frame removal. Use this technique when it enhances the visual appeal and clarity of your data.
Maintain necessary information: If removing the frame also removes important information (like axis labels or ticks), consider using a method that allows you to keep these elements.
Be consistent: If you’re creating multiple plots for a single project or presentation, maintain a consistent style across all visualizations.
Test different methods: Experiment with various frame removal techniques to find the one that best suits your specific plot and data.
Combine with other styling techniques: Frame removal can be combined with other Matplotlib styling options to create truly unique and professional-looking visualizations.
Advanced Techniques for Frame Removal and Plot Customization
Now that we’ve covered the basics of how to remove the frame from a Matplotlib figure in Python, let’s explore some advanced techniques that combine frame removal with other customization options.