How to Clear Plot in Matplotlib
When working with Matplotlib, there are times when you may want to clear the current plot to start fresh or to remove any existing elements. In this article, we will explore various methods to clear a plot in Matplotlib.
Using clf() method
The clf()
method can be used to clear the current figure and start a new plot. Here is an example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
plt.clf()
Output:
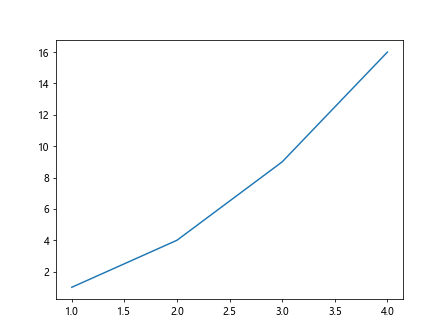
Using close() method
The close()
method can be used to close the current figure window and clear the plot. Here is an example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
plt.close()
Output:
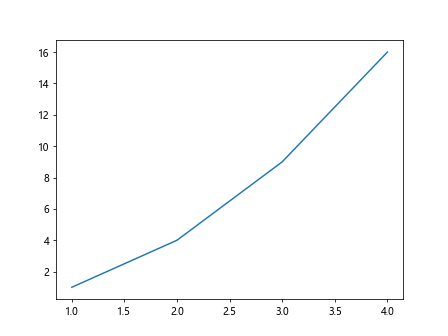
Using cla() method
The cla()
method can be used to clear the current axes of the plot without clearing the entire figure. Here is an example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
plt.cla()
Output:
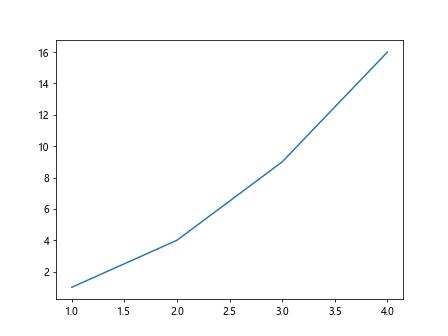
Using clf() and cla() methods together
You can also use the clf()
and cla()
methods together to clear both the figure and the axes. Here is an example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
plt.clf()
plt.cla()
Output:
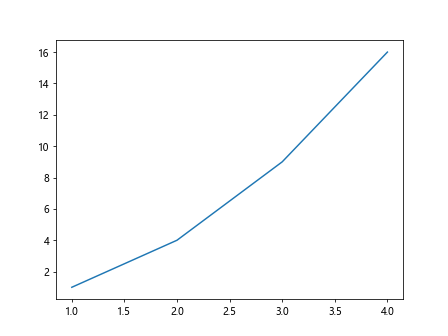
Using clear() method
The clear()
method can be used to clear the current figure and axes at the same time. Here is an example:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
plt.gcf().clear()
Output:
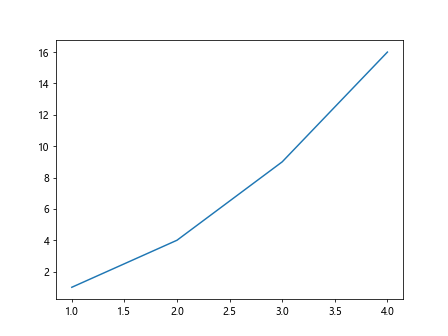
Using set_visible() method for artists
You can also hide or clear specific artists from the plot using the set_visible()
method. Here is an example:
import matplotlib.pyplot as plt
line, = plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
line.set_visible(False)
Output:
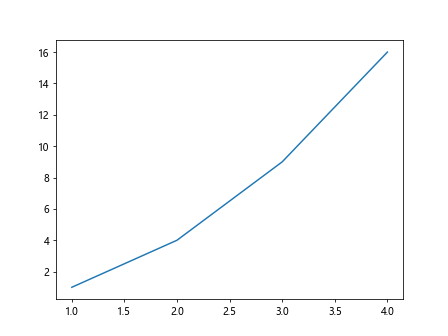
Clearing specific elements
If you want to clear specific elements from the plot, you can set their visibility to False. Here is an example:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
scatter = ax.scatter([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
scatter.set_visible(False)
Output:
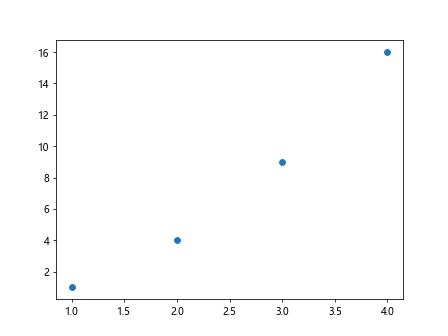
Clearing lines and text elements
You can clear lines and text elements from the plot by setting their visibility to False. Here is an example:
import matplotlib.pyplot as plt
line, = plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
text = plt.text(2, 10, 'how2matplotlib')
plt.show()
line.set_visible(False)
text.set_visible(False)
Output:
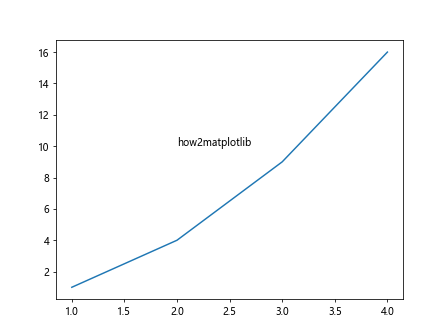
Using del method
You can also clear elements from the plot by deleting them using the del
method. Here is an example:
import matplotlib.pyplot as plt
line, = plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
text = plt.text(2, 10, 'how2matplotlib')
plt.show()
del line
del text
Output:
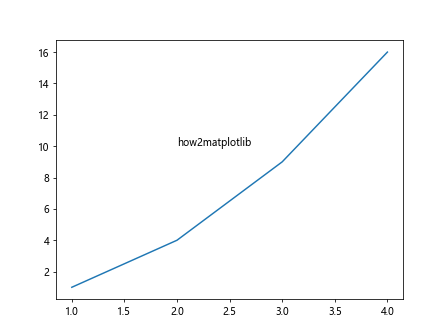
Clearing all elements
To clear all elements from the plot, you can iterate through all the artists and set their visibility to False. Here is an example:
import matplotlib.pyplot as plt
line, = plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
text = plt.text(2, 10, 'how2matplotlib')
plt.show()
for artist in plt.gca().get_children():
artist.set_visible(False)
Output:
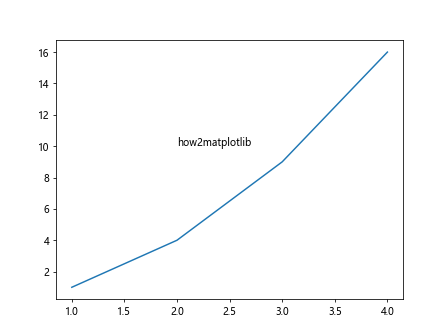
Conclusion
In this article, we have explored various methods to clear a plot in Matplotlib. Whether you want to clear the entire plot, specific elements, or hide certain artists, Matplotlib provides a variety of options to help you achieve the desired results. Experiment with these methods in your own projects to see how you can effectively manage and clear plots in Matplotlib.