How to Create Multiple Buttons in Matplotlib
Create Multiple Buttons in Matplotlib is an essential skill for data visualization enthusiasts and professionals alike. This article will delve deep into the process of creating multiple buttons in Matplotlib, providing you with a thorough understanding of the concept and its implementation. We’ll explore various techniques, best practices, and real-world examples to help you master the art of creating multiple buttons in Matplotlib.
Understanding the Basics of Creating Multiple Buttons in Matplotlib
Before we dive into the intricacies of creating multiple buttons in Matplotlib, it’s crucial to understand the fundamental concepts. Matplotlib is a powerful plotting library for Python that allows users to create a wide range of static, animated, and interactive visualizations. When it comes to creating multiple buttons in Matplotlib, we’re essentially adding interactive elements to our plots, enabling users to control various aspects of the visualization dynamically.
Let’s start with a simple example of creating multiple buttons in Matplotlib:
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
fig, ax = plt.subplots()
plt.subplots_adjust(bottom=0.2)
def button1_click(event):
print("Button 1 clicked - how2matplotlib.com")
def button2_click(event):
print("Button 2 clicked - how2matplotlib.com")
ax_button1 = plt.axes([0.2, 0.05, 0.1, 0.075])
ax_button2 = plt.axes([0.7, 0.05, 0.1, 0.075])
button1 = Button(ax_button1, 'Button 1')
button2 = Button(ax_button2, 'Button 2')
button1.on_clicked(button1_click)
button2.on_clicked(button2_click)
plt.show()
Output:
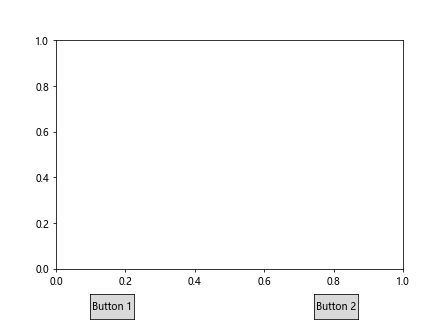
In this example, we create two buttons using the Button
class from matplotlib.widgets
. Each button is associated with a specific function that gets called when the button is clicked. This demonstrates the basic structure of creating multiple buttons in Matplotlib.
Advanced Techniques for Creating Multiple Buttons in Matplotlib
Now that we’ve covered the basics, let’s explore some advanced techniques for creating multiple buttons in Matplotlib. These techniques will allow you to create more complex and interactive visualizations.
Creating a Grid of Buttons
When creating multiple buttons in Matplotlib, you might want to arrange them in a grid layout. Here’s an example of how to achieve this:
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
import numpy as np
fig, ax = plt.subplots()
plt.subplots_adjust(bottom=0.3)
def button_click(label):
print(f"{label} clicked - how2matplotlib.com")
n_rows, n_cols = 2, 3
buttons = []
for i in range(n_rows):
for j in range(n_cols):
ax_button = plt.axes([0.1 + j*0.3, 0.05 + i*0.1, 0.1, 0.075])
label = f"Button {i*n_cols + j + 1}"
button = Button(ax_button, label)
button.on_clicked(lambda event, label=label: button_click(label))
buttons.append(button)
plt.show()
Output:
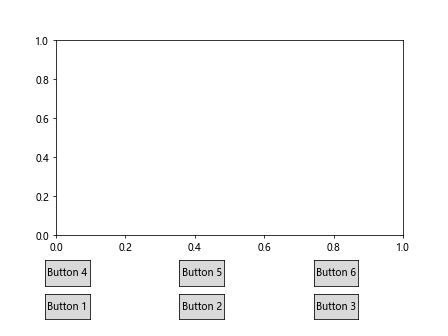
This example creates a 2×3 grid of buttons, demonstrating how to create multiple buttons in Matplotlib arranged in a structured layout.
