How to Change Axis Font Size in Matplotlib
Matplotlib is a popular data visualization library in Python that provides a wide variety of customization options to create visually appealing plots. One common customization task is changing the font size of the axis labels in a plot. In this article, we will explore how to change the axis font size in Matplotlib.
Changing Axis Font Size
To change the font size of the axis labels in Matplotlib, you can use the fontsize
parameter in the xlabel()
and ylabel()
functions. This parameter allows you to specify the font size of the labels in points.
Here is an example code snippet that demonstrates how to change the font size of the x-axis label:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.xlabel('X-axis Label', fontsize=12)
plt.show()
Output:
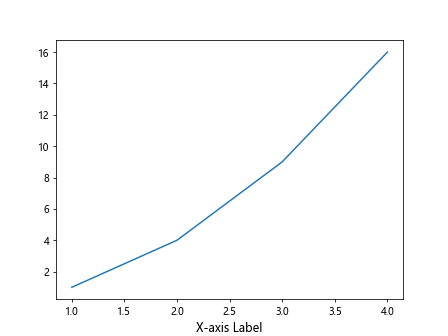
Similarly, you can change the font size of the y-axis label using the ylabel()
function like this:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.ylabel('Y-axis Label', fontsize=14)
plt.show()
Output:
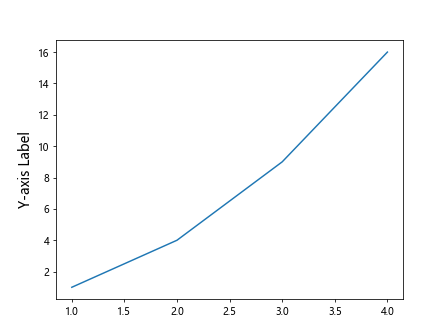
Using set_xlabel()
and set_ylabel()
Another way to change the axis font size in Matplotlib is by using the set_xlabel()
and set_ylabel()
methods of the Axes
object. These methods allow you to customize the axis labels after the plot has been created.
Here is an example code snippet that demonstrates how to change the font size of the x-axis label using set_xlabel()
:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_xlabel('X-axis Label', fontsize=16)
plt.show()
Output:
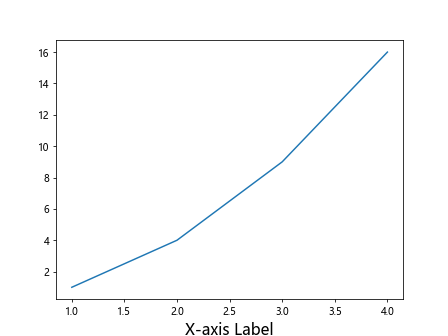
Similarly, you can change the font size of the y-axis label using set_ylabel()
like this:
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
ax.plot([1, 2, 3, 4], [1, 4, 9, 16])
ax.set_ylabel('Y-axis Label', fontsize=18)
plt.show()
Output:
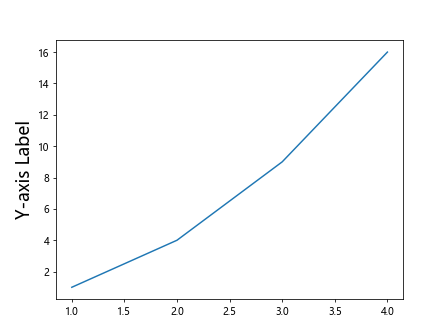
Customizing Axis Ticks
In addition to changing the font size of the axis labels, you may also want to customize the font size of the axis ticks. Matplotlib provides the tick_params()
method that allows you to adjust various properties of the ticks, including the font size.
Here is an example code snippet that demonstrates how to change the font size of the axis ticks:
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.xticks(fontsize=10)
plt.yticks(fontsize=12)
plt.show()
Output:
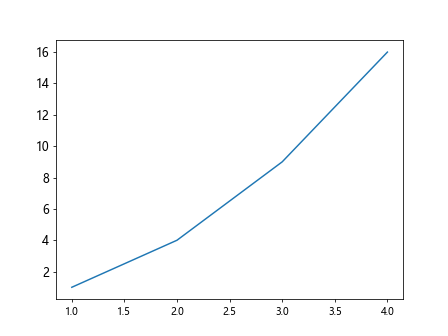
Changing Font Size Globally
If you want to change the font size of the axis labels and ticks globally for all plots in your script, you can modify the default settings of Matplotlib using the rcParams
configuration.
Here is an example code snippet that demonstrates how to change the default font size for axis labels and ticks:
import matplotlib.pyplot as plt
import matplotlib
matplotlib.rcParams.update({'font.size': 14})
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.xlabel('X-axis Label')
plt.ylabel('Y-axis Label')
plt.show()
Output:
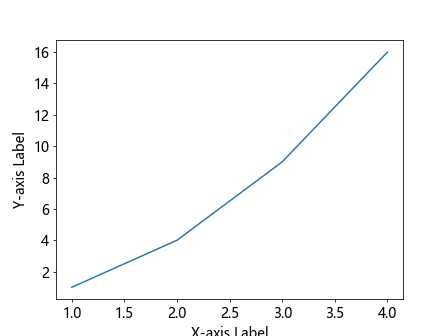
Conclusion
In this article, we have explored different ways to change the axis font size in Matplotlib. By using the fontsize
parameter in the xlabel()
and ylabel()
functions, or by using the set_xlabel()
and set_ylabel()
methods of the Axes
object, you can easily customize the font size of the axis labels in your plots. Additionally, you can adjust the font size of the axis ticks using the tick_params()
method or modify the default settings globally using rcParams
. These techniques allow you to create visually appealing plots with properly sized axis labels and ticks.
Remember to experiment with different font sizes to find the optimal values for your plots, and don’t hesitate to explore other customization options offered by Matplotlib to enhance the visual quality of your data visualizations.