Creating Toggle Buttons
Toggle buttons are a special type of button that can be in one of two states: on or off. Here’s how you can create multiple toggle buttons in Matplotlib:
import matplotlib.pyplot as plt
from matplotlib.widgets import CheckButtons
fig, ax = plt.subplots()
plt.subplots_adjust(left=0.2)
x = range(10)
lines = [plt.plot(x, [i*x for x in range(10)])[0] for i in range(3)]
def toggle_visibility(label):
index = labels.index(label)
lines[index].set_visible(not lines[index].get_visible())
plt.draw()
labels = ['Line 1 - how2matplotlib.com', 'Line 2 - how2matplotlib.com', 'Line 3 - how2matplotlib.com']
visibility = [True, True, True]
ax_check = plt.axes([0.05, 0.4, 0.1, 0.15])
check_buttons = CheckButtons(ax_check, labels, visibility)
check_buttons.on_clicked(toggle_visibility)
plt.show()
Output:
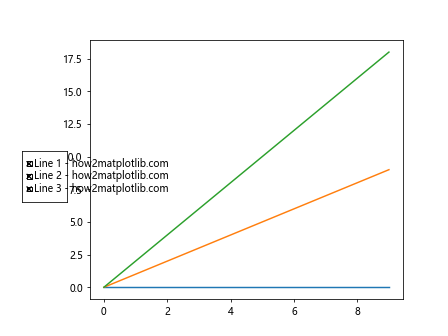
This example demonstrates how to create multiple toggle buttons in Matplotlib to control the visibility of different plot lines.
Customizing Button Appearance when Creating Multiple Buttons in Matplotlib
When creating multiple buttons in Matplotlib, you might want to customize their appearance to match your visualization’s style or to make them more visually appealing. Let’s explore some ways to achieve this.
Changing Button Colors
You can change the colors of your buttons when creating multiple buttons in Matplotlib. Here’s an example:
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
fig, ax = plt.subplots()
plt.subplots_adjust(bottom=0.2)
def button_click(label):
print(f"{label} clicked - how2matplotlib.com")
colors = ['red', 'green', 'blue', 'yellow']
buttons = []
for i, color in enumerate(colors):
ax_button = plt.axes([0.1 + i*0.2, 0.05, 0.1, 0.075])
label = f"Button {i+1}"
button = Button(ax_button, label, color=color, hovercolor='0.975')
button.on_clicked(lambda event, label=label: button_click(label))
buttons.append(button)
plt.show()
Output:
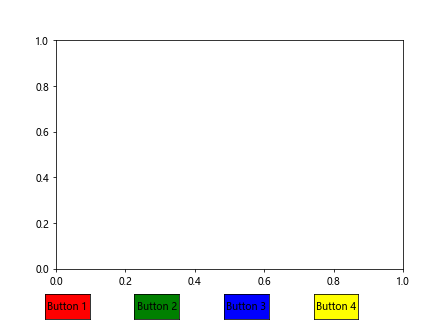
This example creates multiple buttons in Matplotlib with different colors, demonstrating how to customize the appearance of each button individually.
Adding Icons to Buttons
To make your buttons more intuitive, you might want to add icons when creating multiple buttons in Matplotlib. Here’s how you can do that:
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
import numpy as np
fig, ax = plt.subplots()
plt.subplots_adjust(bottom=0.2)
def button_click(label):
print(f"{label} clicked - how2matplotlib.com")
icons = ['→', '←', '↑', '↓']
buttons = []
for i, icon in enumerate(icons):
ax_button = plt.axes([0.1 + i*0.2, 0.05, 0.1, 0.075])
label = f"Button {i+1}"
button = Button(ax_button, icon)
button.label.set_fontsize(20)
button.on_clicked(lambda event, label=label: button_click(label))
buttons.append(button)
plt.show()
Output:
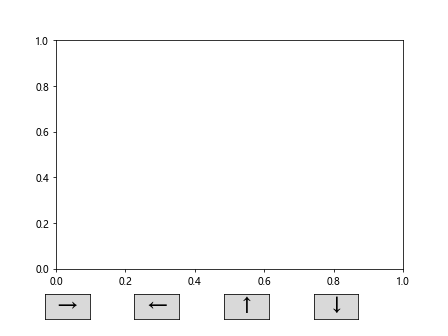
This example demonstrates how to create multiple buttons in Matplotlib with custom icons, enhancing the visual appeal and intuitiveness of your buttons.
Implementing Button Functionality when Creating Multiple Buttons in Matplotlib
Creating multiple buttons in Matplotlib is not just about appearance; it’s also about functionality. Let’s explore how to implement various functionalities for your buttons.
Updating Plot Data
One common use case when creating multiple buttons in Matplotlib is to update the plot data dynamically. Here’s an example:
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
import numpy as np
fig, ax = plt.subplots()
plt.subplots_adjust(bottom=0.2)
x = np.linspace(0, 10, 100)
line, = ax.plot(x, np.sin(x))
def update_sine(event):
line.set_ydata(np.sin(x))
fig.canvas.draw()
def update_cosine(event):
line.set_ydata(np.cos(x))
fig.canvas.draw()
ax_sine = plt.axes([0.2, 0.05, 0.1, 0.075])
ax_cosine = plt.axes([0.7, 0.05, 0.1, 0.075])
btn_sine = Button(ax_sine, 'Sine - how2matplotlib.com')
btn_cosine = Button(ax_cosine, 'Cosine - how2matplotlib.com')
btn_sine.on_clicked(update_sine)
btn_cosine.on_clicked(update_cosine)
plt.show()
Output:
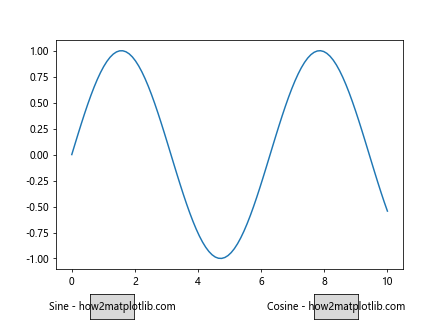
This example shows how to create multiple buttons in Matplotlib that update the plot data between sine and cosine functions when clicked.
Zooming and Panning
Another useful functionality when creating multiple buttons in Matplotlib is to implement zooming and panning controls. Here’s how you can do that:
import matplotlib.pyplot as plt
from matplotlib.widgets import Button
import numpy as np
fig, ax = plt.subplots()
plt.subplots_adjust(bottom=0.2)
x = np.linspace(0, 10, 100)
line, = ax.plot(x, np.sin(x))
def zoom_in(event):
ax.set_xlim(ax.get_xlim()[0] * 1.5, ax.get_xlim()[1] * 0.5)
fig.canvas.draw()
def zoom_out(event):
ax.set_xlim(ax.get_xlim()[0] * 0.5, ax.get_xlim()[1] * 1.5)
fig.canvas.draw()
def pan_left(event):
xlim = ax.get_xlim()
ax.set_xlim(xlim[0] - 1, xlim[1] - 1)
fig.canvas.draw()
def pan_right(event):
xlim = ax.get_xlim()
ax.set_xlim(xlim[0] + 1, xlim[1] + 1)
fig.canvas.draw()
buttons = [
('Zoom In - how2matplotlib.com', zoom_in, [0.15, 0.05, 0.1, 0.075]),
('Zoom Out - how2matplotlib.com', zoom_out, [0.35, 0.05, 0.1, 0.075]),
('Pan Left - how2matplotlib.com', pan_left, [0.55, 0.05, 0.1, 0.075]),
('Pan Right - how2matplotlib.com', pan_right, [0.75, 0.05, 0.1, 0.075])
]
for label, func, pos in buttons:
ax_button = plt.axes(pos)
button = Button(ax_button, label)
button.on_clicked(func)
plt.show()
Output:
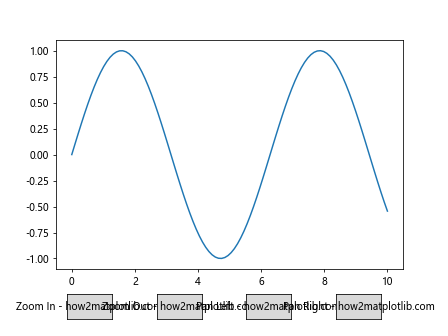
This example demonstrates how to create multiple buttons in Matplotlib that allow users to zoom in, zoom out, and pan the plot.
Best Practices for Creating Multiple Buttons in Matplotlib
When creating multiple buttons in Matplotlib, it’s important to follow some best practices to ensure your visualizations are user-friendly and efficient. Here are some tips to keep in mind:
- Consistent Layout: When creating multiple buttons in Matplotlib, maintain a consistent layout for your buttons. This makes your interface more intuitive and easier to use.
Clear Labels: Use clear and concise labels for your buttons. This helps users understand the function of each button at a glance.
Appropriate Sizing: Make sure your buttons are large enough to be easily clickable, but not so large that they dominate the plot area.
Color Coding: Use color coding wisely when creating multiple buttons in Matplotlib. Colors can help differentiate between button types or functions.
Responsive Design: Ensure your buttons work well with different figure sizes and screen resolutions.
Let’s implement these best practices in an example: